Navigating Microservices: Avoiding Common Integration Pitfalls
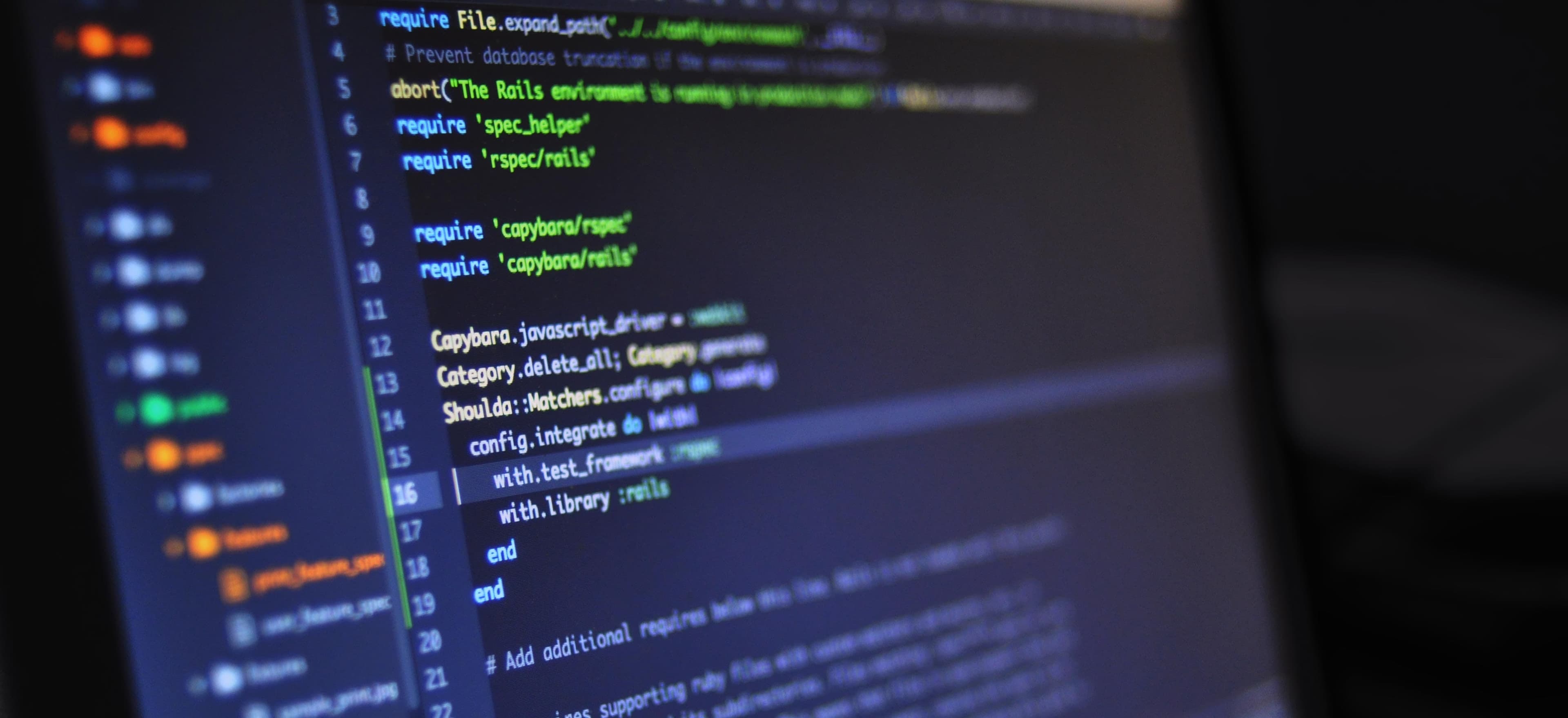
- Published on
Navigating Microservices: Avoiding Common Integration Pitfalls
In recent years, the microservices architecture has gained significant popularity in software development. It offers a flexible, scalable, and maintainable approach to application design. However, despite its advantages, integrating microservices presents several challenges. Understanding these pitfalls can guide you in reducing friction during integrations and enhancing your application's performance.
This blog post delves into common integration pitfalls in microservice architectures and provides practical strategies to navigate them effectively.
What are Microservices?
Before diving into the challenges, it is essential to clarify what microservices are. Microservices are a design pattern in which an application is divided into smaller, loosely coupled services, each responsible for a specific business function. These services communicate through lightweight protocols, often using REST APIs or messaging queues.
Why choose microservices? This architecture enables:
- Scalability: Different services can be scaled independently.
- Flexibility in Technology: Teams can choose different programming languages or databases for different services.
- Improved Team Autonomy: Smaller teams can own and manage specific services.
