Common Coding Style Mistakes and How to Fix Them
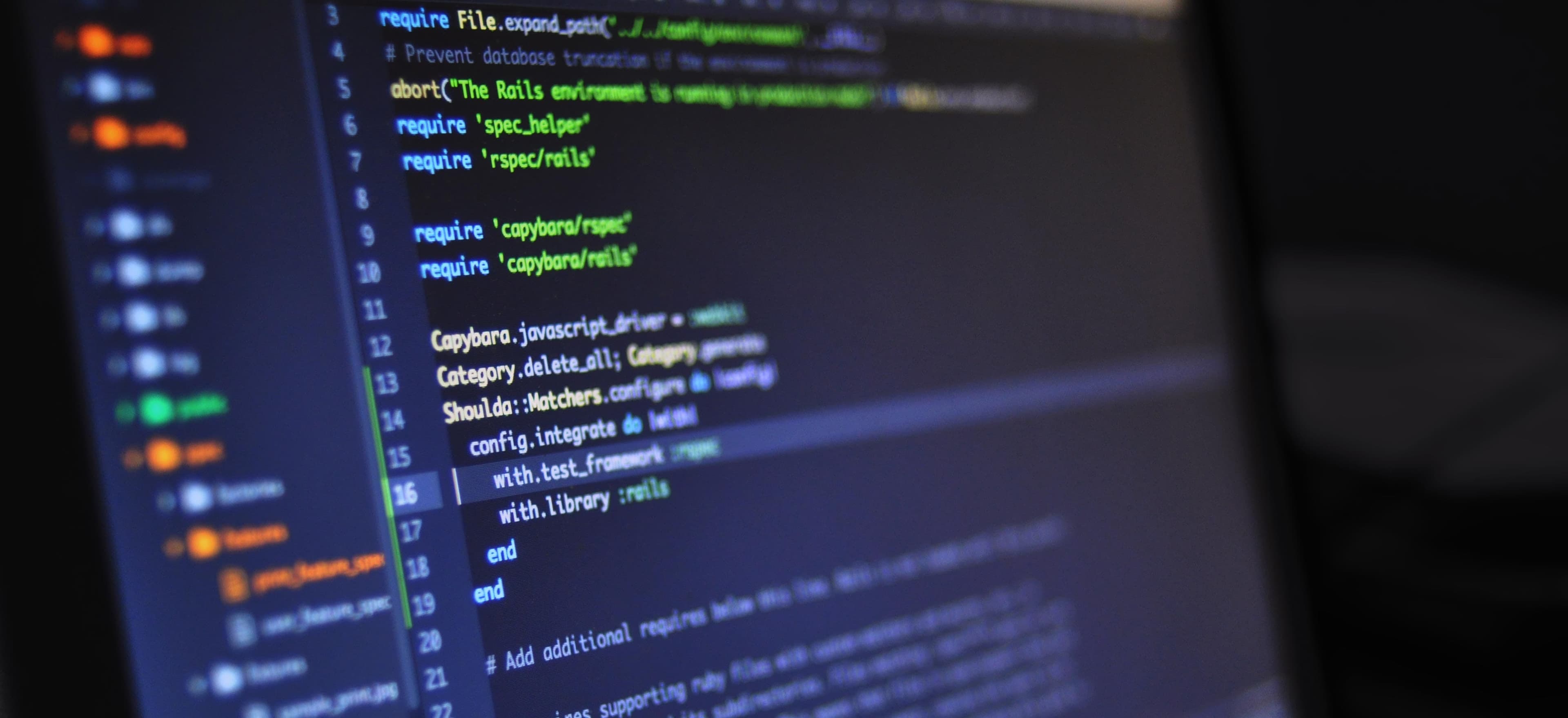
- Published on
Common Coding Style Mistakes and How to Fix Them in Java
Java is a powerful and widely-used programming language. However, writing clean, maintainable, and efficient code in Java can sometimes lead developers astray. One of the chief culprits is poor coding style. In this blog post, we'll explore some common coding style mistakes in Java and provide you with actionable strategies to fix them.
