Unlocking Java Performance: JVM Tuning vs. Code Optimization
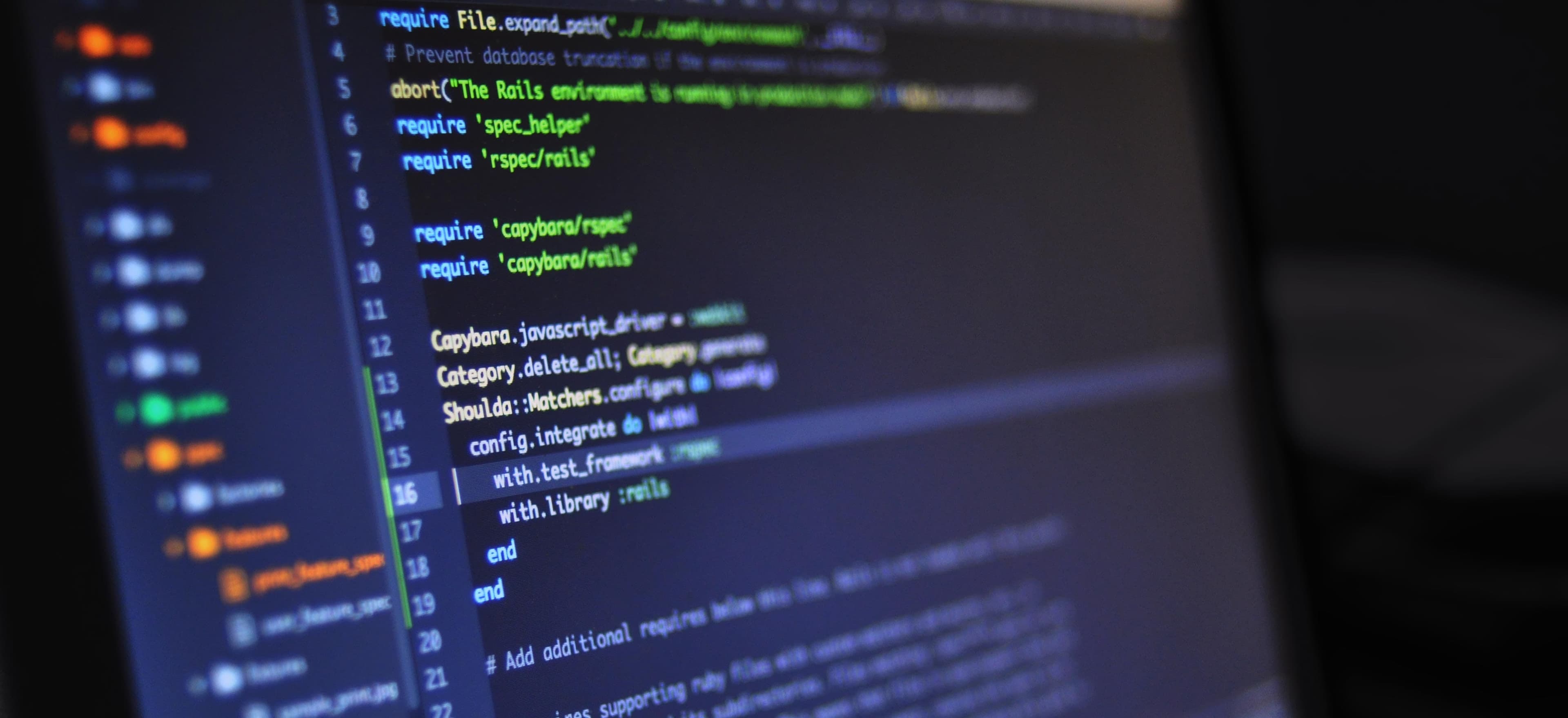
- Published on
Unlocking Java Performance: JVM Tuning vs. Code Optimization
When it comes to enhancing the performance of Java applications, two primary approaches come into play: JVM tuning and code optimization. Each plays an essential role in achieving optimal performance, but they differ significantly in terms of focus and methodology. This blog post will delve into the intricacies of both approaches, providing actionable insights, code snippets, and best practices.
Understanding the JVM
Before we dive into the specifics of tuning and optimization, it's crucial to have a solid understanding of the Java Virtual Machine (JVM). The JVM is an abstract computing machine that enables Java bytecode to run on any platform. It provides a crucial level of abstraction, memory management through garbage collection, and an execution environment.
JVM Characteristics
- Platform Independence: Write once, run anywhere (WORA).
- Automatic Memory Management: The JVM handles memory allocation and garbage collection.
- JIT Compilation: Just-In-Time compilation optimizes performance at runtime.
