Common Pitfalls in Java Memory Model Optimization
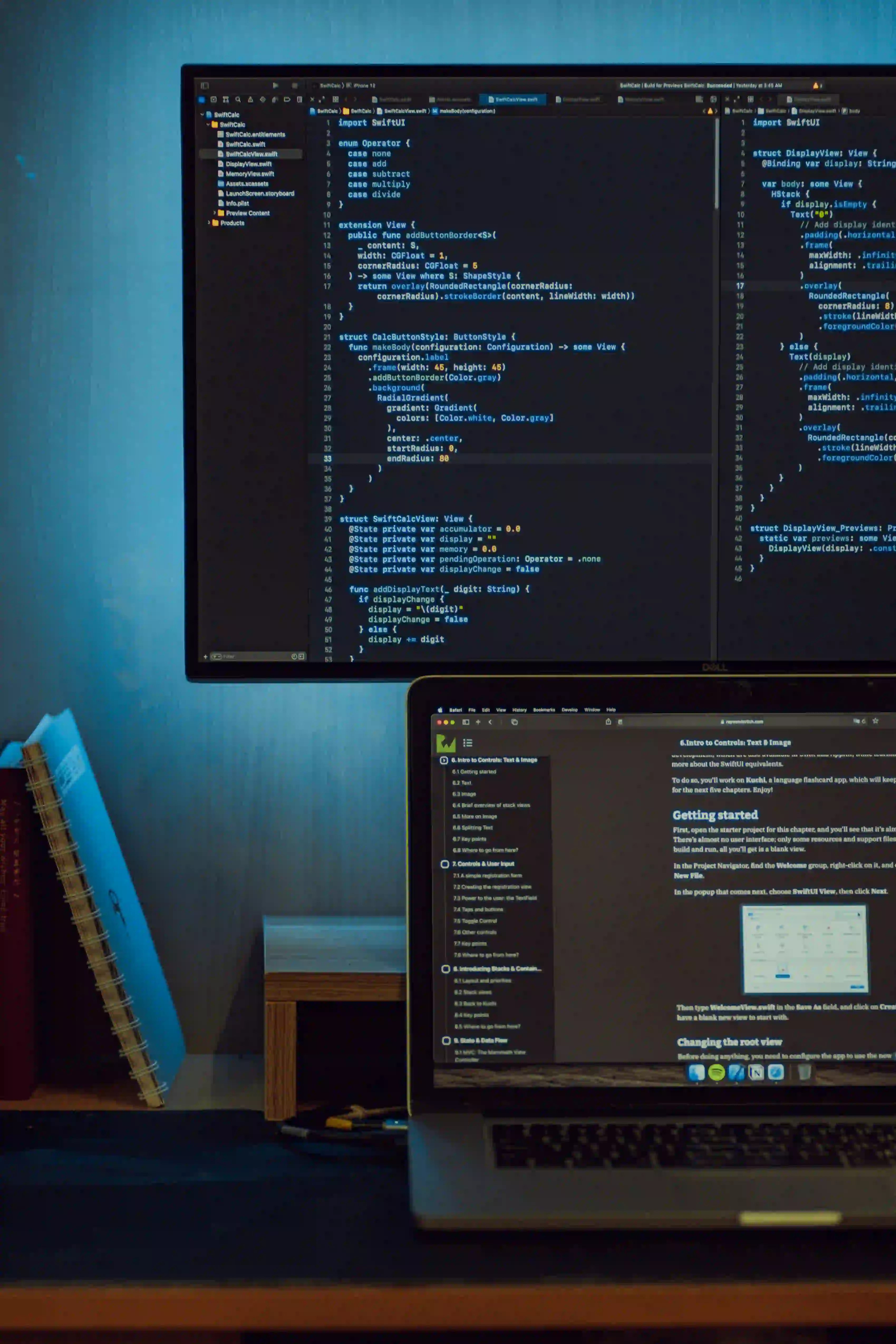
Common Pitfalls in Java Memory Model Optimization
When dealing with Java application performance, memory management plays a crucial role. Understanding the Java Memory Model (JMM) can significantly enhance an application’s execution speed and responsiveness. However, there are several pitfalls developers encounter when attempting to optimize memory usage. In this blog post, we will explore common mistakes, how they impact performance, and best practices for overcoming them.
Understanding the Java Memory Model
Before diving into pitfalls, let’s briefly touch upon what the Java Memory Model entails. The JMM defines how threads interact through memory. It describes the visibility of variables across threads, the ordering of operations, and how the execution of programs can lead to non-intuitive behaviors due to optimizations performed by the Java Virtual Machine (JVM) and CPU architecture.
To ensure reliable multi-threading in Java, you must understand the concepts of visibility, atomicity, and ordering. Here’s a simple breakdown:
- Visibility: Changes made by one thread are visible to other threads after a certain point (synchronization).
- Atomicity: Certain operations execute as a single unit without interference from other threads.
