Common Spring JDBC Mistakes New Developers Make
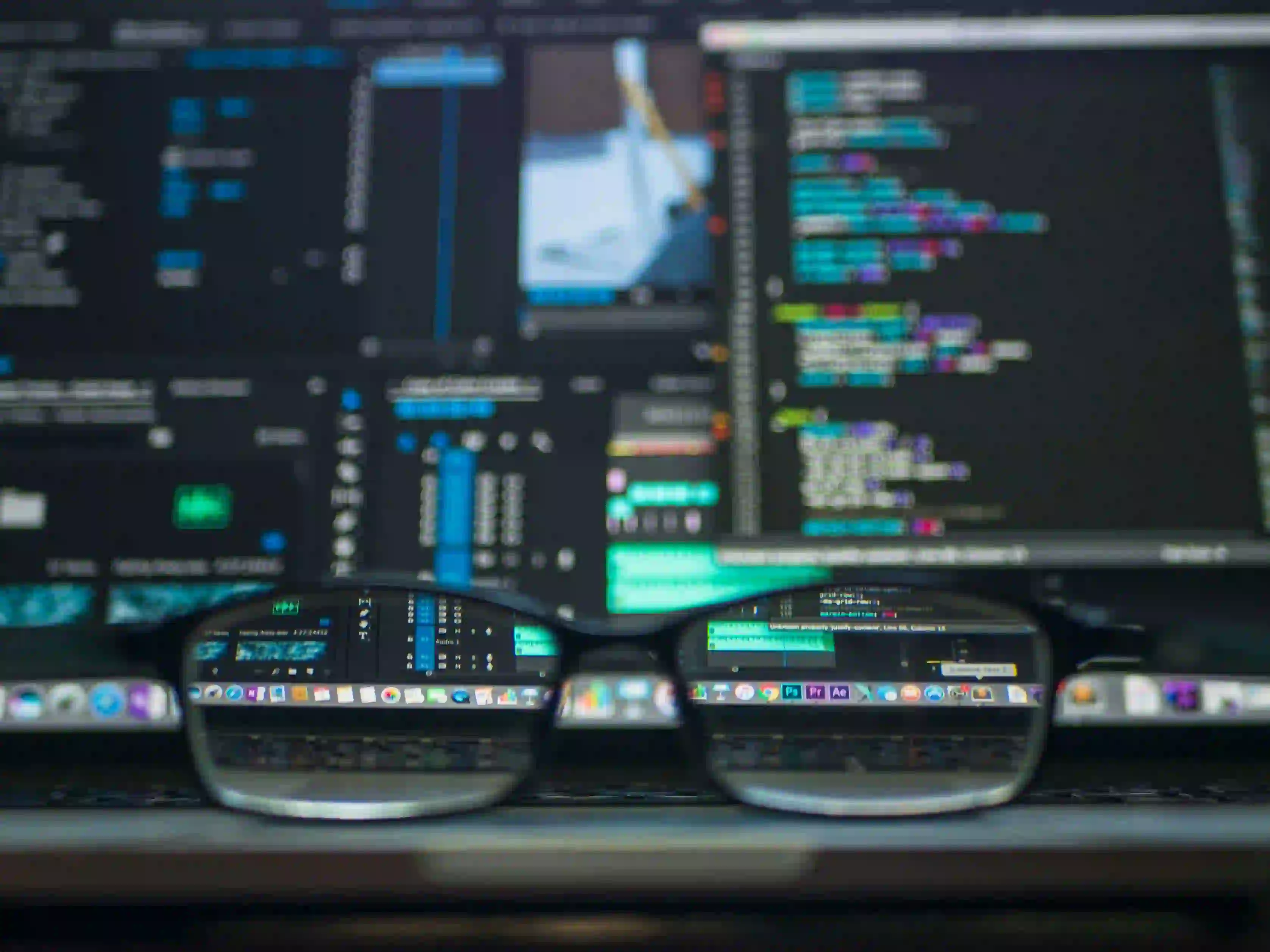
Common Spring JDBC Mistakes New Developers Make
Spring JDBC, an essential part of the Spring Framework, offers a powerful mechanism to interact with relational databases. However, new developers often stumble upon common pitfalls when working with this technology. Understanding these mistakes can greatly enhance your efficiency and effectiveness when developing Java applications with Spring. This blog post will delve into these common issues, providing insights and solutions along the way.
1. Not Using JdbcTemplate
One of the most common mistakes new developers make is not leveraging the JdbcTemplate
class provided by Spring. The JdbcTemplate
simplifies database operations and reduces boilerplate code, allowing for easier and cleaner interaction with the database.
Why Use JdbcTemplate?
Using JdbcTemplate
makes your code more concise and easier to maintain. It handles connection management, statement creation, and exception handling automatically.
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.jdbc.datasource.DriverManagerDataSource;
public class UserDao {
private JdbcTemplate jdbcTemplate;
public UserDao() {
DriverManagerDataSource dataSource = new DriverManagerDataSource();
dataSource.setDriverClassName("com.mysql.cj.jdbc.Driver");
dataSource.setUrl("jdbc:mysql://localhost:3306/mydb");
dataSource.setUsername("user");
dataSource.setPassword("password");
this.jdbcTemplate = new JdbcTemplate(dataSource);
}
public void save(User user) {
String sql = "INSERT INTO users (name, age) VALUES (?, ?)";
jdbcTemplate.update(sql, user.getName(), user.getAge());
}
}
Explanation
In the code above, using JdbcTemplate
simplifies the database operation. The boilerplate code for connection handling is eliminated, and the update
method handles prepared statements internally. This not only makes the code clearer but also protects against SQL injection attacks by using prepared statements.
