Java Caching Strategies: Preventing Overload in Server Apps
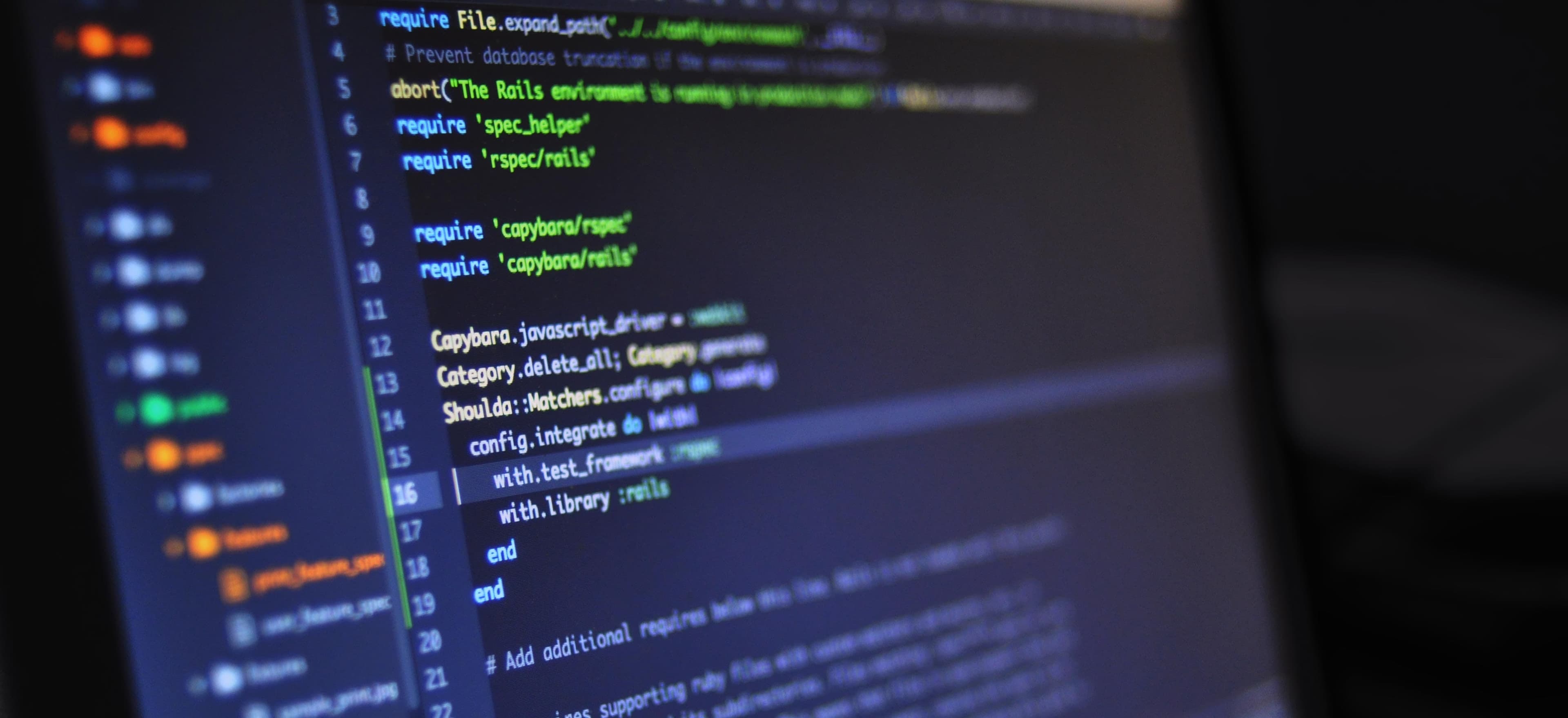
- Published on
Java Caching Strategies: Preventing Overload in Server Apps
In the world of server applications, performance is paramount. As applications scale, efficiently managing resources becomes crucial. One of the most effective strategies employed in achieving optimal performance is caching. In this article, we will explore various caching strategies in Java, discuss their advantages, and provide practical examples — all while keeping an eye on preventing overload in server apps.
What is Caching?
Caching is a technique used to store copies of files or data in temporary storage areas so they can be accessed more quickly. This reduces the need to retrieve data from slower sources such as databases or external services, thus improving application performance.
Why Caching Matters
- Improved Performance: By storing frequently accessed data temporarily, applications can reduce the response time for end-users.
- Resource Management: Efficient use of resources, including network bandwidth and server load, is essential for a smooth operation, especially under high traffic conditions.
- Cost Efficiency: Fewer requests to back-end services or databases can lead to cost savings, especially in cloud environments where resource usage directly translates to costs.
Caching Strategies in Java
When implementing caching in Java applications, several strategies can be deployed. Below are some widely adopted caching strategies, accompanied by code snippets and explanations.
