Debugging JPA Type Converters: Testing Tips with Arquillian
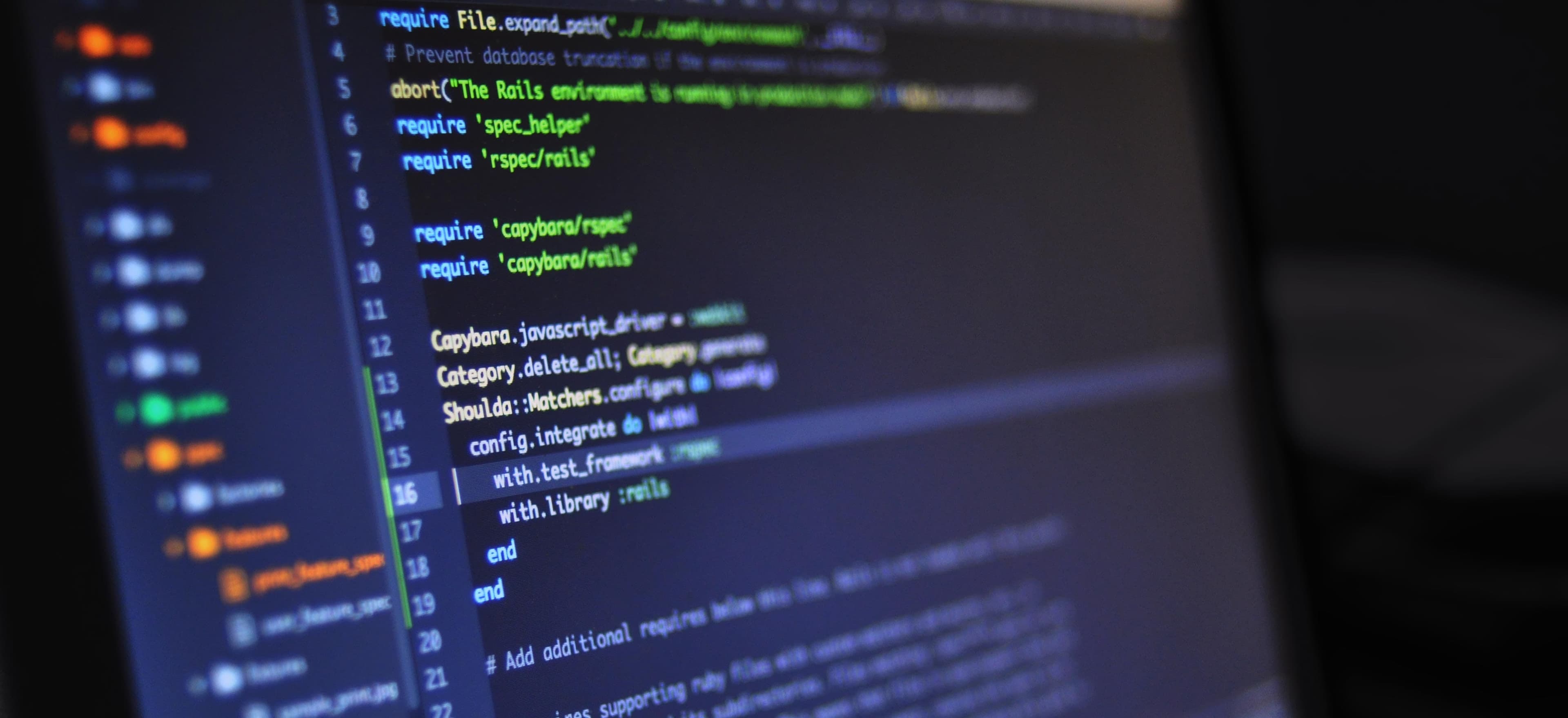
- Published on
Debugging JPA Type Converters: Testing Tips with Arquillian
Java Persistence API (JPA) is a powerful framework for data persistence in Java applications. One of its remarkable features is the ability to implement custom type converters. These converters allow you to handle types that are not natively supported by JPA out of the box. However, debugging issues related to these converters can be a tricky process. In this article, we will dive deep into the world of JPA type converters, uncover common pitfalls, and explore efficient testing practices using Arquillian.
What are JPA Type Converters?
Type converters in JPA allow developers to define how specific entity attributes should be persisted in the database. This is particularly useful when dealing with complex objects or unsupported data types. A basic implementation of a custom type converter might look like this:
import javax.persistence.Converter;
import javax.persistence.AttributeConverter;
import javax.persistence.Converter;
import java.util.Base64;
@Converter(autoApply = true)
public class StringToBase64Converter implements AttributeConverter<String, String> {
@Override
public String convertToDatabaseColumn(String attribute) {
if (attribute == null) {
return null;
}
// Convert the string to Base64
return Base64.getEncoder().encodeToString(attribute.getBytes());
}
@Override
public String convertToEntityAttribute(String dbData) {
if (dbData == null) {
return null;
}
// Convert back from Base64 to string
return new String(Base64.getDecoder().decode(dbData));
}
}
Why Use Type Converters?
