Java Strategies to Prevent Cache Overload in Web Apps
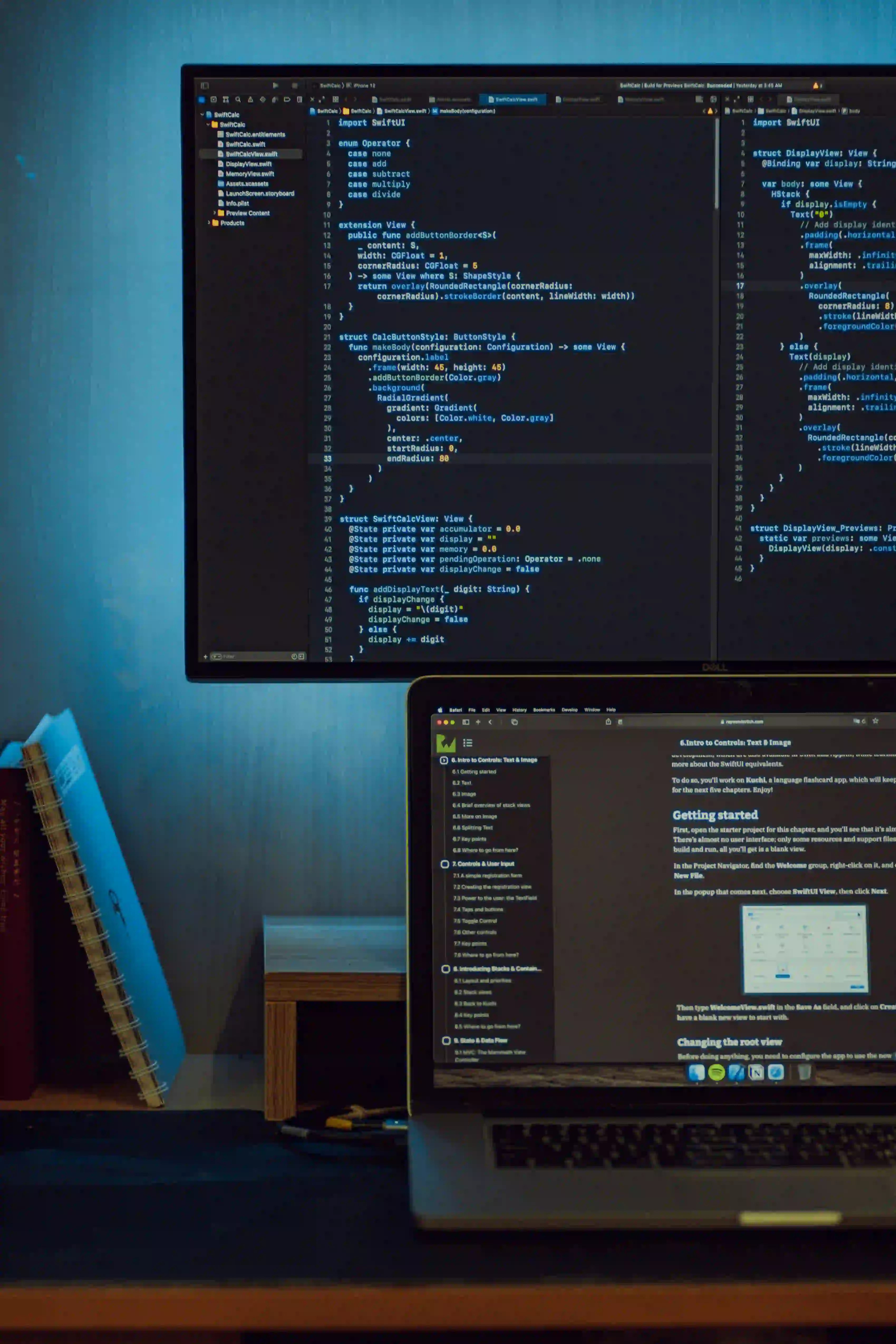
Java Strategies to Prevent Cache Overload in Web Apps
Caching is a powerful technique in web application development that can drastically reduce load times and improve user experience. However, improper caching strategies can lead to cache overload, causing performance degradation rather than enhancement. This article will discuss effective Java strategies to prevent cache overload in your web applications, ensuring smooth and efficient operations.
Understanding Cache Overload
Before diving into the strategies, it’s essential to understand what cache overload is. Cache overload occurs when there is an excessive amount of data stored in the cache, leading to reduced performance and slower response times. This situation can arise from several factors, including poor cache management, unbounded growth of data, or incorrect cache eviction policies.
Here's a brief overview of how cache overload affects a web application:
- Performance Decline: Overloaded caches can take longer to read and write data, increasing latency.
- Increased Memory Usage: A larger cache consumes more memory, potentially causing server resources to be exhausted.
- Stale Data: If data is not refreshed periodically, users may receive outdated information.
To mitigate these issues, developers can leverage several effective strategies.
