Migrating Java Services: Tackling REST to GraphQL Issues
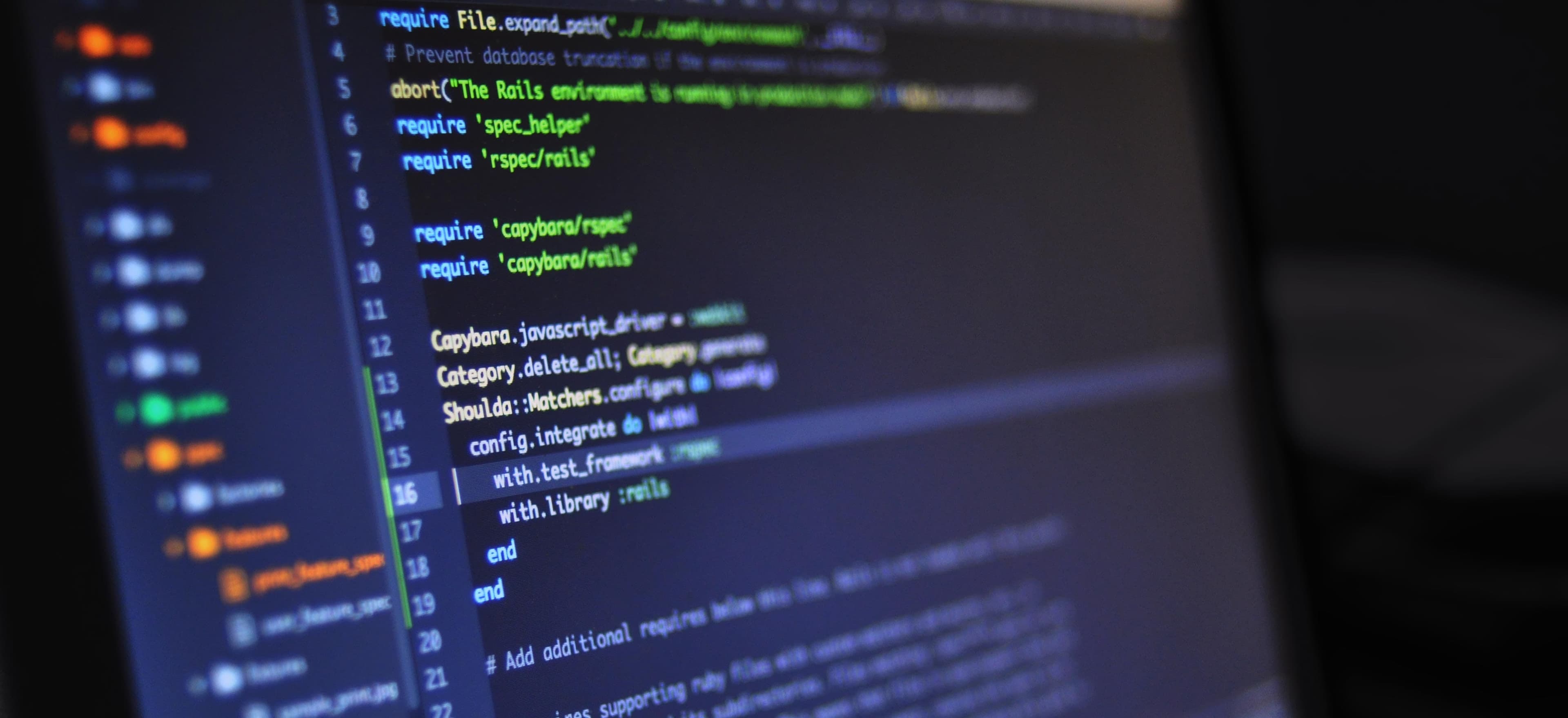
- Published on
Migrating Java Services: Tackling REST to GraphQL Issues
In today's fast-paced software environment, many organizations are considering a transition from RESTful APIs to GraphQL. The reasons for this shift are compelling: GraphQL offers a more efficient means of data retrieval, allowing clients to request exactly the data they need. However, migrating from REST to GraphQL presents its own set of challenges, especially within Java services.
In this comprehensive blog post, we will explore the common hurdles developers face during this migration process, provide practical solutions, and demonstrate relevant Java code snippets. Additionally, we will draw on insights from the article titled Superando Desafíos Comunes al Migrar de REST a GraphQL to enhance our discussion.
Understanding the Differences Between REST and GraphQL
Before delving into migration strategies, it's crucial to grasp the fundamental differences between REST and GraphQL:
-
Endpoint Structure: REST APIs revolve around multiple endpoints, each representing a resource. For example, you might have
/users
,/posts
, and/comments
. In contrast, GraphQL utilizes a single endpoint, typically/graphql
, where all types of data can be queried. -
Data Fetching: With REST, clients often over-fetch or under-fetch data due to the fixed nature of the endpoints. GraphQL allows clients to specify exactly what data they need, resulting in more efficient data usage.
-
Versioning: RESTful services require versioning as changes occur, which can complicate API management. GraphQL promotes a single evolving version, simplifying updates and ensuring backwards compatibility.
