Enhancing Java Graphics: Tackling RGB Component Loss
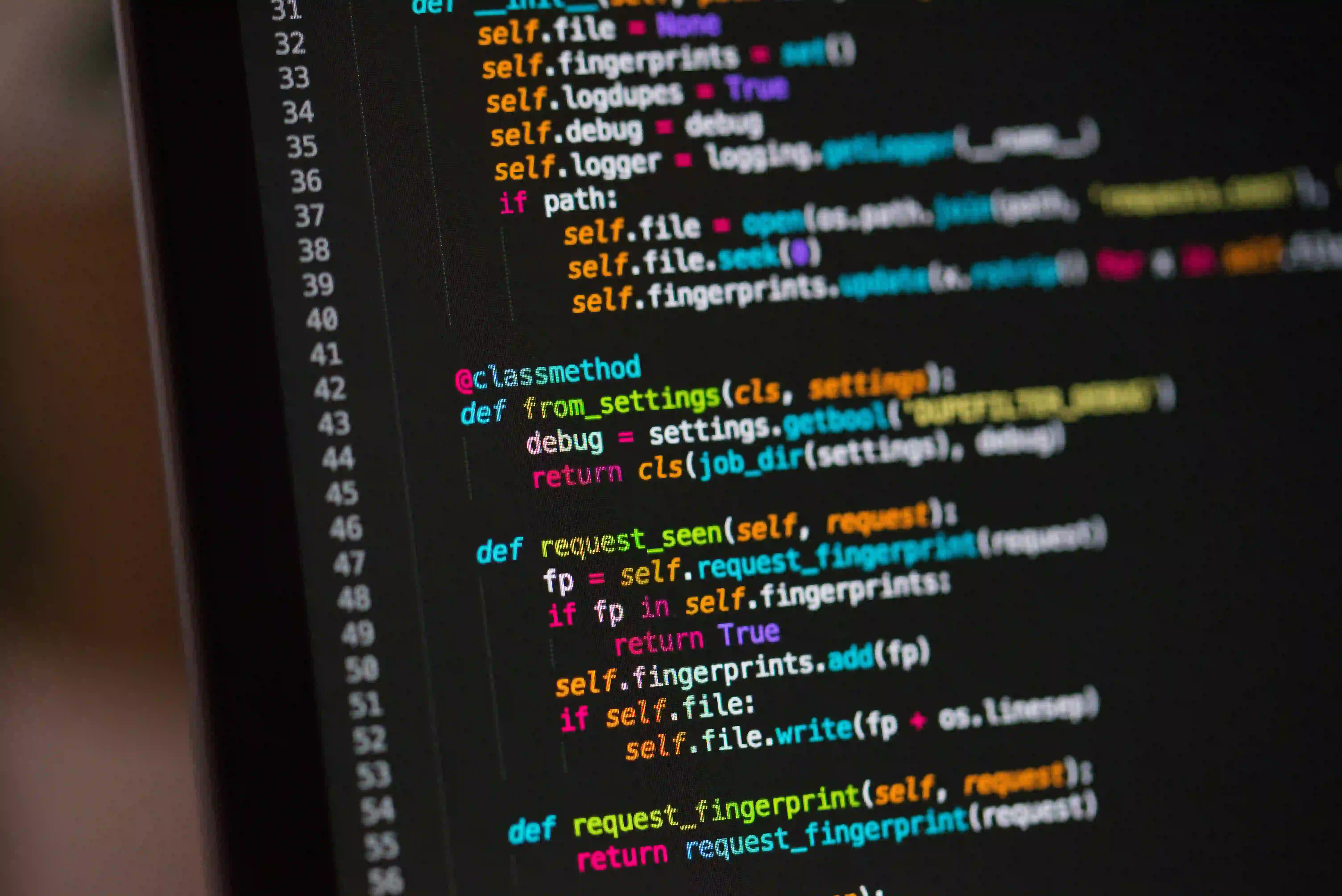
Enhancing Java Graphics: Tackling RGB Component Loss
When working with graphics in Java, one of the most pressing concerns can be the handling of colors, particularly the RGB (Red, Green, Blue) components. Effective manipulation and understanding of these components can make or break the visual quality of your application. In this post, we will explore how to mitigate issues surrounding RGB component loss, drawing upon principles from color theory and programming strategies. If you're struggling with this topic, you may find it interesting to check out Mastering Color Matching: Solving RGB Component Loss for additional insights.
What is RGB Component Loss?
