Unlocking Java's Complexity: Mastering Interfaces vs Abstract Classes
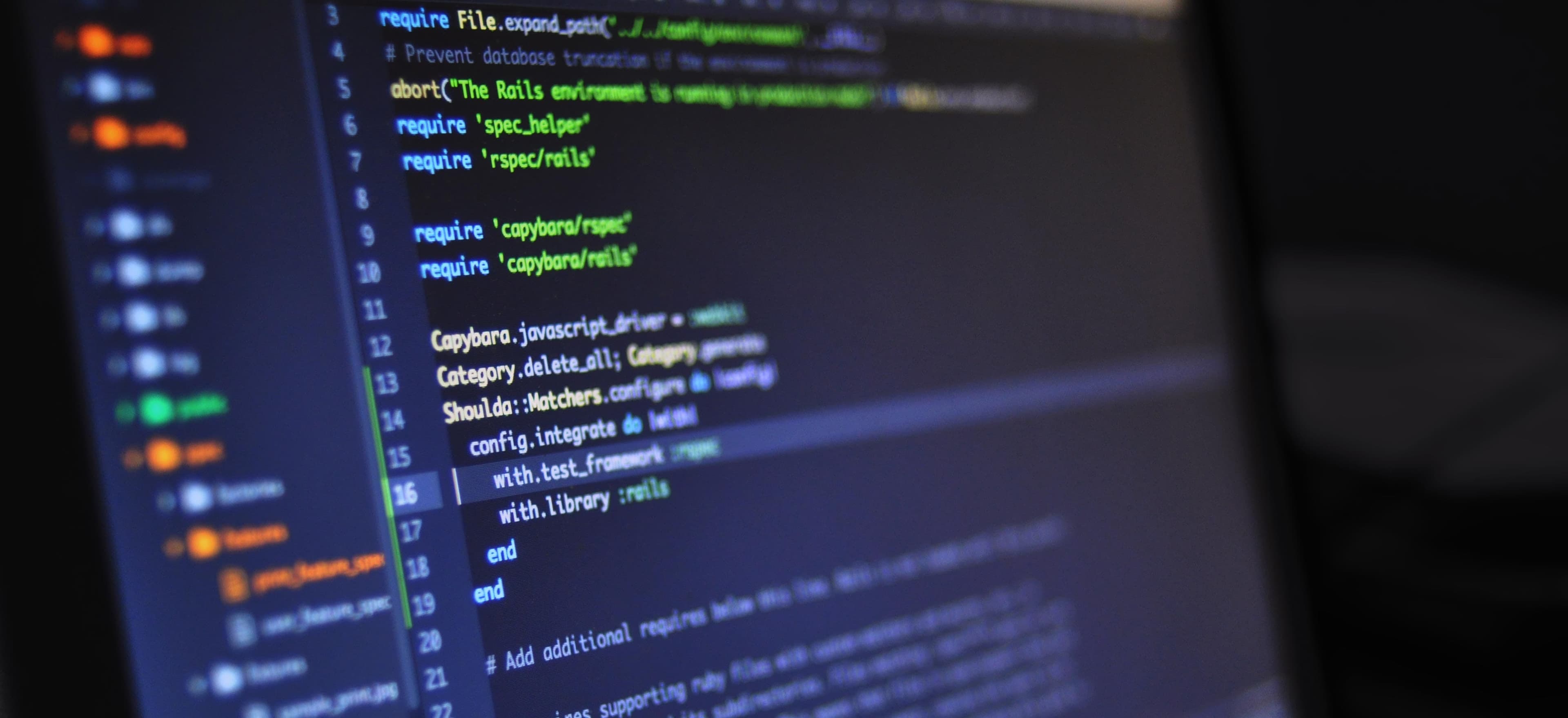
- Published on
Unlocking Java's Complexity: Mastering Interfaces vs Abstract Classes
Java is a powerful programming language that empowers developers to create flexible and reusable code. Two key concepts that form the backbone of Java's object-oriented design are interfaces and abstract classes. While they may seem similar at a glance, understanding their differences and appropriate usage can greatly enhance your programming prowess.
In this blog post, we will dive deep into the intricacies of interfaces and abstract classes in Java, providing clear examples and best practices along the way.
Table of Contents
- What are Interfaces?
- What are Abstract Classes?
- Key Differences Between Interfaces and Abstract Classes
- When to Use Interfaces
- When to Use Abstract Classes
- Conclusion
What are Interfaces?
