Java Meets Biochemistry: Modeling Life's Reactions in Code
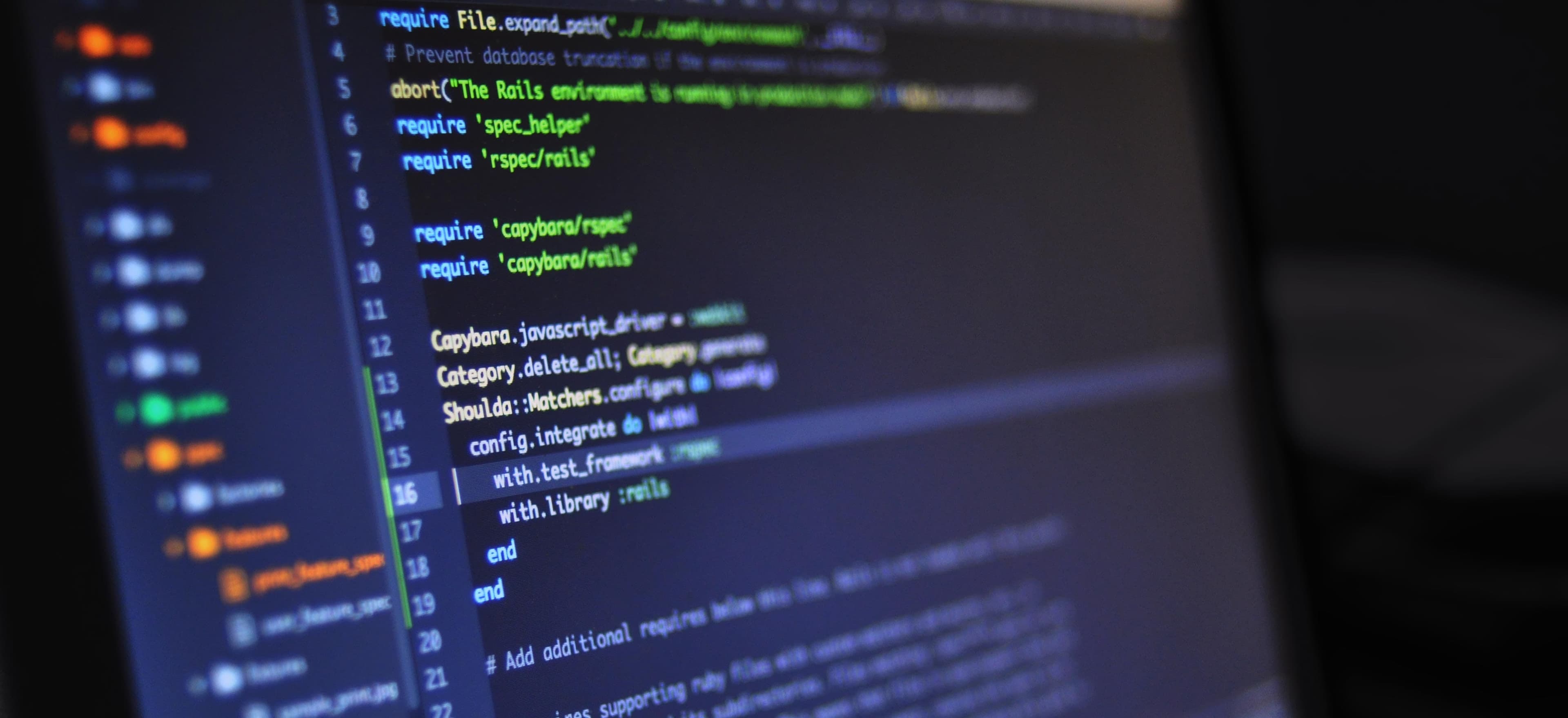
- Published on
Java Meets Biochemistry: Modeling Life's Reactions in Code
Java is a versatile programming language known for its platform independence and robust libraries. But did you know that it can also be a powerful tool for modeling complex biochemical reactions? In this blog post, we will explore how Java can mimic and analyze biological processes, unlocking fascinating insights into the mechanics of life itself.
A compelling reference for this topic is an existing article titled "Das Wunderwerk Leben: Biochemische Reaktionen entschlüsselt", which delves into the mechanisms of biochemical reactions. This piece underscores the importance of understanding these fundamental processes, paving the way for technological advancements in biology.
Why Model Biochemical Reactions?
Before we jump into the code, let’s understand why it is pertinent to model biochemical reactions. By simulating these reactions, we can:
- Predict Outcomes: Anticipating the behavior of biological systems can lead to innovative solutions in healthcare and biotechnology.
- Educate: Visual representation of reactions aids in teaching complex biological concepts.
- Research: Enable scientists to test hypotheses without the need for costly and time-consuming lab experiments.
Setting Up the Java Environment
To begin, ensure you have a Java Development Kit (JDK) installed on your machine. You can download the latest version from Oracle's official site. Also, consider using an Integrated Development Environment (IDE) like IntelliJ IDEA or Eclipse for better productivity.
