Mastering Java: Key Strategies for Ansible Interviews
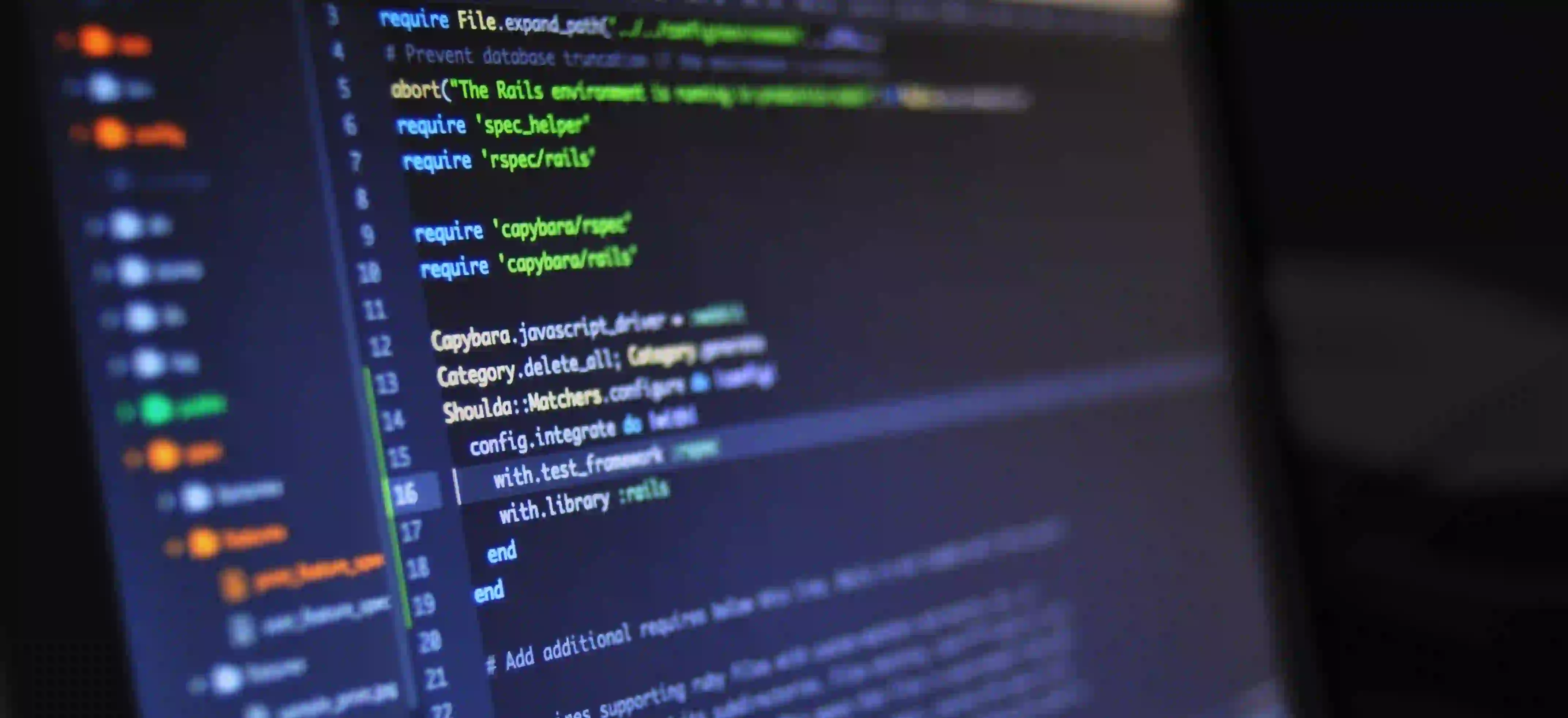
Mastering Java: Key Strategies for Ansible Interviews
As technology continues to evolve, so does the landscape of programming languages and tools that developers need to master. Java remains a staple language due to its versatility, while configuration management tools like Ansible are becoming essential, especially in DevOps roles. This article provides strategies tailored for Java developers gearing up for Ansible interviews, focusing on concepts that can bridge understanding between these two aspects.
Understanding Ansible Basics
Before diving into strategies, it’s essential to understand what Ansible is. Ansible is an open-source automation tool used for configuration management, application deployment, and task automation. It uses a simple, human-readable language based on YAML (Yet Another Markup Language) for its configuration files.
Why Learn Ansible?
- Efficiency: Automate repetitive tasks.
- Scalability: Manage a multitude of servers seamlessly.
- Simplicity: Write configurations in a straightforward language.