However, with these benefits come integration challenges, particularly as services grow in number and complexity.
Common Integration Pitfalls
1. Siloed Services and Lack of Collaboration
One major pitfall is creating siloed services that do not communicate effectively with each other. Each service might evolve differently if teams are not collaborating. This lack of communication can lead to redundancy and incompatible data models, slowing down development.
Solution: Foster Open Communication
Encourage a culture of collaboration across teams. Implement regular meetings to discuss integrations and align goals. Using tools like Slack or Microsoft Teams can facilitate ongoing discussions.
2. Over-Engineering Communication
In microservices, it's tempting to use complex message brokers and protocols to establish communication. However, over-engineering can introduce unnecessary complexity.
Tip: Keep It Simple
For many applications, simple REST APIs are sufficient. Use HTTP and JSON for synchronous communication. For asynchronous communication, consider lightweight message brokers like RabbitMQ or Apache Kafka—but limit the complexity to what is absolutely necessary.
Example: Basic REST API
Here’s a simple example in Java using Spring Boot for a RESTful microservice:
@RestController
@RequestMapping("/api/users")
public class UserController {
private final UserService userService;
@Autowired
public UserController(UserService userService) {
this.userService = userService;
}
@GetMapping("/{id}")
public ResponseEntity<User> getUserById(@PathVariable String id) {
User user = userService.findUserById(id);
return ResponseEntity.ok(user);
}
}
Why This Matters:
This example shows how REST APIs can provide a clear and straightforward way to expose services without unnecessary complexity. It allows easy integration and better understanding across teams.
3. Inconsistent Data Management
In a microservices environment, each service often manages its own data. However, using different data schemas across services can lead to data inconsistency. When one service updates a user’s information, for example, other services may not reflect this update if they operate on a stale data model.
Tip: Adopt Distributed Data Management Strategies
Utilizing event sourcing or change data capture mechanisms can alleviate this issue. By publishing events to a message broker whenever a change occurs, other services can react to those changes in real-time.
Example: Event-Based System
Using an event-driven approach in Java could look like this:
public class UserUpdatedEvent {
private final String userId;
private final String name;
public UserUpdatedEvent(String userId, String name) {
this.userId = userId;
this.name = name;
}
public String getUserId() {
return userId;
}
public String getName() {
return name;
}
}
Why This Matters:
Publishing events when changes occur allows other services to listen to these events and update their data models accordingly. This consistency helps prevent discrepancies and improves inter-service communication.
4. Lack of Service Discovery
Incorporating numerous microservices creates a challenge for locating and communicating with each other. Without an effective service discovery mechanism, hardcoding service endpoints can lead to issues with scalability and flexibility.
Solution: Implement Service Discovery
Tools like Netflix Eureka or Consul can be vital for service discovery. These tools allow microservices to register themselves and locate other services dynamically.
5. Poor API Management
APIs are the lifelines of microservices, yet poor management can lead to confusion, outdated documentation, and broken integrations. If API changes are not communicated effectively, it can break dependent services.
Tip: Use API Gateways and Versioning
Consider using an API gateway to manage traffic and enforce usage policies. Leverage versions for your APIs to ensure backward compatibility.
Example: API Gateway Configuration with Spring Cloud
Here is how you can configure an API gateway using Spring Cloud Gateway:
spring:
cloud:
gateway:
routes:
- id: user-service
uri: lb://user-service
predicates:
- Path=/api/users/**
Why This Matters:
An API gateway centralizes your microservice interactions, streamlining the way clients access your services. Versioning ensures that existing consumers continue to function seamlessly while you iterate on your APIs.
6. Monitoring and Logging Gaps
Microservices inherently complicate monitoring and logging. With multiple services generating logs across different environments, it becomes difficult to trace issues affecting the system.
Tip: Implement Centralized Logging and Monitoring
Utilizing tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Prometheus with Grafana can streamline this process. They provide a consolidated view of logs and metrics for better observability.
Conclusion
Microservices offer myriad advantages but integrating them comes with its share of challenges. By recognizing these common pitfalls—siloed services, over-engineering communication, inconsistent data management, lack of service discovery, poor API management, and monitoring gaps—you can equip your teams with strategies to avoid them.
When adopting microservices, always prioritize simplicity, collaboration, and observability. You can create a robust, scalable architecture that serves your organization’s needs by doing so.
Further Reading
- Microservices Best Practices for Java Developers: link
- Understanding Distributed Systems: link
In summary, navigate the complexities of microservices thoughtfully, leverage existing tools effectively, and foster open communication among your teams. Doing so will lead to lower friction in integration processes, higher productivity, and, ultimately, a successful microservices implementation. Happy coding!