Deploying Java Applications on AWS: Common Pitfalls to Avoid
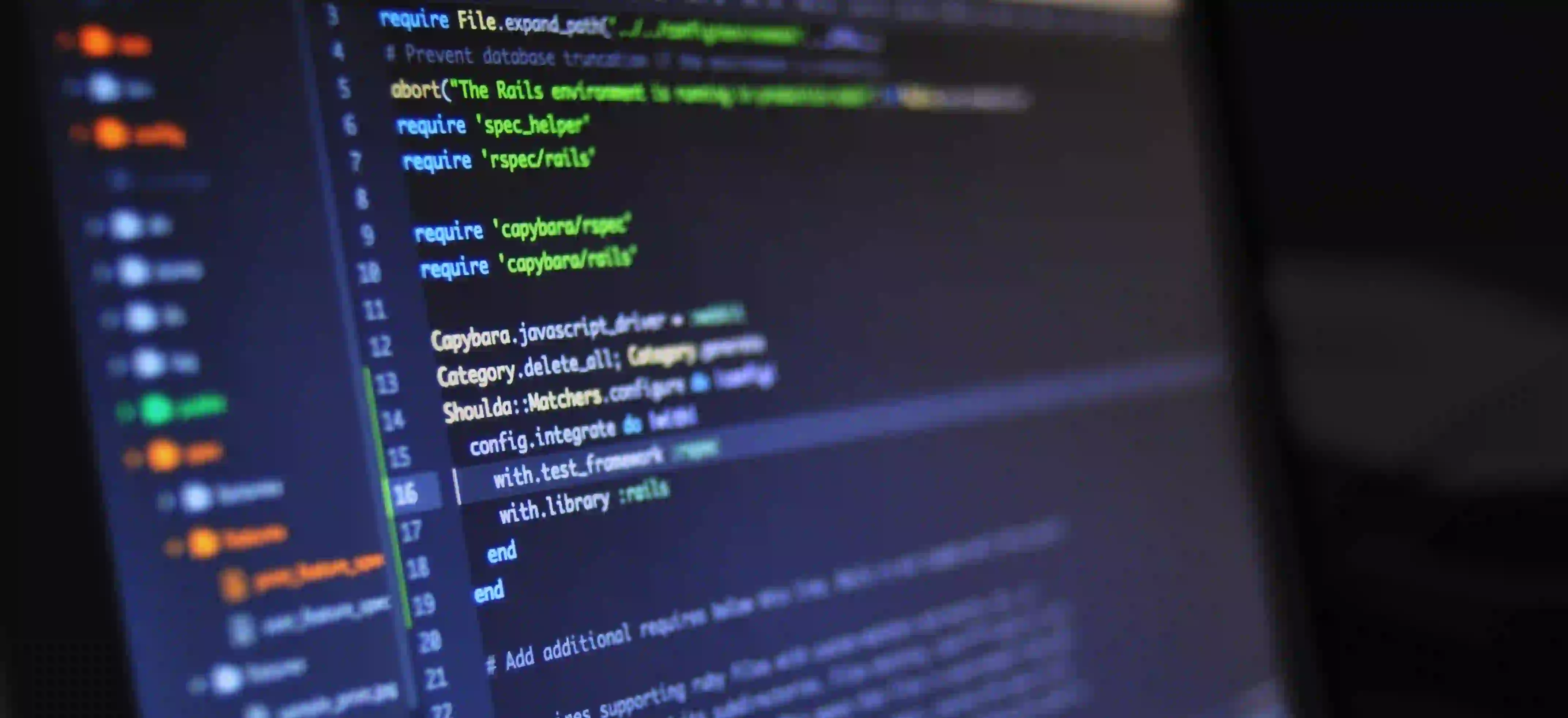
Deploying Java Applications on AWS: Common Pitfalls to Avoid
When it comes to deploying Java applications in the cloud, Amazon Web Services (AWS) stands out as a reliable option. With its extensive set of tools and services, AWS enables developers to build, deploy, and manage applications efficiently. However, like any powerful platform, it comes with its own set of challenges. In this post, we'll explore common pitfalls that you should avoid when deploying Java applications on AWS, ensuring a smoother experience for you and your team.
Why Deploy Java Applications on AWS?
Java, being one of the most popular programming languages, offers a robust ecosystem suitable for various applications, from web services to enterprise applications. Coupled with AWS, developers gain access to:
- Scalability: Automatically adjust resources based on demand.
- Security: Built-in security features to protect your applications.
- Cost-effectiveness: Pay only for the services you use.
