Understanding JSON Pointer: Common Pitfalls to Avoid
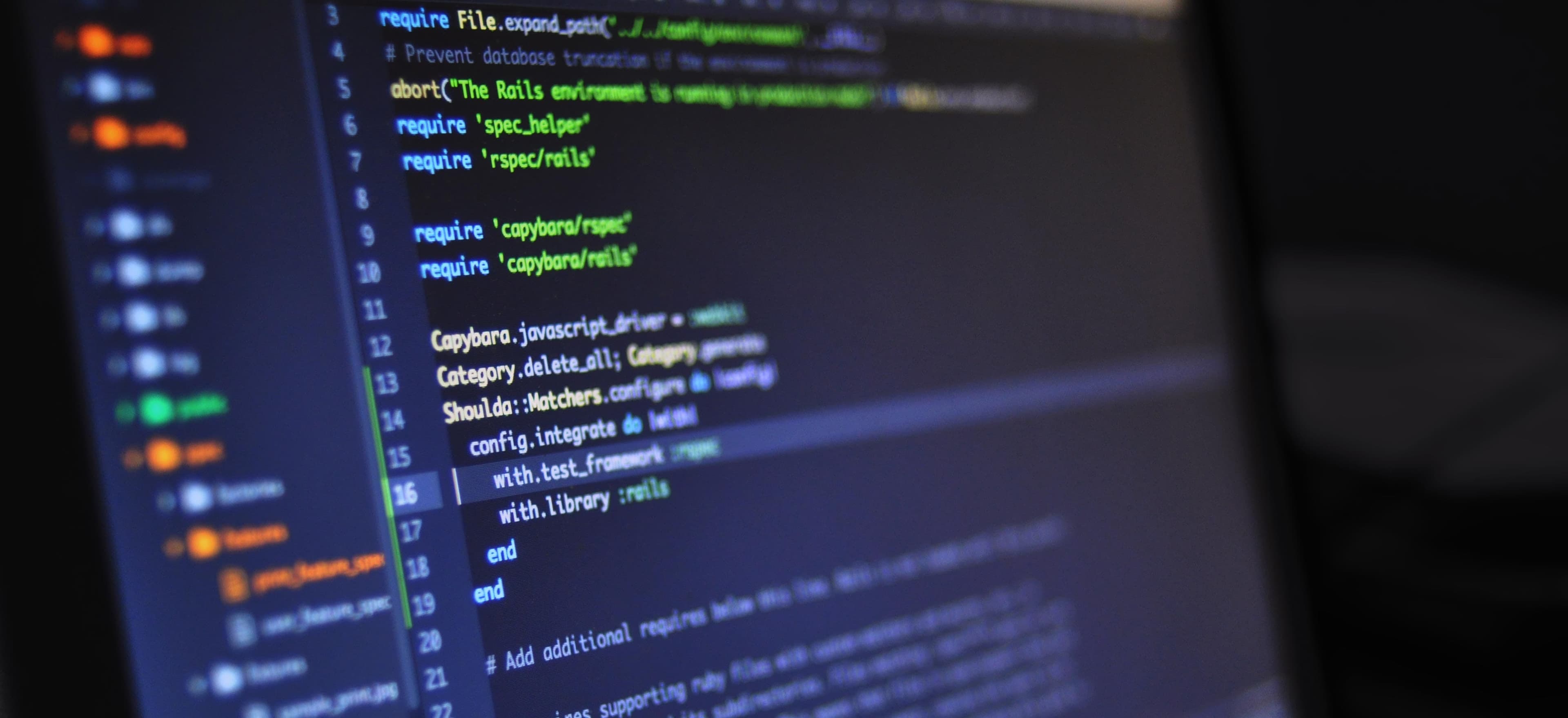
- Published on
Understanding JSON Pointer: Common Pitfalls to Avoid
When working with JSON (JavaScript Object Notation), facilitating access to object properties is vital, especially in REST APIs. JSON Pointer is a syntax for identifying a specific value within a JSON document. However, despite its straightforward concept, there are common pitfalls that developers often encounter. This article will provide an in-depth exploration of JSON Pointer, identifying its benefits, outlining potential screw-ups, and offering effective strategies to avoid them.
What is JSON Pointer?
JSON Pointer is a string format represented by a sequence of tokens separated by slashes (/). Each token corresponds to a key or index within a JSON object. For instance, consider the following JSON document:
{
"foo": {
"bar": "value",
"baz": [1, 2, 3]
}
}
- To access the value of "bar", the JSON Pointer would be
/foo/bar
. - To access the second element of the "baz" array, you would use
/foo/baz/1
.
The syntax allows clear and unambiguous access to different parts of a JSON document, making it incredibly useful for API responses and configurations.
