Boost ApplicationContext Performance: Common Pitfalls to Avoid
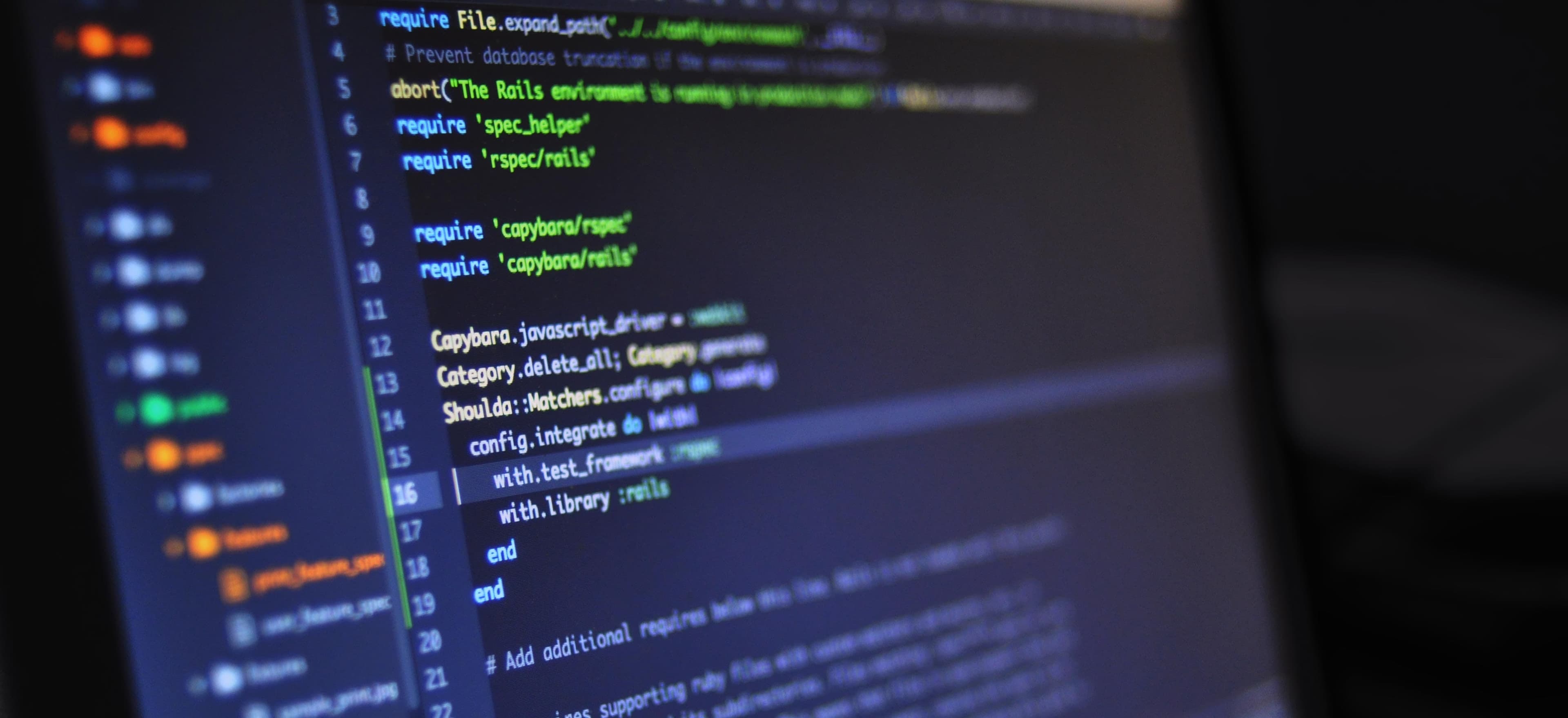
- Published on
Boost ApplicationContext Performance: Common Pitfalls to Avoid
In the world of Java development, the ApplicationContext is a crucial part of the Spring framework’s programming model. It enables you to manage beans, manage the lifecycle of your application components, and facilitate various configurations. However, if not used correctly, it can become a performance bottleneck. In this blog post, we will explore common pitfalls in using ApplicationContext and provide best practices to enhance performance.
Understanding ApplicationContext
Before diving into pitfalls, let us briefly understand the role of ApplicationContext. It serves as a central interface to the Spring framework, providing configuration information for an application. It handles the instantiation, configuration, and lifecycle management of application beans.