1. In-Memory Caching
In-memory caching is one of the simplest forms of caching where data is stored in the RAM. This method offers the fastest access speeds but comes with the limitation of memory constraints.
Example: Using HashMap for In-Memory Cache
import java.util.HashMap;
public class InMemoryCache {
private HashMap<String, String> cache = new HashMap<>();
public void put(String key, String value) {
cache.put(key, value);
}
public String get(String key) {
return cache.get(key);
}
public static void main(String[] args) {
InMemoryCache inMemoryCache = new InMemoryCache();
inMemoryCache.put("key1", "value1");
// Fetching data from the cache
System.out.println(inMemoryCache.get("key1")); // Output: value1
}
}
Why Use HashMap?: A HashMap
provides O(1) time complexity for retrieval, making it a good choice for in-memory caching. However, it is essential to monitor memory usage and implement eviction strategies to prevent cache overload.
2. Caching Libraries
Using libraries can save time and offer advanced features. Libraries like Ehcache and Guava Cache can significantly simplify caching in Java applications.
Example: Guava Cache Implementation
import com.google.common.cache.CacheBuilder;
import com.google.common.cache.CacheLoader;
import com.google.common.cache.LoadingCache;
import java.util.concurrent.TimeUnit;
public class GuavaCachingExample {
private LoadingCache<String, String> cache;
public GuavaCachingExample() {
cache = CacheBuilder.newBuilder()
.maximumSize(100) // Limit cache size
.expireAfterWrite(10, TimeUnit.MINUTES) // Expiry policy
.build(new CacheLoader<String, String>() {
@Override
public String load(String key) {
return fetchDataFromDatabase(key);
}
});
}
private String fetchDataFromDatabase(String key) {
// Simulated database access
return "DatabaseValueFor:" + key;
}
public String getValue(String key) {
return cache.getUnchecked(key);
}
public static void main(String[] args) {
GuavaCachingExample guavaCache = new GuavaCachingExample();
// First call fetches data from the database
System.out.println(guavaCache.getValue("key1"));
// Subsequent calls retrieve from the cache
System.out.println(guavaCache.getValue("key1"));
}
}
Why Use Guava?: Guava's cache allows for setting size limits and expiration times to prevent overload issues that could arise from excessive memory consumption.
3. Distributed Caching
For large-scale applications, a distributed caching system is necessary. This type of caching allows data to be shared across multiple servers, enhancing data availability and scalability.
Example: Using Redis for Distributed Caching
To set up Redis as your caching mechanism, include the Jedis library in your project. You can find it here.
<dependency>
<groupId>redis.clients</groupId>
<artifactId>jedis</artifactId>
<version>3.5.0</version>
</dependency>
Here's a quick implementation using Jedis:
import redis.clients.jedis.Jedis;
public class RedisCacheExample {
private Jedis jedis;
public RedisCacheExample() {
jedis = new Jedis("localhost");
jedis.connect();
}
public void put(String key, String value) {
jedis.set(key, value);
}
public String get(String key) {
return jedis.get(key);
}
public static void main(String[] args) {
RedisCacheExample redisCache = new RedisCacheExample();
redisCache.put("key1", "value1");
// Fetching data from Redis
System.out.println(redisCache.get("key1")); // Output: value1
}
}
Why Use Redis?: Redis is an in-memory data structure store, offering persistence and robustness. Distributed caching with Redis can significantly reduce the load on your databases, especially in high traffic scenarios.
Preventing Cache Overload
While caching can significantly enhance performance, improper management can lead to cache overload, impacting application performance negatively. Here are some strategies to prevent this:
1. Cache Eviction Policies
Implementing effective eviction policies (like Least Recently Used, Least Frequently Used) ensures that older or less accessed data is removed when the cache has reached its limit.
2. Monitoring Cache Usage
Regularly monitor cache metrics to detect overloading scenarios. Tools like Prometheus combined with Grafana can help visualize the cache performance.
3. Adaptive Caching
Consider implementing adaptive caching strategies that dynamically adjust cache settings based on current load and data access patterns.
4. Allocate Sufficient Memory
Lastly, always ensure that adequate memory is allocated to your caching solution. For databases or distributed cache systems like Redis, initial and maximum memory configurations should be tuned to meet current and projected workloads.
Lessons Learned
Caching is an essential technique for enhancing the performance of Java server applications. By understanding different caching strategies — from in-memory caches to distributed systems like Redis — developers can make informed decisions on how to implement caching effectively.
As highlighted in Avoid Cache Overload: Optimizing NodeJS Apps, similar principles apply across languages. Understanding caching not only leads to improved application efficiency but also helps in maintaining system integrity under load, thus providing a seamless experience for users.
By implementing the discussed strategies, you're well on your way to preventing cache overload and optimizing your Java applications for peak performance. Happy coding!
Checkout our other articles