The Importance of Coding Style
Before we dive into the mistakes, let's understand why coding style matters. A consistent coding style:
-
Improves Readability: Code should be easy to read and understand, whether you're the original author or someone new to the codebase.
-
Facilitates Collaboration: In team environments, adhering to a common style fosters teamwork, allowing different developers to easily read and modify each other's code.
-
Prevents Bugs: Poorly written code is not only hard to read but also prone to mistakes or bugs due to misunderstandings.
-
Speeds Up Development: Clean code minimizes the time spent on debugging and clarifies intent, making future code modifications easier.
With that in mind, let’s dive into some common mistakes and how to rectify them.
Mistake 1: Inconsistent Naming Conventions
The Problem
In Java, naming conventions are essential for clarity. Not adhering to these conventions can lead to confusion.
For example:
int myVariable;
int Myvariable; // Inconsistent naming
The Fix
Use consistent naming conventions. Use camelCase for variables and methods:
int myVariable;
int mySecondVariable; // Clear and consistent
For class names, opt for PascalCase:
public class MyClass { }
Adopting a naming convention like Google's Java Style Guide can help maintain consistency across your projects.
Mistake 2: Poor Indentation and Spacing
The Problem
Proper indentation and spacing enhance readability. Inconsistent formatting might look like this:
public class Example {
public void method() {
if(true){System.out.println("Hello");}
}
}
The Fix
Use a standard indentation style (usually four spaces are recommended) and place your braces correctly:
public class Example {
public void method() {
if (true) {
System.out.println("Hello");
}
}
}
Make use of IDEs (Integrated Development Environments) like Eclipse or IntelliJ IDEA that can automatically format code for you.
Mistake 3: Overusing Comments
The Problem
Comments can aid understanding, but excessive commenting or poorly written comments can clutter the code. For instance:
// This function adds two numbers
int add(int a, int b) {
return a + b; // returning the sum of a and b
}
The Fix
Comment only when necessary. Your code should be self-explanatory. However, when a complex block of code exists, clarify its purpose:
/**
* Adds two integers and returns the result.
* @param a First integer to add.
* @param b Second integer to add.
* @return The sum of a and b.
*/
int add(int a, int b) {
return a + b;
}
Code Quality Tools
Consider using tools like SonarLint or Checkstyle, which can help you maintain a clean codebase.
Mistake 4: Forgetting to Use Access Modifiers
The Problem
Failing to use access modifiers can lead to unauthorized access to class members, making your code less secure. Without clarity, this can happen:
public class User {
String name; // No access modifier, default is package-private
}
The Fix
Always define access modifiers overtly. For example, if you want name
to be private, do so:
public class User {
private String name;
// Public method to access the private variable
public String getName() {
return name;
}
}
This fosters encapsulation, a core principle of object-oriented programming (OOP).
Mistake 5: Using Magic Numbers
The Problem
Using magic numbers can lead to confusion. For instance:
double circumference = 2 * 3.14 * radius; // What does 3.14 represent?
The Fix
Replace magic numbers with named constants. This enhances clarity significantly:
public static final double PI = Math.PI;
double circumference = 2 * PI * radius; // Clearer code
Using constants avoids confusion and aids future developers in understanding your intentions.
Mistake 6: Writing Long Methods
The Problem
Long methods can quickly become unmanageable, leading to decreased readability. Consider:
public void calculateAndDisplay() {
// Multiple lines of calculation and display logic
}
The Fix
Break down long methods into smaller, more manageable methods. Each method should perform a distinct task:
public void calculate() {
// Calculation logic
}
public void display() {
// Display logic
}
This modular approach simplifies troubleshooting and improves code maintenance.
The Closing Argument
Avoiding these common coding style mistakes will enhance your coding practices, foster greater collaboration, and produce cleaner code for current and future projects. Remember, adopting best practices is a journey. Regularly revisiting your work and learning from seasoned professionals will only strengthen your skills as a Java developer.
For those looking to dive deeper, check out additional resources like Effective Java by Joshua Bloch which provides comprehensive advice on best practices for Java coding.
Happy coding!