Understanding the Pitfalls of Accumulator Classes in Code
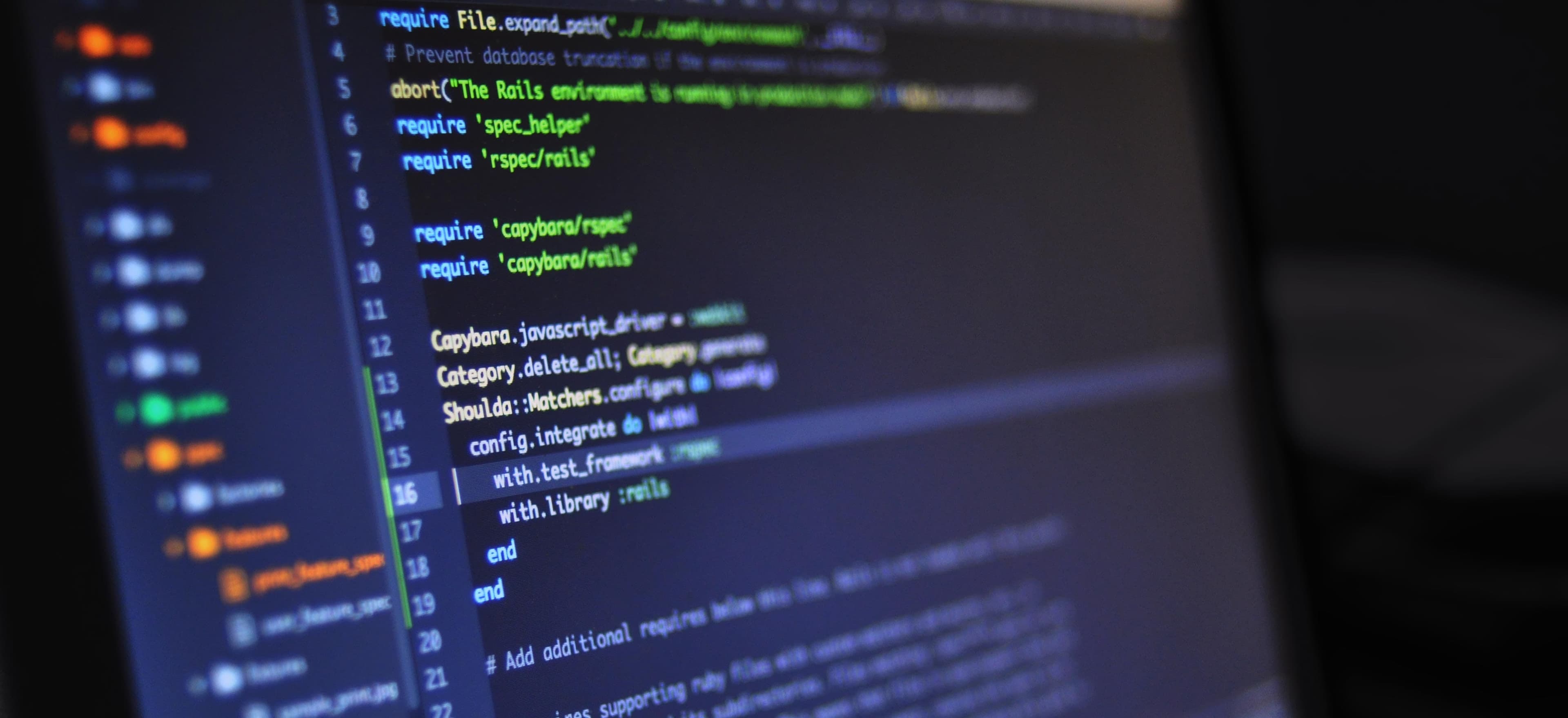
- Published on
Understanding the Pitfalls of Accumulator Classes in Java
In the world of programming, especially in object-oriented languages like Java, we often come across the concept of accumulator classes. An accumulator class is designed to gather or accumulate data and may often include methods to manipulate or summarize that data. While the design pattern appears straightforward and useful, it can introduce pitfalls that developers must be wary of. In this blog post, we will explore these pitfalls and how to avoid them while also providing clear examples along the way.
What is an Accumulator Class?
