Struggling with Code Complexity? Try AOP Today!
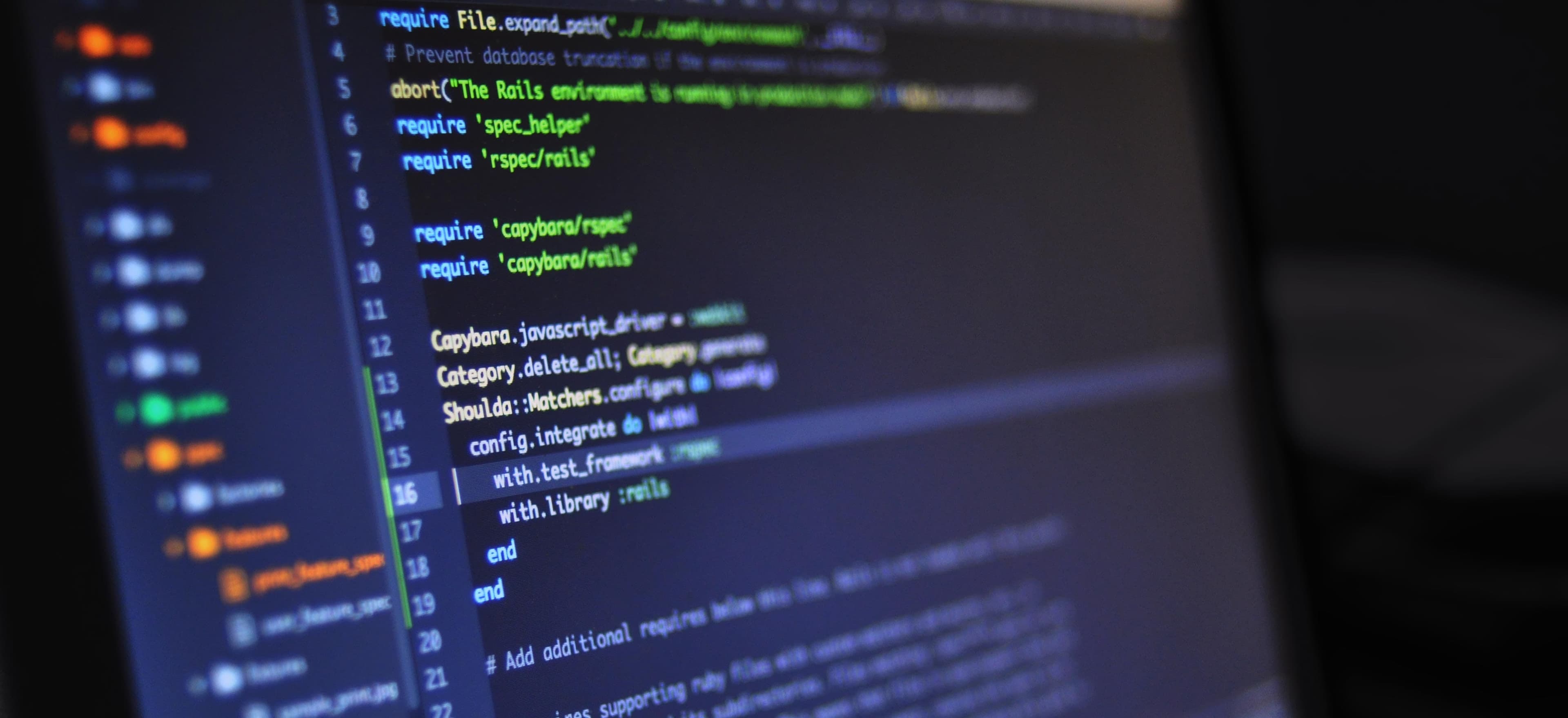
- Published on
Struggling with Code Complexity? Try AOP Today!
In the vast domain of software development, code complexity can often be the Achilles' heel of even the most well-structured applications. As applications grow, the intertwining of various functionalities can create a tangle that is hard to unravel. Enter Aspect-Oriented Programming (AOP), a paradigm designed specifically to tackle these issues by separating cross-cutting concerns. Let's dive into how AOP can help simplify your code while maintaining a clean and manageable architecture.