To delve deeper into the underlying mechanisms of the JVM, consider reviewing this detailed overview.
JVM Tuning
JVM tuning involves adjusting various parameters and settings of the JVM to improve application performance. This can include configuring heap size, garbage collection strategies, and other runtime options. Let us explore some key aspects of JVM tuning.
1. Heap Size Configuration
The heap is the area of memory used for dynamic memory allocation. For many applications, setting the right heap size is critical for optimal performance.
java -Xms512m -Xmx2048m -jar YourApp.jar
- -Xms: Sets the initial heap size.
- -Xmx: Sets the maximum heap size.
Why: Allocating too little memory can lead to frequent garbage collection cycles, degrading performance. Conversely, setting it too high can increase the time it takes to perform garbage collection.
2. Garbage Collection Tuning
Java 8 introduced various garbage collection strategies, including G1
, CMS
, and Z
garbage collections. Selecting the correct algorithm based on your application’s needs is paramount.
java -XX:+UseG1GC -jar YourApp.jar
- G1 Garbage Collector: Suitable for applications with large heaps that require low pause times.
Why: Different garbage collectors cater to different performance needs. Knowing when and how to adjust this can significantly impact throughput and latency.
For a more in-depth guide on garbage collectors, refer to this comprehensive documentation.
3. Thread Configuration
Multithreading can enhance performance but can also create performance bottlenecks if not configured appropriately.
java -XX:ParallelGCThreads=8 -jar YourApp.jar
- ParallelGCThreads: Configures the number of threads used for garbage collection.
Why: Increasing the number of threads can help utilize multicore processors effectively, reducing garbage collection pause times.
Tools for JVM Monitoring
Utilizing monitoring tools like VisualVM, JConsole, or even command-line tools like jstat
can provide valuable insights into memory usage, thread activity, and garbage collection performance.
Code Optimization
While JVM tuning is about tuning the environment in which Java runs, code optimization focuses on improving the performance of the Java code itself. Let's explore some strategies for optimizing Java code.
1. Algorithm and Data Structure Optimization
Selecting the right algorithm and data structure can drastically reduce runtime complexity.
List<Integer> numbers = new ArrayList<>();
for (int i = 0; i < 1000; i++) {
numbers.add(i);
}
Collections.sort(numbers);
Why: Here, using ArrayList
and sorting it uses a time complexity of O(n log n). Selecting more efficient structures or algorithms could reduce that further.
Consider revisiting the choice of algorithms in your application and analyzing their time complexity.
2. Avoiding Unnecessary Object Creation
Object creation in Java takes time and memory. Reducing unnecessary object creation can enhance performance.
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 1000; i++) {
sb.append("Some text").append(i);
}
String result = sb.toString();
Why: In this example, StringBuilder
is used instead of string concatenation. This reduces the number of intermediate String
objects, optimizing memory and time efficiency.
3. Using Caching Mechanisms
Implementing caching for frequently accessed data can significantly enhance performance.
private Map<String, String> cache = new HashMap<>();
public String getData(String key) {
if (cache.containsKey(key)) {
return cache.get(key);
}
String data = fetchDataFromDatabase(key);
cache.put(key, data);
return data;
}
Why: In this example, the fetch operation is minimized by attempting to retrieve the value from cache first. This can dramatically reduce database load and response times.
4. Concurrency and Parallelism
Java provides various tools for concurrency, such as the java.util.concurrent
package. Utilizing these can lead to performance improvements.
ExecutorService executor = Executors.newFixedThreadPool(10);
for (int i = 0; i < 100; i++) {
final int index = i;
executor.submit(() -> {
processTask(index);
});
}
executor.shutdown();
Why: By using an ExecutorService
, tasks are handled in parallel rather than sequentially, optimizing execution time.
5. Optimize I/O Operations
I/O operations can often be a bottleneck in Java applications. Using buffering and optimizing file access can help.
try (BufferedReader reader = new BufferedReader(new FileReader("input.txt"))) {
String line;
while ((line = reader.readLine()) != null) {
processLine(line);
}
}
Why: Buffered I/O greatly reduces the number of I/O calls and can speed up the file processing, leading to improved performance in I/O-heavy applications.
A Final Look
In summary, improving Java application performance involves a two-pronged approach: JVM tuning and code optimization. Tuning the JVM settings can help in managing resource allocation, while optimizing the code ensures efficient execution.
Employing both strategies in tandem can lead to significant performance gains.
Additional Resources
- Java Performance Tuning Guide
- Effective Java by Joshua Bloch
- Java Concurrency in Practice by Brian Goetz
Explore these resources to deepen your understanding and hone your Java performance skills. Happy coding!