SpringRunner vs SpringBootTest: Choosing the Right Tool
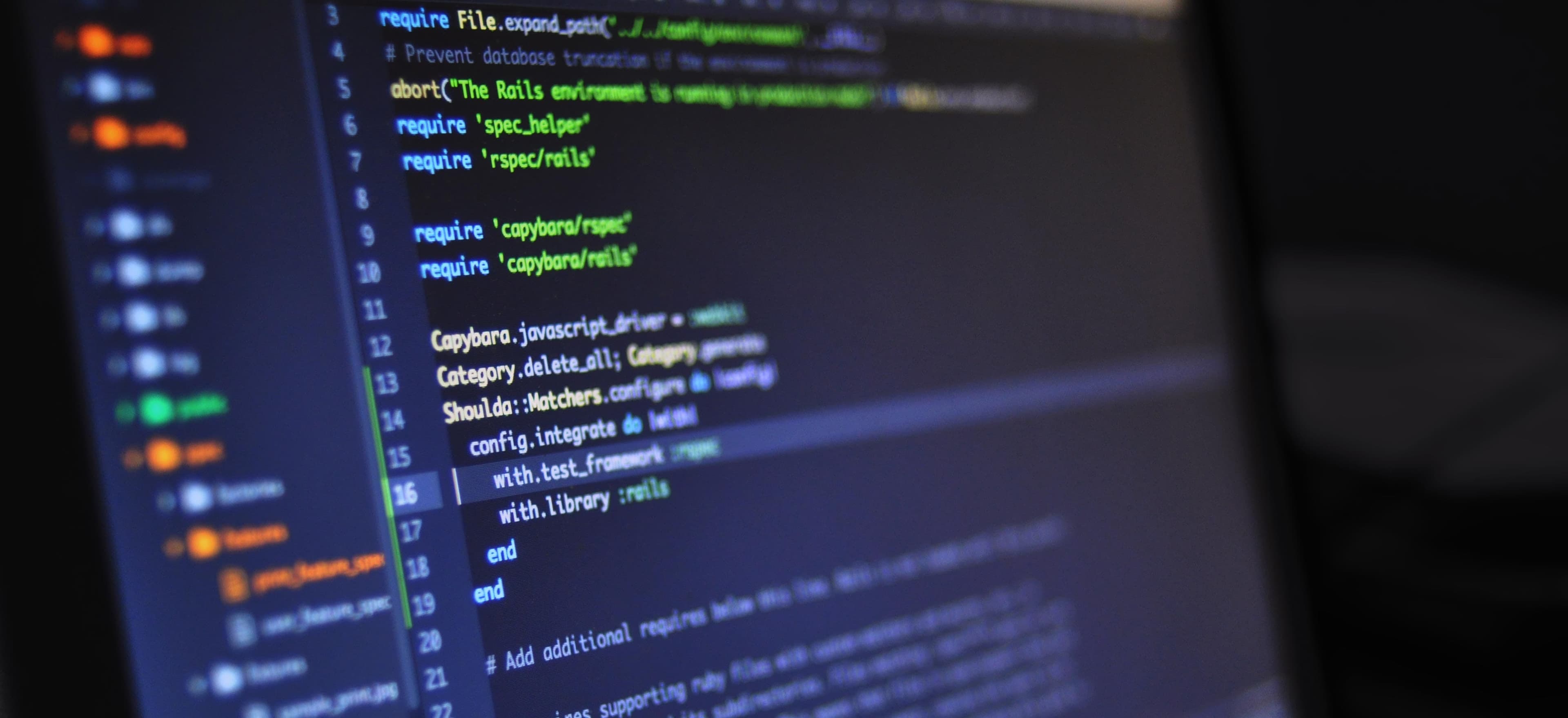
- Published on
SpringRunner vs SpringBootTest: Choosing the Right Tool
When it comes to testing Spring applications, developers are often faced with the choice between using SpringRunner
and SpringBootTest
. Both play crucial roles in the testing lifecycle, but they serve different purposes. Understanding the differences between these two tools can lead to more effective testing, quicker feedback loops, and ultimately a more robust application.
