Java Developers: Conquer Bash Command Line Hurdles
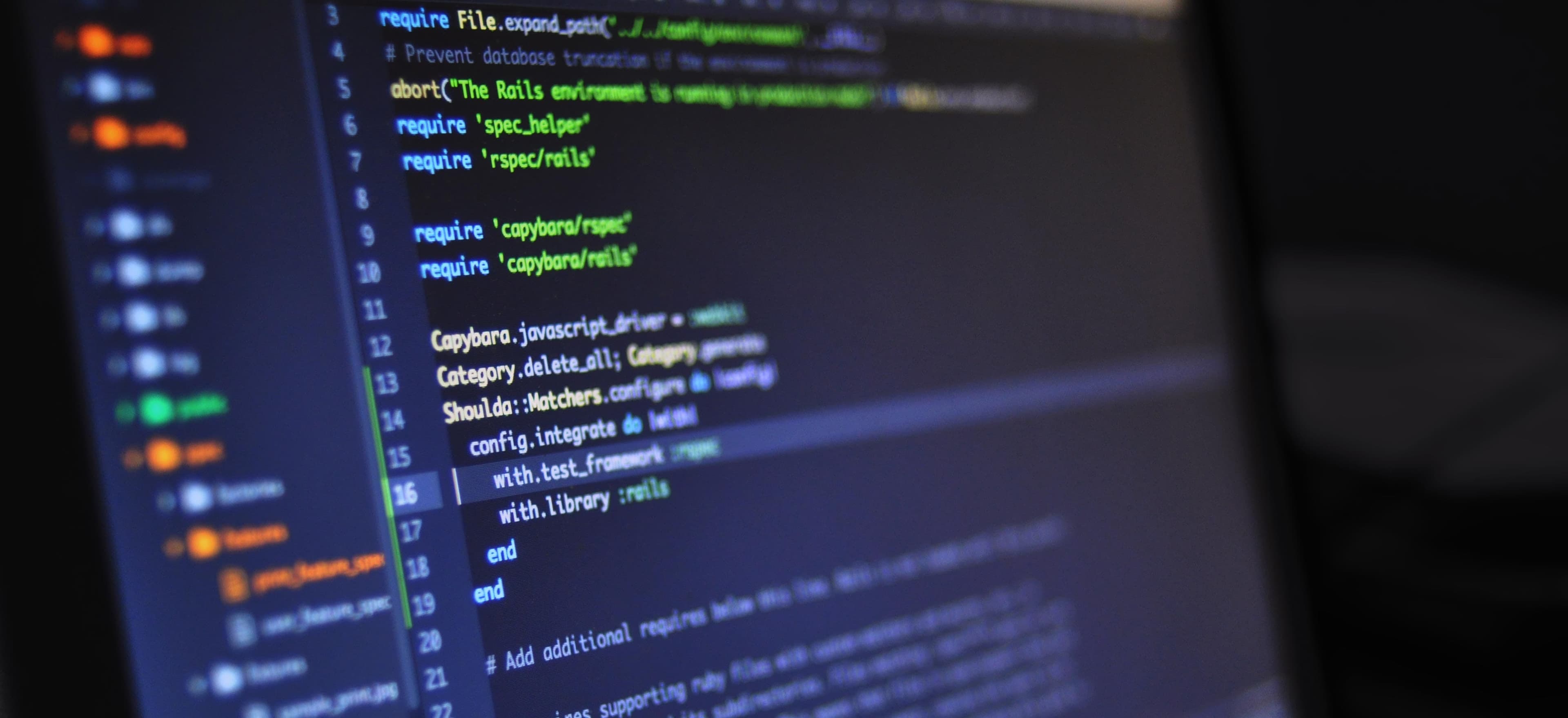
- Published on
Java Developers: Conquer Bash Command Line Hurdles
As Java developers, you might often find yourself juggling between writing code, managing files, and compiling projects. While Java is a powerful language for building applications, the command line (often referred to as Bash in Unix-like systems) remains an essential tool for many tasks—especially when automating workflows or managing server environments.
In this blog post, we will explore how Java developers can overcome common Bash command line challenges. By integrating command line proficiency with Java development skills, you can significantly streamline your workflow.
Understanding the Power of Bash
Bash is a command language interpreter that executes commands read from the standard input or from a file. Unlike Java, which is a high-level programming language, Bash allows you to interact directly with your operating system. This versatility enables you to perform tasks like file management, process control, and system monitoring quickly and efficiently.
