Decoding Thread Dumps: A Guide to Smart Analysis
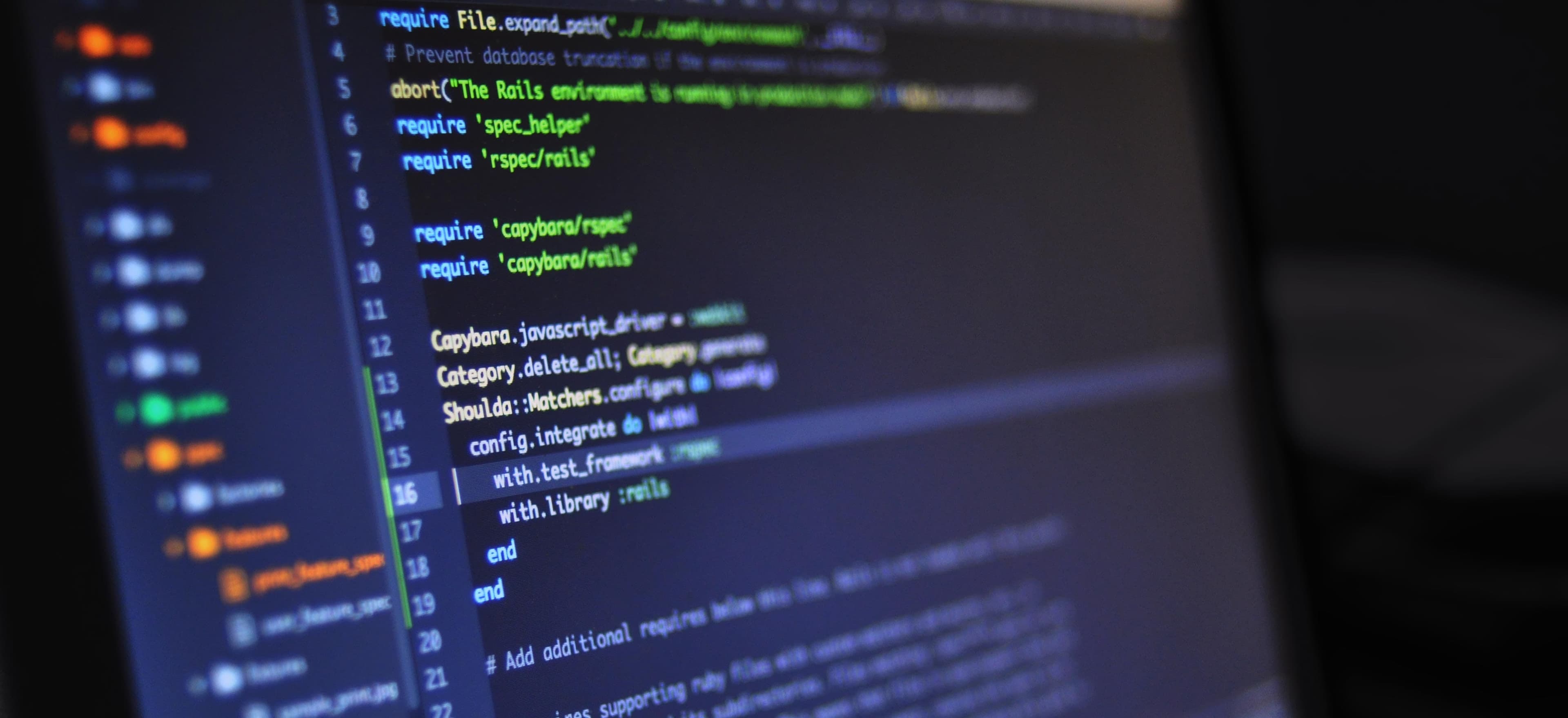
- Published on
Decoding Thread Dumps: A Guide to Smart Analysis
In the world of Java programming, performance and stability are paramount. One of the most effective tools for diagnosing issues within a Java application is the thread dump. But what exactly is a thread dump, and how can it be analyzed to improve your application's performance? In this guide, we'll decode the art of thread dumps and explore how to read and analyze them intelligently.
What is a Thread Dump?
A thread dump is a snapshot of all the threads that are currently in a Java Virtual Machine (JVM) at a specific moment in time. It includes information such as thread names, states, stack traces, and whether the threads are waiting, blocked, or runnable. Essentially, it’s a weather report for your application's concurrency model.
