Java Solutions for Common Cloud Deployment Issues
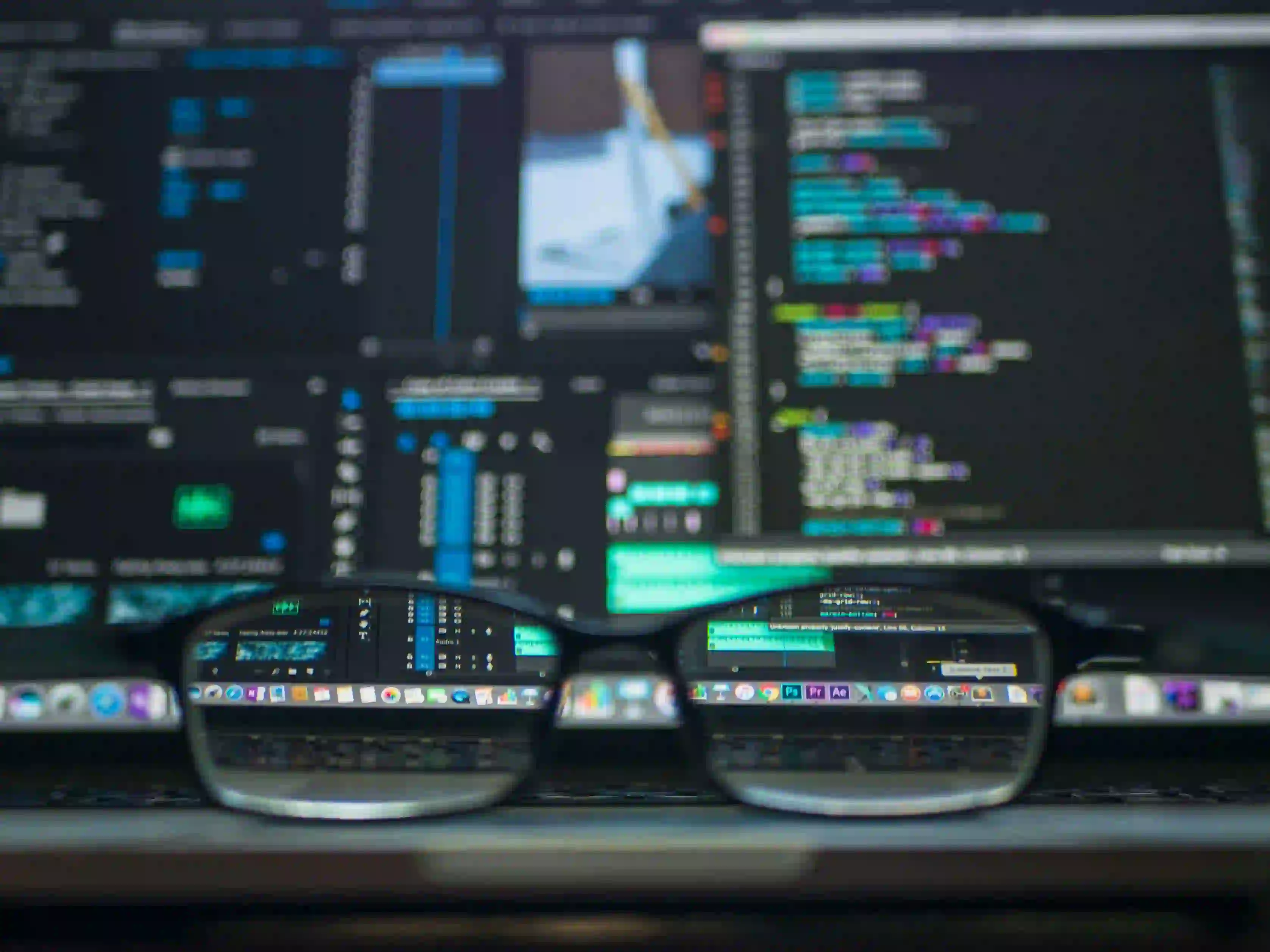
Java Solutions for Common Cloud Deployment Issues
In the ever-evolving world of cloud computing, developers frequently encounter deployment challenges that can hinder productivity. From configuration mishaps to environment inconsistencies, these obstacles can be especially pronounced when working with frameworks like Java. In this blog post, we will explore some common cloud deployment issues faced by Java developers and discuss effective solutions to navigate these challenges.
Understanding the Cloud Deployment Landscape
Before diving into specific solutions, it is essential to grasp the cloud deployment landscape. Cloud deployment refers to the method by which applications are delivered to users over the internet. There are various models for cloud deployments, including Infrastructure as a Service (IaaS), Platform as a Service (PaaS), and Software as a Service (SaaS). Each model presents unique challenges but also showcases the flexibility that Java applications can leverage.
Why Choose Java for Cloud Deployment?
Java is a popular choice for cloud deployments due to its platform independence, robust security features, and rich ecosystem of libraries and frameworks. Moreover, its scalability makes it well-suited for cloud environments where applications may need to scale in response to varying loads. However, deploying Java applications to the cloud can bring about its own set of challenges.
