Overcoming SQL BLOB Size Limitations in Java Applications
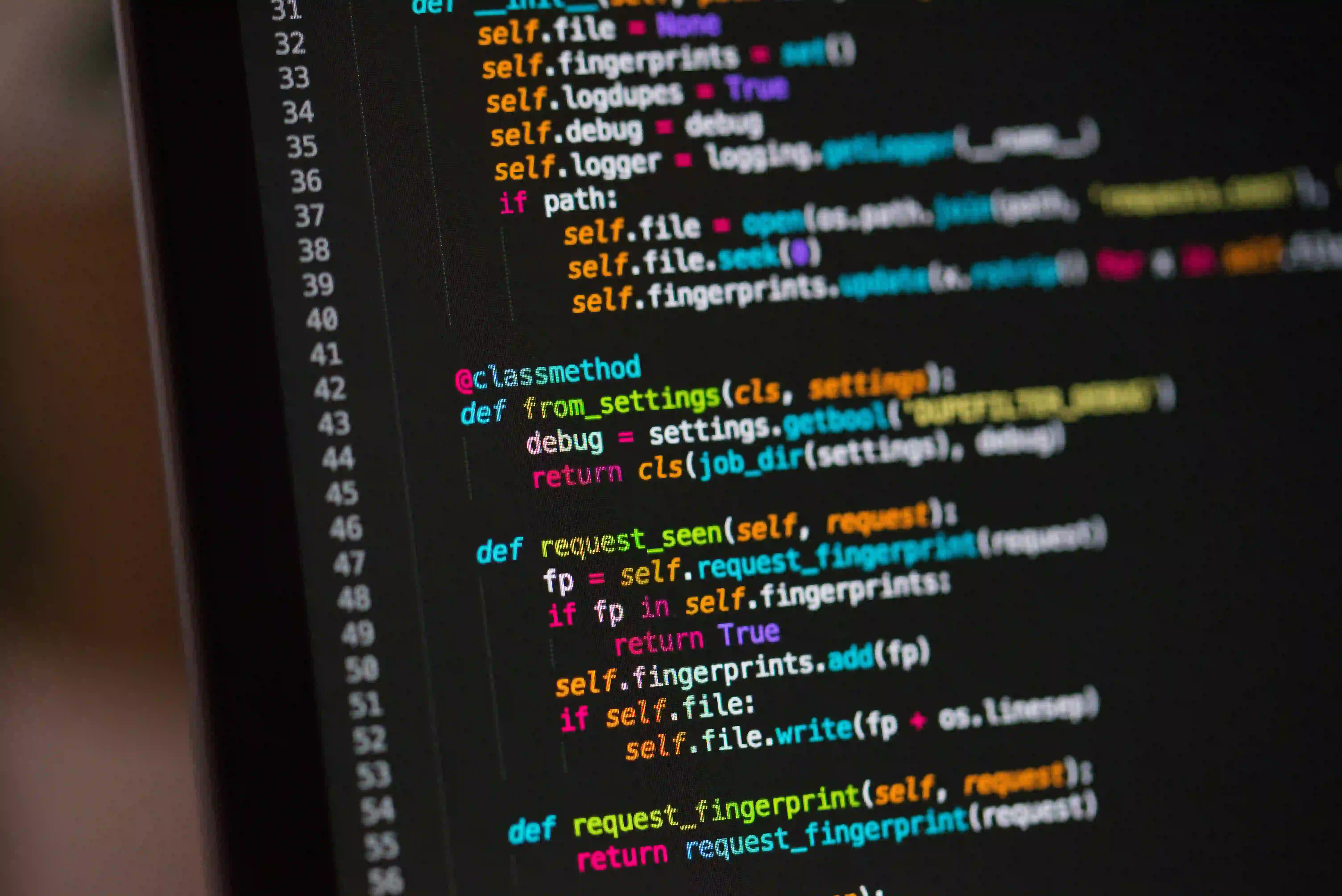
Overcoming SQL BLOB Size Limitations in Java Applications
When working with databases, it's common to encounter situations where you need to store large binary objects (BLOBs), such as images, videos, or other types of multimedia data. However, most databases impose size limitations on these BLOBs. In this blog post, we'll explore these limitations and, more importantly, how to overcome them in your Java applications.
Understanding BLOBs and Their Limitations
BLOB stands for Binary Large Object. It's a data type that can store a large amount of binary data, often exceeding the limits of traditional data types. Different databases have different size limitations for BLOBs. For example:
- MySQL can store BLOBs up to 65,535 bytes for TINYBLOB and up to 4GB for LONGBLOB.
- PostgreSQL allows BLOBs up to 1GB using the BYTEA data type or unlimited using Large Objects.
- Oracle Database supports up to 4GB with the BLOB data type.
As you can see, different systems may enforce different limits, and thus developers need to be cognizant of these restrictions when designing their schema and application logic.
