Navigating Common Challenges in REST API Automation Testing
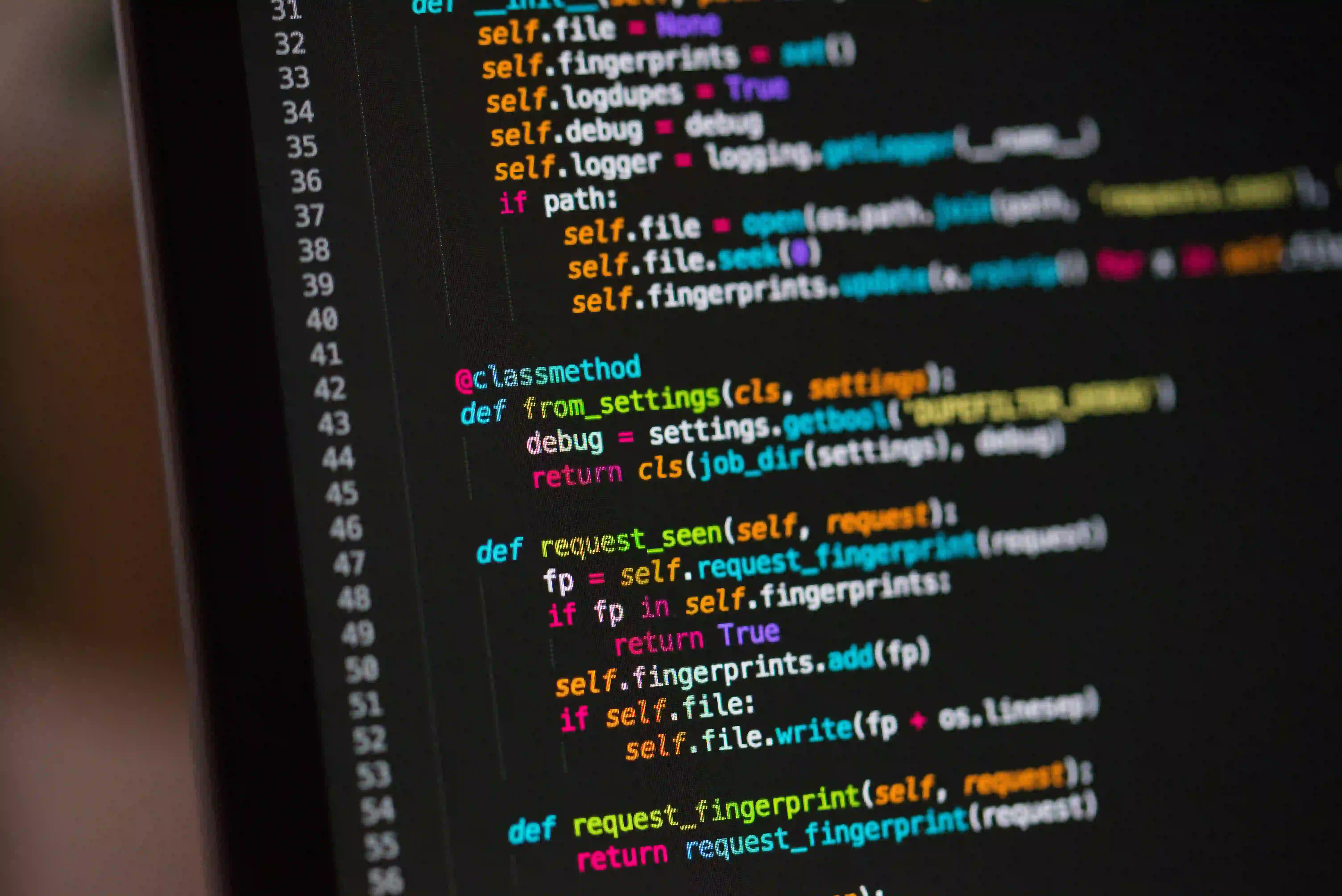
Navigating Common Challenges in REST API Automation Testing
In today's technology-driven world, REST APIs have become the backbone of web services. They facilitate communication between client and server, making our applications more flexible and scalable. However, with the rise in API usage comes the challenge of ensuring these APIs function correctly and efficiently. This is where automation testing enters the scene, helping developers and QA engineers deliver high-quality software. In this blog post, we will discuss common challenges faced during REST API automation testing and provide practical solutions to navigate them.
Understanding REST API Testing
Before diving into the challenges, it's essential to understand what REST API testing involves. REST (Representational State Transfer) APIs are designed to handle various types of requests, including GET, POST, PUT, and DELETE. Testing these APIs ensures they return the expected responses, handle errors gracefully, and operate correctly under various scenarios.
Key Objectives of REST API Testing
- Verification of API Responses: Ensure the API returns the correct data in the expected format.
- Performance Testing: Test the API under load conditions to identify any performance bottlenecks.
- Security Testing: Identify vulnerabilities in the API that could lead to data breaches or other security issues.
- Reliability Testing: Ensure that the API is dependable and functions as expected under various conditions.
