Top Programming Interview Mistakes to Avoid
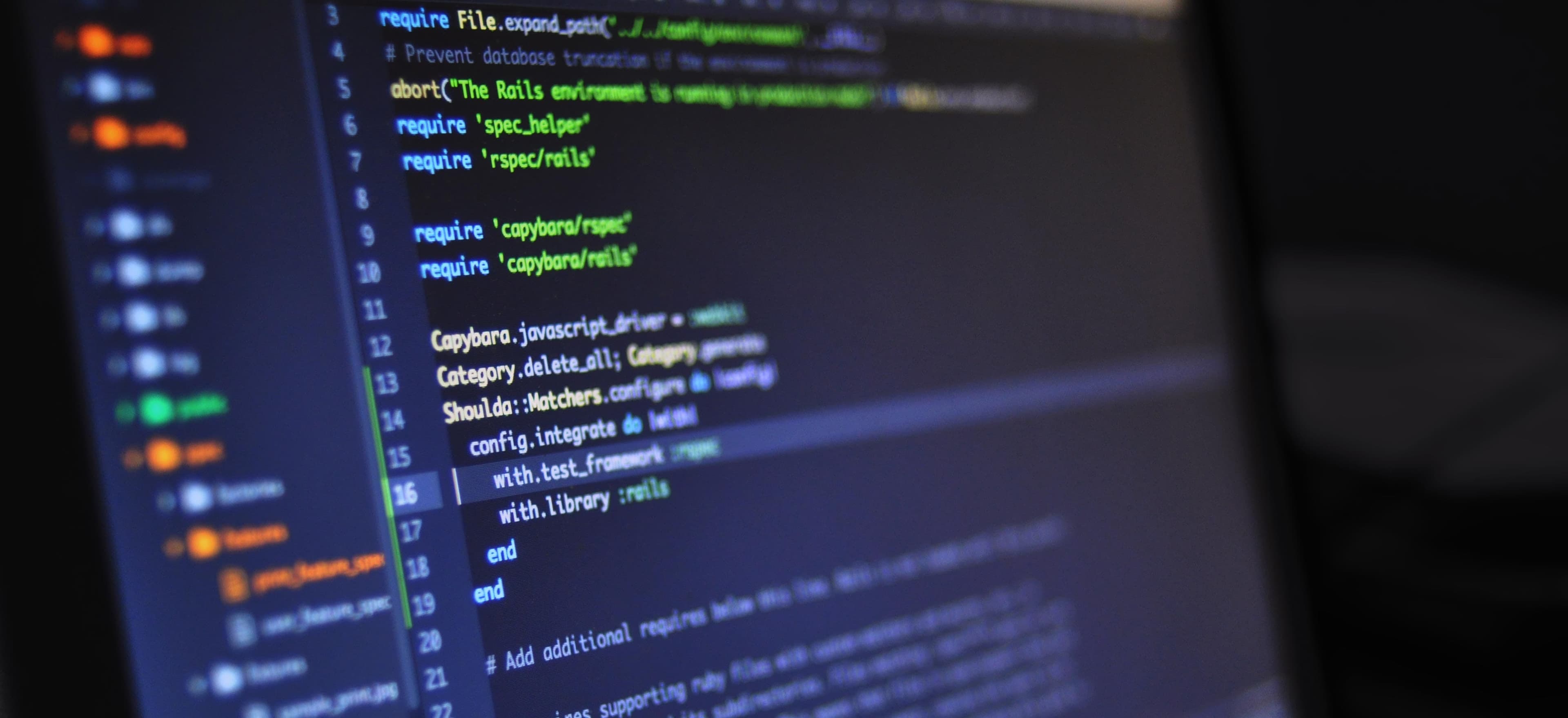
- Published on
Top Programming Interview Mistakes to Avoid
Preparing for a programming interview can be a daunting task. You might have solid coding skills, but sometimes even the best candidates fall short due to common mistakes. In this blog post, we will discuss the top programming interview mistakes to avoid, helping you enhance your performance and leave a lasting impression. Whether you are an experienced developer or just starting your coding journey, it's essential to be aware of these pitfalls.
1. Lack of Preparation
One of the gravest mistakes candidates make is failing to prepare adequately. Going into an interview without studying the basics can hinder your ability to demonstrate your skills.
Why Preparation Matters
Preparation gives you the confidence to tackle questions and highlights your problem-solving abilities. Familiarize yourself with algorithms, data structures, and coding challenges that are commonly asked in interviews. Utilize resources like LeetCode and HackerRank for practice.
Example of Preparation
Engaging in mock interviews can prepare you for the pressure of a real interview.