In this blog post, we'll dive deep into the features, benefits, and scenarios for using SpringRunner
and SpringBootTest
, with practical code snippets and explanations to illustrate their applications.
What is SpringRunner?
SpringRunner
is a testing framework that integrates the Spring TestContext Framework into JUnit. By using SpringRunner
, we can test Spring components in a more controlled environment. It provides support for loading the Spring ApplicationContext and enabling dependency injection within our test cases.
Key Features of SpringRunner:
- Context Management:
SpringRunner
ensures that your application context is loaded and reused during your tests, which saves time. - Dependency Injection: It allows you to inject Spring-managed beans directly into your test code.
- Integration with JUnit: Being a JUnit runner, it provides all the benefits of JUnit alongside Spring’s testing features.
Sample Code Using SpringRunner
Here's a sample snippet demonstrating how to use SpringRunner
:
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.SpringRunner;
@RunWith(SpringRunner.class)
@ContextConfiguration(classes = {YourSpringConfiguration.class})
public class MyServiceTest {
@Autowired
private MyService myService;
@Test
public void testServiceMethod() {
String result = myService.performAction();
assertEquals("Expected Result", result);
}
}
Why Use SpringRunner?
Using SpringRunner
is ideal when you want to test individual components, ensure that your configuration is correct, and validate the behavior of your Spring beans without launching the entire Spring Boot application.
For more information about how to set up Spring testing configurations, check out the Spring Framework documentation.
What is SpringBootTest?
SpringBootTest
, on the other hand, is a specialized annotation for Spring Boot applications. It provides a more comprehensive testing approach, integrating the Spring Boot test features into JUnit 5 or JUnit 4 tests by leveraging the larger application context.
Key Features of SpringBootTest:
- Full Application Context: It can load the complete application context, including all beans and configurations.
- Web Environment Support:
SpringBootTest
offers options to run the test with a mock servlet environment, making it useful for testing controllers and web endpoints. - Configurability: You can customize the test environment further using properties, mock beans, and profiles.
Sample Code Using SpringBootTest
Here's an example illustrating how to use SpringBootTest
:
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.TestPropertySource;
import static org.assertj.core.api.Assertions.assertThat;
@SpringBootTest
@TestPropertySource(locations = "classpath:application-test.properties")
public class MyControllerTest {
@Autowired
private MyController myController;
@Test
public void testControllerEndpoint() {
String response = myController.handleRequest();
assertThat(response).isEqualTo("Expected Response");
}
}
Why Use SpringBootTest?
SpringBootTest
is your go-to option when you need to run integration tests or when you want to test the entire application stack together. It's especially beneficial for testing the behavior of controllers, services, or repositories interacting as a whole.
For more nuanced comprehension of your testing strategies with Spring Boot, navigate to the Spring Boot Testing documentation.
Comparing SpringRunner and SpringBootTest
Now that we've explained both concepts, let’s draw a direct comparison to help you make an informed decision:
| Feature | SpringRunner | SpringBootTest | |------------------------|------------------------------------|----------------------------------| | Application Context | Loads specific context | Loads full application context | | Usage | Unit testing individual components | Integration testing with entire stack | | Dependency Injection | Supports DI for Spring beans | Supports DI including mock or real beans | | Testing Layer | More suited for service/repo testing | Ideal for controller and full service tests | | Configuration | Custom configuration via annotations | Custom properties, profiles, etc. |
When to Use Each
- Use SpringRunner When:
- You want to isolate a service or repository for unit testing.
- Your focus is on specific bean behavior rather than end-to-end functionality.
- Use SpringBootTest When:
- You need to validate the interaction between multiple components.
- You are testing controller endpoints or need a complete context for integration tests.
Bringing It All Together
Both SpringRunner
and SpringBootTest
are powerful tools in the Spring ecosystem, each with its distinctive approach to testing.
By analyzing the features and appropriate contexts for their use, you can significantly enhance your testing strategy. Remember to choose wisely based on the type of testing you are conducting.
In conclusion, when deciding between SpringRunner
and SpringBootTest
, ask yourself: What are you testing? What's your objective? The right choice can lead to more effective tests and, ultimately, a more reliable application.
Happy coding, and may your tests run smoothly!