An interface in Java is a reference type, similar to a class, that can contain only constants, method signatures, default methods, static methods, and nested types. Interfaces cannot contain instance fields or constructors.
Key Characteristics of Interfaces:
- Method Declarations: Interfaces can define methods but cannot provide implementations (until Java 8, where default methods were introduced).
- Multiple Inheritance: A class can implement multiple interfaces, allowing for a form of multiple inheritance.
- No State: Interfaces cannot maintain any state; they are purely a contract.
Example of an Interface
public interface Animal {
void eat();
void makeSound();
}
In the above example, the Animal
interface defines two method signatures: eat()
and makeSound()
. Any class that implements the Animal
interface must provide concrete implementations of these methods.
Implementation of the Interface
public class Dog implements Animal {
@Override
public void eat() {
System.out.println("Dog is eating");
}
@Override
public void makeSound() {
System.out.println("Woof");
}
}
In this code snippet, the Dog
class implements the Animal
interface and provides the necessary method definitions. By using interfaces, you allow for flexible class hierarchies and make it easier to switch implementations later.
What are Abstract Classes?
An abstract class in Java is a class that cannot be instantiated on its own. It can contain both fully defined methods (with implementations) and method signatures (without implementations). Abstract classes are often used as a base class where subclasses can derive common behavior.
Key Characteristics of Abstract Classes:
- Can Have State: Abstract classes can declare fields and maintain state.
- Default Implementation: They can provide default behavior, which can be overridden by subclasses.
- Single Inheritance: A class can only extend one abstract class, limiting the inheritance model.
Example of an Abstract Class
public abstract class Shape {
abstract void draw(); // abstract method
void area() { // concrete method
System.out.println("Calculating area");
}
}
In this code snippet, the Shape
class is defined as an abstract class. It has one abstract method (draw()
) that must be implemented by subclasses, and one concrete method (area()
) with a default implementation.
Implementation of the Abstract Class
public class Circle extends Shape {
@Override
public void draw() {
System.out.println("Drawing Circle");
}
}
The Circle
class extends the Shape
abstract class and implements the draw()
method. It can also take advantage of the area()
method inherited from the abstract class.
Key Differences Between Interfaces and Abstract Classes
Understanding the differences is crucial in deciding when to use each construct:
| Feature | Interface | Abstract Class | |---------------------------------|-----------------------------------------------|--------------------------------------------| | Implementation | No implementation until Java 8 (default methods allowed) | Can have different levels of implementation | | Inheritance | Multiple inheritance of interfaces allowed | Single inheritance of abstract classes | | Fields/State | Cannot have instance variables/state | Can have instance variables/state | | Access Modifiers | All methods are public by default | Can have any visibility (protected, public, private) | | Use Case | Define a contract for what a class can do | Base class for shared code and state |
When to Use Interfaces
- Defining a Contract: Use interfaces when you need to define a contract that multiple classes can implement, focusing on what the class can do rather than how it does it.
- Multiple Implementations: If your design requires a class to implement functionalities from multiple types, interfaces are the way to go.
- Flexibility: Interfaces provide flexibility in swapping implementations without changing the interface itself.
Example Use Case
public interface Payment {
void pay(double amount);
}
Classes like CreditCardPayment
, PaypalPayment
, etc., would implement the Payment
interface, providing different payment methods.
When to Use Abstract Classes
- Common Base: Choose an abstract class when you have a common base with shared code that you want subclasses to inherit.
- Shared State: If you need to maintain state that can be shared among subclasses, abstract classes are the choice.
- Default Behavior: When you want to provide a default implementation that subclasses can override, an abstract class may be the best option.
Example Use Case
public abstract class Vehicle {
protected String brand;
public Vehicle(String brand) {
this.brand = brand;
}
public abstract void start();
}
Classes like Car
and Truck
can extend Vehicle
, inheriting the brand
property while providing their specific implementation of the start()
method.
My Closing Thoughts on the Matter
Both interfaces and abstract classes are vital components of Java's object-oriented programming paradigm. Understanding the distinct characteristics, benefits, and use cases for each will enable you to design more robust, flexible, and maintainable applications.
As you continue your journey in mastering Java, keep practicing these concepts. Implement interfaces and abstract classes in your projects to solidify your understanding. You may also find additional resources helpful, such as Oracle's Java Documentation and Java's Abstract Classes Guide.
By mastering interfaces and abstract classes, you can unlock the full potential of Java and craft elegant, efficient solutions to complex problems. Happy coding!
Checkout our other articles