Migrating from REST to GraphQL: Key Java-based Solutions
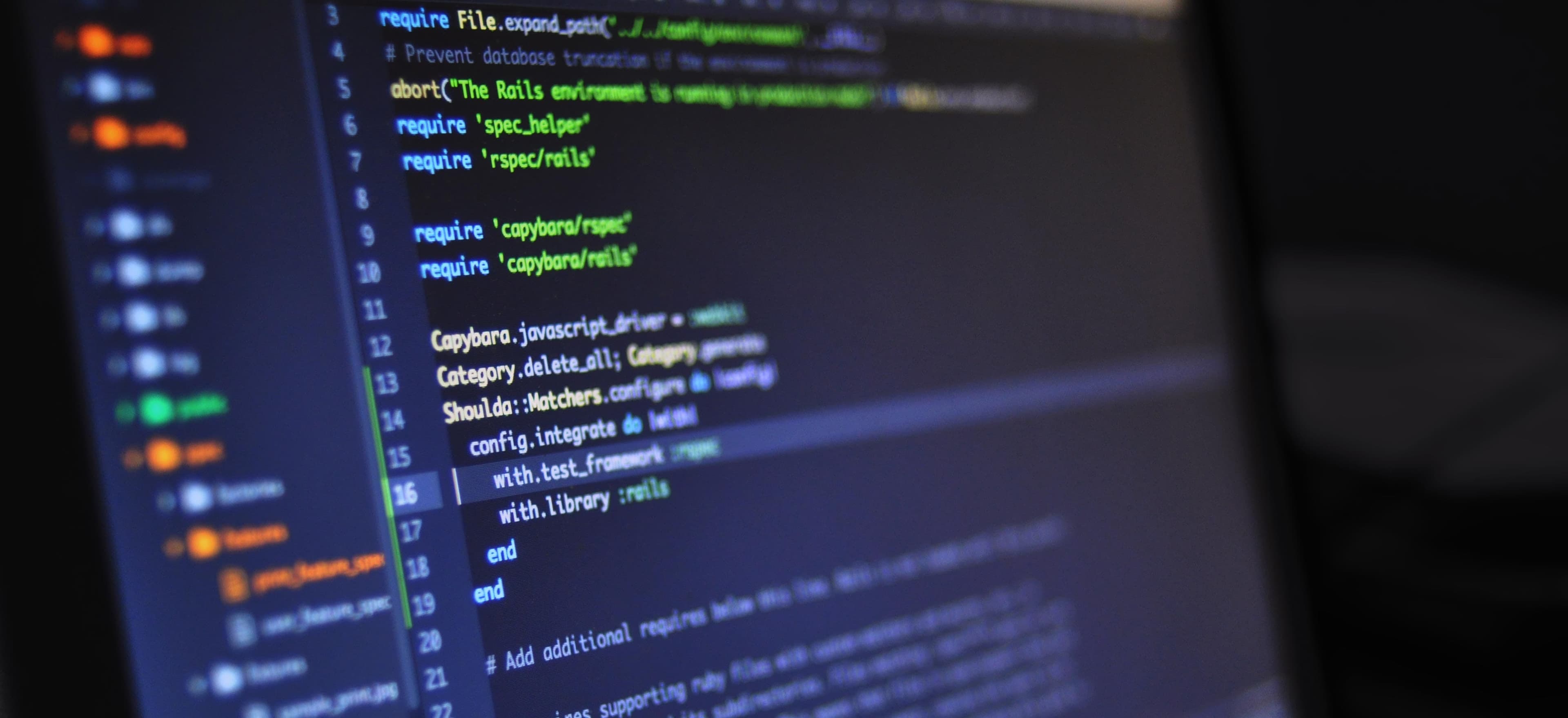
- Published on
Migrating from REST to GraphQL: Key Java-based Solutions
In the rapidly evolving landscape of web services, the shift from REST (Representational State Transfer) to GraphQL has captured the attention of developers and organizations looking for more efficient data-fetching mechanisms. This blog post navigates through the various aspects of migrating from REST to GraphQL using Java, discussing actionable solutions, code samples, and best practices.
Understanding REST and GraphQL
REST
REST has been a popular choice for designing networked applications. It follows a straightforward architecture style that relies on standard HTTP methods. However, REST can lead to over-fetching or under-fetching of data, resulting in sub-optimal performance and user experience.
GraphQL
In contrast, GraphQL allows clients to request exactly the data they need. This flexibility reduces wasted bandwidth and increases responsiveness. However, it also introduces complexity, especially when transitioning from a REST architecture.
Why Migrate from REST to GraphQL?