Simply put, an accumulator class is an object that gathers values over time. A classic use case might be a class that sums up numbers, collects statistics, or even stores logs. Here is a simple example of an accumulator class in Java:
public class Accumulator {
private int total;
public Accumulator() {
this.total = 0;
}
public void add(int number) {
total += number;
}
public int getTotal() {
return total;
}
}
In this example, the Accumulator
class has a private integer total
that accumulates the values passed to its add
method. While this is a straightforward implementation, there are several pitfalls that developers should be aware of.
Pitfalls of Accumulator Classes
1. State Management
One of the main issues with accumulator classes arises from the management of their internal state. Because these classes maintain state over their lifespan, they can lead to unexpected behavior, especially when instances are shared across different parts of your application.
For instance, consider the following scenario:
Accumulator accumulator1 = new Accumulator();
Accumulator accumulator2 = new Accumulator();
accumulator1.add(10);
accumulator2.add(20);
System.out.println(accumulator1.getTotal()); // Outputs 10
System.out.println(accumulator2.getTotal()); // Outputs 20
This behavior seems straightforward, but what if we accidentally pass a shared instance of an accumulator to multiple threads? This can lead to race conditions where concurrent modifications can corrupt the state, resulting in unreliable data.
2. Lack of Immutability
Accumulator classes are often mutable, meaning their state can change over time. This mutability means that they cannot be easily passed around in a safe manner, as their internal state might change unexpectedly.
Consider this version of the accumulator class with an immutability fix:
public class ImmutableAccumulator {
private final int total;
public ImmutableAccumulator(int total) {
this.total = total;
}
public ImmutableAccumulator add(int number) {
return new ImmutableAccumulator(this.total + number);
}
public int getTotal() {
return total;
}
}
Here, the ImmutableAccumulator
does not maintain state but instead returns a new instance every time the add
method is invoked. This approach eliminates concerns about unintended state changes but may incur performance overhead.
3. Single Responsibility Principle
Another common issue is that accumulator classes often take on multiple responsibilities, which can make them more complex than necessary. For example, an Accumulator
class might also implement logging or statistics calculations, violating the Single Responsibility Principle from SOLID design principles.
Instead of cluttering a single class with multiple responsibilities, it is better to break down your designs into smaller, single-purpose classes. This approach not only makes the class easier to understand and maintain but also adheres to good design practices.
public class Statistics {
private final int count;
private final int sum;
public Statistics(int count, int sum) {
this.count = count;
this.sum = sum;
}
public Statistics add(int number) {
return new Statistics(this.count + 1, this.sum + number);
}
public int getCount() {
return count;
}
public int getSum() {
return sum;
}
}
In this example, we have separated the responsibilities by creating a Statistics
class. Now, we can also create a Log
class that handles logging separately.
4. Testing Challenges
Testing mutable classes like accumulator classes can prove to be challenging. If your tests are dependent on the order of method calls or the current state of an object, they can lead to brittle tests that are difficult to maintain.
By using immutable designs, the state is encapsulated, and you can easily verify outputs based on input without worrying about side effects from other method calls:
public class AccumulatorTest {
public static void main(String[] args) {
ImmutableAccumulator acc1 = new ImmutableAccumulator(0);
ImmutableAccumulator acc2 = acc1.add(10).add(20);
System.out.println(acc1.getTotal()); // Outputs 0
System.out.println(acc2.getTotal()); // Outputs 30
}
}
In this test, you can see that the state for acc1
remains unchanged, ensuring clarity in the tests.
5. Performance Considerations
When designing your accumulator classes, performance can be a crucial aspect. When using immutable classes, each addition creates a new object, which can lead to overhead in both time and memory if not managed correctly. Utilizing StringBuilder
for concatenating strings or implementing a pool of reusable objects can help mitigate performance issues.
The Last Word
Accumulator classes can be powerful tools in your Java toolbox, but they come with their own set of pitfalls. Being aware of issues related to mutable state, violating design principles, and testing challenges can lead to better software design.
Consider immutable designs, adhere to the Single Responsibility Principle, and always strategize performance. Good design not only makes your code more efficient but also allows it to be easier to maintain, extend, and test.
For further reading about design patterns in Java, check out Java Design Patterns and explore best practices in writing clean code at Clean Code Java. By integrating these practices into your development workflow, you will create robust applications that stand the test of time.
Happy coding!