Common Challenges in REST API Automation Testing
1. Dynamic Data Handling
One of the primary challenges in REST API testing is handling dynamic data. APIs often return data that changes frequently, which can lead to flaky tests if not managed properly.
Solution: Use Mock Servers
Mock servers can simulate API responses, allowing you to run tests consistently without the need for real-time data. Tools like WireMock or Postman can help set this up.
// Example of using WireMock to create a mock server
WireMockServer wireMockServer = new WireMockServer(8080);
wireMockServer.start();
stubFor(get(urlEqualTo("/api/resource"))
.willReturn(aResponse()
.withHeader("Content-Type", "application/json")
.withBody("{ \"id\": 1, \"name\": \"test\" }")));
This code snippet sets up a WireMock server that returns a consistent JSON response for a GET request. By using mock servers, you can focus tests on specific scenarios without worrying about the external data.
2. Complex Authentication Mechanisms
Modern APIs often require complex authentication methods such as OAuth2 or JWT. Automating tests against these APIs can be cumbersome and error-prone.
Solution: Use Libraries for Authentication
Many libraries simplify the process of handling authentication. For Java-based projects, using libraries like RestAssured can make the process seamless.
import io.restassured.RestAssured;
RestAssured
.given()
.auth()
.oauth2("YOUR_ACCESS_TOKEN")
.when()
.get("https://api.example.com/protected/resource")
.then()
.statusCode(200);
This code shows how to send a GET request with an OAuth2 token using RestAssured. This approach enables you to keep your tests clean while handling authentication effectively.
3. Insufficient Test Coverage
Another challenge is ensuring comprehensive test coverage that encompasses all possible scenarios, including edge cases and error conditions.
Solution: Implement Test-Driven Development (TDD)
Leveraging TDD encourages you to write tests before the actual code. This methodology helps identify edge cases early in the development lifecycle.
@Test
public void testGetUserById_NotFound() {
given()
.pathParam("id", "999")
.when()
.get("/api/users/{id}")
.then()
.statusCode(404)
.body("error", equalTo("User not found"));
}
This JUnit test checks that a 404 status is returned when trying to retrieve a user that doesn’t exist. By adopting TDD, you ensure that your tests cover a wide range of scenarios.
4. Lack of Documentation
Well-documented APIs ease the testing process. However, many APIs lack comprehensive documentation, making it challenging to understand the endpoints and expected behaviors.
Solution: Use API Documentation Tools
Tools like Swagger or Postman provide clear documentation of your APIs and allow you to generate test cases directly from these specifications.
Here’s how you might use Swagger to document an API:
swagger: "2.0"
info:
description: "API documentation for user management"
version: "1.0.0"
title: "User API"
paths:
/api/users/{id}:
get:
summary: "Get a user by ID"
parameters:
- name: id
in: path
required: true
type: string
responses:
200:
description: "User found"
404:
description: "User not found"
Using such tools ensures you have a reference that helps reduce guesswork during testing. Review Swagger for more insights on API documentation.
5. Environment Configurations
Testing environments must closely mimic production settings. Environment discrepancies can lead to faulty tests, thereby causing inconsistencies between development and production.
Solution: Use Containerization
Leveraging Docker to create consistent and reproducible environments can help mitigate these issues. By running your API tests in Docker containers, you can ensure consistency across different stages of development.
# Dockerfile example to set up a testing environment
FROM openjdk:11-jre-slim
WORKDIR /usr/src/app
COPY . .
CMD ["java", "-jar", "my-api-tests.jar"]
The above Dockerfile sets up a slim runtime environment for your Java application. Keeping your test environment consistent across your development lifecycle leads to more reliable automation testing.
6. Performance Testing
Automation testing often focuses on functionality, but performance testing is equally crucial. Failing to assess how an API performs under load can lead to user dissatisfaction.
Solution: Implement Load Testing Tools
Using tools like JMeter or Gatling can help simulate high traffic to your APIs and measure response times effectively.
// Example load test configuration with Gatling
object UserAPISimulation extends Simulation {
val httpProtocol = http.baseUrl("https://api.example.com")
val scn = scenario("User API Load Test")
.exec(http("Get User")
.get("/api/users/1"))
setUp(
scn.inject(atOnceUsers(100))
).protocols(httpProtocol)
}
This code defines a simulation that sends requests to the API and provides insights into how it handles concurrent users. Load testing ensures that your API can handle the expected user demand efficiently.
In Conclusion, Here is What Matters
REST API automation testing is crucial in modern software development. By navigating the challenges such as dynamic data, complex authentication mechanisms, insufficient test coverage, lack of documentation, inconsistent environments, and performance issues, you can build reliable and high-quality APIs.
Whether you're utilizing mock servers, adopting TDD, using API documentation tools, or implementing load testing, countless practices can enhance your automation strategy. Ensure your APIs are robust, because a well-tested API is foundational to successful applications.
For further reading, consider these resources:
Together, let's strive to improve API quality and ensure a smoother user experience!