Java Solutions for Preventing Cache Overload in Spring Apps
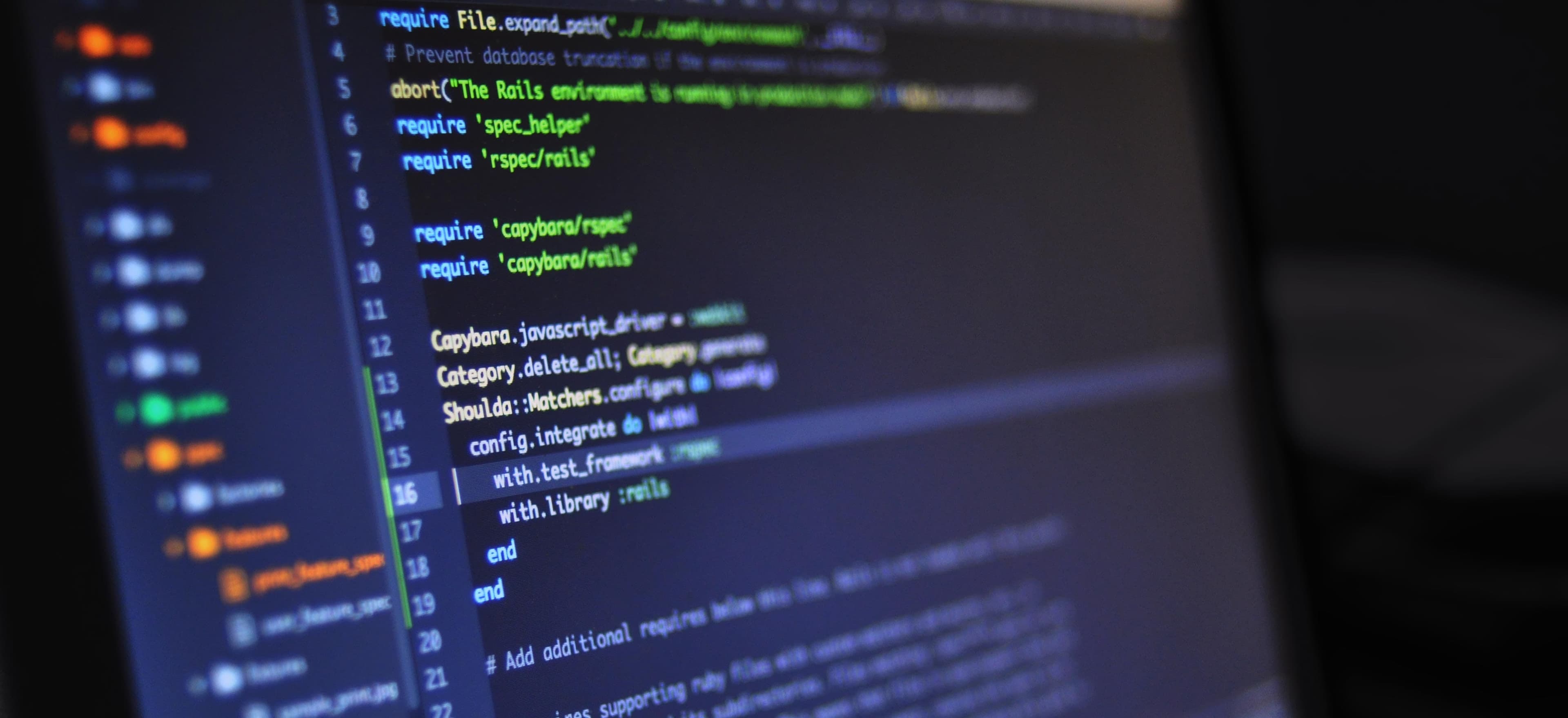
- Published on
Java Solutions for Preventing Cache Overload in Spring Apps
In an era of rapid application deployment and increased user demands, developers often face challenges in maintaining an efficient caching mechanism. Overloading can lead to performance bottlenecks and degrade user experiences. This article will explore effective strategies for preventing cache overload in Java Spring applications, paralleling insights shared in Avoid Cache Overload: Optimizing NodeJS Apps.
Understanding Cache Overload
Caches store frequently accessed data to improve read operations and reduce latency. In Spring applications, caching mechanisms, such as EHCache and Caffeine, enhance performance. However, if not correctly managed, caches can become overloaded.
Overloaded caches may result from storage limits being breached or because high traffic exceeds what the cache can handle. When these issues occur, your application experiences slowdowns, increased error rates, or undesirable resource consumption.
Why Prevent Cache Overload?
Preventing cache overload is crucial for:
- Performance: A well-managed cache improves application speed.
- Scalability: Efficient caching translates to better resource utilization.
- User Satisfaction: A responsive application fosters a positive user experience.