Understanding these distinctions will guide you as you navigate the complexities of migrating Java services.
Common Challenges in Migrating from REST to GraphQL
1. Schema Design
Designing a GraphQL schema can be daunting, especially for large applications. REST endpoints are usually structured around resources, but GraphQL schemas need to reflect the relationships between these resources.
Solution:
Spend time understanding your data model and how entities interact with one another. Define types for your GraphQL schema clearly. Below is a basic example of how you might define a simple GraphQL schema in Java using the graphql-java
library:
import graphql.schema.GraphQLObjectType;
GraphQLObjectType userType = GraphQLObjectType.newObject()
.name("User")
.description("A user in the system")
.field(field -> field
.name("id")
.type(GraphQLNonNull(GraphQLString)))
.field(field -> field
.name("name")
.type(GraphQLNonNull(GraphQLString)))
.build();
Why: By clearly defining types, you ensure that your schema reflects the underlying data relationships, making it more intuitive for clients to query. The GraphQLNonNull
type ensures that certain fields are mandatory, promoting data integrity.
2. Query Complexity and Performance
GraphQL's flexibility also introduces complexity concerning query performance. Clients can generate intricate queries that could cause performance bottlenecks if not managed correctly.
Solution: Implement query depth limiting, query complexity analysis, and batching.
Consider the following Java implementation for limiting query depth:
private int maxDepth = 5;
public void validateQueryDepth(GraphQLDocument document, int currentDepth) {
if (currentDepth > maxDepth) {
throw new GraphQLException("Query depth exceeds maximum limit");
}
for (Field field : document.getFields()) {
validateQueryDepth(field, currentDepth + 1);
}
}
Why: By incorporating depth validation, you proactively safeguard your server from excessively complex queries that could degrade performance. Being proactive allows you to retain control over resource consumption.
3. Caching Strategies
Caching strategies differ significantly between REST and GraphQL. REST relies heavily on HTTP caching mechanisms, while GraphQL requires custom caching solutions based on the structure of the queries.
Solution: You can incorporate a caching layer that understands GraphQL queries or utilize libraries like Apollo Client for caching on the client side.
Here’s a simple example showing how to cache results based on GraphQL queries in a Java service using an in-memory cache:
import com.github.benmanes.caffeine.cache.Cache;
import com.github.benmanes.caffeine.cache.Caffeine;
Cache<String, Object> cache = Caffeine.newBuilder()
.maximumSize(10_000)
.expireAfterWrite(5, TimeUnit.MINUTES)
.build();
public Object queryGraphQL(String query) {
return cache.get(query, k -> executeGraphQLQuery(k));
}
private Object executeGraphQLQuery(String query) {
// Logic to execute the query
}
Why: This caching mechanism optimizes performance by reducing redundant queries to the database, allowing your service to respond faster to client requests.
4. Security Concerns
Transitioning to GraphQL can expose services to new security risks. Clients can craft complex queries that unauthorized data access.
Solution: Use middleware for authentication and authorization. Each GraphQL resolver should be secured based on the user's permissions.
Here’s a simple example of a secure resolver:
import graphql.schema.DataFetcher;
import graphql.schema.DataFetchingEnvironment;
DataFetcher<User> userFetcher = env -> {
User user = getUserFromDb(env.getArgument("id"));
authenticateUser(env.getContext().getUser(), user); // Ensure the user has access
return user;
};
Why: Securing your data access ensures that only authorized clients can retrieve specific data, thus protecting sensitive information. The use of middleware dramatically simplifies permission management.
5. Tooling and Best Practices
A successful migration involves adopting the right tools and following best practices. Popular tools such as Apollo, GraphiQL, and Postman can help streamline the development process.
- Design with Clients in Mind: Always consider what data clients might need and tailor your schema accordingly.
- Test Queries and Mutations: Ensure your schema effectively supports the queries and mutations expected by the clients. Implement unit and integration tests to validate.
Setting up a simple testing scenario might look something like this:
@GraphQLTest
public class UserQueryTest {
@Autowired
private GraphQL graphQL;
@Test
void testGetUser() {
String query = "{ user(id: \"1\") { id, name } }";
ExecutionInput executionInput = ExecutionInput.newBuilder().query(query).build();
ExecutionResult result = graphQL.execute(executionInput);
assertNotNull(result.getData());
}
}
Why: Testing ensures your GraphQL implementation remains stable and behaves as expected. This practice is vital to fostering confidence both within your team and with clients.
A Final Look
Migrating from REST to GraphQL can be a challenging endeavor, especially within Java services. However, by understanding the intricacies of both architectures and employing effective solutions, organizations can embrace GraphQL's advantages while mitigating risks.
For a thorough examination of common issues encountered during this migration, refer to the insightful article "Superando Desafíos Comunes al Migrar de REST a GraphQL".
The world of software development is increasingly leaning towards GraphQL, and being well-prepared can make all the difference as you pave your way into the future of API development.
Checkout our other articles