Mastering Java Generics: Avoiding Common Mapping Mistakes
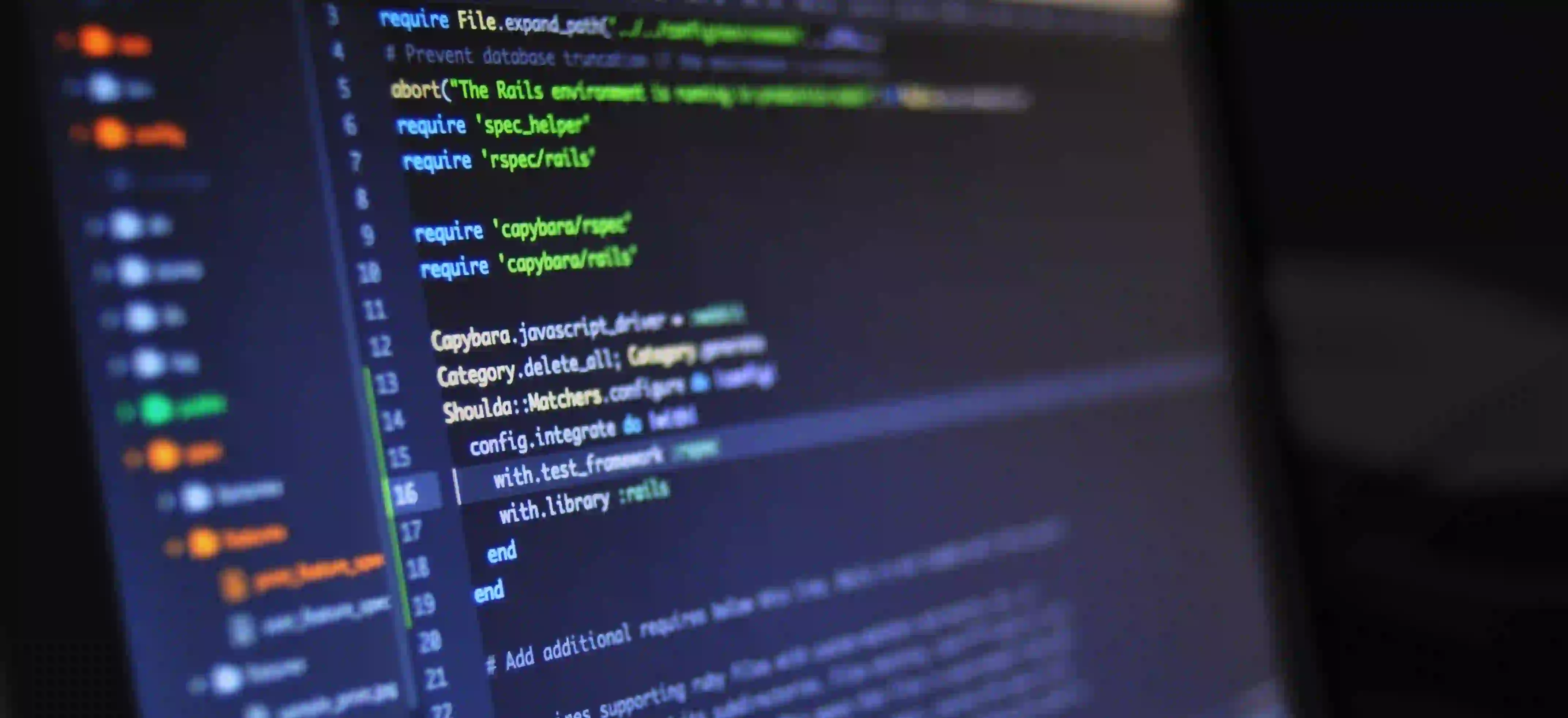
Mastering Java Generics: Avoiding Common Mapping Mistakes
Java Generics are a powerful feature that helps programmers create classes, interfaces, and methods with a placeholder for types. When used correctly, generics promote code reusability, maintainability, and type safety. However, they can also lead to common pitfalls, especially in mapping scenarios. In this blog post, we will explore these common mistakes and provide solutions to master Java Generics effectively.
Understanding Java Generics
Generics allow you to define a class with a type parameter that can be specified when the class is instantiated. This makes your code more flexible and type-safe, alleviating the need for type casting. Let’s look at a simple example:
public class Box<T> {
private T item;
public void setItem(T item) {
this.item = item;
}
public T getItem() {
return item;
}
}
Why Use Generics?
