Unlocking Java's Potential: Biochemical Algorithms Explained
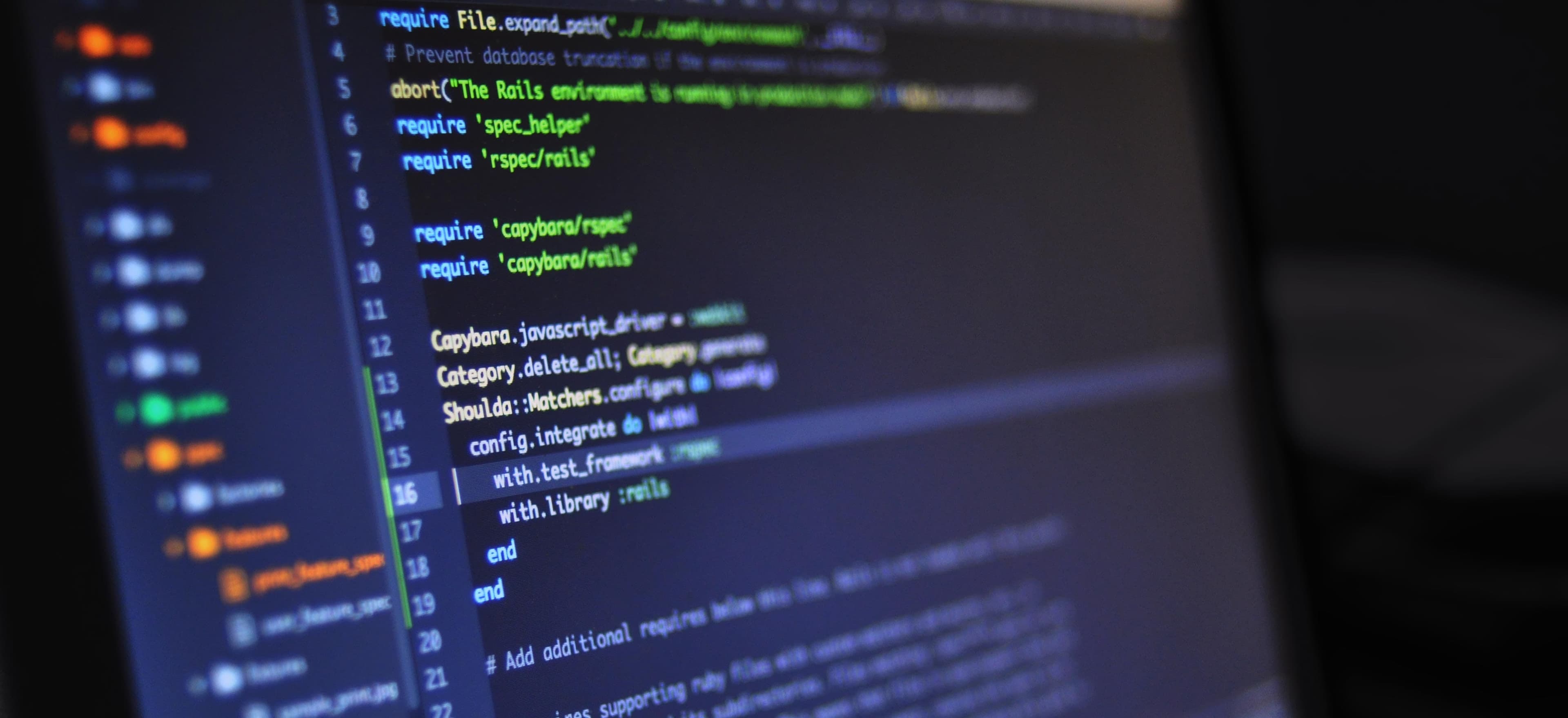
- Published on
Unlocking Java's Potential: Biochemical Algorithms Explained
Java has long been revered as a powerful, versatile programming language. With its ability to run on any platform and its robust libraries, it's a popular choice among developers. But have you ever considered how Java can be utilized to model complex biochemical processes? Drawing inspiration from the fascinating field of biochemistry, particularly the article "Das Wunderwerk Leben: Biochemische Reaktionen entschlüsselt," we’ll delve into some Java programming concepts that can help simulate biochemical reactions and algorithms.
Understanding Biochemical Reactions
Biochemical reactions are the processes that sustain life. They involve complex interactions among various molecules in living organisms. By modeling these reactions with algorithms, we can better understand their dynamics and possibly design better systems for drug discovery and synthetic biology.
To simulate biochemical reactions in Java, we often utilize concepts from object-oriented programming (OOP), such as classes and objects, to represent different molecules and reactions. The power of Java lies in its ability to handle large datasets and perform complex calculations efficiently.
Setting Up Your Java Environment
Before we dive into the algorithms, ensure you have the following setup:
- Java Development Kit (JDK) installed on your system.
