Mastering Chemistry Concepts: Overcoming Java's Learning Hurdles
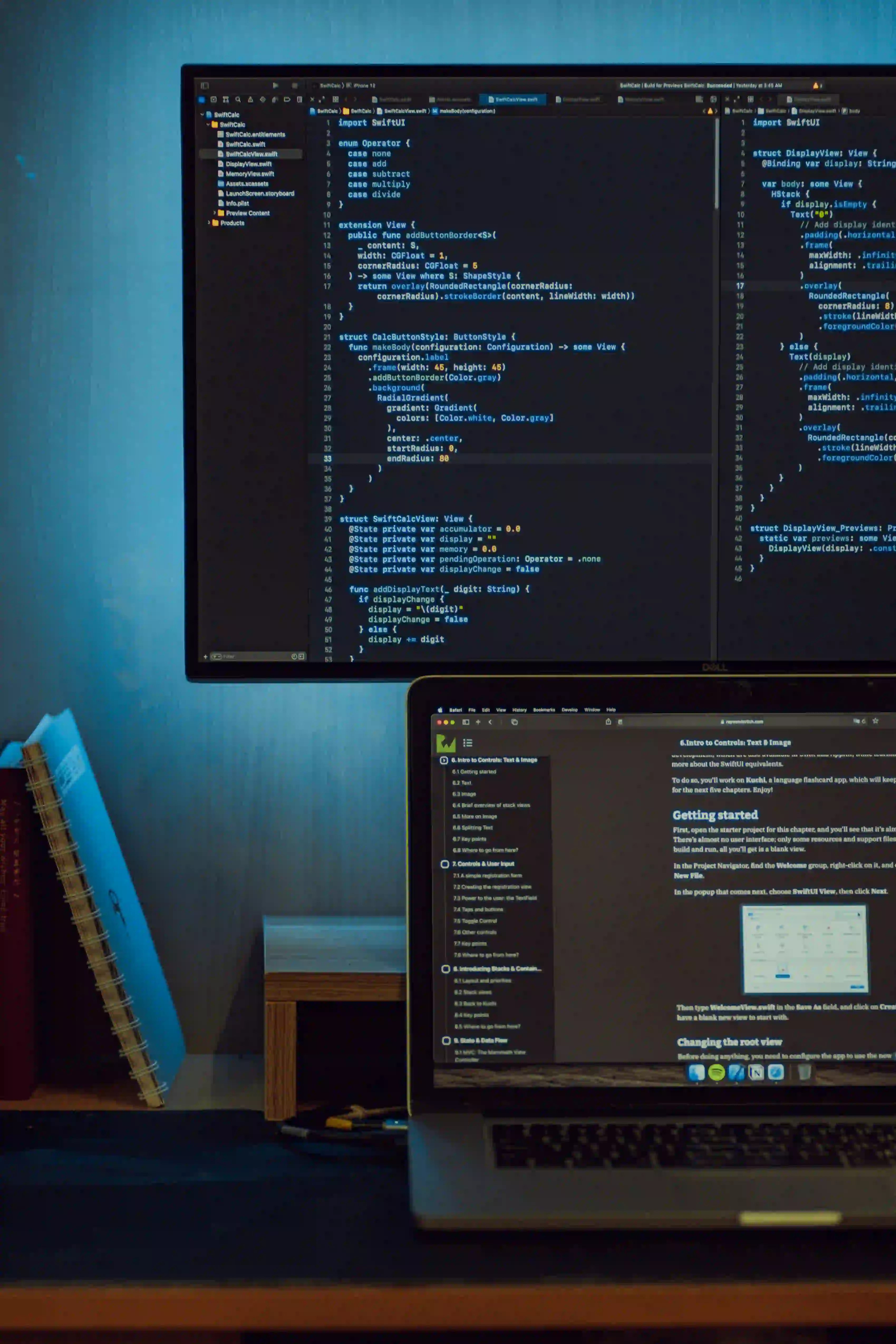
Mastering Chemistry Concepts: Overcoming Java's Learning Hurdles
When transitioning from traditional chemistry concepts to programming with Java, many students and professionals face several challenges. The intricacies of Java may remind one of complicated chemistry theories, which can sometimes feel overwhelming. In light of that, this blog aims to demystify Java by drawing parallels with chemistry while providing informative examples. By doing so, we can address similar hurdles faced while learning both disciplines.
