Unlocking JDK 12 Javadoc: Mastering Tag and Property Issues
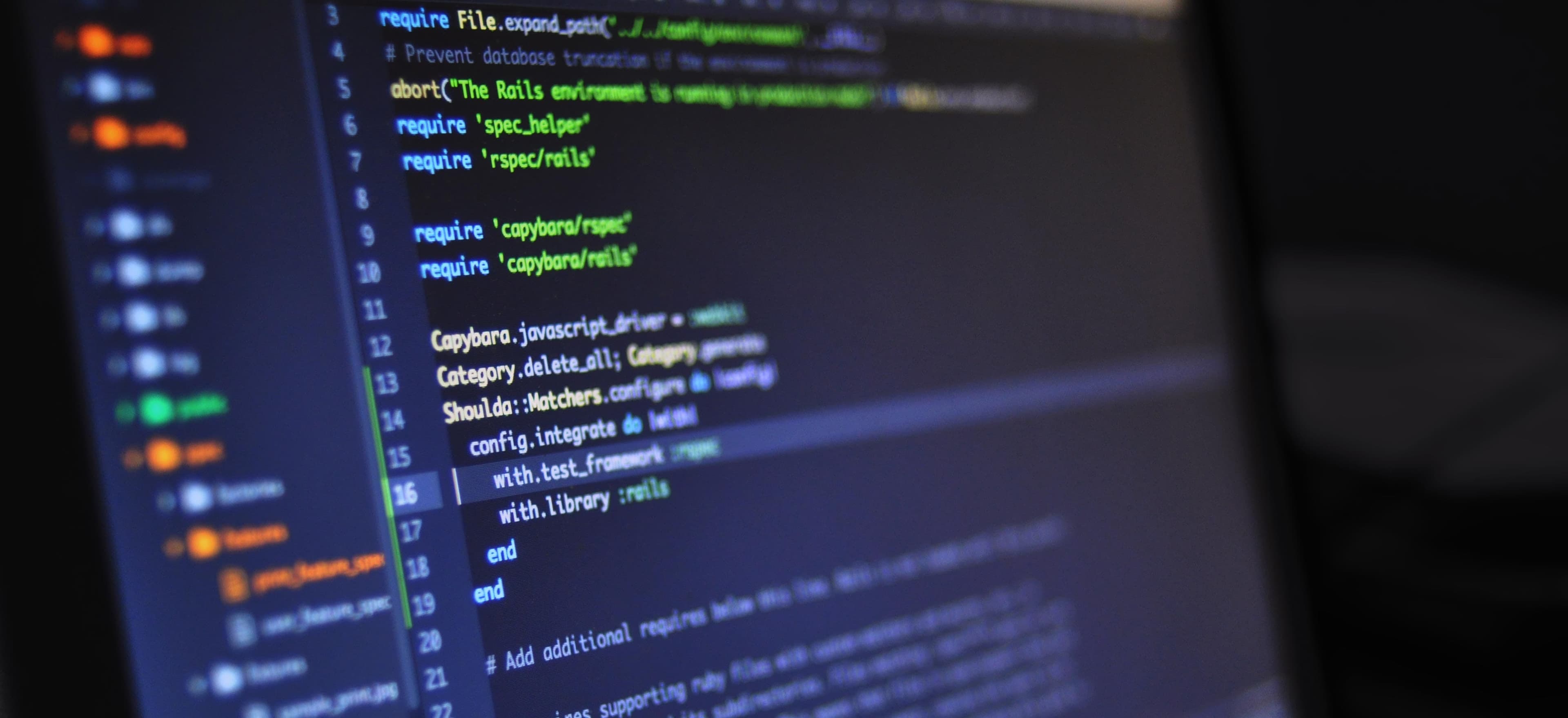
- Published on
Unlocking JDK 12 Javadoc: Mastering Tag and Property Issues
When working with Java development, documentation is a crucial aspect that often gets overlooked. The Javadoc tool, a standard tool for generating API documentation in HTML format from Java source code, has evolved over the years, especially with the introduction of JDK 12. In this post, we will explore common issues regarding tags and properties in Javadoc, as well as how to effectively troubleshoot and improve your documentation quality.
What is Javadoc?
Javadoc is a documentation generator that extracts comments from Java code to generate HTML documents. By using special tags in the comments, developers can provide readers with useful information about classes, methods, fields, and other elements of their code. The clarity and comprehensiveness of these comments can greatly enhance the usability of your software projects.
