Mastering JSON in Java for Efficient MySQL Integration
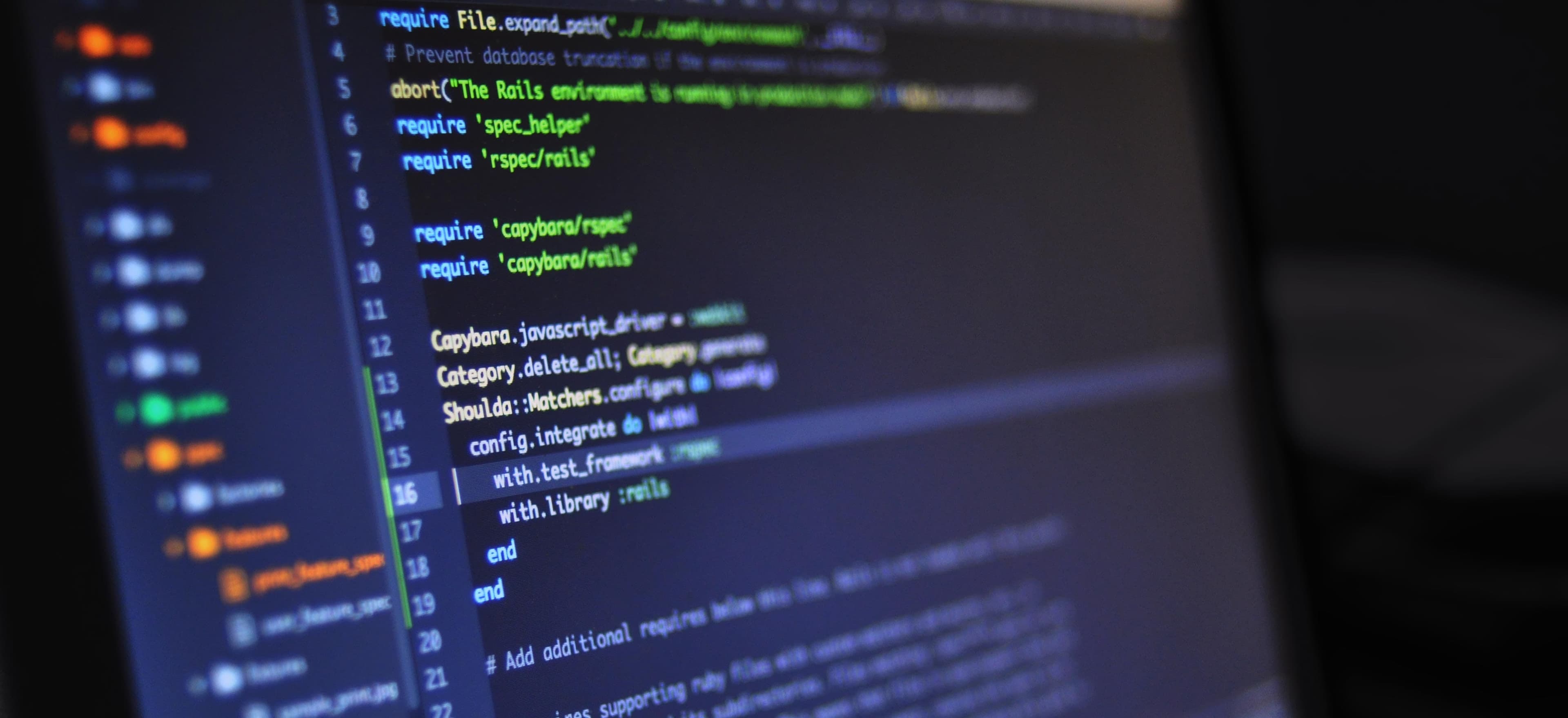
- Published on
Mastering JSON in Java for Efficient MySQL Integration
In today's data-driven world, integrating Java applications with MySQL databases, especially when handling JSON data, is becoming increasingly crucial. JSON (JavaScript Object Notation) is not just a lightweight data interchange format; it has become a standard for many web services and applications. In this article, we will explore the effective manipulation of JSON in Java while integrating with MySQL databases.
Why JSON?
JSON is favored for a multitude of reasons:
- Human-Readable: JSON is easy to read and write, making it an excellent choice for configuration files and data exchange.
- Language Agnostic: Supported by almost every programming language, JSON is versatile.
- Hierarchical Structure: JSON allows for nesting of data, making it easier to represent complex data structures.
In the realm of MySQL, JSON support means that we can store, retrieve, and manipulate JSON data directly within our tables, facilitating dynamic applications.