- An Integrated Development Environment (IDE) such as Eclipse or IntelliJ IDEA for ease of coding.
You can download the JDK from Oracle's website.
Example: Creating a Molecular Class
First, let us define a class to represent a molecule. This will be the foundation upon which we build our biochemical simulations.
public class Molecule {
private String name;
private double concentration; // in mol/L
public Molecule(String name, double concentration) {
this.name = name;
this.concentration = concentration;
}
public String getName() {
return name;
}
public double getConcentration() {
return concentration;
}
public void setConcentration(double concentration) {
this.concentration = concentration;
}
}
Why This Matters
The Molecule
class encapsulates the essential properties of a biochemical molecule: its name and concentration. OOP principles allow us to create multiple instances of Molecule
, representing different substances in our algorithm.
Modeling Biochemical Reactions
Now that we have a foundation, let's model a simple biochemical reaction. The common format for a reaction can be expressed as:
A + B ↔ C + D
In Java, we can create a class that represents a reaction involving multiple molecules.
import java.util.HashMap;
import java.util.Map;
public class BiochemicalReaction {
private Map<Molecule, Integer> reactants;
private Map<Molecule, Integer> products;
public BiochemicalReaction() {
reactants = new HashMap<>();
products = new HashMap<>();
}
public void addReactant(Molecule molecule, int coefficient) {
reactants.put(molecule, coefficient);
}
public void addProduct(Molecule molecule, int coefficient) {
products.put(molecule, coefficient);
}
// Method to simulate the progression of the reaction
public void simulateReaction(double time, double rateConstant) {
// Simple linear dynamics for illustration purposes
double change = rateConstant * time;
// Update concentrations based on a fixed rate of reaction
for (Molecule reactant : reactants.keySet()) {
double newConcentration = reactant.getConcentration() - change * reactants.get(reactant);
reactant.setConcentration(newConcentration);
}
for (Molecule product : products.keySet()) {
double newConcentration = product.getConcentration() + change * products.get(product);
product.setConcentration(newConcentration);
}
}
}
Why This Approach?
The BiochemicalReaction
class uses HashMap
to associate a Molecule
with its stoichiometric coefficients. This allows for a flexible representation of reactions with various quantities of reactants and products. The simulateReaction
method offers a simplistic approach to update concentrations based on a fixed rate, capturing the essence of dynamic systems.
Bringing It All Together
Let’s create a simulation of a simple reaction using the classes we just defined. For this example, we'll model the reaction between glucose (C6H12O6) and oxygen (O2) producing carbon dioxide (CO2) and water (H2O).
public class Main {
public static void main(String[] args) {
// Create molecules
Molecule glucose = new Molecule("Glucose (C6H12O6)", 1.0); // 1 mol/L
Molecule oxygen = new Molecule("Oxygen (O2)", 0.5); // 0.5 mol/L
Molecule carbonDioxide = new Molecule("Carbon Dioxide (CO2)", 0.0);
Molecule water = new Molecule("Water (H2O)", 0.0);
// Create a reaction
BiochemicalReaction reaction = new BiochemicalReaction();
reaction.addReactant(glucose, 1);
reaction.addReactant(oxygen, 1);
reaction.addProduct(carbonDioxide, 1);
reaction.addProduct(water, 2);
// Simulate the reaction
reaction.simulateReaction(10, 0.1); // Simulate for 10 seconds
// Output concentrations
System.out.println("Glucose concentration: " + glucose.getConcentration());
System.out.println("Oxygen concentration: " + oxygen.getConcentration());
System.out.println("Carbon Dioxide concentration: " + carbonDioxide.getConcentration());
System.out.println("Water concentration: " + water.getConcentration());
}
}
Explanation
In the example above, we instantiated a few Molecule
objects and linked them to a BiochemicalReaction
. After simulating the reaction for a specific time, we print out the updated concentrations of each substance. While this simulation is basic, it serves to illustrate how you can use Java to model more complex biochemical processes.
Closing Remarks
Understanding biochemical reactions and algorithms is essential for various fields, including biochemistry, pharmacology, and systems biology. By leveraging Java's capabilities, researchers can model these reactions effectively, gaining insights that inform both academic and practical applications.
If you’re excited about diving deeper into the biochemical aspects of life, I highly recommend reading the article "Das Wunderwerk Leben: Biochemische Reaktionen entschlüsselt."
By integrating programming with biochemistry, we unlock new potential for innovation and understanding. The example we've explored today is just the tip of the iceberg—imagine the possibilities when we start to incorporate machine learning and big data analytics into our models!
References:
In this journey through Java and biochemistry, we’ve merely scratched the surface. With endless opportunities for further exploration, the fusion of these two fields can pave the way for groundbreaking advancements. Happy coding!
Checkout our other articles