In Spring, the context can be configured to handle different concerns such as:
- Bean Management
- Event Propagation
- Resource Localization
- Integration with Spring AOP
Each of these functionalities can be heavy if not carefully managed. Let’s delve into common pitfalls that can hinder the efficiency of your ApplicationContext setup.
Pitfall 1: Prolific Bean Creation
One major performance concern arises from the excessive or improper bean creation. Developers often create singleton beans incorrectly, leading to multiple instances being created when only one is required.
Solution: Prefer Singleton Scope
Using singleton scope is often beneficial. By default, Spring beans are singleton-scoped. However, if you need different instances, consider using other scopes sparingly, as shown below:
@Component
@Scope("singleton") // Ensures only one instance is created
public class MyBean {
// Your bean's properties and methods
}
By ensuring that your application uses singleton beans where appropriate, you reduce overhead associated with object creation.
Additional Resources
- Learn more about Bean Scopes.
Pitfall 2: Ignoring Lazy Initialization
When it comes to loading beans, eager initialization can be a performance killer. This means that every single bean is loaded at startup, regardless of whether it's used immediately.
Solution: Enable Lazy Initialization
Enabling lazy initialization can significantly improve application startup time and reduce the memory footprint. You can achieve this with the following annotation:
@Configuration
public class AppConfig {
@Bean
@Lazy // This bean will be initialized only when first requested
public MyService myService() {
return new MyService();
}
}
Setting the single beans to lazy initialization prevents them from being created until they are explicitly needed.
Additional Resources
- Read more on Lazy Initialization in Spring.
Pitfall 3: Overusing Autowiring
While Spring's autowiring feature is powerful, using it excessively can lead to tight coupling and reduced clarity in your codebase. It can also increase the complexity of your dependency graph.
Solution: Use Constructor Injection
By favoring constructor injection over field injection, you can gain better control over bean dependencies. It provides more clear readability and enhances testability.
@Component
public class MyController {
private final MyService myService;
@Autowired
public MyController(MyService myService) {
this.myService = myService;
}
}
Using this approach, your dependencies remain clear, and you avoid the pitfalls of excessive autowiring.
Additional Resources
- Discover more about Dependency Injection.
Pitfall 4: Not Using Profiles
Managing different environments—development, testing, production—without using Spring profiles can complicate your configurations and slow down your application.
Solution: Define Profiles
By utilizing Spring profiles, you can create environment-specific configurations and load only what's necessary. Here is how you can define profiles:
@Configuration
@Profile("dev") // Only loaded in development profile
public class DevConfig {
@Bean
public MyService myService() {
return new MyServiceDevImpl();
}
}
@Configuration
@Profile("prod") // Only loaded in production profile
public class ProdConfig {
@Bean
public MyService myService() {
return new MyServiceProdImpl();
}
}
Using profiles can streamline context loading and make your application lighter and faster.
Additional Resources
- Learn more about Spring Profiles.
Pitfall 5: Mismanaging Resource Loader
The ResourceLoader is a key component of the Spring framework. It can load files, classpath resources, and more. However, mishandling this can lead to performance issues.
Solution: Optimize Resource Access Patterns
Instead of accessing resources repeatedly, consider caching them if they are used frequently. This prevents unnecessary I/O operations and can significantly improve performance.
@Service
public class FileService {
private Resource resource;
@Value("classpath:data/myfile.txt")
private ResourceLoader resourceLoader;
public void loadFile() throws IOException {
if (resource == null) {
resource = resourceLoader.getResource("classpath:data/myfile.txt");
}
// Processing the file
}
}
This approach not only reduces access time but also optimizes resource management.
Additional Resources
- Explore more on Spring Resource Loading.
Key Takeaways
Optimizing the performance of your ApplicationContext in Spring involves being aware of common pitfalls related to bean creation, initialization practices, dependency injection, profile management, and resource handling. By adopting best practices such as singleton scopes, lazy initialization, constructor injection, profile usage, and optimized resource loading, you can ensure that your application runs smoothly and efficiently.
For further reading and in-depth understanding, refer to the Spring Framework’s official documentation. Embrace these strategies, and you will notice marked improvements in your application's performance.
Happy coding!
Checkout our other articles