Using type converters streamlines the codebase by abstracting complex data transformations away from the entity bean and encapsulating them into dedicated classes. This enhances code readability and maintainability, following the Single Responsibility Principle.
Debugging JPA Type Converters
While developing custom converters, you might encounter various issues such as:
- Incorrect Data Conversions: Data might not be converted properly between entity and database formats.
- Null Pointer Exceptions: These can occur if null values are not handled properly.
- Performance Issues: Inefficient conversions can lead to performance bottlenecks, especially in large datasets.
Strategies for Debugging Type Converters
Here are some tips to help you effectively debug and test your JPA type converters:
-
Log Inputs and Outputs: Always log the input attributes and the output values of your conversion methods. This will help you trace where the issue lies.
@Override public String convertToDatabaseColumn(String attribute) { // Log input System.out.println("Converting to DB: " + attribute); // Convert and log output String encoded = Base64.getEncoder().encodeToString(attribute.getBytes()); System.out.println("Converted: " + encoded); return encoded; }
-
Unit Testing: Thoroughly unit test your converter methods with various input cases, including edge cases like empty strings, null values, and very long strings.
-
Integration Testing with Arquillian: Arquillian allows you to run your tests in a container-managed environment. This is particularly useful for JPA tests involving persistence context.
Setting Up Arquillian
To start using Arquillian for your testing needs, you need to set it up in your project. Ensure you have the necessary dependencies in your Maven or Gradle configuration:
Maven Dependencies
<dependency>
<groupId>org.jboss.arquillian.junit</groupId>
<artifactId>arquillian-junit-container</artifactId>
<version>1.7.0.Final</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.jboss.arquillian.container</groupId>
<artifactId>arquillian-wildfly-remote</artifactId>
<version>1.7.0.Final</version>
</dependency>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>5.4.22.Final</version>
</dependency>
<!-- Add more dependencies as necessary -->
Creating a Test Class with Arquillian
Once your environment is set up, you can write an integration test for the JPA type converter using Arquillian:
import org.jboss.arquillian.container.test.api.Deployment;
import org.jboss.arquillian.junit.Arquillian;
import org.junit.Test;
import org.junit.runner.RunWith;
import javax.persistence.EntityManager;
import javax.persistence.PersistenceContext;
import static org.junit.Assert.assertEquals;
@RunWith(Arquillian.class)
public class StringToBase64ConverterTest {
@PersistenceContext
private EntityManager entityManager;
@Deployment
public static JavaArchive createDeployment() {
return ShrinkWrap.create(JavaArchive.class)
.addClasses(StringToBase64Converter.class, Entity.class) // Add your entity here
.addAsManifestResource(EmptyAsset.INSTANCE, "beans.xml");
}
@Test
public void testStringToBase64Conversion() {
Entity entity = new Entity();
entity.setAttribute("hello");
entityManager.persist(entity);
String dbValue = entityManager.createQuery("SELECT e.attribute FROM Entity e", String.class).getSingleResult();
assertEquals("aGVsbG8=", dbValue); // This is the Base64 for "hello"
entityManager.remove(entity);
}
}
Breakdown of the Test Class
- @RunWith(Arquillian.class): This annotation tells JUnit to use Arquillian as the test runner.
- @PersistenceContext: This injects the EntityManager that interacts with the database.
- @Deployment: This method defines the deployment of your app, specifying the required classes and resources.
- @Test: Each method annotated with this is a test case. It validates your type converter functionality by persisting an entity and verifying the correct conversion.
Common Errors and How to Fix Them
While testing, you may encounter some common issues:
-
Transaction Management Errors: Ensure you handle transactions correctly. Annotate your test methods with
@Transactional
if needed or properly manage within the test setup. -
Entity Not Found: If you receive an error indicating that the entity could not be found, ensure that you correctly specify the entity state and manage the persistence context.
-
Encoding Issues: What you store in the database must match what you expect in your tests. Always verify encoding.
Bringing It All Together
Debugging JPA type converters is imperative to ensure that your applications function as expected. By utilizing strategies like logging, unit testing, and integration tests with Arquillian, you can diagnose and resolve issues efficiently. Remember to handle edge cases and potential exceptions to refine your converters further.
For further reading:
With these tools and practices at your disposal, you're on your way to mastering JPA type conversions in your Java applications. Happy coding!