Thread dumps can be especially useful in diagnosing performance bottlenecks, deadlocks, or high CPU usage. To generate a thread dump, you can use several methods, including:
- jstack tool that comes with the JDK.
- Integrated Development Environments (IDEs) like IntelliJ IDEA or Eclipse.
- Management tools like VisualVM or JConsole.
jstack <pid> > thread_dump.txt
Replace <pid>
with your Java process ID. This command captures the state of all threads and outputs it to a file, which you can then analyze.
Understanding Thread States
Understanding the states of threads is vital for effective analysis. Here are the key states you will encounter in a thread dump:
- RUNNABLE: The thread is executing.
- BLOCKED: The thread is waiting to acquire a lock that another thread holds.
- WAITING: The thread is waiting indefinitely for another thread to perform a particular action.
- TIMED_WAITING: The thread is waiting for another thread to perform an action for up to a specified waiting time.
- TERMINATED: The thread has finished executing.
Analyzing a Thread Dump
To dive into decoding a thread dump, follow these steps:
Step 1: Capture a Thread Dump
Before you analyze, you'll need to capture a thread dump. Here’s an example command you might use in the terminal:
jstack 12345 > thread_dump.txt
In this example, 12345
is a placeholder for your specific Java process ID. The output will contain various detailed entries for threads.
Step 2: Read the Thread Dump
Let’s look at a sample snippet from a thread dump:
"Thread-1" #11 prio=5 os_prio=0 tid=0x00007f8e200a4000 nid=0x2aa0 waiting for monitor entry (m=0x00007f8e40042f70)
java.lang.Object.wait(Native Method)
java.lang.Object.wait(Object.java:502)
my.package.MyClass.myMethod(MyClass.java:32)
...
- Thread Name: "Thread-1" indicates the thread's name. It's often useful to give threads descriptive names according to their functionality.
- Priority:
prio=5
indicates the thread's priority. - Stack Trace: This tells us what the thread was doing when the dump was captured. Note the method
myMethod
inMyClass
—that's where we might focus our analysis.
Step 3: Identifying Issues
One crucial thing to note is examining the thread states.
- If you see many threads in the BLOCKED state, a particular method might be locking resources aggressively.
- Too many WAITING threads can indicate an issue with not signaling other threads correctly.
Example of Troubleshooting with a Thread Dump
Let’s assume you find that a large number of threads are stuck in the BLOCKED state. Consider this example thread dump:
"Thread-3" #15 prio=5 os_prio=0 tid=0x00007f8e200a5000 nid=0x3abc waiting for monitor entry (m=0x00007f8e40042fc0)
- waiting to lock <0x00007f8e40080b98> (a java.lang.Object) held by "Thread-2"
"Thread-2" #13 prio=5 os_prio=0 tid=0x00007f8e200a6000 nid=0x3abc in Object.wait() ...
In this case, Thread-3 is waiting for a lock held by Thread-2.
Focus on Synchronization
This type of issue often points to synchronization problems. To alleviate this, you might consider:
- Reducing the scope of synchronized blocks.
- Using
java.util.concurrent
packages to manage threads efficiently.
Code Example: Improving Synchronization
Instead of this:
public synchronized void updateData() {
// critical section
}
You can reduce contention with this approach using ReentrantLock:
public void updateData() {
Lock lock = new ReentrantLock();
lock.lock();
try {
// critical section
} finally {
lock.unlock();
}
}
In this revised code, we explicitly lock a block of code and ensure it gets released, thereby reducing the chances of deadlock and improving concurrency.
Tools for Thread Dump Analysis
If manual analysis isn't your cup of tea, various tools can assist in visualizing and analyzing thread dumps, such as:
- VisualVM: Offers visualization, monitoring, and troubleshooting capabilities.
- JConsole: A simple tool that comes with JDK.
- Thread Dump Analyzer: A dedicated tool for examining thread dumps.
These tools can help you quickly pinpoint problems in your thread management, offering a UI-based approach rather than textual analysis.
Wrapping Up
Interpreting thread dumps isn’t just about reading technical jargon. It’s about understanding the underlying patterns of your application’s behavior. By becoming proficient at analyzing thread dumps, you can identify and address performance bottlenecks, improve your application's stability, and enhance user experience.
So the next time you encounter a performance issue, don’t forget to generate a thread dump and utilize its rich insights to keep your Java application running smoothly.
For more in-depth resources on Java concurrency, consider checking out the Java Concurrency Tutorial and explore various threading models that can enhance performance and responsiveness.
Happy coding!