Common Spring Boot & Angular Integration Pitfalls
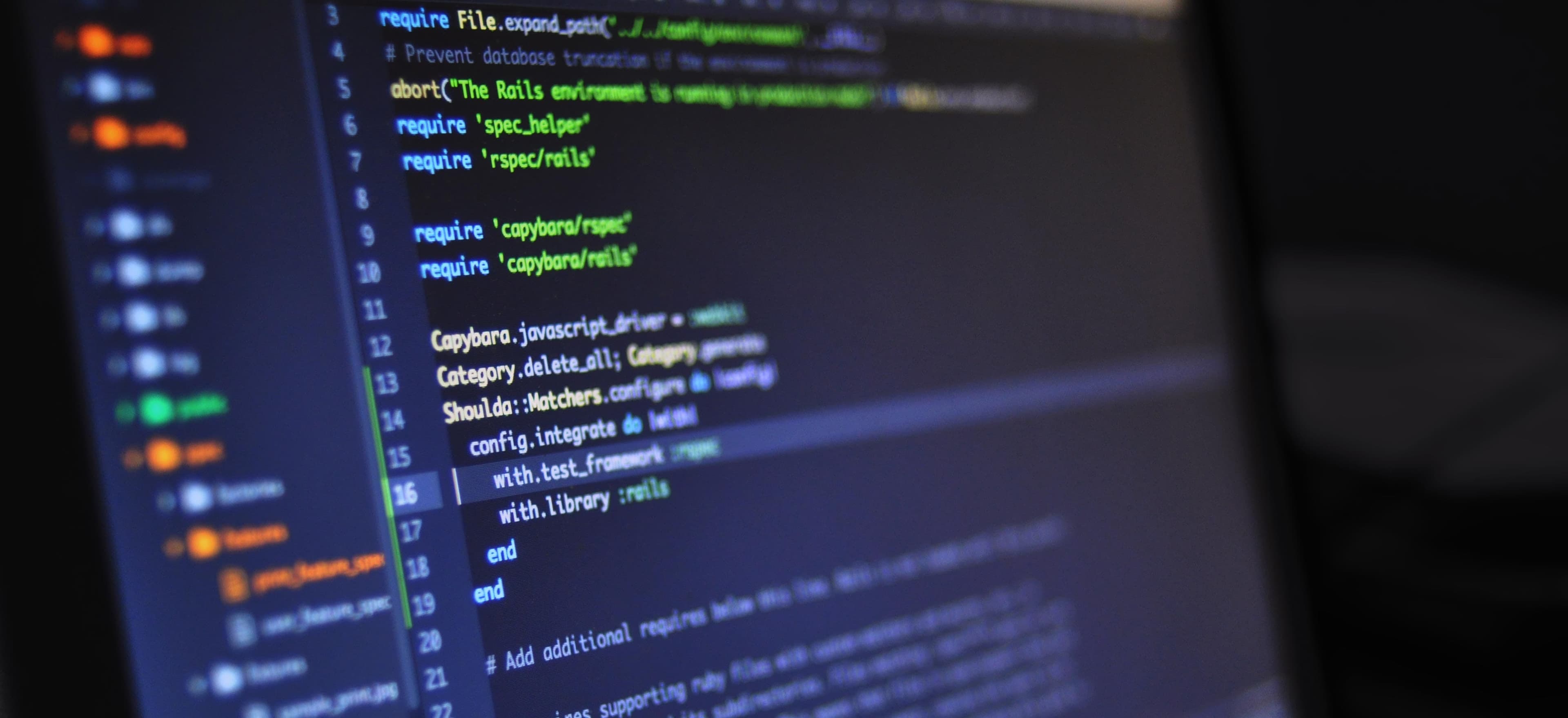
- Published on
Common Spring Boot & Angular Integration Pitfalls
Integrating Spring Boot and Angular can create a powerful combination for developing modern web applications. Spring Boot handles the backend efficiently, while Angular provides a highly responsive front end. However, like any technology stack, there are several pitfalls developers commonly face during integration. This post will discuss these challenges and offer practical solutions, ensuring a smooth integration process.
Table of Contents
- Understanding CORS Issues
- API Versioning
- Data Binding Mismatches
- Error Handling Consistency
- Security with JWT
- Not Using Environment-Specific Properties
- Inefficient API Calls
- Conclusion
Understanding CORS Issues
Cross-Origin Resource Sharing (CORS) issues emerge when your Angular front end makes requests to the Spring Boot back end hosted on a different origin. Browsers block these requests by default for security reasons.
Solution
To resolve this, you need to configure CORS in your Spring Boot application. Here’s how you can do that:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/api/**") // Specify the paths to allow CORS.
.allowedOrigins("http://localhost:4200") // Your Angular app's URL.
.allowedMethods("GET", "POST", "PUT", "DELETE", "OPTIONS")
.allowedHeaders("*")
.allowCredentials(true);
}
}
Why This Matters
Enabling CORS allows your Angular app to communicate seamlessly with your Spring Boot API. Failing to do so results in frustrating errors that can deter development.