2. Ignoring Exception Handling
Another serious mistake is the lack of proper exception handling. New developers often overlook the fact that database operations can fail for various reasons, such as connection issues, SQL syntax errors, or constraint violations.
Prepare for the Unexpected
Using the Spring DataAccessException
hierarchy can help you deal with exceptions elegantly.
import org.springframework.dao.DataAccessException;
public void save(User user) {
String sql = "INSERT INTO users (name, age) VALUES (?, ?)";
try {
jdbcTemplate.update(sql, user.getName(), user.getAge());
} catch (DataAccessException e) {
// Log error and handle exception as necessary
System.err.println("Database error occurred: " + e.getMessage());
}
}
Explanation
Here, we're catching DataAccessException
, allowing you to respond to any exceptions related to database access more appropriately. This not only improves user experience by handling potential failures gracefully but also helps in debugging.
3. Overusing Raw SQL Queries
While writing raw SQL queries can seem straightforward, it isn't the best practice for maintainability and readability. Using the JdbcTemplate
means that you can leverage its support for named parameters or even use RowMapper
for better abstraction.
Using RowMapper for Better Readability
import org.springframework.jdbc.core.RowMapper;
public User findById(int id) {
String sql = "SELECT * FROM users WHERE id = ?";
return jdbcTemplate.queryForObject(sql, new RowMapper<User>() {
@Override
public User mapRow(ResultSet rs, int rowNum) throws SQLException {
User user = new User();
user.setId(rs.getInt("id"));
user.setName(rs.getString("name"));
user.setAge(rs.getInt("age"));
return user;
}
}, id);
}
Explanation
Using a RowMapper
helps from a separation of concerns standpoint. It keeps your SQL clean and your mapping logic organized. This modularity makes it easier to maintain and scale your application as your data model evolves.
4. Forgetting to Close Resources
While Spring JDBC manages the data source connection pool, developers must still be mindful about resource management. Failing to close resources can lead to memory leaks or exhausted connections.
Closing Resources Properly
Using try-with-resources
makes sure that all database resources are closed appropriately.
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public void saveUser(User user) {
String sql = "INSERT INTO users (name, age) VALUES (?, ?)";
try (Connection conn = dataSource.getConnection();
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setString(1, user.getName());
pstmt.setInt(2, user.getAge());
pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
Explanation
In this snippet, the try-with-resources
ensures that the Connection
and PreparedStatement
are closed automatically, relieving developers from manual resource management. This reduces the chances of leaks and keeps the application more stable.
5. Not Using Transactions
Many new developers inadvertently skip transactions, believing that individual database operations are sufficient. However, operations across multiple database tables or services should be atomic, requiring proper transaction management.
Implementing Transactions
Spring provides a straightforward way to manage transactions declaratively.
import org.springframework.transaction.annotation.Transactional;
@Transactional
public void updateUserAndAccount(User user, Account account) {
save(user);
accountService.update(account);
}
Explanation
In this example, both save(user)
and accountService.update(account)
will operate under one transaction. If either operation fails, the transaction rolls back, preserving data integrity.
6. Not Configuring DataSource Properly
Incorrectly configuring your DataSource can lead to connection issues and inefficient database access. It's one of the leading causes of performance bottlenecks.
Proper DataSource Configuration
Make sure you configure your DataSource correctly to manage connections effectively.
import org.apache.commons.dbcp2.BasicDataSource;
public DataSource dataSource() {
BasicDataSource dataSource = new BasicDataSource();
dataSource.setDriverClassName("com.mysql.cj.jdbc.Driver");
dataSource.setUrl("jdbc:mysql://localhost:3306/mydb");
dataSource.setUsername("user");
dataSource.setPassword("password");
dataSource.setInitialSize(5);
dataSource.setMaxTotal(10);
return dataSource;
}
Explanation
In this configuration, we're setting up a connection pool with initial and maximum sizes. This is crucial for efficient resource usage, especially in a multi-threaded application context.
7. Lack of Proper Logging
Ignoring the importance of logging can lead to challenges in diagnosing issues. Each database interaction should provide insight into both requests and responses.
Using SLF4J for Logging
Incorporating logging frameworks like SLF4J makes it easy to track database operations.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class UserDao {
private static final Logger logger = LoggerFactory.getLogger(UserDao.class);
public void save(User user) {
String sql = "INSERT INTO users (name, age) VALUES (?, ?)";
try {
jdbcTemplate.update(sql, user.getName(), user.getAge());
logger.info("User saved: {}", user);
} catch (DataAccessException e) {
logger.error("Error while saving user: {}", e.getMessage(), e);
}
}
}
Explanation
Here, SLF4J allows for scalable logging. Proper logging practices improve traceability, which proves invaluable for both debugging and performance tuning.
Lessons Learned
Spring JDBC serves as a robust framework for database interactions, but new developers often stumble upon critical practices that can significantly affect application performance and maintainability. By avoiding these common mistakes—including not using JdbcTemplate
, ignoring exception handling, and overlooking transaction management—you can build more reliable, maintainable, and efficient Java applications.
For further reading on Spring JDBC best practices, you may find Spring's official documentation particularly useful. Additionally, check out comprehensive resources on Java database programming to enhance your knowledge.
Happy coding!