Understanding Tags in Javadoc
Tags in Javadoc play a vital role in structuring the documentation. Some commonly used tags include:
@param
: Describes a parameter for a method.@return
: Describes the return type of a method.@throws
or@exception
: Documents the exceptions thrown by a method.@see
: Provides a reference to related classes or methods.@deprecated
: Indicates that a method or class should no longer be used.
Example of Using Tags
To illustrate the use of tags, let’s look at a simple example of a Java class:
/**
* This class performs operations on mathematical integers.
*/
public class MathOperations {
/**
* Adds two integers.
*
* @param a First integer
* @param b Second integer
* @return The sum of a and b
* @throws IllegalArgumentException if a or b are negative
*/
public int add(int a, int b) {
if (a < 0 || b < 0) {
throw new IllegalArgumentException("Integers must be non-negative.");
}
return a + b;
}
}
In this example, we have documented the add
method thoroughly. The use of @param
, @return
, and @throws
tags provides clear information about the method's behavior and expectations.
Why Tags Matter
Properly using tags enhances the readability and usability of your API documentation. Moreover, they allow tools and IDEs to provide better assistance for developers who use your code. When you incorporate these tags, you make your code self-documenting, which saves time for both you and others in the long run.
Common Property Issues in Javadoc
Properties in Javadoc refer to the configuration options you can apply to customize the generated documentation. Issues often arise when incorrect or deprecated properties are used, leading to generation errors or suboptimal documentation output. Below are some common property issues and how to resolve them.
1. -author
and -version
Tags
Developers often forget to add the @author
and @version
tags in their code. These tags are essential in identifying the creator and version of the class or interface. Without them, the generated documentation lacks crucial context.
Example
/**
* @author John Doe
* @version 1.0
*/
public class ExampleClass {
// Class implementation
}
2. Missing or Misconfigured -doctitle
When generating documentation, you can use the -doctitle
property to set a custom title for your generated documentation. Failing to configure this can make your Javadoc appear generic.
javadoc -d docs -doctitle "My Project API Documentation" -sourcepath src -subpackages com.myproject
Setting a meaningful document title enhances clarity when multiple libraries or documentation sets are available.
3. Incorrectly Specified -sourcepath
If your source files are not organized correctly or the source path is incorrectly specified, the tool may fail to locate the files you want to include in the documentation.
javadoc -d docs -sourcepath src -subpackages com.myproject
Ensure that the path correctly points to your Java files. Simple typo mistakes can lead to frustrating debugging sessions.
Creating Javadoc with JDK 12
With JDK 12, several new features and improvements have been introduced. To create Javadoc, you can use the command line:
javadoc --release 12 -d docs -sourcepath src -subpackages com.myproject
Key Options:
--release
: Generates documentation for a specific release.-d
: Specifies the destination directory for the generated files.-sourcepath
: Defines the source path to find your Java files.-subpackages
: Allows you to include all classes within specified packages.
Enhancements in JDK 12 Javadoc
JDK 12 comes with new features aimed at improving the usability of the generated documentation. Here are a few notable enhancements:
- HTML5 Support: Javadoc now generates documentation based on HTML5, providing better UI on various devices.
- Search Functionality: Enhanced search features allow developers to locate classes and methods more easily.
- Custom Formatting: You can now apply custom themes for better aesthetics.
The Java Platform, Standard Edition 12 documentation provides detailed insights into these enhancements.
Troubleshooting Javadoc Issues
At times, you may run into various issues while using Javadoc. Here are some basic troubleshooting steps:
- Check the Console Output: Javadoc will provide error messages in the console. Pay close attention to these.
- Review Your Annotations: Ensure that you are correctly using Javadoc annotations. If errors persist, revisit the Javadoc documentation.
- Keep JDK Up to Date: Ensure that you’re using the latest version of JDK and have all the necessary updates installed.
Closing the Chapter
Mastering Javadoc is invaluable for any Java developer who aims to build software that stands the test of time. In JDK 12, new features make this documentation tool even more powerful. By properly utilizing tags, configuring properties, and understanding common pitfalls, you can ensure that your Java documentation is effective and user-friendly.
In turn, this will not only streamline your development process but also enhance the overall user experience with your APIs. As you incorporate these best practices, you will find yourself crafting clearer, more maintainable, and valuable code.
For more detailed reading, check out the official Java Documentation. As you navigate the complexities of Java programming, remember: the better your documentation, the smoother your coding experience will be. Happy coding!