Java Developers: Tackle Command Line Hurdles with Ease
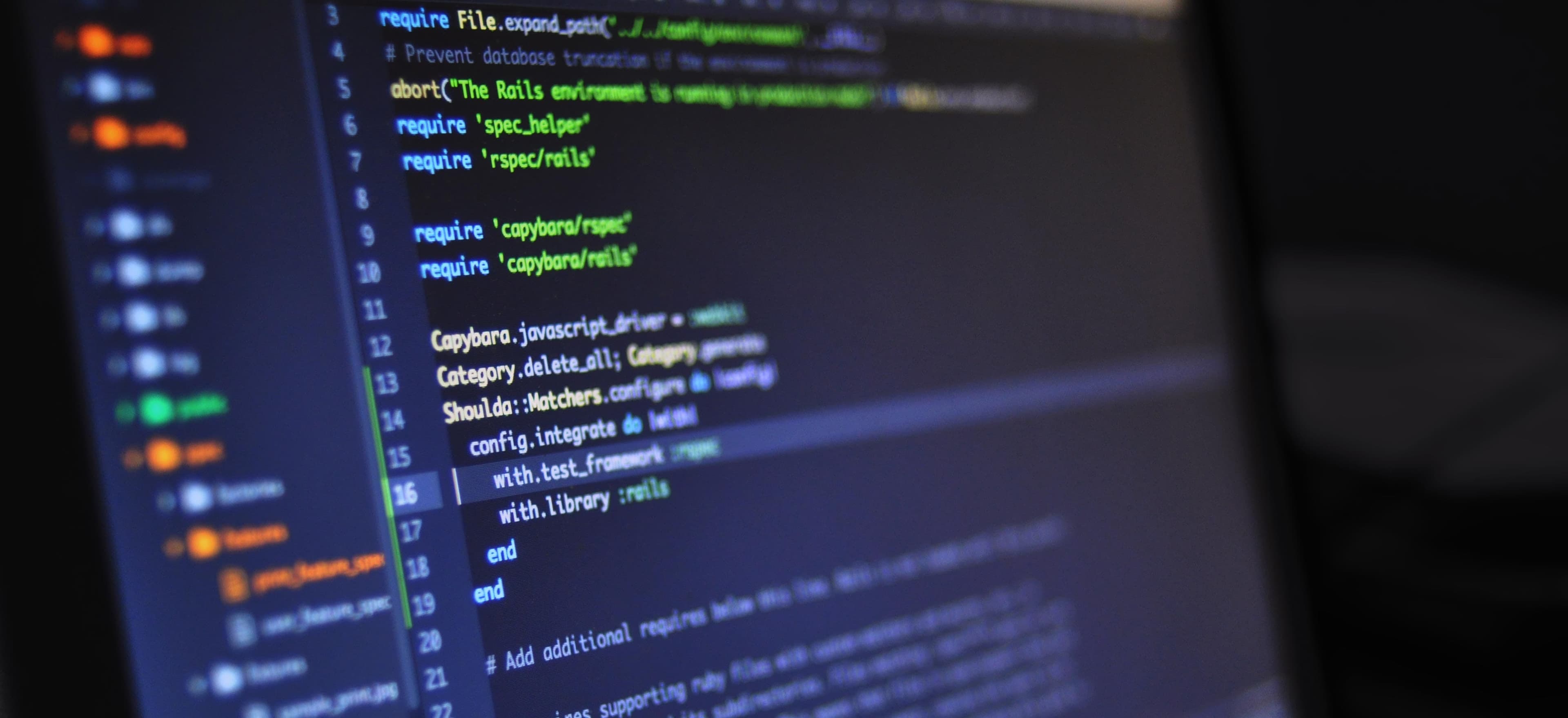
- Published on
Java Developers: Tackle Command Line Hurdles with Ease
As a Java developer, you may find yourself diving into command-line operations more often than you anticipate. From managing build tools like Maven and Gradle to deploying applications, mastering command line usage can significantly enhance your productivity. This post aims to help you overcome common command line hurdles, ensuring you are even more efficient in your Java development journey.
Why Command Line Proficiency Matters
The command line is not just a tool; it's an interface to efficiently interact with your system. Engaging with your development environment through the command line can seem daunting, especially for those who are used to GUIs. However, proficiency in command line operations allows for faster execution of commands, easier automation, and better troubleshooting capabilities.
