Overcoming Common Pitfalls in Bridge Design Pattern
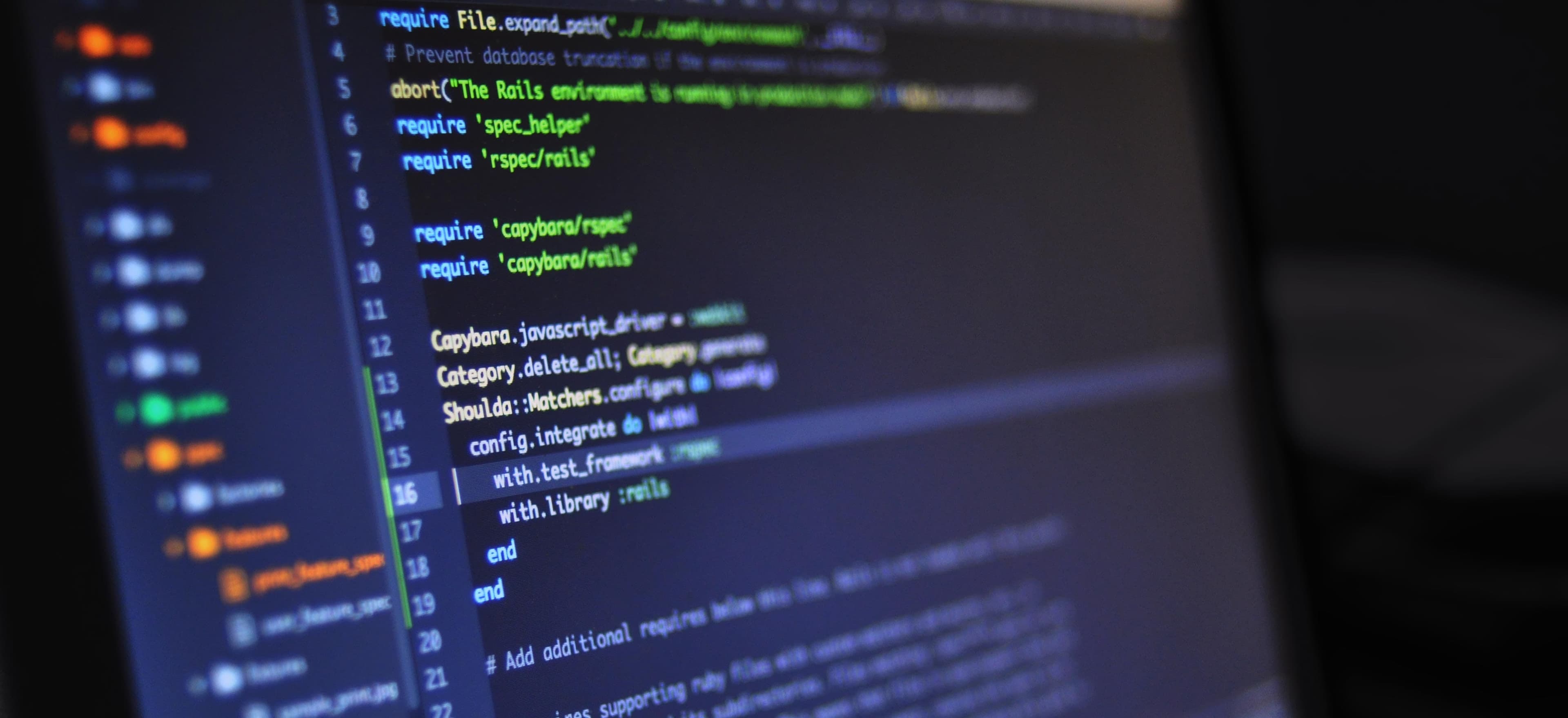
- Published on
Overcoming Common Pitfalls in Bridge Design Pattern
The Bridge Design Pattern is a structural design pattern that aims to separate an abstraction from its implementation, allowing both to evolve independently. In this blog post, we'll delve into the Bridge Design Pattern, explore common pitfalls that developers face when implementing it, and provide solutions to overcome these challenges. This insightful approach is crucial for improving your programming practices and enhancing the flexibility of your code.
Understanding the Bridge Design Pattern
Before discussing pitfalls, let’s briefly understand what the Bridge Design Pattern is and why it’s essential.
What is the Bridge Design Pattern?
The Bridge Pattern is classified under structural design patterns. It involves creating a bridge (or interface) between abstract classes and their implementations. By doing this, you prevent a permanent binding between the abstraction and its implementation, allowing them to evolve separately.
