Essential Java Practices for Building Robust Emergency Kits
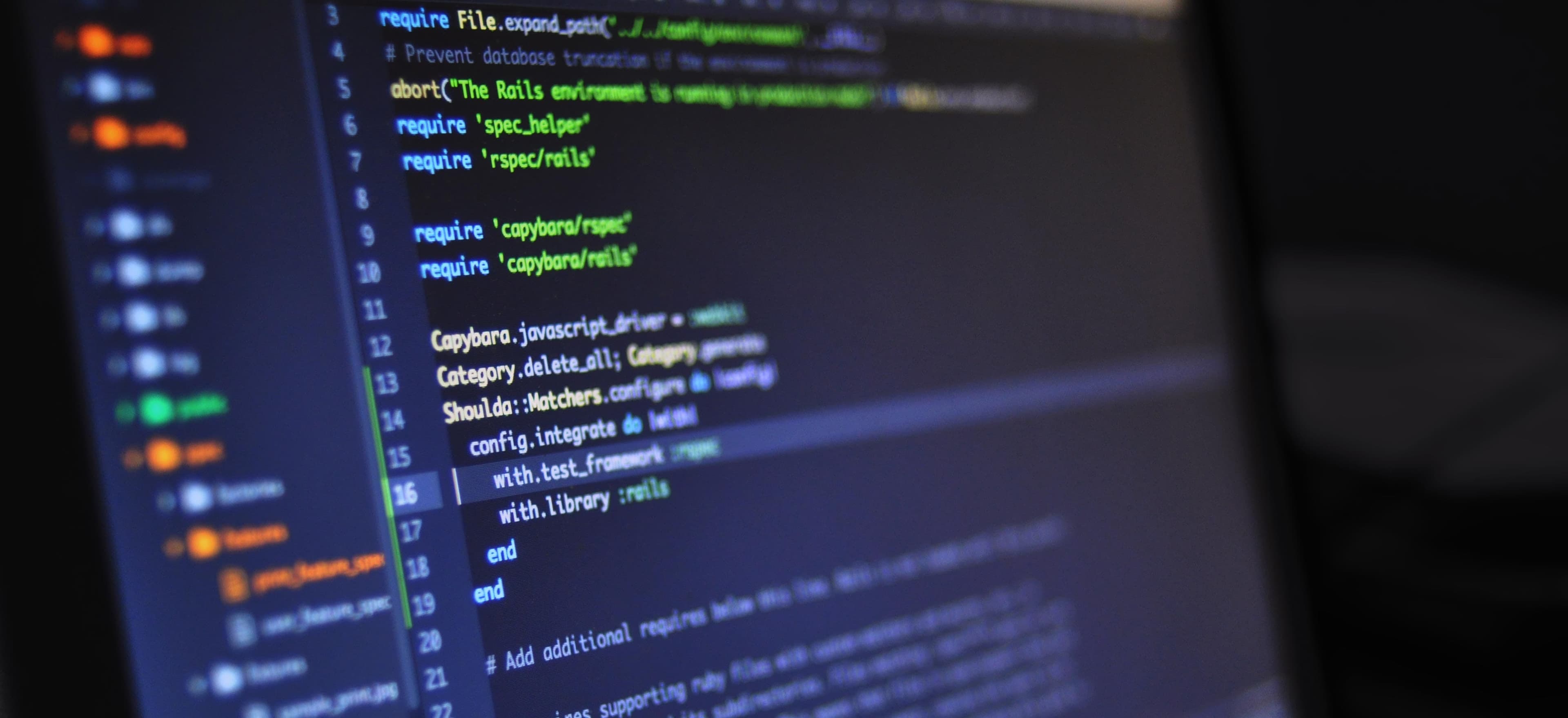
- Published on
Essential Java Practices for Building Robust Emergency Kits
In an increasingly unpredictable world, being prepared for emergencies is paramount. Whether you are a seasoned prepper or just starting out, understanding how to effectively manage, organize, and implement an emergency kit can make a significant difference. In this blog post, we will explore essential Java practices that can help you build robust applications focusing on emergency kits. Our discussion will shift between comprehensive insights and succinct guidance to keep you engaged.
Why Use Java for Emergency Kit Management?
