Avoiding Common Pitfalls in Java Shell Scripting
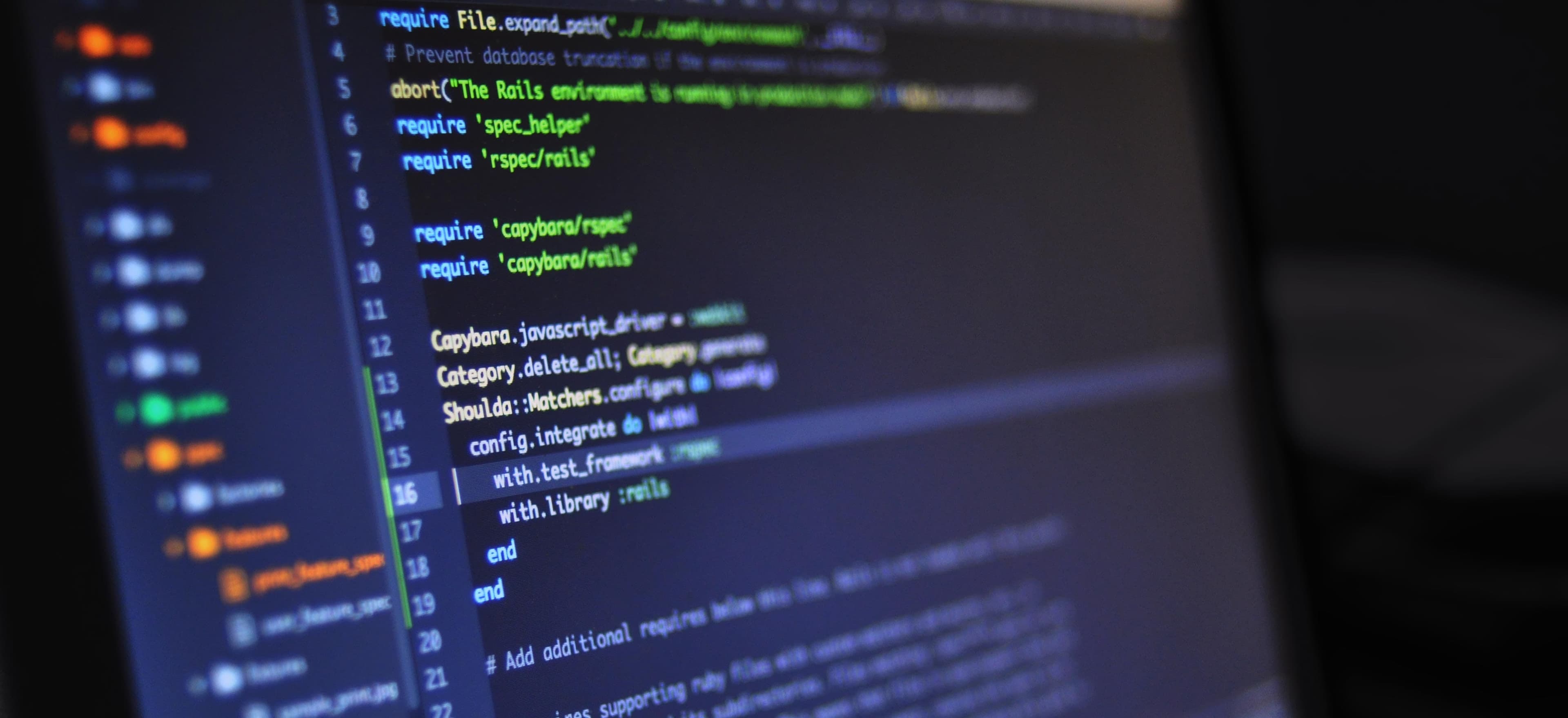
- Published on
Avoiding Common Pitfalls in Java Shell Scripting
In the ever-evolving landscape of software development, the synergy between Java and shell scripting presents numerous opportunities for developers to enhance their productivity. However, as with any powerful tool, there are common pitfalls that can lead to frustration and inefficiency. In this blog post, we will explore these pitfalls in detail, offering best practices to overcome them while writing Java shell scripts. Additionally, we will reference useful resources such as Mastering Shell Scripts: Overcoming Common Beginner Mistakes (which you can find at configzen.com/blog/mastering-shell-scripts-beginner-mistakes) for further reading.
Understanding the Basics of Shell Scripting in Java
Before diving into the common pitfalls of Java shell scripting, it's crucial to understand what shell scripting entails. Shell scripts are built to automate repetitive tasks in a UNIX-like environment. When combined with Java, shell scripts can run Java applications, manage resources, and even conduct error handling. However, in this ecosystem, developers may encounter several challenges.