- Ordering: The execution order of operations might not always match the source code due to optimizations.
Now, let’s jump into the common pitfalls:
1. Overusing Synchronization
The Mistake
Synchronization is often necessary for shared variables, but overusing it can lead to poor performance. Excessive synchronization causes thread contention, significantly slowing down your application.
Example
Consider the following code:
public class Counter {
private int count = 0;
public synchronized void increment() {
count++;
}
public int getCount() {
return count;
}
}
Why It Matters
While the increment
method is thread-safe, every call requires acquiring a lock. If many threads try to access increment
, they must wait for one another, creating a bottleneck. A better approach may be:
import java.util.concurrent.atomic.AtomicInteger;
public class Counter {
private AtomicInteger count = new AtomicInteger(0);
public void increment() {
count.incrementAndGet();
}
public int getCount() {
return count.get();
}
}
Using AtomicInteger
allows you to maintain thread safety without traditional locking overhead.
2. Ignoring Escape Analysis
The Mistake
Escape analysis is a performance optimization that determines if an object can be allocated on the stack instead of the heap. When objects are allocated on the stack, garbage collection is no longer required, which can lead to performance benefits.
Example
Let’s say we have this code:
public class Person {
private String name;
public Person(String name) {
this.name = name;
}
public void display() {
System.out.println(name);
}
}
public void process() {
for (int i = 0; i < 1000; i++) {
Person person = new Person("John");
person.display();
}
}
Why It Matters
Here, the Person
object is created on the heap during each iteration. This way, every new instance contributes to heap memory pressure. By modifying the code to:
public void process() {
for (int i = 0; i < 1000; i++) {
display("John");
}
}
public void display(String name) {
System.out.println(name);
}
You eliminate unnecessary object creation, promoting better memory management.
3. Neglecting the Use of Final Variables
The Mistake
Final variables in Java are immutable; once assigned, they cannot be changed. Declaring variables as final can improve optimization because the compiler has more information about their state.
Example
Consider this situation:
public class Example {
private int variable; // not final
public void updateVariable(int newValue) {
variable = newValue;
}
}
Why It Matters
Here, other threads might read an old version of variable
if it gets updated by another thread. However, if variable
were final
:
public class Example {
private final int variable;
public Example(int variable) {
this.variable = variable;
}
public int getVariable() {
return variable;
}
}
Since it is final, threads will see the same consistent snapshot of the variable which enhances both readability and avoids synchronization overhead.
4. Incorrectly Using Collections
The Mistake
Using non-thread-safe collections in a multi-threaded environment can lead to unpredictable behavior. Instead, developers might use synchronized wrappers, which can degrade performance.
Example
Using synchronized collections can also lead to lock contention. Consider:
import java.util.Collections;
import java.util.List;
import java.util.ArrayList;
public class SynchronizedListExample {
private List<Integer> list = Collections.synchronizedList(new ArrayList<>());
public void add(Integer number) {
list.add(number);
}
}
Why It Matters
The synchronized version locks the entire list for every operation. A better approach is to utilize ConcurrentHashMap
or CopyOnWriteArrayList
to allow better concurrency.
import java.util.concurrent.CopyOnWriteArrayList;
public class ConcurrentListExample {
private CopyOnWriteArrayList<Integer> list = new CopyOnWriteArrayList<>();
public void add(Integer number) {
list.add(number);
}
}
The CopyOnWriteArrayList
performs better by allowing readers to access data without being blocked by writers.
5. Failing to Optimize Garbage Collection
The Mistake
Garbage Collection (GC) is a vital aspect of Java memory management. Failing to tune the GC settings can lead to performance bottlenecks in long-running applications.
Example
A naive approach would be to ignore GC settings entirely. An application with high object churn might end up in long GC pauses.
Why It Matters
Consider configuring the JVM parameters depending on your application needs. For example:
java -Xms256m -Xmx2g -XX:+UseG1GC -XX:MaxGCPauseMillis=50 -jar MyApp.jar
This command sets the initial and maximum heap sizes and specifies the G1 garbage collector, which is more efficient for applications requiring low pause times.
Final Considerations
Optimizing Java applications through effective memory management requires careful consideration of the Java Memory Model. Avoiding common pitfalls—such as overusing synchronization, neglecting escape analysis, failing to utilize final variables, improperly using collections, and overlooking garbage collection setup—can lead to significant performance improvements.
By applying best practices, leveraging the right data structures, and being mindful of memory allocation, you can develop applications that are not only efficient but also robust.
For further reading on Java performance tuning, consider visiting Oracle’s Java Performance Tuning Guide or the Java Concurrency Package Documentation to deepen your understanding of concurrency features.
Final Thoughts
The beauty of Java lies not only in its vast capabilities but also in the communities and resources surrounding it. Continue exploring, learning, and implementing best practices to harness the full potential of Java in your applications.
By understanding these common pitfalls, you are well on your way to becoming a more effective Java developer, adept at optimizing your applications for better performance and efficiency. Happy coding!