Tackling Color Matching Issues in Java Graphics Rendering
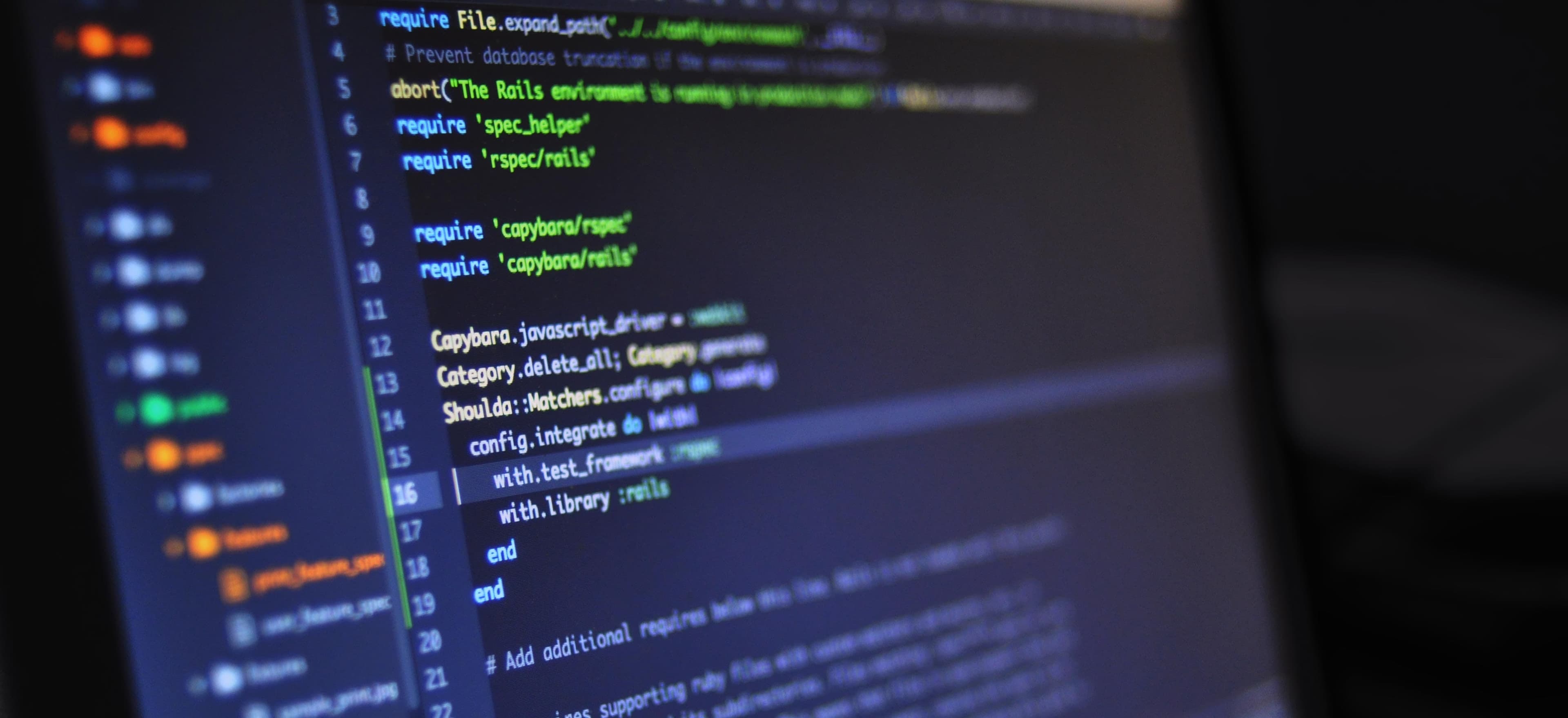
- Published on
Tackling Color Matching Issues in Java Graphics Rendering
Color matching is one of the most intricate challenges in graphics rendering, particularly in Java. Many developers often face issues with RGB component loss, leading to incorrect color representation on the screen. If you’re navigating similar waters, it’s imperative to understand how to manage color effectively in Java. This blog post will guide you through resolving color matching issues, improving the visual quality of your output, and referencing expert advice, including insights from an article titled Mastering Color Matching: Solving RGB Component Loss.
Understanding Color Representation
In Java, colors are represented using the RGB (Red, Green, Blue) model. Each color is defined by three components, each in the range from 0 to 255. Therefore, the color black is (0, 0, 0), while white is (255, 255, 255).
Why Use the RGB Model?
The RGB model is favored in digital screens because it aligns with how most displays emit light. However, when dealing with graphic rendering, discrepancies in color output are common, primarily due to issues like:
- Scaling and Clipping: As pixel colors are manipulated, some values may exceed their allowable range (0-255).
- Color Profiles: Differences in devices' color profiles can lead to unexpected variations in color representation.