-
Type Safety: With generics, Java ensures that you only store the specified type within a data structure.
-
Code Reusability: A single class or method can operate on different types, eliminating the need to write several overloaded versions for different types.
-
Reduce Runtime Errors: Generics catch type-related errors at compile time, reducing runtime exceptions.
For further reading, Oracle's documentation provides a comprehensive overview of Generics in Java.
Common Mapping Mistakes
Mistake 1: Inconsistent Use of Wildcards
One common mistake in generic mapping is using wildcards inconsistently. Wildcards (like ? extends T
or ? super T
) allow you to create more flexible methods, but if misused, they can lead to confusion.
Incorrect Example:
public void processItems(List<?> items) {
// Trying to modify the items list will result in a compile-time error.
items.add(new Item()); // Error: Cannot add to a List<?>
}
Correct Approach:
To fix this, you should manage what you intend to do with the list. If you want to read items from the list, using List<? extends Item>
is appropriate. If you want to add items, use List<? super Item>
.
public void addItem(List<? super Item> items) {
items.add(new Item()); // This works because we can add Items to a list of parents.
}
Mistake 2: Forgetting to Specify Type Parameters
Another frequent mistake is neglecting to specify type parameters when interacting with Generic classes. This can lead to unchecked warnings and potential runtime errors.
Incorrect Example:
Box box = new Box(); // Raw type, no warning, but not type-safe.
box.setItem("Hello");
String item = (String) box.getItem(); // Cast might fail at runtime.
Correct Approach:
Always use the typed version of the class.
Box<String> box = new Box<>(); // Type-safe.
box.setItem("Hello");
String item = box.getItem(); // No cast needed, guaranteed safe.
Mistake 3: Misunderstanding Type Erasure
Java utilizes a concept called type erasure, where generic type information is removed at runtime. This means that the actual type parameters are not available, leading to potential issues when performing reflection.
Example:
If you defined two classes with different type parameters, they would be treated the same at runtime.
class GenericBox<T> {}
class StringBox extends GenericBox<String> {}
class IntegerBox extends GenericBox<Integer> {}
At runtime, StringBox
and IntegerBox
are both treated as GenericBox
.
Solution
To safely use generics in restricted scenarios, consider using interfaces or specific factory methods that retain the necessary type information, rather than relying solely on class generic parameters.
public class TypeFactory {
public static <T> GenericBox<T> createBox() {
return new GenericBox<>();
}
}
Practical Example: Mapping Data
Now let’s take a look at a more practical implementation where generics can be utilized in mapping data between two different object types.
Scenario
Imagine you have two classes: UserDTO
and UserEntity
. You want to map data from UserDTO
to UserEntity
.
public class UserDTO {
private String username;
private String email;
// Getters and Setters
}
public class UserEntity {
private String name;
private String emailAddress;
// Getters and Setters
}
Creating a Generic Mapper
You can create a generic mapper class to handle this mapping.
public class Mapper<T, U> {
private final Function<T, U> mappingFunction;
public Mapper(Function<T, U> mappingFunction) {
this.mappingFunction = mappingFunction;
}
public U map(T input) {
return mappingFunction.apply(input);
}
}
Implementation
Now you can create a specific mapping for the UserDTO
to UserEntity
.
Mapper<UserDTO, UserEntity> userMapper = new Mapper<>(dto -> {
UserEntity entity = new UserEntity();
entity.setName(dto.getUsername());
entity.setEmailAddress(dto.getEmail());
return entity;
});
And you can leverage the mapper.
UserDTO userDTO = new UserDTO();
userDTO.setUsername("john_doe");
userDTO.setEmail("john@example.com");
UserEntity userEntity = userMapper.map(userDTO);
Lessons Learned
Java Generics play an integral role in building robust, type-safe applications. Understanding and avoiding the common mapping mistakes we discussed can drastically improve both your code quality and the reliability of your applications.
Key Takeaways:
- Use wildcards intelligently.
- Always specify type parameters when using generic classes.
- Be mindful of type erasure, especially in reflection-based scenarios.
- Implementing generic mappers can greatly streamline data conversion between different types.
Additional Resources
To further enhance your understanding of Java Generics, consider checking out:
- Baeldung on Java Generics
- Vogella’s Guide to Java Generics
By mastering these concepts, you'll not only avoid common mistakes but also harness the full potential of Java's type system in your projects. Happy coding!