Common Pitfalls in Java Shell Scripting
1. Environment Misconfiguration
Issue: One of the most frequent issues is misconfigured environments. When running Java applications from a shell script, your script needs to know where the Java executable is located. If the path is incorrect, your script may fail without clear error messages.
Solution: Always set the JAVA_HOME environment variable in your script, and echo it to confirm the path.
#!/bin/bash
# Set JAVA_HOME
export JAVA_HOME=/usr/lib/jvm/java-11-openjdk-amd64
# Check for correct JAVA_HOME path
if [ -d "$JAVA_HOME" ]; then
echo "JAVA_HOME is set to $JAVA_HOME"
else
echo "JAVA_HOME is not set correctly. Please check the path."
exit 1
fi
By confirming the JAVA_HOME path, you reduce the chance of runtime errors.
2. Hard-Coding Values
Issue: Another common mistake is hard-coding values into your scripts, leading to inflexible and difficult-to-maintain code. This makes it challenging to adapt to changes in the environment or configurations.
Solution: Use parameters and configuration files instead of hard-coding values.
#!/bin/bash
# Use parameters for flexibility
JAR_FILE=$1
if [ -z "$JAR_FILE" ]; then
echo "Please provide the path to the JAR file."
exit 1
fi
java -jar "$JAR_FILE"
This approach facilitates easier updates as no changes are needed in the script when configurations vary.
3. Ignoring Exit Codes
Issue: Developers often overlook exit codes in shell scripting. When a command in a shell script fails, it usually returns an exit status other than zero, indicating an error.
Solution: Check the exit codes after running commands to handle failures gracefully.
#!/bin/bash
# Execute a Java program
java -jar my-application.jar
# Capture exit status
EXIT_STATUS=$?
if [ $EXIT_STATUS -ne 0 ]; then
echo "Java application failed with exit status $EXIT_STATUS"
exit $EXIT_STATUS
fi
Monitoring exit statuses helps ensure that errors are captured and addressed promptly.
4. Not Using set -e
Issue: Another misstep involves not using the set -e
command at the beginning of scripts. This command causes scripts to exit immediately if a command fails.
Solution: Enable set -e
to avoid running commands after a failure is detected.
#!/bin/bash
# Exit the script if any command fails
set -e
# Run Java program
java -jar my-application.jar
This leads to safer script execution and reduces unexpected behavior in your program.
5. Poor Input Validation
Issue: Input validation is often a neglected aspect of scripting. If user input is not validated correctly, it could lead to runtime errors or security vulnerabilities.
Solution: Implement thorough input validation to ensure your scripts behave as expected.
#!/bin/bash
# Input validation
if [ "$#" -ne 1 ]; then
echo "Usage: $0 <path-to-jar>"
exit 1
fi
JAR_FILE="$1"
if ! [[ -f "$JAR_FILE" ]]; then
echo "Error: File '$JAR_FILE' does not exist."
exit 1
fi
# Run the Java application
java -jar "$JAR_FILE"
By validating inputs rigorously, we can avert potential runtime errors and improve user interactions.
6. Not Using Comments
Issue: Skipping comments in scripts can lead to confusion for those who may read or maintain the code later. It can be especially troublesome in complex scripts.
Solution: Use comments generously to explain the purpose of each section or complicated logic.
#!/bin/bash
# This script runs a Java application specified by the user
# Set JAVA_HOME variable
export JAVA_HOME=/usr/lib/jvm/java-11-openjdk-amd64
# Check if the required arguments are provided
if [ "$#" -ne 1 ]; then
echo "Usage: $0 <path-to-jar>"
exit 1
fi
# Run the Java application
java -jar "$1"
Comments can enhance readability and maintainability, making it easier for others—or even yourself—to revisit the code later.
7. Ignoring Long-Running Processes
Issue: When using shell scripts to run long-running Java applications, it's crucial to either monitor the process or run it in a way that allows you to reattach later.
Solution: Use tools like nohup
, screen
, or tmux
to manage long-running applications.
#!/bin/bash
# Run the Java application in the background
nohup java -jar my-application.jar &
echo "Java application is running in the background. Check nohup.out for logs."
Using nohup
allows your application to continue running even if the current session ends.
The Bottom Line
Java shell scripting comes with its share of pitfalls, but understanding these challenges can drastically improve your scripting efficiency. By adhering to best practices—such as configuring your environment correctly, avoiding hard-coded values, checking exit codes, validating inputs, and documenting your code—you can write better shell scripts that are robust, reliable, and easier to maintain.
For further insights into avoiding common beginner mistakes, you may also want to read Mastering Shell Scripts: Overcoming Common Beginner Mistakes available at configzen.com/blog/mastering-shell-scripts-beginner-mistakes.
Armed with these strategies, you should be well-equipped to navigate the landscape of Java shell scripting, avoiding common pitfalls and enhancing your overall development experience. Happy scripting!
Checkout our other articles