Streamline Your Gradle Projects: SonarQube Analysis in Docker
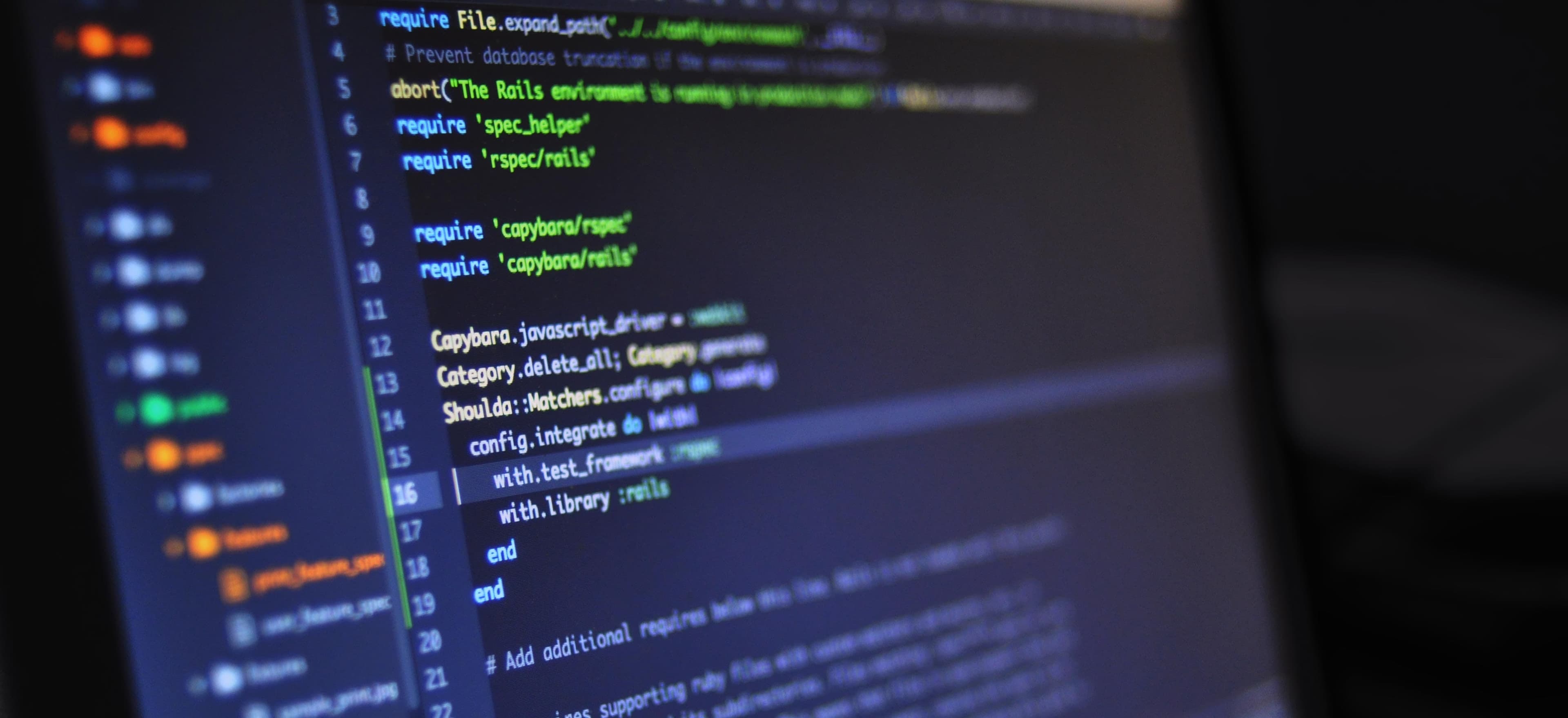
- Published on
Streamline Your Gradle Projects: SonarQube Analysis in Docker
As a software developer, you’re likely always on the lookout for ways to improve code quality, maintainability, and overall project hygiene. One of the most powerful tools at your disposal is SonarQube, which provides comprehensive code analysis to highlight issues and improve the quality of your codebase. In this blog post, we’ll explore how to integrate SonarQube analysis into your Gradle projects using Docker, streamlining your development workflow and enhancing code quality.
What is SonarQube?
