Solving Java Complexity: Tips for Clearer Code Understanding
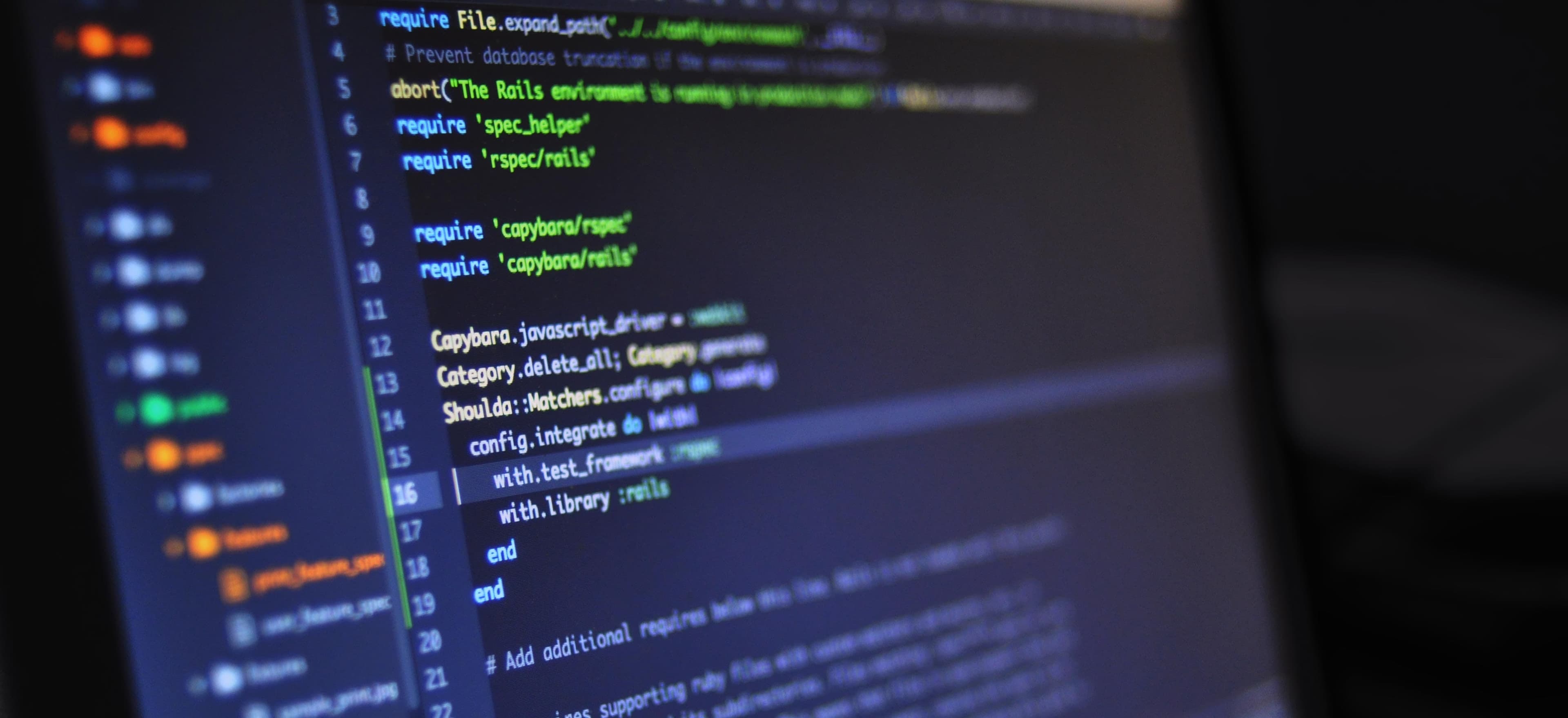
- Published on
Solving Java Complexity: Tips for Clearer Code Understanding
Java is a powerful programming language widely used for its readability and robust feature set. However, as applications grow in complexity, developers often face challenges that can lead to misunderstandings and miscommunications within teams. In this article, we'll explore ways to simplify the complexities of Java programming, enabling clearer code understanding. We will provide practical tips along with code snippets illustrating key concepts.
Understanding the Importance of Readable Code
The foundation of any successful Java application is clean, maintainable, and understandable code. Readable code reduces the cognitive load on the developers, which makes onboarding new team members easier, minimizes the potential for bugs, and enhances overall productivity.