1. Use an Intelligent Caching Strategy
Implement Time-Based Expiration
One straightforward method to prevent cache overload is to utilize time-based expiration. By setting expiration times, you automatically evict stale data that no longer needs to be stored in the cache.
import java.util.concurrent.TimeUnit;
import com.github.benmanes.caffeine.cache.Cache;
import com.github.benmanes.caffeine.cache.Caffeine;
public class CacheExample {
private Cache<String, String> cache = Caffeine.newBuilder()
.expireAfterWrite(10, TimeUnit.MINUTES) // Evict after 10 minutes
.maximumSize(1000) // Maximum cache size
.build();
public void put(String key, String value) {
cache.put(key, value);
}
public String get(String key) {
return cache.getIfPresent(key);
}
}
Why This Works
By implementing a time-based expiration policy, you ensure that your cache does not retain data entries longer than necessary. This method reduces memory consumption and allows for newly generated data to be cached without overflowing.
Additionally, combine this with a maximum size for the cache to avoid overloading the memory. The strategies used in Avoid Cache Overload: Optimizing NodeJS Apps can inspire your approach to maintain cache efficiency (infinitejs.com/posts/optimize-nodejs-apps-cache-avoidance).
2. Implement Least Recently Used (LRU) Eviction Policy
An LRU eviction policy is another intelligent way to handle cache storage. This policy evicts the least recently accessed items first, keeping frequently accessed items readily available.
Using the same Caffeine library in Java, you can set up an LRU policy as follows:
import com.github.benmanes.caffeine.cache.Caffeine;
import java.util.concurrent.TimeUnit;
public class LRUCache {
private Cache<String, String> cache = Caffeine.newBuilder()
.maximumSize(500) // Maximum cache size
.expireAfterAccess(5, TimeUnit.MINUTES) // Eviction based on access
.build();
public void cacheItem(String key, String value) {
cache.put(key, value);
}
public String fetchItem(String key) {
return cache.getIfPresent(key);
}
}
Why This Works
The LRU eviction strategy adapts dynamically to usage patterns. As users access certain data, these items stay in cache, while less pertinent items get evicted automatically. This direct correlation with user behavior ensures your cache remains relevant and efficiently sized.
3. Cache Granularity
Not every piece of data requires caching. Understanding what to cache is crucial to prevent redundancy and cache overload.
Identify What Data to Cache
Instead of caching everything, focus on data that:
- Is expensive to retrieve or compute.
- Is accessed frequently.
- Is static or infrequently updated.
Example Code Snippet
Below is an example of a cache implementation that selectively caches user profiles.
import java.util.HashMap;
import java.util.Map;
public class UserProfileCache {
private Map<String, UserProfile> userProfileCache = new HashMap<>();
public UserProfile getUserProfile(String userId) {
if (userProfileCache.containsKey(userId)) {
return userProfileCache.get(userId); // Utilize cached profile
} else {
UserProfile profile = fetchUserProfileFromDB(userId); // Expensive operation
userProfileCache.put(userId, profile);
return profile;
}
}
private UserProfile fetchUserProfileFromDB(String userId) {
// Simulated database fetch
return new UserProfile(userId, "John Doe");
}
}
Why This Works
By caching only user profiles, you reduce unnecessary data in your cache and ensure that the cached entries have high value for users. This approach streamlines performance and resource management.
4. Monitor Cache Usage and Performance
It’s vital to monitor your cache usage periodically. By analyzing cache hit rates, memory usage, and eviction rates, you can adjust your caching strategies proactively.
Metrics to Monitor
- Cache Hit Rate: Measures the percentage of requests served from the cache.
- Eviction Rate: How often items are removed from the cache.
- Memory Usage: Tracks how much memory your caching mechanism consumes in real-time.
Example of Monitoring with a Simple Logger
public class CacheMonitor {
private Cache<String, String> cache;
public CacheMonitor(Cache<String, String> cache) {
this.cache = cache;
}
public void logCacheUsage() {
System.out.println("Cache Size: " + cache.estimatedSize());
System.out.println("Hit Rate: " + cache.stats().hitRate());
System.out.println("Eviction Count: " + cache.stats().evictionCount());
}
}
Why This Works
By monitoring cache statistics, you can make informed decisions regarding cache size and eviction policies, preventing overwhelming or ineffective caching.
The Closing Argument
Preventing cache overload in Java web applications requires well-considered strategies that include the effective implementation of caching policies, monitoring, and granularity of cache storage. Time-based expiration, LRU eviction, selective caching, and performance monitoring can help alleviate potential performance issues associated with cache overload.
By following these guidelines, web developers can ensure a more responsive application, enhance user experience, and keep their caching strategy aligned with best practices—an approach mirrored from other programming languages, as discussed in Avoid Cache Overload: Optimizing NodeJS Apps (infinitejs.com/posts/optimize-nodejs-apps-cache-avoidance).
Incorporating these practices into your Java applications will safeguard against inefficiencies in caching, leading to better resource management and a user-friendly experience. Happy coding!