For Java developers, having a solid grasp of Ansible can significantly enhance your profile, as many companies look for candidates proficient in both programming and infrastructure management.
Bridging Java and Ansible
Java developers can leverage their programming skills in many ways when learning Ansible. The object-oriented principles and design patterns familiar to Java will aid in understanding Ansible's modular architecture. Below are key strategies that Java developers should consider for Ansible interviews.
1. Understand Playbooks and Modules
Ansible playbooks are the heart of Ansible automation. They contain a set of instructions in YAML format, and modules are the tools run within those playbooks.
Here’s an example of a simple playbook that installs Apache on a target machine:
---
- name: Install Apache
hosts: webservers
tasks:
- name: Install httpd
yum:
name: httpd
state: present
Why This Matters: Understanding how to structure playbooks and utilize modules is crucial. Just like Java classes encapsulate data and functions, playbooks encapsulate tasks and logic. This foundational knowledge will showcase your understanding during interviews.
2. Leverage Java’s Object-Oriented Concepts
As a Java developer, you are already familiar with concepts like encapsulation, inheritance, and polymorphism. Apply these concepts in Ansible by organizing your playbooks and roles effectively.
For example, roles can be thought of as packages in Java:
my_role/
tasks/
main.yml
handlers/
main.yml
templates/
myconfig.j2
Why This Matters: Modular roles allow code reuse just as Java packages do. During your interview, referencing your experience in building reusable components in Java can demonstrate that you are thinking like a developer in the context of automation tools.
3. Practice with Variables and Facts
Ansible allows you to use variables and gather information about target systems through facts. For a Java developer, this is similar to defining and using objects.
Here’s how you might declare a variable and use it:
---
- hosts: all
vars:
apache_port: 80
tasks:
- name: Install Apache on a specific port
template:
src: myconfig.j2
dest: /etc/httpd/conf.d/myconfig.conf
Why This Matters: Variables bring flexibility and scalability to your playbooks. By showcasing your understanding of variables, facts, and their usage in different environments, you can highlight your critical thinking and problem-solving skills.
4. Error Handling in Ansible
Error handling is crucial, much like in Java, where you use try-catch blocks. In Ansible, the ignore_errors
directive or the failed_when
clause is used.
Here’s an error-handling example:
- name: Ensure Apache is stopped
service:
name: httpd
state: stopped
ignore_errors: yes
Why This Matters: Custom error management demonstrates your ability to produce robust automation scripts. Being able to discuss error handling strategies from both Java and Ansible perspectives can provide a well-rounded view of your programming approach.
5. Familiarize Yourself with Ansible Galaxy
Ansible Galaxy is a repository for sharing roles and collections. As a Java developer, consider it akin to using libraries or frameworks in Java, such as Maven packages.
ansible-galaxy install geerlingguy.apache
Why This Matters: Understanding Ansible Galaxy and its community can set you apart in an interview. Being proactive by having your own roles or collections can display your willingness to contribute to the community.
6. Tackle Continuous Integration/Continuous Deployment (CI/CD)
Many companies are looking for candidates who are comfortable with CI/CD pipelines. As you might know, these pipelines automate the integration and deployment process.
Here's how you can integrate Ansible with CI/CD:
- name: Deploy application
hosts: app_servers
tasks:
- name: Pull latest code from repo
git:
repo: 'https://github.com/myrepo.git'
dest: /var/www/myapp
Why This Matters: Understanding how Ansible can fit into CI/CD will prepare you for discussions on deployment strategies in your interviews. Be ready to share experiences or project insights where you leveraged Ansible in a production environment.
7. Understand Inventory Files
Inventory files in Ansible can be compared to data structures in Java, where you define the components you will work with.
The simplest inventory file looks like this:
[webservers]
server1.example.com
server2.example.com
Why This Matters: Knowing how to configure your inventory effectively can save time and minimize errors. Discussing how you managed complex environments using inventory files can demonstrate your depth of knowledge in practical applications.
Bringing It All Together
For Java developers transitioning into roles involving Ansible, the key is to leverage your existing knowledge while being open to the nuances of automation. The strategies outlined above will not only help you in understanding the core aspects of Ansible but also gear you up for interviews.
Remember, confidence and clarity are essential in explaining how your programming background enriches your Ansible capabilities. In the context of preparation, you might find it beneficial to read articles such as How to Ace Ansible Interview Questions? for additional insights.
By mastering these strategies, you will position yourself as a well-rounded candidate in the ever-evolving tech landscape. Happy coding and good luck with your interviews!