API Versioning
Another common pitfall is neglecting API versioning. APIs evolve over time, and without proper version management, breaking changes can lead to front-end applications malfunctioning.
Solution
Implement versioning in your API endpoints:
@RestController
@RequestMapping("/api/v1/users") // Versioning in the URL
public class UserController {
@GetMapping
public ResponseEntity<List<User>> getAllUsers() {
// Logic to retrieve users
}
}
Why This Matters
Versioning your APIs creates stability and allows you to introduce new versions without breaking existing clients. It makes your application more maintainable and user-friendly.
Data Binding Mismatches
Data binding mismatches happen when the structure of the JSON returned from the Spring Boot API does not match what the Angular app expects. This can lead to null values or runtime errors in your Angular application.
Solution
Always align your DTO (Data Transfer Object) structures in Spring with your Angular models. Here’s an example of a DTO in Spring Boot:
public class UserDTO {
private Long id;
private String name;
private String email;
// Getters and Setters
}
And the corresponding Angular model:
export class User {
constructor(public id: number, public name: string, public email: string) {}
}
Why This Matters
Consistent data structures will enhance data integrity across both the front end and back end, preventing runtime errors and improving the user experience.
Error Handling Consistency
Inconsistent error handling can lead to a frustrating experience for users. For instance, if an error occurs on the server, and the front-end does not handle it properly, the application may crash or display confusing messages.
Solution
Set up a global exception handler in Spring Boot:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<String> handleResourceNotFound(ResourceNotFoundException ex) {
return ResponseEntity.status(HttpStatus.NOT_FOUND).body(ex.getMessage());
}
}
Then, in Angular:
this.http.get<User[]>(this.apiUrl).subscribe(
(data) => this.users = data,
(error) => {
console.error('API error:', error);
this.errorMessage = 'An error occurred loading users.';
}
);
Why This Matters
Consistent error handling ensures that users receive clear and understandable messages and that developers can debug issues efficiently. A well-thought-out error handling strategy contributes to a professional user experience.
Security with JWT
Security is paramount in any application, especially when dealing with user data. Using JWT (JSON Web Token) for authentication can help secure your Spring Boot APIs.
Solution
Generate a JWT when a user logs in:
public String generateToken(User user) {
return Jwts.builder()
.setSubject(user.getUsername())
.setIssuedAt(new Date())
.setExpiration(new Date(System.currentTimeMillis() + EXPIRATION_TIME))
.signWith(SignatureAlgorithm.HS256, SECRET_KEY)
.compact();
}
Then ensure your APIs are secured by validating the token in a filter:
public class JwtRequestFilter extends OncePerRequestFilter {
@Override
protected void doFilterInternal(HttpServletRequest request, HttpServletResponse response, FilterChain chain) {
// Validate token logic
chain.doFilter(request, response);
}
}
Why This Matters
Proper security with JWT not only protects your application from unauthorized access but also instills confidence in users regarding the safety of their data.
Not Using Environment-Specific Properties
Hardcoding configuration values directly in your code makes your application less flexible and harder to manage. By not using environment-specific properties, you run the risk of mistakenly deploying in the wrong environment.
Solution
Utilize Spring profiles to handle different configurations for development, testing, and production:
# application-development.properties
server.port=8080
jwt.secret=mysecretkey
In your Angular app, use environment files:
// environment.ts (development)
export const environment = {
production: false,
apiUrl: 'http://localhost:8080/api',
};
Why This Matters
Separating configurations based on the environment reduces the chances of errors and enhances the maintainability of your application.
Inefficient API Calls
Making too many API calls can severely affect the performance of your application. It’s essential to avoid unnecessary requests and ensure data is fetched efficiently.
Solution
- Batch Requests: Use batch APIs where appropriate.
- Caching: Implement caching at the server or client side.
Here’s an example of how to cache data in Angular:
@Injectable({
providedIn: 'root',
})
export class UserService {
private usersCache: User[];
constructor(private http: HttpClient) {}
getUsers(): Observable<User[]> {
if (this.usersCache) {
return of(this.usersCache);
}
return this.http.get<User[]>(this.apiUrl).pipe(
tap((data) => (this.usersCache = data))
);
}
}
Why This Matters
Efficient API calls reduce latency, improve user experience, and lower server load, making your application scalable.
The Closing Argument
Integrating Spring Boot with Angular can yield robust and maintainable applications, but navigating the common pitfalls is crucial. By understanding and mitigating CORS issues, implementing API versioning, ensuring data binding matches, managing errors effectively, securing your application with JWT, utilizing environment-specific properties, and optimizing API calls, you will set up a solid foundation for your development.
As you progress with your project, consider revisiting these areas to optimize and ensure a smooth integration. For more details on Spring Boot and Angular, check out the Spring Boot Documentation and the Angular Documentation. Happy coding!
Checkout our other articles