Java Frameworks: Building First Aid Apps for Preparedness
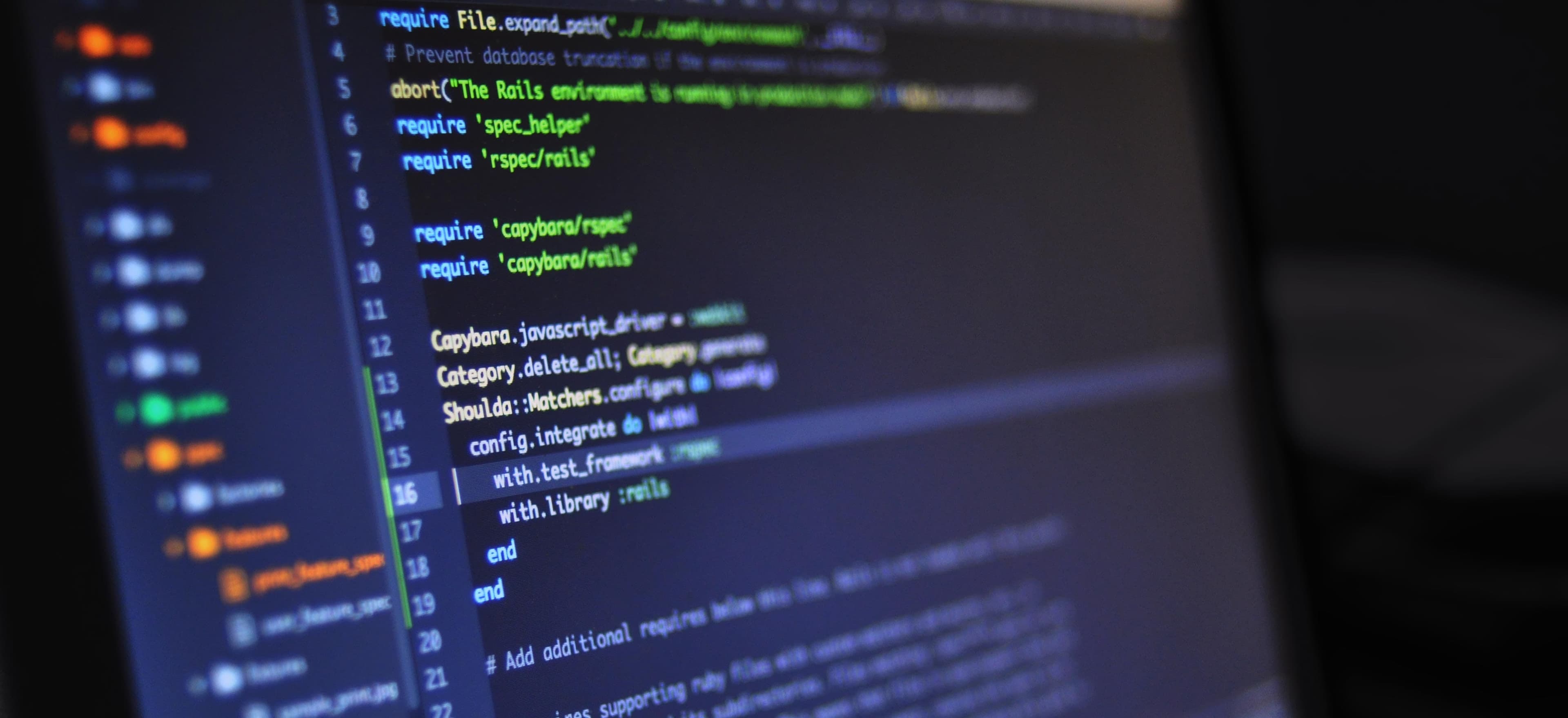
- Published on
Java Frameworks: Building First Aid Apps for Preparedness
In the ever-evolving world of technology, Java remains one of the most dominant programming languages. Its versatility and robustness make it an excellent choice for building applications across various sectors. One area that sees increased interest, especially among preppers and survival enthusiasts, is the development of first aid applications. This is a vital toolset that can assist individuals in emergency situations, ensuring they are better equipped to handle medical emergencies effectively.
In this blog post, we will explore Java frameworks that facilitate building first aid apps, discuss relevant design patterns, and provide examples to get you started. Additionally, we will reference best practices found in the article "Outfitting Your Kit: Avoiding Common Prepper Medical Mistakes" from YouVsWild, which you can read here.
Why Build First Aid Apps?
