Navigating JMX Bean Configuration for Multiple JCache Providers
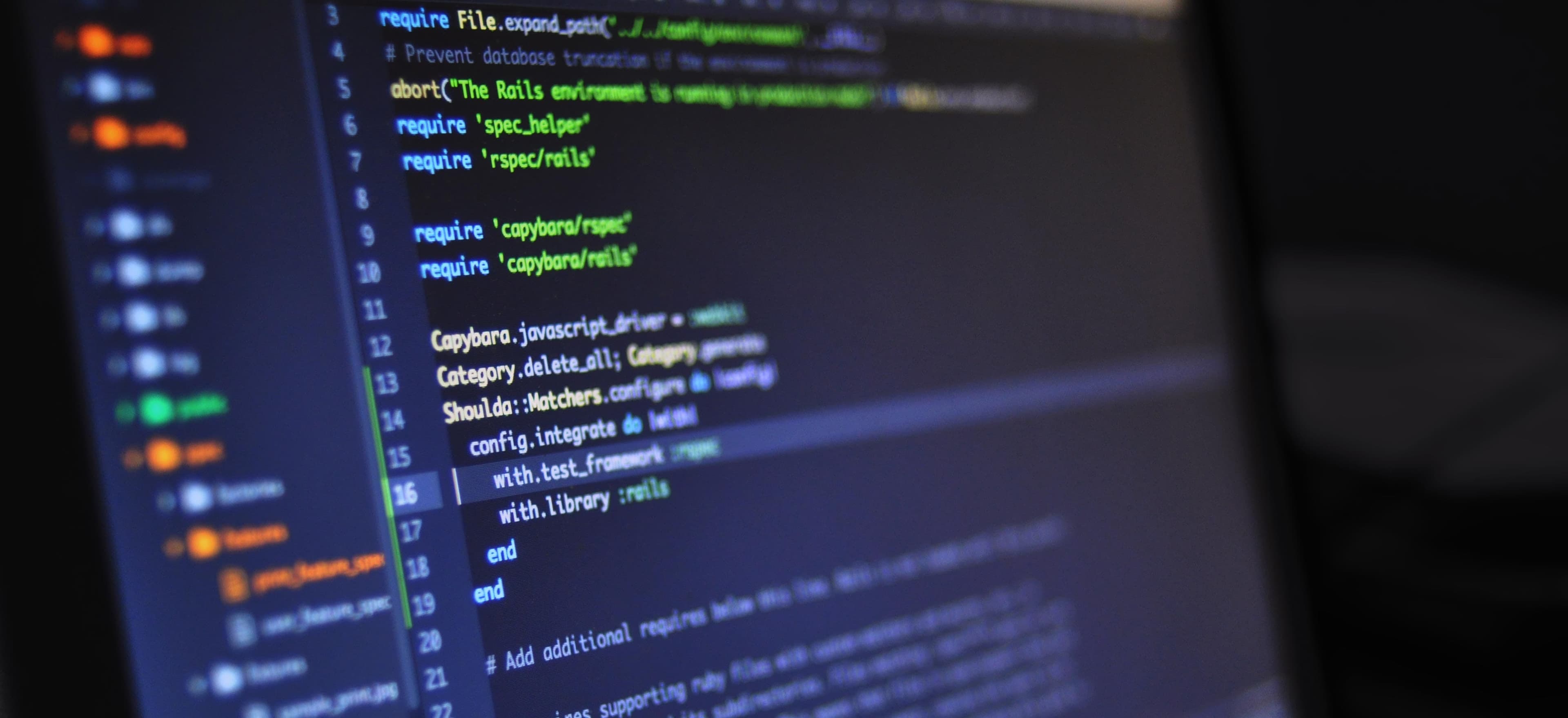
- Published on
Navigating JMX Bean Configuration for Multiple JCache Providers
Java Management Extensions (JMX) is a powerful framework for managing and monitoring Java applications. When working with JCache (JSR 107), which is the standard caching API for Java, configuring JMX Beans can be crucial for gaining insights into cache performance. This post will explore how to configure JMX for multiple JCache providers and leverage the power of JMX for efficient cache management.
What is JCache?
JCache is a standard caching API in Java, allowing developers to work with caches more intuitively. It provides a simple mechanism for caching objects, offering features such as expiry policies, eviction policies, and much more. Several caching providers implement this API, including Hazelcast, Ehcache, and Infinispan.
Why Use JMX with JCache?
Using JMX with JCache offers several benefits:
- Monitoring: Track performance metrics such as hit/miss ratios, cache size, and eviction rates.
- Management: Dynamically adjust cache configurations at runtime.
- Insights: Gain deeper visibility into cache behavior, enabling performance tuning.
However, when working with multiple JCache providers, the configuration can get complex. Below, we offer a comprehensive guide on setting up JMX for these providers effectively.