Practical Example
Let’s explore an example of how to create and manipulate color in a Java application:
import java.awt.Color;
public class ColorExample {
public static void main(String[] args) {
// Create a color using RGB values
Color myColor = new Color(100, 150, 200);
// Print RGB values
System.out.println("Red: " + myColor.getRed()); // Output: 100
System.out.println("Green: " + myColor.getGreen()); // Output: 150
System.out.println("Blue: " + myColor.getBlue()); // Output: 200
}
}
In this snippet, we create a Color
object using RGB values. This is straightforward yet essential: understanding these components helps avoid common pitfalls when rendering.
Common Challenges in Rendering
Component Loss and Clipping
In graphic rendering, it’s essential to manage the color values accurately. If values exceed 255, they clip to the maximum, leading to the loss of detail. This is a typical programming issue where the logic around manipulating these colors can result in out-of-bound exceptions.
Addressing Clipping
Let’s review a method to ensure your RGB values are within the proper range:
public class ColorUtils {
public static Color clipColor(int r, int g, int b) {
r = Math.max(0, Math.min(255, r));
g = Math.max(0, Math.min(255, g));
b = Math.max(0, Math.min(255, b));
return new Color(r, g, b);
}
}
In this code, the clipColor
method ensures that inputs are correctly constrained between 0 and 255. By using Math.max()
and Math.min()
, this approach prevents clipping issues, safeguarding your color values before creating a new Color
object.
Color Blindness and Accessibility
Another often-overlooked factor in color rendering is accessibility. Considering color blindness, involving just the RGB values may not suffice. Using a more inclusive approach will foster better user experiences.
Resources for Color Accessibility
For an insight into web accessibility standards, the Web Content Accessibility Guidelines (WCAG) are excellent references. They provide essential insights into ensuring all users can interact with your graphics effectively.
Exploring Java Graphics
When rendering graphics, Java provides a robust API. The Graphics
class is the backbone:
import javax.swing.*;
import java.awt.*;
public class ColorRender extends JPanel {
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
g.setColor(Color.RED);
g.fillRect(20, 20, 100, 100);
// Fill a blue rectangle with clipped color
Color clippedColor = ColorUtils.clipColor(300, 50, -10);
g.setColor(clippedColor);
g.fillRect(150, 20, 100, 100);
}
public static void main(String[] args) {
JFrame frame = new JFrame("Color Rendering Example");
ColorRender panel = new ColorRender();
frame.add(panel);
frame.setSize(300, 200);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
In this code snippet, we define a ColorRender
class extending JPanel
. The color is set for two rectangles. The first is filled with red, while the second uses the clipped RGB value. This visualization helps developers notice the discrepancies in color rendering leading to component loss.
Layering in Java Graphics
When dealing with advanced graphics, layering is another critical aspect. Utilizing the Java2D API can further enhance your rendering technique.
import javax.swing.*;
import java.awt.*;
import java.awt.geom.Ellipse2D;
public class LayeredGraphics extends JPanel {
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2d = (Graphics2D) g;
// Set the rendering hint for better graphics quality
g2d.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON);
// Draw a circle
g2d.setColor(Color.BLUE);
g2d.fill(new Ellipse2D.Double(50, 50, 100, 100));
// Layering on a semi-transparent red rectangle
g2d.setColor(new Color(255, 0, 0, 128)); // 128 for transparency
g2d.fillRect(75, 75, 100, 100);
}
public static void main(String[] args) {
JFrame frame = new JFrame("Layered Graphics Example");
LayeredGraphics panel = new LayeredGraphics();
frame.add(panel);
frame.setSize(300, 300);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
In this example, we utilize Java2D to draw an antialiased circle and overlay a semi-transparent rectangle. Layering is crucial for effects like shadows and highlights, thereby improving the overall aesthetics of your graphics.
Debugging Color Issues
When rendering issues arise, debugging is vital. Common debugging tools include:
-
Visual Debugging Tools: Utilize tools that can help visualize how your graphics render colors and shapes.
-
Logging: Implement diagnostics to log color values before rendering. This ensures the values used in the rendering process are within the expected range.
Adding Logging
Consider adding simple logging:
// Inside your rendering class before drawing
System.out.println("Current Color: " + clippedColor);
This can reveal underlying problems in your rendering pipeline.
Wrapping Up
Color rendering in Java, while powerful, comes with its challenges, particularly around matching and component loss. Understanding how to manage RGB values effectively and employing techniques for debugging can significantly enhance the quality of your graphical applications.
For more advanced insights into tackling color matching specifically, refer to the detailed examination and strategies in the article Mastering Color Matching: Solving RGB Component Loss.
The relationship between color and graphics is intricate, yet with patience and practice, you can master it in your Java applications! Happy coding!