Handling JSON with Java: A Guide to Database Integration
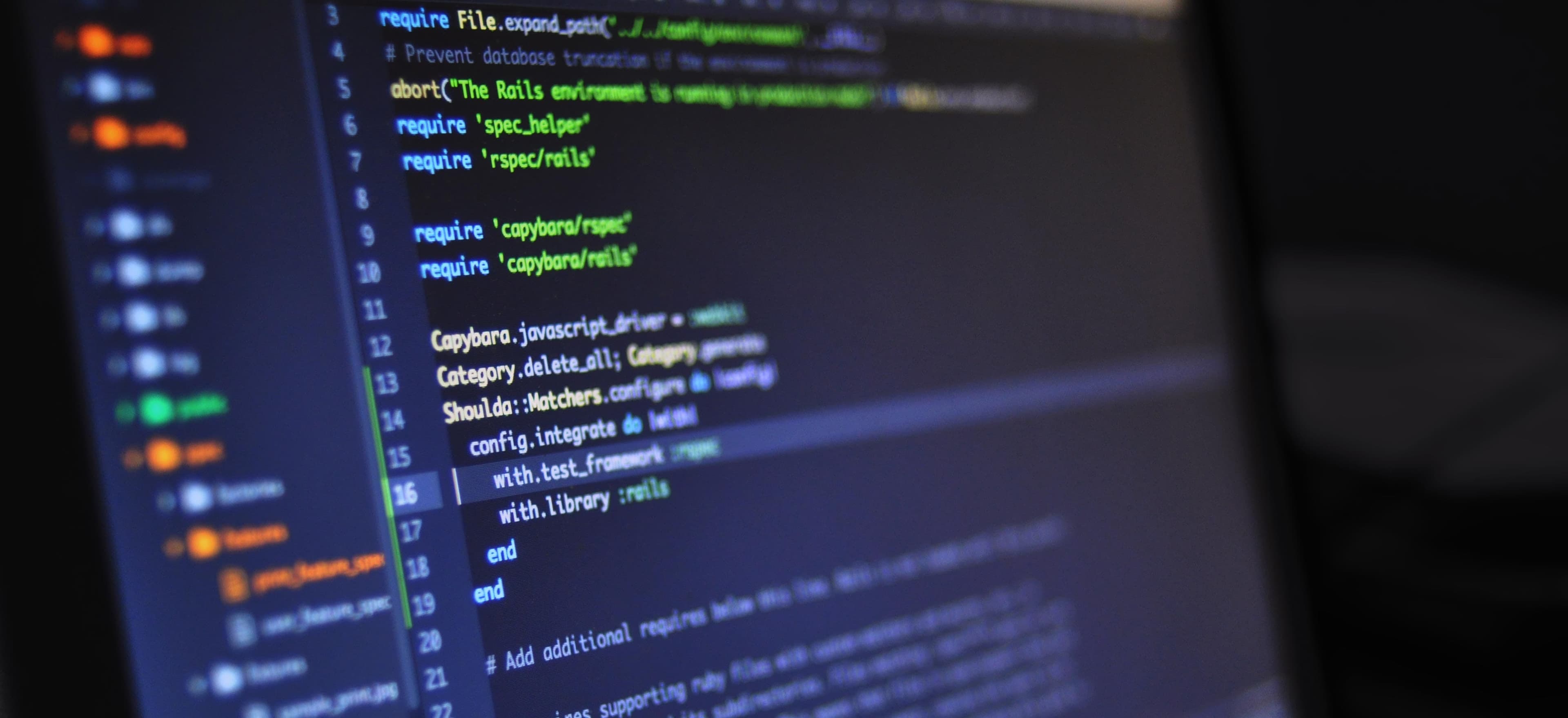
- Published on
Handling JSON with Java: A Guide to Database Integration
JSON (JavaScript Object Notation) has become the standard data interchange format for web applications. Its lightweight nature and human-readable structure make it a preferred choice for transmitting data between a client and a server. As applications grow, developers encounter scenarios where they need to store and manipulate JSON data within relational databases. This blog post will discuss how to handle JSON in Java when interfacing with databases, offering practical code snippets and best practices.
Why JSON?
Before diving into the integration of JSON with Java and databases, let’s explore why JSON has gained widespread acceptance:
- Flexibility: JSON supports a varied data structure that allows complex data types, making it suitable for hierarchical data storage.
- Interoperability: JSON is language-agnostic, which means it can be consumed by various environments like Node.js, Python, and, of course, Java.