Step 1: Choose JCache Providers
Before you can start configuring JMX Beans, you need to decide on the JCache providers you want to use. Let’s take two widely-used providers as examples: Hazlecast and Ehcache.
Adding Dependencies
To use these caching providers, you will need to include their respective dependencies in your pom.xml
if you’re using Maven.
<dependency>
<groupId>com.hazelcast</groupId>
<artifactId>hazelcast</artifactId>
<version>5.0</version>
</dependency>
<dependency>
<groupId>org.ehcache</groupId>
<artifactId>ehcache</artifactId>
<version>3.10.5</version>
</dependency>
Step 2: Configure JMX for Each Provider
Hazelcast Configuration
First, let’s configure JMX for Hazelcast. You can enable JMX by setting the management.center.enabled
configuration to true
.
import com.hazelcast.config.Config;
import com.hazelcast.config.ManagementCenterConfig;
import com.hazelcast.core.Hazelcast;
import com.hazelcast.core.HazelcastInstance;
public class HazelcastJMXConfig {
public static void main(String[] args) {
Config config = new Config();
ManagementCenterConfig managementCenterConfig = new ManagementCenterConfig();
managementCenterConfig.setEnabled(true);
config.setManagementCenterConfig(managementCenterConfig);
HazelcastInstance hz = Hazelcast.newHazelcastInstance(config);
// ... other configurations and usage
}
}
Explanation
In the code above:
- We create a
Config
object which holds the configuration for your Hazelcast instance. - The
ManagementCenterConfig
class is used to enable JMX features. - Finally, we create a new instance of
HazelcastInstance
which will now be configured to expose its metrics via JMX.
Ehcache Configuration
Setting up JMX for Ehcache is just as straightforward. You can enable JMX by defining a CacheManager
as follows:
import org.ehcache.CacheManager;
import org.ehcache.config.CacheConfiguration;
import org.ehcache.config.builders.CacheManagerBuilder;
import org.ehcache.config.builders.ResourcePoolsBuilder;
public class EhcacheJMXConfig {
public static void main(String[] args) {
CacheConfiguration<Long, String> configuration =
org.ehcache.config.builders.CacheConfigurationBuilder.newCacheConfigurationBuilder(Long.class, String.class,
ResourcePoolsBuilder.heap(10))
.withSizeOfMaxObjectGraph(5)
.build();
CacheManager cacheManager = CacheManagerBuilder.newCacheManagerBuilder()
.withCache("sampleCache", configuration)
.using(new JMXConfiguration(true)) // Enabling JMX support
.build(true);
// ... other configurations and usage
}
}
Explanation
Here, we:
- Create a
CacheConfiguration
which specifies the properties of our cache. - Use
CacheManagerBuilder
to build aCacheManager
that holds our cache. - Enable JMX by using the
JMXConfiguration
class.
Step 3: Accessing JMX Beans
Once you have everything configured, you can access the JMX beans using tools such as JConsole or VisualVM. These tools allow you to view the metrics collected by JMX, inspect JCache statistics, and modify configurations at runtime.
Example Metrics to Monitor
When monitoring your JCache instances via JMX, you may find it helpful to track the following metrics:
- Cache Hit Ratio: How often requests are satisfied by the cache.
- Eviction Count: The number of entries removed from the cache.
- Cache Size: The number of entries currently held in the cache.
Custom JMX Beans
In some cases, the default JMX beans provided by the JCache implementations may not provide all the details you require. You can create custom JMX beans to expose additional properties or aggregated metrics.
Creating Custom MBeans
Here’s an example of how to create a simple custom MBean for monitoring the total cache hits.
import javax.management.MBeanServer;
import javax.management.ObjectName;
import java.lang.management.ManagementFactory;
public class CacheMonitor implements CacheMonitorMBean {
private long totalHits;
public void incrementHits() {
totalHits++;
}
public long getTotalHits() {
return totalHits;
}
public static void main(String[] args) throws Exception {
CacheMonitor monitor = new CacheMonitor();
MBeanServer mbs = ManagementFactory.getPlatformMBeanServer();
ObjectName name = new ObjectName("com.example:type=CacheMonitor");
mbs.registerMBean(monitor, name);
// Increment cache hits as needed
// monitor.incrementHits();
}
}
Explanation
In this code:
- We define a simple interface
CacheMonitorMBean
that will serve as our MBean interface. - The
CacheMonitor
class implements this interface and tracks cache hits. - We register it in the MBean server, allowing you to monitor cache hits dynamically.
To Wrap Things Up
Configuring JMX for multiple JCache providers can streamline how you monitor and manage your caching solutions. By enabling JMX, you gain access to powerful monitoring capabilities, allowing for more informed decision-making.
Remember, always review the documentation of your specific JCache provider for details about which metrics are available and how to configure them correctly. Tools like JConsole and VisualVM will be invaluable for observing your JMX Beans.
Adapting JMX configurations suited to your JCache provider's capabilities could significantly enhance your application's performance and maintainability. Happy caching!