Why Should Java Developers Learn Bash?
-
Improved Automation: Routine tasks can be automated using shell scripts, reducing repetitive work and increasing productivity.
-
Server Management: Many Java applications run on servers; knowing Bash commands allows you to manage these servers more effectively.
-
Tool Integration: Combine Java applications with command-line tools for enhanced functionality, whether it's for testing, deployment, or integration.
-
Debugging: Sometimes, issues arise outside the code. A good understanding of Bash can help you navigate logs and system settings quickly.
Common Bash Command Line Challenges
While Bash offers powerful capabilities, it can also present several challenges, especially for those unfamiliar with command line environments. Here are some of the hurdles Java developers might face:
-
File Management: Navigating directories and managing files can be cumbersome in Bash.
-
Scripting: Writing effective Bash scripts requires a different mindset than Java.
-
Command Syntax: The syntax can be cryptic at times, leading to confusion and mistakes.
-
Environment Variables: Understanding how to manage and use them for your applications can be tricky.
Preparing Your Environment
Before diving into overcoming specific challenges, let’s ensure your development environment is set up correctly. Ensure you have a terminal emulator installed with Bash, like Terminal on macOS or Git Bash on Windows.
File Management in Bash
Managing files is a key aspect of using Bash. For example, to list files in a directory, you would use the command:
ls -la
Why:
ls
: This command lists directory contents.-l
: This option triggers the "long" format, displaying detailed information about files.-a
: This option includes hidden files (those starting with a dot).
For more specialized file operations, you can leverage commands like cp
, mv
, and rm
:
cp source.txt destination.txt
mv oldname.txt newname.txt
rm unwantedfile.txt
Why:
cp
: Copies files from one location to another.mv
: Moves files or renames them.rm
: Deletes files.
To manage your projects effectively, consider scripting repetitive tasks for backup or cleanup purposes.
Example Bash Script for Backup
Remember that you can automate these tasks with scripts. Here's an exemplary script to back up a directory of your Java projects:
#!/bin/bash
# This script backs up Java projects
SOURCE_DIR="/path/to/java/projects"
BACKUP_DIR="/path/to/backup/$(date +%Y-%m-%d)"
mkdir -p "$BACKUP_DIR"
cp -r "$SOURCE_DIR"/* "$BACKUP_DIR"
echo "Backup completed successfully to $BACKUP_DIR"
Why:
mkdir -p
: Creates the backup directory using the current date.cp -r
: Recursively copies files and directories from source to backup.echo
: Provides feedback upon completion.
Scripting Basics
Many Java developers grapple with the scripting aspect of Bash, especially concepts like loops and conditionals. Here’s a simple example using a loop:
for file in *.java; do
echo "Compiling $file..."
javac "$file"
done
Why:
for file in *.java
: Iterates over all Java files in the directory.javac "$file"
: Compiles each Java file.
Using functions can also help organize your Bash scripts:
compile() {
for file in "$1"/*.java; do
echo "Compiling $file..."
javac "$file"
done
}
compile "/path/to/java/project"
Why:
compile()
: Defines a function to compile Java files from a specified directory.- This abstraction improves script readability and reuse.
Command Syntax Challenges
Bash syntax can be confusing, with many commands having unique flags and options. Utilizing the man
(manual) command is essential when you encounter unfamiliar commands. For example, to learn more about the grep
command, you would type:
man grep
Why:
- Provides comprehensive documentation about the command’s functionality, syntax, and available options.
Environment Variables
Setting and using environment variables is another area where Java developers may find challenges. Environment variables allow you to configure settings that your Java applications can leverage:
export JAVA_HOME=/path/to/java
To access this variable in your Java program:
String javaHome = System.getenv("JAVA_HOME");
System.out.println("Java Home: " + javaHome);
Why:
export
: Sets the variable in the current session.System.getenv()
: Retrieves the value of the desired environment variable in Java.
Closing Remarks
Navigating the Bash command line can initially seem daunting for Java developers. However, as you become familiar with file management, scripting basics, and command syntax, you’ll find that your productivity and efficiency can enhance significantly.
For an in-depth look at Bash and its powerful features, don’t hesitate to check out the article Mastering Bash: Overcoming Common Command Line Challenges. Coupling those insights with your newly acquired Bash skills will place you on an accelerated path toward becoming a more effective developer.
Embrace the command line, experiment, and continuously learn. Your journey as a Java developer can become increasingly rewarding with a strong command of Bash. Remember, every command line challenge is merely an opportunity for growth. Happy coding!