Java is a versatile, platform-independent language that has been widely utilized in various applications, including those focused on emergency management. Its object-oriented programming paradigm allows for clear organization and manageable code, making it an excellent choice for building software that handles emergency kits. With Java, you can create applications that track resources, assess needs, and even provide essential updates in crisis situations.
Key Features of Java to Consider
-
Platform Independence: Java runs on any device with the Java Virtual Machine (JVM). This means your emergency management application can work across various platforms, ensuring it is accessible when you need it most.
-
Robust Standard Libraries: Java has an extensive suite of libraries that can simplify tasks such as networking, file I/O, and data manipulation.
-
Strong Community Support: With millions of developers worldwide, finding support and resources is easier than ever. For instance, you can integrate guidance from resources like "Outfitting Your Kit: Avoiding Common Prepper Medical Mistakes" available at youvswild.com/blog/prepper-medical-mistakes.
Designing Your Emergency Kit Application
Step 1: Define Your Requirements
Before diving into coding, clearly outline the requirements of your application. What essential features do you want? Some potential functionalities might include:
- Item tracking
- Reminders for expiration dates
- Inventory reports
Step 2: Drafting the Class Structure
Good design starts with a solid foundation. Here is a simple representation of how you might structure an emergency kit application using classes.
public class EmergencyKit {
private String kitName;
private List<Supply> supplies;
public EmergencyKit(String kitName) {
this.kitName = kitName;
this.supplies = new ArrayList<>();
}
public void addSupply(Supply supply) {
supplies.add(supply);
}
public List<Supply> getSupplies() {
return supplies;
}
}
Why this structure?
- Encapsulation: The
EmergencyKit
class keeps kit details and supply management internal, promoting data integrity. - Scalability: By using lists, you can easily add more supplies.
Step 3: Supply Class
Next, let’s define what a Supply
class might look like:
public class Supply {
private String name;
private int quantity;
private LocalDate expirationDate;
public Supply(String name, int quantity, LocalDate expirationDate) {
this.name = name;
this.quantity = quantity;
this.expirationDate = expirationDate;
}
public String getName() {
return name;
}
public int getQuantity() {
return quantity;
}
public LocalDate getExpirationDate() {
return expirationDate;
}
}
Why include expiration dates?
- Preparedness: Knowing when supplies expire can help you maintain a relevant kit. This ties back into the importance of avoiding common prepper medical mistakes.
Step 4: Implementing a Basic User Interface
Providing an easy-to-use interface is critical. While a graphical user interface (GUI) can be complex, a simple console-based option can still serve your purpose effectively in the beginning.
import java.util.Scanner;
public class EmergencyKitManager {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
EmergencyKit kit = new EmergencyKit("My Emergency Kit");
System.out.println("Enter a supply name: ");
String name = scanner.nextLine();
System.out.println("Enter quantity: ");
int quantity = scanner.nextInt();
System.out.println("Enter expiration date (YYYY-MM-DD): ");
String date = scanner.next();
LocalDate expirationDate = LocalDate.parse(date);
kit.addSupply(new Supply(name, quantity, expirationDate));
System.out.println("Supplies in your kit:");
for (Supply supply : kit.getSupplies()) {
System.out.println(supply.getName() + ": " + supply.getQuantity() + " (Expires on " + supply.getExpirationDate() + ")");
}
scanner.close();
}
}
Why use a console UI?
- Simplicity: Implementing a console application allows you to test your features quickly before moving to a more complex interface.
- Immediate Feedback: Users can see their input reflected right away, facilitating learning.
Step 5: Future Features - Enhancing the Application
Once you have a working application, consider these additional enhancements:
- Database Integration: Store supplies in a database for persistence across sessions.
// Code for connecting to a database and inserting items would go here.
- Web Application: Transition to a Java-based web application using frameworks like Spring to allow for remote access.
The Bottom Line
Java provides a robust framework for building emergency kit management applications. By following the strategies outlined in this blog post, you can create a system tailored to your readiness needs. When designing such tools, always avoid the common mistakes mentioned in articles like "Outfitting Your Kit: Avoiding Common Prepper Medical Mistakes" at youvswild.com/blog/prepper-medical-mistakes.
Whether you're planning for a natural disaster or just want to stay prepared, leveraging Java can streamline your efforts. Feel free to expand upon the functionalities and design as you see fit, ensuring your emergency kit is both effective and efficient. Happy coding!
Checkout our other articles