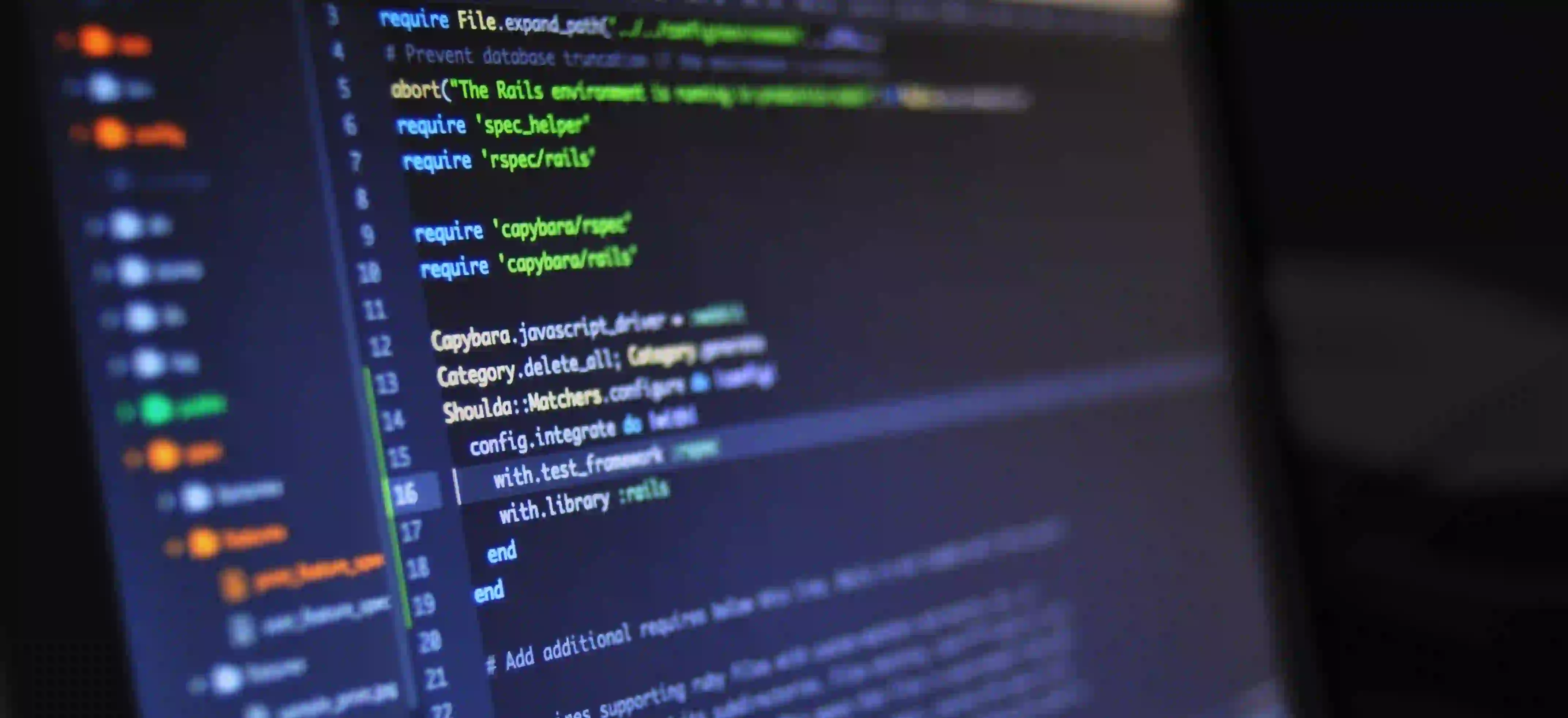
Why Your Spring Boot Password Encoder May Not Be Secure
In today’s world, where data breaches are frequent and identity theft is rampant, securing user credentials is paramount. One of the first lines of defense for any application is how it manages passwords. In the realm of Java applications, particularly those built with Spring Boot, the PasswordEncoder
interface is integral to password security. However, simply using a password encoder does not guarantee security. In this blog post, we will delve deep into why your Spring Boot password encoder may not be as secure as you think.