SonarQube is an open-source platform that helps developers manage technical debt and improve code quality. It provides continuous inspection of code quality and offers features such as:
- Static code analysis
- Code coverage
- Duplication detection
- Code metrics
- Vulnerability detection
With SonarQube, your team can keep track of code quality, facilitating better decision-making and ensuring higher code standards.
Why Use Docker for SonarQube?
Using Docker to manage SonarQube has several advantages:
- Isolation: Each container runs in its own isolated environment, meaning you can avoid conflicts with other services.
- Simplicity: Deploying SonarQube becomes much more manageable with Docker. You can set it up with a single command and have it run anywhere.
- Scalability: Docker containers can be easily scaled to meet the requirements of your application.
Prerequisites
Before we dive into the integration process, ensure you have the following installed:
Make sure that Docker is running on your machine, and you have a basic Gradle project ready to analyze.
Setting Up SonarQube with Docker
Let’s start by running a SonarQube server using Docker. Open your terminal and run the following command:
docker run -d --name sonarqube -p 9000:9000 sonarqube
Explanation of the Command:
docker run -d
: This command starts a Docker container in detached mode.--name sonarqube
: This assigns a name to the container, making it easier to manage.-p 9000:9000
: This maps port 9000 on your host machine to port 9000 on the container.sonarqube
: This specifies the SonarQube image to use.
After running this command, you should have a SonarQube instance accessible at http://localhost:9000.
Configuring Your Gradle Project
Next, we need to set up your Gradle project to use SonarQube for analysis. Begin by modifying your build.gradle
file to include the necessary plugins.
Step 1: Add the SonarQube Plugin
You can apply the SonarQube plugin by adding it to your build.gradle
:
plugins {
id "org.sonarqube" version "3.2.0"
}
Step 2: Configure SonarQube Properties
Now configure the SonarQube properties within your build.gradle
. Modify it to look as follows:
sonarqube {
properties {
property "sonar.projectKey", "your_project_key"
property "sonar.host.url", "http://localhost:9000"
property "sonar.login", "your_sonar_token" // optional if you use anonymous access
property "sonar.sources", "src/main/java"
property "sonar.tests", "src/test/java"
property "sonar.java.binaries", "build/classes/java/main"
}
}
Explanation of Properties:
- sonar.projectKey: This uniquely identifies your project in SonarQube.
- sonar.host.url: The URL of your SonarQube server.
- sonar.login: This is used for authentication purposes if you have set up security on your SonarQube server.
- sonar.sources: Path to your source code.
- sonar.tests: Path to your test code.
- sonar.java.binaries: Path to compiled Java classes.
Performing the Code Analysis
Once you have configured your build.gradle
file, you can run your SonarQube analysis. Execute the following command in your terminal:
./gradlew sonarqube
What Happens During the Analysis:
- Gradle compiles the code and collects metrics.
- SonarQube sends these metrics to the SonarQube server.
- The server processes the received data, performing static analysis and reporting any issues.
You can monitor the analysis in your terminal. Upon successful completion, browse to http://localhost:9000 and log in to check the analysis results.
Continuous Integration and SonarQube
Integrating SonarQube analysis into your CI/CD pipeline can further enhance your workflow. Here’s how to do that.
Step 1: Modify Your CI/CD Configuration
If you’re using a CI tool like Jenkins, Travis CI, or GitHub Actions, you can set it up to run the SonarQube analysis at every build. Here's an example configuration for GitHub Actions:
name: Build and Analyze
on:
push:
branches: [ main ]
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up JDK
uses: actions/setup-java@v1
with:
java-version: '11'
- name: Build with Gradle
run: ./gradlew build sonarqube
env:
SONAR_HOST_URL: http://localhost:9000
SONAR_TOKEN: ${{ secrets.SONAR_TOKEN }}
Explanation of the CI Configuration:
- The job triggers on every push to the
main
branch. - It checks out the repository, sets up the JDK, builds the project, and runs the SonarQube analysis.
- Environment variables for
SONAR_HOST_URL
andSONAR_TOKEN
are set for authentication.
Key Takeaways
By integrating SonarQube analysis into your Gradle projects using Docker, you create a robust mechanism to ensure high-quality, maintainable code. With its rich features, SonarQube allows your team to keep technical debt in check and embrace best coding practices.
Make sure to keep your SonarQube Docker container running during development for continuous feedback on code quality. Dive into SonarQube's official documentation for more advanced configurations, rules, and integrations!
Happy coding!