The switch from REST to GraphQL offers numerous benefits, including:
- Efficient Data Retrieval: Clients can request precisely what they need.
- Single Endpoint: Unlike REST, which usually requires multiple endpoints, GraphQL allows fetching data from a single endpoint.
- Strongly Typed Schema: GraphQL uses a schema to define data types, providing more predictable responses.
- Versioning: With GraphQL, you don’t need to version your API as frequently as with REST.
For a deeper understanding of common challenges faced during migration, check out Superando Desafíos Comunes al Migrar de REST a GraphQL.
Java-based Solutions for Migration
When considering a migration strategy, there are several Java frameworks and libraries that can facilitate the transition from REST to GraphQL.
1. Spring Boot with GraphQL
Spring Boot is a popular choice for building Java applications, and with the help of Spring GraphQL, the migration becomes more seamless.
Setting Up Spring Boot with GraphQL
First, you need to include the necessary dependencies in your pom.xml
:
<dependency>
<groupId>com.graphql-java-kickstart</groupId>
<artifactId>graphql-spring-boot-starter</artifactId>
<version>12.0.0</version>
</dependency>
This will allow you to leverage the power of GraphQL within your Spring Boot application.
Creating a GraphQL Schema
In GraphQL, you define how clients can interact with your data through a schema. Here's a simple example:
type Query {
hello: String
}
This schema indicates that clients can fetch a "hello" field, which will return a string.
Implementing the Query
Next, create a resolver to handle the query:
import org.springframework.graphql.data.method.annotation.QueryMapping;
import org.springframework.stereotype.Controller;
@Controller
public class HelloController {
@QueryMapping
public String hello() {
return "Hello, GraphQL!";
}
}
This implementation allows you to fetch a simple hello message using GraphQL. The @QueryMapping
annotation connects the GraphQL "hello" query to our Java method.
2. Using GraphQL Java
If you're looking for a lower-level implementation, GraphQL Java is a robust option that offers more control.
Setting Up GraphQL Java
Add the GraphQL Java dependency:
<dependency>
<groupId>com.graphql-java-kickstart</groupId>
<artifactId>graphql-java</artifactId>
<version>17.3</version>
</dependency>
Defining GraphQL Schema Programmatically
You can create a schema programmatically in Java:
import graphql.schema.GraphQLObjectType;
import graphql.schema.GraphQLSchema;
public class SchemaProvider {
public GraphQLSchema getSchema() {
GraphQLObjectType queryType = GraphQLObjectType.newObject()
.name("Query")
.field(field -> field
.name("hello")
.type(GraphQLString)
.dataFetcher(environment -> "Hello, World!"))
.build();
return GraphQLSchema.newSchema()
.query(queryType)
.build();
}
}
This code defines a simple GraphQL schema with a single query that returns a "hello" message.
3. Handling Queries and Mutations
While fetching data (queries) is essential, you should also consider how to modify data (mutations). Here's how you can add a mutation to your schema:
type Mutation {
createMessage(content: String): String
}
And the corresponding resolver:
public class MessageController {
@MutationMapping
public String createMessage(@Argument String content) {
// Imagine saving the message to a database here
return content;
}
}
4. Error Handling and Security
Migrating to GraphQL involves addressing new challenges, including error handling and security considerations.
GraphQL provides detailed error messages that can expose vulnerabilities, so it's crucial to standardize errors and avoid revealing sensitive information. Implement custom error handlers in your resolvers to manage exceptions gracefully.
For security, consider implementing authentication and authorization mechanisms. This can be approached through JWT (JSON Web Tokens) or OAuth. It's essential to ensure that sensitive data isn’t exposed in responses based on user roles.
Performance Considerations
GraphQL's flexibility can sometimes lead to performance issues, especially if clients make complex queries.
Query Complexity Analysis
Perform analysis on incoming queries and implement query complexity limits. It prevents malicious users from executing overly complex queries which can lead to Denial-of-Service (DoS).
Caching Strategies
Implement caching strategies for your GraphQL queries to improve performance. Utilize tools like Apollo Client for caching in the front-end and consider server-side caching to reduce load on your database.
Closing the Chapter
Migrating from REST to GraphQL offers significant benefits, but it also comes with unique challenges. By leveraging Java frameworks like Spring Boot and GraphQL Java, developers can create efficient APIs that align well with modern application needs.
Through careful planning and implementation of queries, mutations, error handling, and security measures, you can smoothly transition to a GraphQL architecture.
For further insights into the migration process, revisit Superando Desafíos Comunes al Migrar de REST a GraphQL to discover common pitfalls and best practices.
By keeping these strategies in mind, you'll not only enhance your application's performance but also position your team to meet evolving data requirements with confidence. Happy coding!