Use Case for Bridge Pattern
Consider a scenario where you have multiple devices (like TV
and Radio
) that can operate with different remote controls. In a naive implementation, you might have classes that tightly couple the device and the remote. However, with the Bridge Design Pattern, you can create a structure that allows for flexible changes in devices and remotes without affecting each other.
Basic Structure
Here's a conceptual implementation of the Bridge Design Pattern in Java:
// Abstraction
abstract class RemoteControl {
protected Device device;
protected RemoteControl(Device device) {
this.device = device;
}
public abstract void turnOn();
public abstract void turnOff();
}
// Implementor
interface Device {
void powerOn();
void powerOff();
}
// Concrete Implementor
class Television implements Device {
public void powerOn() {
System.out.println("Television is now ON.");
}
public void powerOff() {
System.out.println("Television is now OFF.");
}
}
// Another Concrete Implementor
class Radio implements Device {
public void powerOn() {
System.out.println("Radio is now ON.");
}
public void powerOff() {
System.out.println("Radio is now OFF.");
}
}
// Refined Abstraction
class AdvancedRemoteControl extends RemoteControl {
public AdvancedRemoteControl(Device device) {
super(device);
}
public void turnOn() {
device.powerOn();
}
public void turnOff() {
device.powerOff();
}
}
In this example, we have two main components: RemoteControl
and Device
. The abstraction class RemoteControl
does not depend on concrete classes but instead relies on the Device
interface. This flexibility allows easy changes in either the devices or the remotes without affecting the other.
Common Pitfalls in Bridge Design Pattern
While the Bridge Design Pattern is powerful, developers often encounter several common pitfalls:
1. Over-Complication of Interfaces
One of the most prevalent pitfalls is the over-complication of the interfaces. Developers may create unnecessary methods in the Device
interface that aren't universally applicable to all implementations.
Solution:
Keep the interface focused. Only include methods that are essential for all devices.
interface Device {
void powerOn();
void powerOff();
}
2. Poor Naming Conventions
Using vague names for your classes and methods can lead to confusion. For instance, naming a method execute
without context can make the codebase challenging to understand.
Solution:
Be descriptive in your naming conventions. Use clear and precise names that convey the intent of the classes and methods.
class RemoteControl {
public void turnOn() { /* Implementation */ }
public void turnOff() { /* Implementation */ }
}
3. Ignoring Implementation Variability
Another common pitfall is failing to recognize that implementations may vary significantly. Developers might create too many tightly-coupled classes instead of adequately leveraging the Bridge pattern to isolate variations.
Solution:
Identify the commonality and variability within your classes. Ensure that you design the classes in a way that honors these distinctions.
4. Creating Unnecessary Abstractions
While abstraction is fundamental to the Bridge pattern, you can fall into the trap of creating layers of abstraction that don't add real value, complicating your codebase.
Solution:
Evaluate each layer of abstraction to ensure it serves a purpose. Before introducing an abstraction, ask yourself if it genuinely benefits the software design.
5. Not Taking Full Advantage of the Pattern
Some developers might use the Bridge pattern without fully appreciating its potential. They might create abstractions that are too similar to the concrete implementations.
Solution:
Strive to leverage the benefits of the Bridge pattern by ensuring a clear separation between the abstraction and the implementation. This enhances maintainability and scalability.
Best Practices for Implementing Bridge Design Pattern
To further fortify your implementation of the Bridge Design Pattern, consider the following best practices:
1. Keep Interfaces Simple
Adhere to the Interface Segregation Principle. Ensure your interfaces are simple and focused on specific functionalities. This improves usability and promotes clean code practices.
2. Establish Clear Roles
Establish clear roles for your classes. Have distinct responsibilities for your abstracts, decorators, and implementations. This keeps the code organized and intuitive.
3. Use Concrete Classes Judiciously
Avoid overusing concrete classes. Instead, focus on building your abstraction effectively, thereby ensuring your implementation can evolve independently.
4. Document Your Code
The Bridge pattern can be complex at times. To ease understanding, document your code comprehensively. Explain the purpose, relationships, and behaviors of your classes.
My Closing Thoughts on the Matter
The Bridge Design Pattern is a valuable strategy for fostering the flexibility of code. By being mindful of common pitfalls and adhering to best practices, developers can leverage this pattern to create maintainable and scalable systems.
In today’s fast-paced software development world, understanding design patterns, including the Bridge Design Pattern, can enhance your toolkit and lead to better software design. For more on design patterns, consider visiting Refactoring Guru or SourceMaking.
Implement these insights into your everyday programming and witness the improvement in the structure and adaptability of your code. Happy coding!