Basic Structure of a Reaction Class
Let’s start by creating a basic structure to represent a biochemical reaction in Java. We will create a Reaction
class that holds information about substrates, products, and the rate of the reaction.
import java.util.HashMap;
import java.util.Map;
public class Reaction {
private Map<String, Integer> substrates;
private Map<String, Integer> products;
private double rate;
public Reaction(double rate) {
this.substrates = new HashMap<>();
this.products = new HashMap<>();
this.rate = rate;
}
public void addSubstrate(String name, int quantity) {
substrates.put(name, quantity);
}
public void addProduct(String name, int quantity) {
products.put(name, quantity);
}
public void displayReaction() {
System.out.print("Reactants: ");
substrates.forEach((k, v) -> System.out.print(v + " " + k + " "));
System.out.print(" --> Products: ");
products.forEach((k, v) -> System.out.print(v + " " + k + " "));
System.out.println(" | Rate: " + rate);
}
}
Analyzing the Code
- Map Usage: We’ve used
HashMap
to store the substrates and products. This allows for easy modification and retrieval of quantities associated with each chemical compound. - Dynamic Storage: With the
addSubstrate
andaddProduct
methods, we can dynamically add components to our reaction model. - Rate of Reaction: The
rate
variable enhances our model by allowing us to capture the kinetics of the reaction, a crucial component in biochemistry.
Implementing the Reaction Class
Let’s create a simple implementation of the reaction class that models glucose metabolism, a fundamental biological process.
public class Main {
public static void main(String[] args) {
Reaction glucoseMetabolism = new Reaction(2.0);
glucoseMetabolism.addSubstrate("C6H12O6", 1); // Glucose
glucoseMetabolism.addSubstrate("O2", 6); // Oxygen
glucoseMetabolism.addProduct("CO2", 6); // Carbon Dioxide
glucoseMetabolism.addProduct("H2O", 6); // Water
glucoseMetabolism.displayReaction();
}
}
Output Explanation
When you run the above code, you will see an output that displays the reactants, products, and the rate of reaction, neatly encapsulating the essential details of glucose metabolism. This simple structure lays the groundwork for more complex biochemical modeling.
Simulating a Reaction Over Time
Now that we have a basic representation of a reaction, let’s simulate how it progresses over time. The following is a method that updates the amounts of substrates and products based on reaction kinetics.
public void simulateReaction(double time) {
// Assuming first-order kinetics for simplicity
double change = rate * time;
substrates.forEach((k, v) -> {
int newQuantity = Math.max(0, v - (int) change);
substrates.put(k, newQuantity);
});
// Typically, we would also increase products, but we'll keep this simple.
// For real reactions, a more complex logic to calculate product formation would be needed.
}
Explanation of Simulation
This method assumes first-order kinetics, where the change in concentration is proportional to the amount of substrate present.
- Debiting Substrates: The method reduces the substrate levels as the reaction progresses.
- Safety Check: The
Math.max(0, v - (int) change)
ensures we do not end up with negative quantities.
Incorporating User Interaction
For a more engaging program, we can add user input to modify reaction parameters. We will use the Scanner
class for input purposes.
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter reaction rate:");
double reactionRate = scanner.nextDouble();
Reaction glucoseMetabolism = new Reaction(reactionRate);
glucoseMetabolism.addSubstrate("C6H12O6", 1); // Glucose
glucoseMetabolism.addSubstrate("O2", 6); // Oxygen
glucoseMetabolism.addProduct("CO2", 6); // Carbon Dioxide
glucoseMetabolism.addProduct("H2O", 6); // Water
glucoseMetabolism.displayReaction();
System.out.println("Simulating for how long?");
double time = scanner.nextDouble();
glucoseMetabolism.simulateReaction(time);
glucoseMetabolism.displayReaction();
scanner.close();
}
}
The User Interaction
- Dynamic Input: Users can specify the reaction rate and simulation time, making the model more flexible.
- Immediate Feedback: After simulating the reaction, users can see the updated quantities of substrates and products.
Key Takeaways
By following this framework, we have successfully modeled a biochemical reaction using Java. The example of glucose metabolism highlights the language’s capabilities in simulating and understanding complex biological processes.
As you explore this further, consider diving into more complex kinetics and multi-step reactions to push the boundaries of your simulations.
For those intrigued by the biochemical underpinnings of life, I recommend checking out the article "Das Wunderwerk Leben: Biochemische Reaktionen entschlüsselt" to gain more insights into the fascinating world of biochemistry.
Further Reading
To enhance your understanding of Java, consider visiting the following resources:
- Java Tutorials by Oracle
- Learn About Chemical Kinetics
Feel free to modify the code and expand the model to include enzyme activity, inhibitors, or other biochemical compounds. The scope is vast, and your imagination is the only limit! Happy coding!
Checkout our other articles