Common Strategies for Handling BLOBs in Java
Java provides several approaches to handle BLOBs effectively. Let's dive into a few common strategies:
1. Use Streaming to Handle Large BLOBs
A prevalent approach to deal with large BLOBs in Java is to use streaming. Reading and writing BLOB data in chunks prevents out-of-memory errors and enhances performance. Here's how to retrieve a BLOB using JDBC streaming:
import java.sql.*;
import java.io.*;
public class BlobStreamingExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/your_database";
String user = "your_username";
String password = "your_password";
String sql = "SELECT blob_column FROM your_table WHERE id = ?";
try (Connection connection = DriverManager.getConnection(url, user, password);
PreparedStatement statement = connection.prepareStatement(sql)) {
statement.setInt(1, 1); // Replace with the appropriate ID
ResultSet resultSet = statement.executeQuery();
if (resultSet.next()) {
Blob blob = resultSet.getBlob("blob_column");
try (InputStream inputStream = blob.getBinaryStream();
FileOutputStream outputStream = new FileOutputStream("output_file")) {
byte[] buffer = new byte[4096];
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
}
}
} catch (SQLException | IOException e) {
e.printStackTrace();
}
}
}
Why Streaming?
- Memory Efficient: Prevents loading large datasets into memory entirely, reducing the risk of
OutOfMemoryError
. - Performance: Reading in chunks allows your application to handle large data loads more effectively.
2. Use File System to Store BLOBs
Instead of storing large BLOBs directly in the database, an alternative is to store the files on the server's file system and save their paths in the database. This reduces database size and improves performance while ensuring fast access to BLOBs.
Here’s an example of how you could create a simple mechanism to store and retrieve file paths in Java:
import java.sql.*;
public class FileSystemStorageExample {
public static void main(String[] args) {
String url = "jdbc:postgresql://localhost:5432/your_database";
String user = "your_username";
String password = "your_password";
String filePath = "path/to/your/file.jpg"; // Replace with your file path
// Store file path in DB
storeFilePath(filePath, url, user, password);
// Retrieve file path from DB
String retrievedPath = retrieveFilePath(url, user, password, 1); // Assuming ID 1
System.out.println("Retrieved Path: " + retrievedPath);
}
private static void storeFilePath(String filePath, String url, String user, String password) {
String sql = "INSERT INTO your_table (file_path) VALUES (?)";
try (Connection connection = DriverManager.getConnection(url, user, password);
PreparedStatement statement = connection.prepareStatement(sql)) {
statement.setString(1, filePath);
statement.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
private static String retrieveFilePath(String url, String user, String password, int id) {
String sql = "SELECT file_path FROM your_table WHERE id = ?";
String filePath = null;
try (Connection connection = DriverManager.getConnection(url, user, password);
PreparedStatement statement = connection.prepareStatement(sql)) {
statement.setInt(1, id);
ResultSet resultSet = statement.executeQuery();
if (resultSet.next()) {
filePath = resultSet.getString("file_path");
}
} catch (SQLException e) {
e.printStackTrace();
}
return filePath;
}
}
Why Use the File System?
- Scalability: Well-suited for applications where BLOB sizes can grow excessively; large files stored externally won’t bloat the database size.
- Backup and Recovery: Easier to manage, as file systems may offer better options for backing up large files than databases.
3. Use a BLOB Storage Service
Cloud providers such as Amazon S3 or Google Cloud Storage offer BLOB storage services designed specifically for handling large binary files. This method is particularly useful for web applications or when working with a distributed architecture.
Here's a conceptual example that illustrates how you might use AWS S3 to upload files.
import com.amazonaws.auth.AWSStaticCredentialsProvider;
import com.amazonaws.auth.BasicAWSCredentials;
import com.amazonaws.services.s3.AmazonS3;
import com.amazonaws.services.s3.AmazonS3ClientBuilder;
import com.amazonaws.services.s3.model.PutObjectRequest;
import java.io.File;
public class S3UploadExample {
public static void uploadFileToS3(String bucketName, String keyName, String filePath) {
BasicAWSCredentials awsCreds = new BasicAWSCredentials("your_access_key", "your_secret_key");
AmazonS3 s3client = AmazonS3ClientBuilder.standard()
.withRegion("us-west-2") // Specify your region
.withCredentials(new AWSStaticCredentialsProvider(awsCreds))
.build();
File file = new File(filePath);
s3client.putObject(new PutObjectRequest(bucketName, keyName, file));
System.out.println("Upload Completed.");
}
public static void main(String[] args) {
uploadFileToS3("your-bucket-name", "your/key/name.jpg", "path/to/local/file.jpg");
}
}
Why Use BLOB Storage Services?
- Dedication to File Storage: These services are optimized for file storage, compared to traditional databases.
- Cost-Effective: You may find it cheaper to store large amounts of data in specialized services rather than in your database.
Best Practices
When handling BLOBs in your Java applications, consider these best practices:
-
Read/Write in Bytes: Avoid loading entire objects into memory. Always use streams for reading/writing BLOBs.
-
Properly Manage Connections: Always close your database connections, statement objects, and result sets to prevent resource leaks.
-
Consider Database Alternatives: If your application relies heavily on BLOBs, consider using databases specifically designed to handle large binary data.
-
Evaluate Your Data Model: Regularly review your requirements and adapt your data model accordingly. Sometimes, keeping file paths instead of binary data simplifies queries and improves performance.
Final Thoughts
Overcoming SQL BLOB size limitations in Java applications is a significant challenge. However, adopting the right strategies can help you efficiently handle large binary data.
By using streaming techniques, leveraging the file system, or even using specialized storage solutions like AWS S3, you can avoid common pitfalls associated with large data storage.
For further reading on database handling in Java, check out the following resources:
With these tools and strategies, you're equipped to handle BLOBs effectively across your applications!