The Importance of Understanding Fundamentals
Before delving into Java, it's crucial to grasp the basics of programming. Just as in chemistry, where a solid understanding of atomic structure is essential for grasping more complex reactions, understanding the foundational elements of Java will pave the way for mastering the language.
Java is an object-oriented programming language that emphasizes reusability, encapsulation, and inheritance—concepts that may resonate with the varied relationships and classifications in chemistry.
Key Concepts in Java
-
Classes and Objects
In Java, everything revolves around classes and objects. A class is essentially a blueprint for creating objects. Similarly, in chemistry, molecules can be considered as "objects" formed from atoms defined by their "class," or the type of element.Here's a simple Java class example:
☕snippet.javapublic class Molecule { String name; int molecularWeight; Molecule(String name, int molecularWeight) { this.name = name; this.molecularWeight = molecularWeight; } }
Why is this important?
This code snippet shows how to define a new class named 'Molecule.' Understanding this process is fundamental because you will encounter many similar definitions in Java as you create more complex programs. -
Methods
A method in Java is a function that belongs to a class. Methods allow us to perform actions or calculations.Consider the method below, which calculates the molar mass of a compound:
☕snippet.javapublic double calculateMolarMass(int[] atomicWeights) { double molarMass = 0; for (int weight : atomicWeights) { molarMass += weight; } return molarMass; }
Why bother?
This method sums the atomic weights passed to it in an array. Knowing how and when to use methods will simplify your code and enable cleaner, more modular programming—much like balancing equations in chemistry leads to clearer reactions. -
Inheritance
Inheritance allows a new class to inherit properties and methods from an existing class. This is akin to understanding common groups of chemical compounds based on their similarities.Here is an example:
☕snippet.javapublic class OrganicCompound extends Molecule { boolean isFlammable; OrganicCompound(String name, int molecularWeight, boolean isFlammable) { super(name, molecularWeight); this.isFlammable = isFlammable; } }
The significance?
The classOrganicCompound
inherits characteristics fromMolecule
while introducing additional features, much like how organic compounds have unique properties but share distinct elemental combinations.
Debugging: The Chemistry of Finding Errors
Just as a chemist meticulously conducts experiments to identify issues in reactions, debugging in Java is a critical skill. When code errors arise, systematic troubleshooting is necessary.
Consider a simple program that adds elements to an array. Here’s a faulty snippet:
public static void main(String[] args) {
int[] numbers = new int[5];
for (int i = 0; i <= numbers.length; i++) {
numbers[i] = i; // This will throw an ArrayIndexOutOfBoundsException
}
}
Find and Correct Errors
Identifying the Error:
The error arises from attempting to access an index that is out of bounds. Remember, array indices start at zero.
Correcting the Code:
for (int i = 0; i < numbers.length; i++) {
numbers[i] = i; // Corrected to '<' instead of '<='
}
Why debugging is like chemistry:
Whether it’s identifying a chemical imbalance in a solution or isolating a bug in a program, systematic error detection forms the backbone of successful experimentation.
Further Learning Resources
For those grappling with chemistry concepts and finding it difficult, I recommend checking out the article "Chemie leicht gemacht: Tipps gegen Verständnisprobleme" at lernflux.com/post/chemie-leicht-gemacht-tipps-gegen-verstandnisprobleme. This resource provides tips to help streamline your learning process, providing guidance that can equally apply to programming.
When learning Java, don't forget to explore additional languages and tools as they can enhance your overall understanding and problem-solving capabilities. Resources like books, video tutorials, and online platforms can accelerate your grasp of concepts.
Practice Makes Perfect
Like memorizing the periodic table, proficiency in Java requires practice. Here’s a straightforward exercise to try out:
- Create a
Chemical
Class:
Include properties such as name, formula, and molecular weight. - Add Methods:
Implement methods to derive molar mass based on the number of atoms of each element. - Inheritance:
Create subclasses for different types of compounds, like acids and bases, that extend theChemical
class.
In Conclusion, Here is What Matters
By adopting a methodical approach to learning both chemistry and Java, you can mitigate common hurdles. Both fields require patience and continual practice. Remember, programming in Java is not unlike conducting chemistry experiments in the lab: it demands precision, critical thinking, and a willingness to troubleshoot.
As you continue to learn, keep these parallels in mind. They may just provide that extra clarity needed to overcome obstacles. Happy coding!