What is AOP?
Aspect-Oriented Programming is a programming paradigm that provides a way to modularize cross-cutting concerns, which are aspects of a program that affect various modules but don’t fit neatly into the primary responsibilities of those modules. Examples include logging, security, error handling, and transaction management.
Key Concepts in AOP
-
Aspect: A module that encapsulates a cross-cutting concern. It can define both behaviors (advice) and the conditions under which those behaviors are executed (pointcuts).
-
Join Point: A specific point in the execution of the program, such as the execution of a method or the handling of an exception.
-
Advice: Code that is executed at a join point. It can be applied before, after, or around a method execution.
-
Pointcut: An expression that matches join points. Pointcuts define where the advice should be applied.
-
Weaving: The process of integrating aspects with the program code to create a woven class.
Why Use AOP?
AOP provides several advantages that can help ease code complexity:
-
Separation of Concerns: By isolating cross-cutting concerns, AOP allows developers to focus on the core functionality of their code.
-
Reusability: Aspects can be reused across multiple classes and modules without duplicating code.
-
Maintainability: Changes to a concern can be made in one place, and they automatically apply wherever that aspect is used.
AOP in Java: Getting Started
In Java, AOP can be leveraged using frameworks such as Spring AOP or AspectJ. Here, we'll explore how to implement AOP using Spring, as it's one of the most popular options for enterprise applications.
Setting Up a Spring Project
You will need a basic Spring application to implement AOP. You can set this up using Spring Initializr:
- Choose Spring Web dependency.
- Add Spring AOP dependency.
Once you have your Spring Boot project set up, let's start coding!
Example: Implementing AOP for Logging
Consider a simple scenario where you want to log every method execution in your application. Using AOP, you can create a logging aspect that will seamlessly log method calls without cluttering your business logic.
Step 1: Create the Aspect
Here, you'll define your aspect, specifying the pointcut and advice:
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.annotation.After;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Pointcut;
import org.springframework.stereotype.Component;
@Component
@Aspect
public class LoggingAspect {
// Pointcut to define which methods to intercept
@Pointcut("execution(* com.example.service.*.*(..))")
public void serviceMethods() {}
// Advice that gets executed after the join point
@After("serviceMethods()")
public void logAfter(JoinPoint joinPoint) {
System.out.println("Method executed: " + joinPoint.getSignature().getName());
}
}
Commentary on the Code
-
@Aspect: Marks this class as as aspect. The Spring framework processes this class to identify the defined pointcuts and advice.
-
@Pointcut: The execution expression specifies that we want to capture all public methods from any class within the
com.example.service
package. This flexibility allows you to be precise about which methods you want to log. -
@After: This advice runs after the method execution, allowing you to log the method without altering the actual business logic.
Step 2: Create a Sample Service
Now, let’s create a simple service where our aspect can be applied:
import org.springframework.stereotype.Service;
@Service
public class UserService {
public void createUser(String username) {
System.out.println("User created: " + username);
}
public void deleteUser(String username) {
System.out.println("User deleted: " + username);
}
}
Commentary on the Service Code
In the above UserService
:
- It has simple methods that encapsulate business logic for creating and deleting users.
- Noticeably, there is no logging code mixed in, keeping the responsibilities clean and clear.
Step 3: Run Your Application
You should now run your Spring Boot application. If you invoke the createUser
and deleteUser
methods from a controller or another service, your aspect will automatically log method executions.
Expected Output
When you call these services, the expected output would be:
User created: user1
Method executed: createUser
User deleted: user1
Method executed: deleteUser
The Bottom Line
Aspect-Oriented Programming is a powerful pattern that can significantly reduce code complexity by architecting your application to support separation of concerns. By integrating AOP with Spring, developers can implement essential functionalities like logging, security, and transaction management cleanly and efficiently without entangling them within business logic.
If you're looking to streamline your development process and enhance maintainability, it's worth exploring AOP further.
For more extensive learning, check out the official Spring AOP documentation or tutorials that deep dive into advanced AOP concepts.
In a world where clean and maintainable code is king, AOP is a worthy tool in your software development toolkit—empower your coding practices today!
Checkout our other articles