Best Practices to Prevent Cache Overload in Spring
1. Implement Cache Expiration
Setting an expiration policy ensures that stale data does not linger in the cache indefinitely. This strategy can decrease cache size over time and mitigate the chances of overload.
Example Code Snippet: Configuring Cache Expiration
import org.springframework.cache.annotation.Cacheable;
import org.springframework.cache.annotation.EnableCaching;
import org.springframework.context.annotation.Configuration;
@Configuration
@EnableCaching
public class CacheConfig {
@Cacheable(value = "myCache", key = "#id", unless = "#result == null")
public MyObject findById(Long id) {
// fetch the object logic
}
}
In this example, we utilize Spring's built-in caching through annotations. The @Cacheable
annotation helps store the cache, while the unless
attribute prevents null results from being cached—reducing unnecessary data.
2. Use Size-Based Eviction Policies
Implementing a size-based eviction policy allows you to remove the least-recently-used entries once a cache size threshold is met. This strategy ensures the cache remains manageable.
Example Code Snippet: Configuring Caffeine Cache with Size Limit
import com.github.benmanes.caffeine.cache.CacheLoader;
import com.github.benmanes.caffeine.cache.Caffeine;
import com.github.benmanes.caffeine.cache.LoadingCache;
import java.util.concurrent.TimeUnit;
public class CaffeineCacheConfig {
private final LoadingCache<Long, MyObject> cache = Caffeine.newBuilder()
.maximumSize(1000) // Max cache size
.expireAfterWrite(10, TimeUnit.MINUTES) // Expire entries after 10 minutes
.build((CacheLoader<Long, MyObject>) this::getObjectFromDb);
private MyObject getObjectFromDb(Long id) {
// Fetch from DB
}
}
This example creates a Caffeine cache that can hold up to 1000 entries, automatically evicting the oldest items when the limit is reached. The configuration also specifies an expiration time for entries, ensuring old data does not take up space.
3. Optimize the Cache Strategy
Consider different caching strategies based on the use cases of your application. For example, using a hybrid of in-memory cache and distributed caching can balance the load.
Local Cache vs. Distributed Cache
- Local Cache: Faster access, but limited to individual instances. Suitable for non-critical data.
- Distributed Cache: Suitable for shared data across multiple services, at the cost of increased latency.
Example Code Snippet: Configuring Redis as a Distributed Cache
import org.springframework.cache.annotation.EnableCaching;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.redis.cache.RedisCacheConfiguration;
import org.springframework.data.redis.repository.configuration.EnableRedisRepositories;
import java.time.Duration;
@Configuration
@EnableCaching
@EnableRedisRepositories
public class RedisConfig {
@Bean
public RedisCacheConfiguration cacheConfiguration() {
return RedisCacheConfiguration.defaultCacheConfig()
.entryTtl(Duration.ofMinutes(5)) // Set expiration time
.disableCachingNullValues(); // Avoid caching nulls
}
}
This configuration connects Spring Cache with Redis, setting a consistent expiration time and ensuring no null values are cached.
4. Monitor and Analyze Cache Performance
Regularly monitoring cache performance indicates when overload situations may arise. Performance can be tracked by observing cache hit ratios, the size of cached data, and eviction counts.
You may leverage tools like Spring Boot Actuator, which assists in providing insight into your application's health metrics.
5. Adjust Caching Strategies Based on Traffic Patterns
Implement adaptive caching strategies that analyze traffic patterns. For instance, you could allocate more cache resources during peak hours and subsequently reduce them during off-peak hours.
Example: Combining Strategies
A practical implementation might combine several strategies mentioned above to form a cohesive system for managing cache within a Spring application.
Example Code Snippet: Comprehensive Cache Management
import org.springframework.cache.annotation.Cacheable;
import org.springframework.cache.annotation.EnableCaching;
import org.springframework.stereotype.Service;
@Service
@EnableCaching
public class MyCachingService {
@Cacheable(value = "myCompositeCache", key = "#id", unless = "#result == null")
public MyObject findResource(Long id) {
// Logic to retrieve the resource
}
// Additional methods maintaining TTL and eviction strategies
}
In this configuration, you could further enhance the methods and details (like TTL handling) for better resource management, thereby executing a cost-effective caching strategy.
A Final Look
Preventing cache overload in Java Spring applications is critical for ensuring top-notch performance and user satisfaction. By implementing cache expiration, size-based policies, tailored caching strategies, performance monitoring, and adaptive measures, you can maintain a healthy cache system.
For additional insights on managing performance in high-demand applications, you may want to check out the Avoid Cache Overload: Optimizing NodeJS Apps for contrasting approaches that can influence cache management efforts in Java applications.
By taking these steps, you can create a more responsive, efficient, and scalable application that successfully meets user demands while minimizing overhead. Remember, caching is not just a performance feature; it’s a fundamental architecture component that, when utilized effectively, becomes a powerful asset in your developer toolkit.
Checkout our other articles