- Human-Readable: Its simplicity allows developers and non-developers alike to understand the data structure quickly.
Connecting Java to a Database
To manipulate JSON data, we need a Java application that can communicate with a database. We'll use JDBC (Java Database Connectivity) for database operations. Below is an example of how to establish a connection to a MySQL database.
Example: Establishing a Database Connection
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DatabaseConnection {
private static final String URL = "jdbc:mysql://localhost:3306/your_database";
private static final String USER = "your_username";
private static final String PASSWORD = "your_password";
public static Connection connect() {
try {
Connection conn = DriverManager.getConnection(URL, USER, PASSWORD);
System.out.println("Database connected successfully!");
return conn;
} catch (SQLException e) {
System.err.println("Connection failed: " + e.getMessage());
return null;
}
}
}
Explanation
In this snippet, we create a DatabaseConnection
class that uses JDBC to connect to a MySQL database. It uses DriverManager
to handle the connection. If successful, it returns a Connection
object; if not, it captures the SQLException
and prints an error message.
Storing JSON in MySQL
Managing JSON in MySQL has become more accessible with the introduction of JSON as a data type in MySQL 5.7. You can store JSON documents in a JSON
column, which allows you to perform efficient queries and manipulations.
Creating a Table with a JSON Column
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(100),
data JSON
);
This SQL statement creates a users
table with three columns: id
, name
, and a data
column of type JSON
. Now we can store structured data about users.
Inserting JSON Data into the Database
Let’s insert a JSON object into our MySQL table from Java. We'll use the PreparedStatement
class to avoid SQL injection.
Example: Inserting JSON Data
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class InsertJSON {
public static void insertUser(Connection conn, String name, String jsonData) {
String sql = "INSERT INTO users (name, data) VALUES (?, ?)";
try (PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setString(1, name);
pstmt.setString(2, jsonData);
pstmt.executeUpdate();
System.out.println("User inserted successfully!");
} catch (SQLException e) {
System.err.println("Error inserting user: " + e.getMessage());
}
}
}
Explanation
In insertUser
, we prepare an SQL statement to insert user data. The jsonData
variable holds the JSON string that will be stored in the database. By using PreparedStatement
, we prevent SQL injection vulnerabilities while inserting data.
Sample JSON Data
Here’s an example of a JSON object you might insert:
{
"email": "john.doe@example.com",
"preferences": {
"newsletter": true,
"notifications": false
}
}
To insert this data, call the insertUser
method like this:
String jsonData = "{\"email\":\"john.doe@example.com\",\"preferences\":{\"newsletter\":true,\"notifications\":false}}";
InsertJSON.insertUser(DatabaseConnection.connect(), "John Doe", jsonData);
Querying JSON Data
Once you’ve stored JSON data in your database, querying it efficiently becomes crucial. MySQL provides several functions to handle JSON data.
Example: Fetching JSON Data
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class FetchJSON {
public static void fetchUsers(Connection conn) {
String sql = "SELECT id, name, data FROM users";
try (PreparedStatement pstmt = conn.prepareStatement(sql);
ResultSet rs = pstmt.executeQuery()) {
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
String jsonData = rs.getString("data");
System.out.println("ID: " + id + ", Name: " + name + ", Data: " + jsonData);
}
} catch (SQLException e) {
System.err.println("Error fetching users: " + e.getMessage());
}
}
}
Explanation
In this snippet, we execute a SELECT statement to fetch user records. Each record is printed to the console, demonstrating how to retrieve JSON data.
Manipulating JSON within MySQL
MySQL enables direct manipulation of JSON data, allowing you to access and update JSON attributes directly via SQL. For instance, you can query specific attributes from JSON objects using the JSON_EXTRACT
function, as discussed in the article "Manipulating JSON Arrays in MySQL Tables".
Example: Extracting Data from JSON
SELECT name, JSON_EXTRACT(data, '$.email') AS email
FROM users;
This SQL query selects the name and email from the JSON structure. Using JSON_EXTRACT
, you can handle your JSON data easily.
Key Takeaways
Handling JSON with Java and its integration into relational databases, particularly MySQL, is a powerful duo for developers. JSON enables flexible and complex data structures, while JDBC provides a clean way to interact with databases.
By following the examples provided, you can set up a robust system for storing and manipulating JSON data effectively.
If you want to dive deeper into JSON manipulation within MySQL, check out the article "Manipulating JSON Arrays in MySQL Tables". This knowledge will empower you to handle various use cases efficiently, leveraging the full potential of JSON data structures in your Java applications.
Happy coding!
Checkout our other articles