Consider the following code snippet, which retrieves a user's information from a database:
public User getUserById(int userId) {
User user = null;
Connection connection = null;
PreparedStatement statement = null;
ResultSet resultSet = null;
try {
connection = DriverManager.getConnection(DB_URL, USER, PASS);
statement = connection.prepareStatement("SELECT * FROM users WHERE id = ?");
statement.setInt(1, userId);
resultSet = statement.executeQuery();
if (resultSet.next()) {
user = new User(resultSet.getInt("id"), resultSet.getString("name"), resultSet.getString("email"));
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (resultSet != null) resultSet.close();
if (statement != null) statement.close();
if (connection != null) connection.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
return user;
}
Commentary
- Connection Management: Always close database connections, prepared statements, and result sets to prevent memory leaks.
- Error Handling: Consider more robust error handling rather than just printing the stack trace.
- Code Clarity: Keep your code structured and well-organized for better future reference.
Best Practices for Clearer Code
1. Follow Naming Conventions
Using meaningful and descriptive names for classes, methods, and variables can significantly improve code readability. For instance, a variable named u
offers little insight compared to userName
.
Example:
String userName; // Clear
String u; // Vague
2. Break Down Complex Methods
Large methods can be daunting. Decomposing a complex method into smaller, manageable ones improves clarity and makes debugging simpler.
Example:
Instead of this long method:
public void processUserData(User user) {
// Validation
if (user.getName() == null) return;
// Formatting
String formattedName = user.getName().trim().toUpperCase();
// Save to database
saveUser(formattedName);
}
Break it into smaller methods:
public void processUserData(User user) {
if (isValidUser(user)) {
String formattedName = formatName(user.getName());
saveUser(formattedName);
}
}
private boolean isValidUser(User user) {
return user.getName() != null;
}
private String formatName(String name) {
return name.trim().toUpperCase();
}
3. Utilize Java Documentation
Java supports documentation comments that allow developers to explain classes, methods, and other elements. Adding Javadoc comments helps others understand the purpose and usage of your code.
Example:
/**
* Retrieves a user by their ID from the database.
*
* @param userId the ID of the user to retrieve
* @return the User object, or null if not found
*/
public User getUserById(int userId) {
// method implementation
}
Emphasizing Object-Oriented Principles
Java is inherently an object-oriented language, and adhering to its principles can lead to cleaner, more modular code. Here are some core principles to focus on:
1. Encapsulation
Encapsulation involves bundling the data (variables) with the methods that operate on that data. This can mitigate external interference and misuse of methods.
Example:
public class User {
private String name;
private String email;
// Constructor
public User(String name, String email) {
this.name = name;
this.email = email;
}
// Public getter for name
public String getName() {
return name;
}
// Public method for updating email
public void updateEmail(String newEmail) {
if (validateEmail(newEmail)) {
email = newEmail;
}
}
private boolean validateEmail(String email) {
// Email validation logic
return true; // Assume valid for this example
}
}
2. Inheritance and Polymorphism
Using inheritance allows you to create a new class by extending an existing class, and polymorphism allows a single interface to control access to a general class of actions. These principles can significantly reduce code duplication.
Example:
public class AdminUser extends User {
private int adminLevel;
public AdminUser(String name, String email, int adminLevel) {
super(name, email);
this.adminLevel = adminLevel;
}
@Override
public void updateEmail(String newEmail) {
// Admin-specific email update logic
super.updateEmail(newEmail);
// Log the update for auditing
}
// Other admin-specific methods
}
Additional Resources
For those struggling with understanding complexities in various subjects, it's essential to leverage existing articles and resources. One notable resource is the article “Chemie leicht gemacht: Tipps gegen Verständnisprobleme”. This provides tips on chemical concepts that can also metaphorically apply to programming - understanding foundational ideas to encourage clarity.
The Last Word
In Java, reducing complexity enhances understanding and maintainability. By adhering to naming conventions, breaking down complex methods, providing thorough documentation, and following object-oriented principles, we can drastically improve the clarity of our code.
Remember, clear and simple code is not only easier for others to read but also healthier for your problem-solving agility in future development. As the field of software development continues to evolve, the principles of clarity and simplicity will be more vital than ever. Applying these practices creates a collaborative environment where developers can thrive, ultimately leading to more successful projects.
Happy coding!
Checkout our other articles