Understanding Password Encoding in Spring Boot
Before discussing potential pitfalls, let’s clarify what a password encoder is and how it works in Spring Boot. Spring Security provides a PasswordEncoder
interface that is responsible for encoding passwords before they are stored in a database. Here’s a common implementation using BCrypt:
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
public class UserService {
private BCryptPasswordEncoder passwordEncoder = new BCryptPasswordEncoder();
public String encodePassword(String rawPassword) {
return passwordEncoder.encode(rawPassword);
}
}
Why BCrypt?
BCrypt is widely recommended due to its adaptive nature; it is designed to be slow, which mitigates brute-force attacks. The slowness is adjustable via a work factor, allowing developers to increase resource requirements over time.
However, the implementation of BCrypt in the above example is only the first step. There are several aspects to consider to ensure that your password encoder is genuinely secure.
Potential Security Risks
1. Using Default Parameters
Often, developers might choose to use the default configuration of the BCryptPasswordEncoder
. While these defaults are a good starting point, they may not be sufficient for all applications. When you instantiate BCryptPasswordEncoder
without parameters, it uses a default strength of 10.
BCryptPasswordEncoder passwordEncoder = new BCryptPasswordEncoder(); // Strength is 10 by default
The Fix
To fortify security, consider increasing the strength factor based on your application’s needs. A recommended range is between 12 and 14. Here’s how to customize it:
int strength = 12; // Adjust the strength as needed
BCryptPasswordEncoder passwordEncoder = new BCryptPasswordEncoder(strength);
2. Not Updating the Encoder
As technology progresses, so do attacks and vulnerabilities. If your application was built several years ago, the default password encoding mechanisms may not be adequate anymore. If you have hard-coded your password encoder with a specific strength, that could become a problem.
The Fix
Regularly review your password encoding strategy and update your encoder’s strength, if necessary. Additionally, ensure that you can re-encode existing passwords with the new configurations without disrupting user access.
3. Lack of Salt Management
A critical aspect of secure password storage is the use of a unique salt for each password. While BCrypt automatically handles salting, not all encoders do.
The Fix
If you choose or need to implement a custom PasswordEncoder
, always ensure to include salting. Here’s how to securely manage salt:
import org.springframework.security.crypto.password.MessageDigestPasswordEncoder;
public class SecurePasswordEncoder implements PasswordEncoder {
private static final String SALT = "RandomSaltValue"; // Use a unique salt strategy
@Override
public String encode(CharSequence rawPassword) {
return new MessageDigestPasswordEncoder("SHA-256").encode(rawPassword.toString() + SALT);
}
@Override
public boolean matches(CharSequence rawPassword, String encodedPassword) {
return encodedPassword.equals(encode(rawPassword));
}
}
4. Improper Error Handling
Another often-overlooked aspect is how you handle authentication errors. Simply returning a generic "invalid credentials" message can give attackers hints about your system.
The Fix
Ensure that your error messages do not reveal too much. Use logging at a level that does not disclose sensitive user data while still allowing you to monitor anomalies.
5. Ignoring Password Policies
A common mistake is to allow weak passwords. Even a robust encoder cannot protect against weak passwords.
The Fix
Implement and enforce strong password policies. Ensure users create passwords that fulfill minimum criteria, such as:
- Length (at least 8-12 characters)
- A mix of uppercase and lowercase letters
- Inclusion of numbers and special characters
A Final Look
Securing user passwords is crucial, and while Spring Boot offers tools like PasswordEncoder
, developers must be vigilant. By being aware of the potential pitfalls such as using default settings, failing to manage salts, neglecting to update encoders, handling errors incorrectly, and ignoring password policies, you can significantly increase the security of your applications.
Incorporating techniques like those discussed in this post will better position your application against cyber threats. Security is an ongoing process, not a one-off task. Stay informed about the latest security practices and continuously evaluate your password management strategies.
For more information on Spring Security, consider visiting the official Spring Security Documentation. As threats evolve, so should your approach to securing user credentials.
Remember, a secure application is a success; don’t neglect the fundamentals. Start by evaluating your password handling now and safeguard your application against future vulnerabilities. Happy coding!