Setting Up Your Java Environment
Before we dive into JSON manipulation, let’s ensure we have the necessary environment setup. You will need:
- Java JDK: At minimum, version 8 is recommended.
- MySQL Server: Version 5.7 or later for JSON support.
- Maven: To manage project dependencies.
- IDE: Such as Eclipse or IntelliJ IDEA for coding.
Let’s add the required dependencies to your Maven pom.xml
file:
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.26</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.12.3</version>
</dependency>
The mysql-connector-java
dependency allows us to connect to MySQL, while jackson-databind
facilitates working with JSON.
Creating a MySQL Table with JSON Data
To take advantage of MySQL's JSON capabilities, let’s create a simple table to store JSON objects.
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(255) NOT NULL,
data JSON
);
In this example, we define a table called users
that has a data
column of the JSON type. This is where we’ll store user-specific data such as preferences and settings.
Working with JSON in Java
Now, let's see how to insert and retrieve JSON data from the users
table using Java.
Inserting JSON Data
Here’s a basic example of inserting data into the MySQL table:
import com.fasterxml.jackson.databind.ObjectMapper;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
public class JsonInsert {
public static void main(String[] args) {
ObjectMapper objectMapper = new ObjectMapper();
// Creating a sample JSON object
UserData user = new UserData("dark_mode", true, 15);
try {
String jsonData = objectMapper.writeValueAsString(user);
// Database connection setup
Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/yourdb", "username", "password");
String query = "INSERT INTO users (name, data) VALUES (?, ?)";
PreparedStatement statement = connection.prepareStatement(query);
statement.setString(1, "John Doe");
statement.setString(2, jsonData);
statement.executeUpdate();
System.out.println("User inserted: " + user);
} catch (Exception e) {
e.printStackTrace();
}
}
}
class UserData {
private String preferenceType;
private boolean enabled;
private int size;
public UserData(String preferenceType, boolean enabled, int size) {
this.preferenceType = preferenceType;
this.enabled = enabled;
this.size = size;
}
// Getters and setters
}
Explanation of the Code
- ObjectMapper: We use Jackson’s ObjectMapper to convert Java objects to JSON strings effortlessly.
- Connection: Establish a connection to the MySQL database.
- PreparedStatement: This allows us to run parameterized queries, which prevent SQL injection.
- Insert JSON: Finally, we serialize our
UserData
object into a JSON string and insert it into the database.
Retrieving JSON Data
Retrieving JSON data is slightly more straightforward. Here’s how it can be accomplished:
import com.fasterxml.jackson.databind.ObjectMapper;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
public class JsonRetrieve {
public static void main(String[] args) {
ObjectMapper objectMapper = new ObjectMapper();
try {
// Database connection setup
Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/yourdb", "username", "password");
String query = "SELECT data FROM users WHERE name=?";
PreparedStatement statement = connection.prepareStatement(query);
statement.setString(1, "John Doe");
ResultSet resultSet = statement.executeQuery();
if (resultSet.next()) {
String jsonData = resultSet.getString("data");
UserData user = objectMapper.readValue(jsonData, UserData.class);
System.out.println("Retrieved User Data: " + user);
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
What Happens Here?
In the retrieval code:
- PreparedStatement: Again, we use prepared statements for safety.
- ResultSet: This is used to store the result of our query. Upon executing, we check if there are results, and if so, we retrieve our JSON string.
- Deserialization: We convert the JSON string back into a
UserData
object.
Advanced JSON Manipulation in MySQL
MySQL provides several functions for manipulating JSON data directly in SQL. For example, if you want to retrieve a specific key from the JSON document, you can do:
SELECT JSON_UNQUOTE(JSON_EXTRACT(data, '$.preferenceType')) AS preferenceType FROM users WHERE id = 1;
This will return the value of preferenceType
from the JSON stored in the data
column. By employing such functions, you can effectively work with JSON data without the need for extensive data handling in Java.
For more extensive discussions on working with complex JSON data in MySQL, check out the article titled Manipulating JSON Arrays in MySQL Tables.
The Last Word
Mastering JSON in Java is integral for modern applications, particularly those integrating with databases like MySQL. The power to seamlessly store, retrieve, and manipulate JSON data helps developers create responsive, dynamic applications that meet user needs effectively.
As we've seen, using libraries like Jackson and the JSON capabilities offered by MySQL can dramatically simplify how we handle data. Start implementing these techniques today, and you'll quickly find your applications benefiting from cleaner data structures, improved performance, and enhanced user experiences.
By leveraging the techniques discussed in this article, you can harness the full power of JSON in your Java applications, which is integral for effective MySQL integration. Happy coding!
Checkout our other articles