// Example of a mock interview preparation code
public class MockInterview {
public static void main(String[] args) {
System.out.println("Don't forget to practice common data structure problems!");
// Focus on arrays, strings, linked lists...
String[] commonStructures = {"Arrays", "Strings", "Linked Lists", "Trees", "Graphs"};
for (String structure : commonStructures) {
System.out.println("Have a solid understanding of " + structure);
}
}
}
2. Over-Complicating Solutions
When presented with a problem, it’s tempting to come up with a complex solution. However, interviewers typically favor straightforward solutions that are easy to understand and implement.
The Importance of Simplicity
A simple solution is often more effective and easier to debug. Always aim for clarity over complexity.
Example of a Simple Approach
Consider a problem requiring you to find the largest number in an array. A simple solution would look like this:
public class LargestNumberFinder {
public static int findLargest(int[] numbers) {
int largest = numbers[0]; // Initialize the largest number
for (int number : numbers) {
if (number > largest) {
largest = number; // Update if we find a new largest number
}
}
return largest; // Return the largest value
}
public static void main(String[] args) {
int[] numbers = {5, 3, 8, 1, 9};
System.out.println("The largest number is: " + findLargest(numbers));
}
}
In this example, we've focused on clarity, ensuring that anyone can understand our logic.
3. Ignoring Edge Cases
When solving problems, it’s vital to consider edge cases. Many candidates neglect scenarios where inputs could break their logic, such as empty arrays or null values.
Why Edge Cases Matter
Addressing edge cases demonstrates a deeper understanding of programming concepts and readiness for real-world challenges.
Example of Handling Edge Cases
Here’s how to enhance our previous code to handle an empty array:
public class EnhancedLargestNumberFinder {
public static int findLargest(int[] numbers) {
if (numbers == null || numbers.length == 0) {
throw new IllegalArgumentException("The array cannot be null or empty");
}
int largest = numbers[0];
for (int number : numbers) {
if (number > largest) {
largest = number;
}
}
return largest;
}
public static void main(String[] args) {
int[] numbers = {}; // Test for an empty array
try {
System.out.println("The largest number is: " + findLargest(numbers));
} catch (IllegalArgumentException e) {
System.out.println(e.getMessage());
}
}
}
This code provides a clear error message when the input is invalid, demonstrating robust programming practices.
4. Failing to Communicate Clearly
In interviews, communication is just as important as technical skills. Candidates often underestimate the significance of explaining their thought process.
Communication Breeds Understanding
Effective communication allows interviewers to understand your reasoning and approach to problem-solving, even if the final result is not perfect.
How to Communicate Effectively
- Verbalize Your Thought Process: As you code, talk through your ideas.
- Ask Clarifying Questions: If the problem statement is vague, do not hesitate to ask for more information.
- Summarize Your Solution: Upon completion, briefly summarize what your code does.
5. Spending Too Much Time on One Question
Candidates often get bogged down on tough questions, leading to poor time management. It's better to make progress on multiple questions than to dwell on a single problem.
Time Management Tips
- Set a Timer: Practice solving problems within a time limit.
- Prioritize Simpler Problems: If stuck, move on to the next question and return if time permits.
Example of Time Management
Here's how you can manage time during an interview effectively:
public class TimeManagementExample {
public static void main(String[] args) {
long startTime = System.currentTimeMillis();
// Simulate solving a problem
solveProblem();
long endTime = System.currentTimeMillis();
long timeTaken = endTime - startTime;
System.out.println("Time taken to solve the problem: " + timeTaken + " milliseconds");
}
public static void solveProblem() {
// Simulate a problem-solving logic
try {
Thread.sleep(1500); // Simulate time taken to solve
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
This snippet shows how you can introspectively check your time efficiency during practice sessions.
6. Neglecting Follow-Up Questions
After completing your coding task, interviewers may ask follow-up questions. Ignoring these can lead to missed opportunities for demonstrating your depth of knowledge.
Embrace the Challenge
Even if you feel your initial answer was correct, follow-up questions test your adaptability and deeper understanding.
A Follow-Up Example
If you were asked to improve the performance of our earlier example, you could discuss alternative approaches, like using a max-heap.
A Final Look
To sum up, avoiding common interview mistakes is paramount in showcasing your true programming capabilities. By properly preparing, simplifying your solutions, considering edge cases, communicating effectively, managing your time wisely, and engaging with follow-up questions, you can significantly improve your performance.
Additional Resources
For further reading and preparation, consider exploring these resources:
By being aware of these pitfalls and employing the strategies discussed, you're setting yourself up for success in your programming interviews. Remember, preparation is key — so start today!
Checkout our other articles