First aid apps provide users with immediate access to critical information during medical emergencies. As a prepper, having reliable and user-friendly medical apps can be a gamechanger when faced with crises. These apps can offer:
- Instant access to first aid procedures: Helps users react promptly.
- Emergency contact features: Connects directly with local authorities or subscription-based medical services.
- Educational resources: Teaches basics of first aid and preparedness.
Choosing the Right Java Framework
Selecting the proper Java framework for your application is paramount to ensuring scalability, maintainability, and an excellent user experience. Here are some popular Java frameworks that can streamline your app’s development:
1. Spring Boot
Spring Boot is a microservices framework that simplifies the development of Java applications. It’s remarkably user-friendly for building RESTful APIs—ideal for first aid apps that need to fetch information from a server.
Example: Setting Up a Basic Spring Boot Application
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class FirstAidApp {
public static void main(String[] args) {
SpringApplication.run(FirstAidApp.class, args);
}
}
Why Spring Boot?
- Auto-configuration: Eliminates the need for complex setup.
- Microservices capabilities: Supports REST APIs, essential for interactive health resources.
- Ease of testing: Comes with built-in testing tools to ensure your code functions as intended.
2. JavaFX
For those focusing on developing desktop applications, JavaFX offers modern GUI components and is incredibly suitable for building interactive layouts for first aid applications.
Example: Creating a Basic JavaFX Application
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class FirstAidAppGUI extends Application {
@Override
public void start(Stage primaryStage) {
Button btn = new Button("Emergency Instructions");
btn.setOnAction(event -> System.out.println("Display emergency instructions"));
StackPane root = new StackPane();
root.getChildren().add(btn);
Scene scene = new Scene(root, 300, 250);
primaryStage.setTitle("First Aid App");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Why JavaFX?
- Rich UI components: Offers advanced UI elements that can enhance user experience.
- Cross-platform compatibility: Can run on different operating systems.
- Support for media and web: Allows you to integrate videos for instructional content.
3. Hibernate
For apps that require data management, Hibernate is a strong ORM (Object-Relational Mapping) tool that makes it easier to interact with databases.
Example: Basic Entity Setup with Hibernate
import javax.persistence.Entity;
import javax.persistence.Id;
@Entity
public class FirstAidProcedure {
@Id
private Long id;
private String name;
private String description;
// Getters and setters
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
}
Why Hibernate?
- Eliminates boilerplate code: Reduces the amount of code needed to perform CRUD operations.
- Lazy loading: Optimizes the performance of data retrieval.
- Supports complex queries: Easily create complex queries for your medical data.
Additional Features to Consider
When developing a first aid app, consider the following features to improve user accessibility and engagement:
1. User-friendly Interface
Ensure that your app's navigation is intuitive. Users may be under stress during emergencies; thus, clear labeling, button sizes, and colors play a crucial role in user experience.
2. Offline Functionality
Consider implementing offline capabilities, so users can access key information without needing an internet connection. Utilize local databases with Hibernate for offline data.
3. Educational Content
Incorporate instructional videos and articles on first aid techniques. Use a Java binding library like Javasound to display audio cues for hands-free scenarios.
4. Emergency Contacts Integration
Include features to allow users to save and access emergency contacts easily. This may involve integrating with phone directories or utilizing the phone's SMS capabilities.
Testing Your App
The significance of testing cannot be understated. Utilize tools such as JUnit for unit testing and integration testing to ensure that your application functions correctly across all supported devices.
Example: Basic JUnit Test Case
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class FirstAidProcedureTest {
@Test
public void testGetName() {
FirstAidProcedure procedure = new FirstAidProcedure();
procedure.setName("CPR");
assertEquals("CPR", procedure.getName());
}
}
Why Testing Matters?
- Detects issues early: Helps identify bugs before deployment.
- Ensures functionality: Validates that all features operate as expected.
- Enhances reliability: Builds trust with your users.
My Closing Thoughts on the Matter
The landscape of first aid and preparedness has changed significantly over the years, and building a Java-based first aid application can make an immense difference. By utilizing frameworks such as Spring Boot, JavaFX, and Hibernate, you can develop comprehensive and user-friendly solutions.
As you embark on this project, don’t forget the critical medical knowledge. Reference the insights shared in the article "Outfitting Your Kit: Avoiding Common Prepper Medical Mistakes" by visiting youvswild.com/blog/prepper-medical-mistakes for crucial lessons on ensuring you're well-prepared for emergencies.
Happy coding, and may your coding projects not just inspire, but potentially save lives!
Checkout our other articles