RGB component loss occurs when color information is either degraded or inadequately represented, leading to visually incorrect or washed-out images. This issue can arise due to various factors, from insufficient color depth in images to improper manipulation of color values in code.
Understanding how to best manage these RGB values in Java can help developers create more vibrant and accurate graphics.
Basic RGB Manipulation in Java
In Java, colors can be represented in the Color
class:
import java.awt.Color;
Color red = new Color(255, 0, 0); // Full intensity red
Color green = new Color(0, 255, 0); // Full intensity green
Color blue = new Color(0, 0, 255); // Full intensity blue
Why Use the Color Class?
Using the Color
class simplifies the process of defining colors. When you use new Color(int r, int g, int b)
, you get an instance that encapsulates your RGB values, ensuring consistency across your graphics operations.
Adjusting RGB Values
It's not uncommon to want to adjust RGB components. Here's an example of boosting the red component in an image's pixels:
public BufferedImage adjustRedComponent(BufferedImage image, float adjustmentFactor) {
BufferedImage adjustedImage = new BufferedImage(image.getWidth(), image.getHeight(), BufferedImage.TYPE_INT_RGB);
for (int x = 0; x < image.getWidth(); x++) {
for (int y = 0; y < image.getHeight(); y++) {
Color originalColor = new Color(image.getRGB(x, y));
int red = Math.min((int)(originalColor.getRed() * adjustmentFactor), 255);
int green = originalColor.getGreen();
int blue = originalColor.getBlue();
Color newColor = new Color(red, green, blue);
adjustedImage.setRGB(x, y, newColor.getRGB());
}
}
return adjustedImage;
}
Commentary
In this code snippet, we create a new method that takes an image and a float (adjustment factor) as parameters. Inside the method, a loop iterates over each pixel, adjusting the red component according to the specified factor. The Math.min
method ensures the value does not exceed 255, which is critical in avoiding overflow errors that can lead to RGB component loss.
Understanding Color Depth
Color depth refers to the number of bits used to represent the color of a single pixel. In many cases, a higher color depth allows for more accurate representation of RGB values.
Working with Different Color Depths
Consider this scenario where you're working with an 8-bit color depth. It means each color channel (R, G, B) has 256 possible values. However, when transitioning to a 24-bit color depth (commonly used), each channel can now have 256 x 256 x 256 combinations. This increased flexibility greatly minimizes the risk of RGB component loss.
BufferedImage img = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
In this example, we specify the image type as TYPE_INT_RGB
, allowing for a richer representation of colors in your graphics.
Techniques for Preventing RGB Component Loss
1. Handling Color Space Correctly
Improper transformation between different color spaces (like RGB, CMYK, and HSV) can lead to component loss. Ensure that you're consistently working within the RGB color space when developing visual applications.
2. Use of Color Profiles
Including color profiles in your application helps preserve color calibration across devices. Libraries like Java Advanced Imaging (JAI) can be useful in embedding these profiles.
3. Image Resampling
When resizing images, you may lose color information if not handled properly. Utilizing libraries or built-in resampling techniques can mitigate component loss.
Here's a snippet using Graphics2D
to scale an image while keeping RGB integrity:
public BufferedImage scaleImage(BufferedImage originalImage, int newWidth, int newHeight) {
BufferedImage scaledImage = new BufferedImage(newWidth, newHeight, BufferedImage.TYPE_INT_RGB);
Graphics2D g = scaledImage.createGraphics();
// Enable anti-aliasing for better quality
g.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON);
g.drawImage(originalImage, 0, 0, newWidth, newHeight, null);
g.dispose();
return scaledImage;
}
Commentary
By using Graphics2D
, you enhance the image quality when scaling. The RenderingHints.KEY_ANTIALIASING
enables smoother edges and preserves color details, counteracting potential RGB losses during the resizing process.
A Final Look
Gaining a solid foothold in RGB manipulation can significantly improve the quality of the graphics in your Java applications. From understanding the pitfalls of component loss to implementing effective strategies, developers have the tools at their disposal to create visually stunning applications.
If you're looking for more detailed guidance in this domain, the article Mastering Color Matching: Solving RGB Component Loss offers an excellent foundation. Remember, whether you're working with basic color adjustments or dealing with complex graphical transformations, the integrity of your RGB values is paramount.
By utilizing best practices and understanding the intricacies of color manipulation, you can fully unlock the potential of Java graphics and enhance the visual fidelity of your projects. Happy coding!