Mastering Data Structures: Common Pitfalls to Avoid
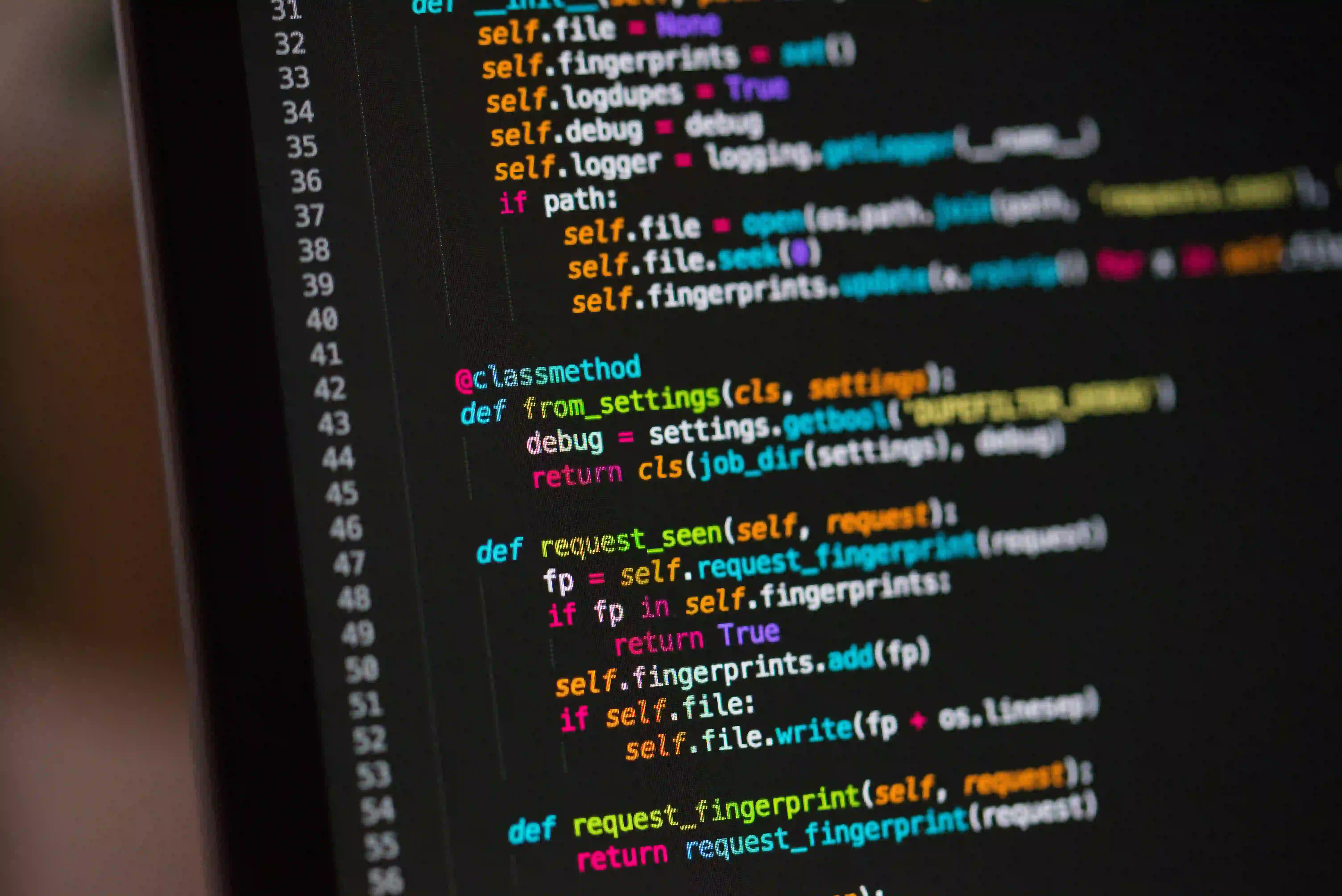
Mastering Data Structures: Common Pitfalls to Avoid
Data structures are fundamental to programming, especially in languages like Java. They are the backbone of efficient algorithms and optimal performance. However, while working with data structures, developers often encounter common pitfalls that can lead to inefficiencies and bugs.
In this blog post, we will explore these pitfalls, providing insights on how to avoid them. We will focus on fundamental data structures like arrays, linked lists, stacks, queues, trees, and hash tables, offering practical code snippets along the way.