However, inadequately managing these features can lead to issues during deployment, which brings us to the first pitfall:
1. Ignoring Resource Management
Code Example: Using AWS SDK for Java
import com.amazonaws.services.s3.AmazonS3;
import com.amazonaws.services.s3.AmazonS3ClientBuilder;
public class S3Example {
public static void main(String[] args) {
AmazonS3 s3Client = AmazonS3ClientBuilder.standard().build();
// Interact with S3
}
}
Utilizing the AWS SDK for Java simplifies interactions with AWS services, but poor resource management can lead to excessive costs. Always employ resource tagging and monitoring to keep tabs on your usage.
Why this Matters: Proper tagging enables efficient cost management and accountability, which is essential for larger teams and projects.
2. Neglecting the Appropriate Instance Type
Choosing the right EC2 instance type is critical for performance. Developers often pick a generic instance type without assessing their application's needs.
Instance Type Comparison
| Instance Type | Purpose | Memory | CPU Cores | |------------------|-------------------------------|------------------|-----------------| | t3.micro | Low-traffic applications | 1 GB | 2 | | m5.large | Moderate traffic applications | 8 GB | 2 | | c5.xlarge | Compute-intensive applications | 8 GB | 4 |
Tip: Use AWS Cost Explorer to analyze your costs and usage patterns over time.
3. Overlooking Security Best Practices
Security is paramount when deploying any application. Not securing your AWS environment properly can expose your applications to vulnerabilities.
Key Security Practices
- Use IAM roles instead of hardcoding credentials.
- Utilize security groups to control inbound and outbound traffic.
- Enable logging with AWS CloudTrail to monitor API activity.
Example: Setting up an IAM role for an EC2 instance.
{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Action": "s3:ListBucket",
"Resource": "*"
}
]
}
Why this Matters: Utilizing IAM roles minimizes risks associated with credential leakage. Adhering to the principle of least privilege boosts your application's security significantly.
4. Failing to Utilize Automation Tools
Manually configuring and deploying applications is not only cumbersome but also prone to human error. AWS offers several automation tools like AWS CodePipeline and AWS CloudFormation.
Example: Simple CloudFormation Template
AWSTemplateFormatVersion: '2010-09-09'
Resources:
MyS3Bucket:
Type: 'AWS::S3::Bucket'
Properties:
BucketName: my-java-app-bucket
Why this Matters: Infrastructure as Code (IaC) reduces manual errors and makes it easy to replicate environments. This is essential for agile development.
5. Ignoring Monitoring and Logging
Deploying your Java application is just the beginning. Monitoring your application ensures that it runs smoothly post-deployment.
Using Amazon CloudWatch
You can monitor various AWS resources using Amazon CloudWatch. For instance, you can set up alarms for CPU utilization.
CloudWatchClient client = CloudWatchClient.builder()
.region(Region.US_EAST_1)
.build();
Tip: Implement logs using AWS CloudWatch Logs to capture application-level logging.
Why this Matters: Continuous monitoring allows for proactive measures that can help avoid downtime. It can also aid in diagnosing performance bottlenecks.
6. Not Planning for Scalability
Java applications, especially in web environments, must handle varying loads. Failing to plan for scalability can lead to outages.
Auto Scaling Group Example
AutoScalingGroup asg = new AutoScalingGroup("MyAutoScalingGroup")
.desiredCapacity(2)
.minSize(1)
.maxSize(5)
.vpcZoneIdentifier(Arrays.asList("subnet-12345678"));
Why this Matters: Utilizing AWS Auto Scaling ensures that your application can handle spikes in user traffic efficiently, while also saving costs during low usage periods.
7. Not Testing in Staging Environments
Some developers directly push code to production without adequate testing, leading to unexpected failures.
Strategy to Implement
- Create a staging environment that mirrors your production environment.
- Implement CI/CD pipelines to automate testing.
Why this Matters: Testing in a staging environment reduces the risk of deploy-time failures and ensures stable releases.
Additional Resources
For developers looking to avoid pitfalls while deploying Java applications on AWS, useful reading includes the article on troubleshooting deployment issues in Laravel on AWS. It may provide insights into common deployment problems that are, at times, transferable to Java applications as well.
Lessons Learned
Deploying Java applications on AWS can greatly enhance your application's scalability, performance, and reliability. However, understanding and avoiding common pitfalls is essential for a successful deployment. By focusing on resource management, security, automation, monitoring, scalability, and testing, you can ensure a smoother and more reliable deployment process.
Remember, the goal is to enhance the user experience while minimizing potential downtime and costs. By steadily refining your deployment strategies and continuously learning, you strengthen your foundation in cloud-based solutions.
Happy coding!