Benefits:
- Speed: Command-line tools often execute faster than their graphical counterparts.
- Automation: You can script tasks, allowing for repeatability and efficiency.
- Greater Control: Fine-tune operations at a low level.
- Remote Management: Easily manage remote servers via SSH.
Essential Command Line Commands for Java Developers
Whether you are compiling code, executing tests, or deploying your application, the command line offers an array of commands tailored for Java development.
1. Navigating Directories
To navigate the file system, you need to master the use of commands such as cd
, ls
, and pwd
.
Example:
cd ~/Projects/MyJavaApp
Why: cd
(change directory) is essential for accessing your project files. The ~/
indicates the home directory, making it easy to locate your project folder quickly.
2. Compiling Java Code
Compiling Java files from the command line doesn’t have to be a hassle. Use the javac
command to compile your .java
files.
Example:
javac MyApp.java
Why: The javac
command compiles your Java source code into bytecode that can run on the Java Virtual Machine (JVM). This is a crucial step in the Java development process.
3. Running Java Applications
Once compiled, running your Java application can be easily accomplished using the java
command.
Example:
java MyApp
Why: This command initiates the JVM and runs the specified Java class. It's the gateway to seeing the output of your code.
4. Using Build Tools Efficiently
Maven and Gradle are widely used build tools in Java development. Understanding their command line interfaces can streamline your workflow.
Maven Example:
mvn clean install
Why: This command cleans the target directory and installs the project, ensuring that all dependencies are properly imported. Regularly using clean
eliminates compilation errors caused by cached files.
Gradle Example:
gradle build
Why: The build
command compiles and packages your application, allowing you to deploy it with ease.
Shell Scripting and Automation
To accelerate your workflow, you can create shell scripts that automate mundane tasks. Here’s a simple script that echoes a message and runs your Java application:
Example Script (run.sh):
#!/bin/bash
echo "Starting MyJavaApp..."
java MyApp
Why: This script simplifies the execution process, allowing for easy reuse without typing the command each time. It also provides a way to include additional commands later.
Making the Script Executable:
After creating the script, you'll need to make it executable using:
chmod +x run.sh
Why: The chmod
command changes the permissions of the script, making it executable from the command line.
Command Line Utilities to Enhance Your Productivity
There are several command line utilities that can significantly improve your efficiency as a Java developer:
1. Grep
grep
allows you to search through files for specific patterns or keywords.
Example:
grep "public class" *.java
Why: This command helps you locate where classes are defined in your project, improving debugging and code review processes.
2. Find
Use find
to search for files in your project's directory.
Example:
find . -name "*.jar"
Why: This command finds all JAR files in the current directory and its subdirectories, which is helpful for managing dependencies.
3. Diff
If you are comparing two versions of a file or directory, diff
will show you the differences.
Example:
diff version1.java version2.java
Why: This command is crucial for version control, allowing you to see changes and understand code evolution.
4. Top
Monitor system resource usage, especially useful in performance profiling.
Example:
top
Why: This command helps to track system resource consumption in real-time, allowing for timely optimizations in your application.
Overcoming Common Command Line Hurdles
Despite the benefits, many developers face several hurdles when interacting with the command line. Here are some tips to overcome common challenges:
1. Familiarize Yourself with Shortcuts
Learning keyboard shortcuts can drastically cut down the time you spend entering commands. For instance:
- Tab: Autocomplete file and directory names.
- Ctrl + C: Stop a running process.
- Ctrl + Z: Suspend a running process.
2. Error Messages
Understanding error messages is vital. Always read them carefully; they often guide you towards the source of the problem.
3. Reference Resources
Articles like Mastering Bash: Overcoming Common Command Line Challenges provide in-depth insight that can enhance your command line skills.
Closing the Chapter
Mastering the command line is an invaluable asset for Java developers. By understanding and utilizing common commands, automating tasks through scripting, and leveraging powerful utilities, you can significantly enhance your efficiency and productivity.
Although the command line may seem intimidating at first, practice and familiarity will lead you to mastery. So, start exploring these commands and integrate them into your development workflow today.
Start Your Journey
Ready to tackle command line hurdles? Get started now and discover how you can make your Java development process smoother and more efficient. Dive into practice, learn from online resources, and enjoy the journey of mastering your Java environment!
Checkout our other articles