Understanding Data Structures
Before jumping into pitfalls, let's briefly discuss the importance of data structures. A data structure is a way of organizing and storing data so that it can be accessed and modified efficiently. The choice of data structure can significantly impact the performance of your application.
Why Java?
Java is an object-oriented programming language that provides a rich set of built-in data structures in the Java Collections Framework. This makes it an excellent choice for implementing various data structures efficiently.
By mastering data structures, you can improve your code's speed and efficiency, making it robust and scalable.
Common Pitfalls in Data Structures
1. Inappropriate Data Structure Choice
One of the most common pitfalls is choosing the wrong data structure for the task at hand. Each data structure has its strengths and weaknesses, and using the wrong one can lead to suboptimal performance.
Example: Let's say you need to frequently insert and delete elements. Using an array to store these elements would be inefficient because adding or removing elements requires shifting elements, leading to O(n) complexity.
Better Choice: Instead, consider using a LinkedList where you can perform insertions and deletions in O(1) time.
import java.util.LinkedList;
public class LinkedListExample {
public static void main(String[] args) {
LinkedList<Integer> list = new LinkedList<>();
list.add(1); // O(1)
list.add(2); // O(1)
// Inserting 3 at index 1
list.add(1, 3); // O(1) insertion
System.out.println(list); // Output: [1, 3, 2]
// Removing an element
list.remove(1); // O(1) deletion
System.out.println(list); // Output: [1, 2]
}
}
2. Ignoring Edge Cases
When implementing data structures, it is crucial to account for edge cases. Neglecting these scenarios can lead to bugs and crashes.
Example: Consider a stack operation. If you attempt to pop an element from an empty stack, it should not just execute without checks.
Correct Approach: Implement checks to handle such cases.
import java.util.Stack;
public class StackExample {
public static void main(String[] args) {
Stack<Integer> stack = new Stack<>();
// Attempting to pop from an empty stack
try {
if (stack.isEmpty()) {
throw new IllegalStateException("Cannot pop from an empty stack");
}
stack.pop();
} catch (IllegalStateException e) {
System.out.println(e.getMessage()); // Output: Cannot pop from an empty stack
}
}
}
3. Failing to Optimize Memory Usage
Inefficient memory usage can slow down an application. For example, an array is of fixed size. If an array is too large, it wastes memory; if it’s too small, it will need to be resized, which is costly.
Solution: Utilize dynamic data structures such as ArrayList which can resize automatically.
import java.util.ArrayList;
public class ArrayListExample {
public static void main(String[] args) {
ArrayList<Integer> arrayList = new ArrayList<>();
arrayList.add(1);
arrayList.add(2);
System.out.println(arrayList); // Output: [1, 2]
// Adding more elements
for (int i = 3; i <= 10; i++) {
arrayList.add(i);
}
System.out.println(arrayList); // Output: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
}
}
4. Overlooking Thread Safety
In multi-threaded environments, data structures can lead to race conditions if not handled properly. It is vital to ensure thread safety.
Solution: Use concurrent data structures from the java.util.concurrent
package or wrap them using synchronized
.
import java.util.concurrent.ConcurrentHashMap;
public class ConcurrentHashMapExample {
public static void main(String[] args) {
ConcurrentHashMap<String, String> map = new ConcurrentHashMap<>();
map.put("key1", "value1");
// A thread-safe operation
map.compute("key1", (key, value) -> value + " updated");
System.out.println(map.get("key1")); // Output: value1 updated
}
}
5. Neglecting Complexity Analysis
Understand the time and space complexities associated with the data structures you implement. Many developers overlook this, leading them to write inefficient code.
Example: If you need to check the existence of an element frequently, using a HashSet is optimal due to its O(1) average time complexity for operations.
import java.util.HashSet;
public class HashSetExample {
public static void main(String[] args) {
HashSet<Integer> hashSet = new HashSet<>();
hashSet.add(1);
hashSet.add(2);
// Check presence of element
boolean exists = hashSet.contains(2); // O(1) operation
System.out.println("Does HashSet contain 2? " + exists); // Output: true
}
}
Wrapping Up
Mastering data structures in Java is not only about implementing them correctly but also understanding the common pitfalls associated with their usage. By keeping these insights in mind, developers can enhance their coding practices, ensuring better performance, reliability, and maintainability of their applications.
For further reading on data structures, consider checking out resources such as GeeksforGeeks, and Java Documentation.
By paying attention to appropriate data structure selection, handling edge cases, optimizing memory usage, ensuring thread safety, and performing complexity analysis, any developer can harness the full potential of data structures in Java. Happy coding!