Common Deployment Challenges in Java
1. Dependency Management
One of the most common issues developers face when deploying Java applications is managing dependencies. Various Java libraries may have conflicting versions, leading to "jar hell." This issue is particularly pertinent when deploying applications in cloud environments where multiple services may interact with the same dependencies.
Solution: Using Build Tools
To tackle dependency management, leverage popular build tools like Maven or Gradle. These tools help define dependencies in a concise manner and resolve version conflicts automatically.
<!-- Sample Maven dependency configuration -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>5.3.10</version>
</dependency>
In this Maven configuration, the Spring Core library is added as a dependency. By specifying the version, we ensure that our application uses a consistent library version during deployment. Proper dependency management is crucial to avoid runtime errors related to version mismatches.
2. Configuration Management
Configuration files manage various application settings, including database connections, API keys, and environment details. Hardcoding configurations can lead to significant deployment headaches, especially when moving between environments like staging and production.
Solution: External Configuration Management
Instead of hardcoding configurations, consider using tools such as Spring Cloud Config or Consul. These solutions allow you to externalize configuration, making it easy to update settings without redeploying your application.
// Externalized configuration example using Spring
@Configuration
public class ApplicationConfig {
@Value("${database.url}")
private String databaseUrl;
@Bean
public DataSource dataSource() {
DriverManagerDataSource dataSource = new DriverManagerDataSource();
dataSource.setUrl(databaseUrl);
return dataSource;
}
}
In this example, the @Value
annotation fetches the database URL from an external configuration source. This approach ensures clean separation of code and configuration, allowing your application to adapt seamlessly across different environments.
3. API Rate Limiting
When deploying a Java application that interacts with external APIs, developers may encounter issues related to rate limiting. Most APIs enforce limits on the number of requests within a specific time frame, and exceeding these limits can lead to connection errors.
Solution: Implementing Circuit Breaker Patterns
To mitigate API rate-limiting issues, use the Circuit Breaker pattern to handle failures gracefully. libraries like Resilience4j can be particularly helpful.
// Example of using Circuit Breaker to handle API calls
CircuitBreaker circuitBreaker = CircuitBreaker.ofDefaults("apiService");
String result = circuitBreaker.executeSupplier(() -> {
// API call logic (e.g., using RestTemplate)
});
In this snippet, a Circuit Breaker is employed around the API call logic. If the number of failed calls exceeds a threshold, the Circuit Breaker will prevent further calls for a specified period, decreasing the chance of incurring penalties for exceeding rate limits.
4. Logging and Monitoring
Without proper logging and monitoring, diagnosing issues in your deployed applications can feel like finding a needle in a haystack. Cloud platforms often introduce unique complexities that necessitate effective monitoring solutions.
Solution: Centralized Logging
Implement centralized logging solutions such as ELK Stack (Elasticsearch, Logstash, Kibana) or Splunk to collect and analyze logs from your cloud-deployed Java applications.
// Example logging configuration using Logback
<configuration>
<appender name="FILE" class="ch.qos.logback.core.FileAppender">
<file>springboot.log</file>
<encoder>
<pattern>%date %level [%thread] %logger{0} - %message%n</pattern>
</encoder>
</appender>
<root level="DEBUG">
<appender-ref ref="FILE" />
</root>
</configuration>
This Logback configuration saves your logging messages to a file named springboot.log
. Centralized logging provides better visibility into your application's performance and issues, enabling proactive monitoring.
5. Continuous Deployment Challenges
As organizations embrace continuous deployment approaches, ensuring that Java applications are easily deployable can become complicated. Automation failures can occur due to various reasons, from environment mismatches to testing errors.
Solution: Containerization
Containerization using Docker can significantly alleviate continuous deployment issues. By creating a Docker image of your Java application, you encapsulate all its dependencies and configurations.
# Sample Dockerfile for a Java application
FROM openjdk:11-jre
COPY target/myapp.jar /usr/local/myapp.jar
ENTRYPOINT ["java", "-jar", "/usr/local/myapp.jar"]
In this Dockerfile, the application is packaged into an OpenJDK container. This creates a consistent environment across all stages of deployment, minimizing the risk of environment-related issues.
Additional Resources
For further inspiration on troubleshooting deployment issues, consider reading the article titled Troubleshooting Laravel Deployment in AWS. Although it focuses on Laravel, many of the concepts discussed are applicable to Java applications deployed in cloud environments.
Lessons Learned
Deploying Java applications in the cloud can present unique challenges. From managing dependencies and configurations to ensuring effective logging and monitoring, developers must deploy a multifaceted approach to overcome common issues. By utilizing build tools, externalizing configurations, implementing Circuit Breakers, centralizing logging, and embracing containerization, you can enhance the efficiency and reliability of your Java cloud deployments.
Java remains a powerful choice in the cloud deployment arena, and with the right strategies in place, you can mitigate common issues and harness its full potential.