Common Pitfalls
1. Using Incorrect Syntax
One of the primary issues users face with JSON Pointer is incorrect syntax. JSON Pointer has specific rules regarding the format.
- Leading Slash: The pointer must start with a single slash (/) which represents the root of the JSON document.
- Escaped Characters: Certain characters such as '~' and '/' need to be escaped. For instance, the JSON Pointer for a key named 'a/b' would be
/a~1b
(where '~1' is the slash escape).
Here’s an example of a JSON Pointer that would fail due to incorrect syntax:
// Incorrect Pointer
/foo/bar/baz // Missing leading slash
Solution: Always ensure your JSON Pointer starts with a '/' and correctly escape any reserved characters.
2. Misunderstanding Array Indexing
Arrays are indexed numerically, starting from zero. It’s common to confuse this with how you're used to accessing arrays in other programming languages.
{
"numbers": [10, 20, 30]
}
- To access the first number in the array, you need to use the pointer
/numbers/0
, not/numbers/1
.
Failing to recognize this can lead to off-by-one errors, where you might expect a different result.
3. Not Accounting for Missing Keys
When constructing applications that rely on dynamic JSON structures, it’s crucial to consider that certain keys may be absent from the JSON document. Suppose a JSON document looks like this:
{
"user": {
"name": "Alice"
}
}
Trying to access a non-existent key like /user/age
would result in an error or unexpected behavior. It’s vital to implement checks to verify whether the key exists before attempting to access its value.
Example Check in JavaScript:
const user = {
name: "Alice"
};
const agePointer = '/user/age';
const keys = agePointer.split('/').slice(1);
let currentValue = user;
for (const key of keys) {
if (currentValue && key in currentValue) {
currentValue = currentValue[key];
} else {
console.warn('Key does not exist:', key);
currentValue = undefined;
break;
}
}
console.log(currentValue); // Outputs: undefined
4. Confusion With Dot Notation
In some programming contexts, developers might prefer dot notation over JSON Pointer. JSON Pointer can lead to misunderstanding, especially when mixed with dot notation.
- JSON Pointer uses
/
while dot notation uses.
.
To clarify, if you have the following JSON:
{
"user": {
"details": {
"age": 30
}
}
}
- The JSON Pointer:
/user/details/age
- The dot notation:
user.details.age
Tip: Be consistent. Choose one format and stick to it across your project to prevent confusion.
5. Ignoring Context
JSON documents can nest deeply. Thus, it’s essential to maintain context while working with JSON Pointer. For example:
{
"users": [
{
"name": "Alice",
"age": 25
},
{
"name": "Bob",
"age": 30
}
]
}
If you're accessing Bob's data, you might forget to specify which user you're referring to. Using /users/1/name
is crucial here to pinpoint exactly the information you need.
Guidelines for Use
- Check Your Paths: Utilize tools like JSON Pointer Validator to validate the pointers you construct.
- Error Handling: Always implement error checking when accessing values via JSON Pointer. This step helps avoid crashes and unexpected behaviors.
- Consistent Structure: Maintain a consistent JSON structure to simplify data access and manipulation.
JSON Pointer in Action: A Simple REST API Example
Following our discussions on pitfalls, let's see JSON Pointer in action through a basic REST API implementation using Java.
Setting Up the API
Here’s a simple Spring Boot Controller that retrieves user data from a JSON object in a RESTful manner:
import org.springframework.web.bind.annotation.*;
import org.springframework.http.ResponseEntity;
import org.springframework.http.HttpStatus;
@RestController
@RequestMapping("/api")
public class UserController {
private final String userJson = "{ \"users\": [{\"name\": \"Alice\", \"age\": 25}, {\"name\": \"Bob\", \"age\": 30}] }";
@GetMapping("/user/{index}")
public ResponseEntity<String> getUser(@PathVariable int index) {
try {
// Assume parseJson is a utility function to extract data using JSON Pointer
String pointer = "/users/" + index;
String user = parseJson(userJson, pointer);
return ResponseEntity.ok(user);
} catch (Exception e) {
return ResponseEntity.status(HttpStatus.NOT_FOUND).body("User not found");
}
}
private String parseJson(String json, String pointer) {
// Implement a JSON Pointer parsing logic
// For this demonstration, it’s assumed to return the user's name in string format
return "Parsed User"; // Placeholder logic
}
}
A Final Look
Understanding and utilizing JSON Pointer can greatly simplify working with JSON documents, particularly in API development. However, it is essential to be aware of potential pitfalls such as incorrect syntax, array indexing errors, and missing keys. By keeping these common issues in mind and following best practices, you will significantly reduce frustration and enhance your JSON data handling capabilities.
For further reading on JSON Pointer and its specifications, consider RFC 6901, which provides detailed information about its specification. With careful implementation, JSON Pointer can be a powerful tool in your programming toolkit. Happy coding!