Common H2 Database Issues in Spring Boot Projects
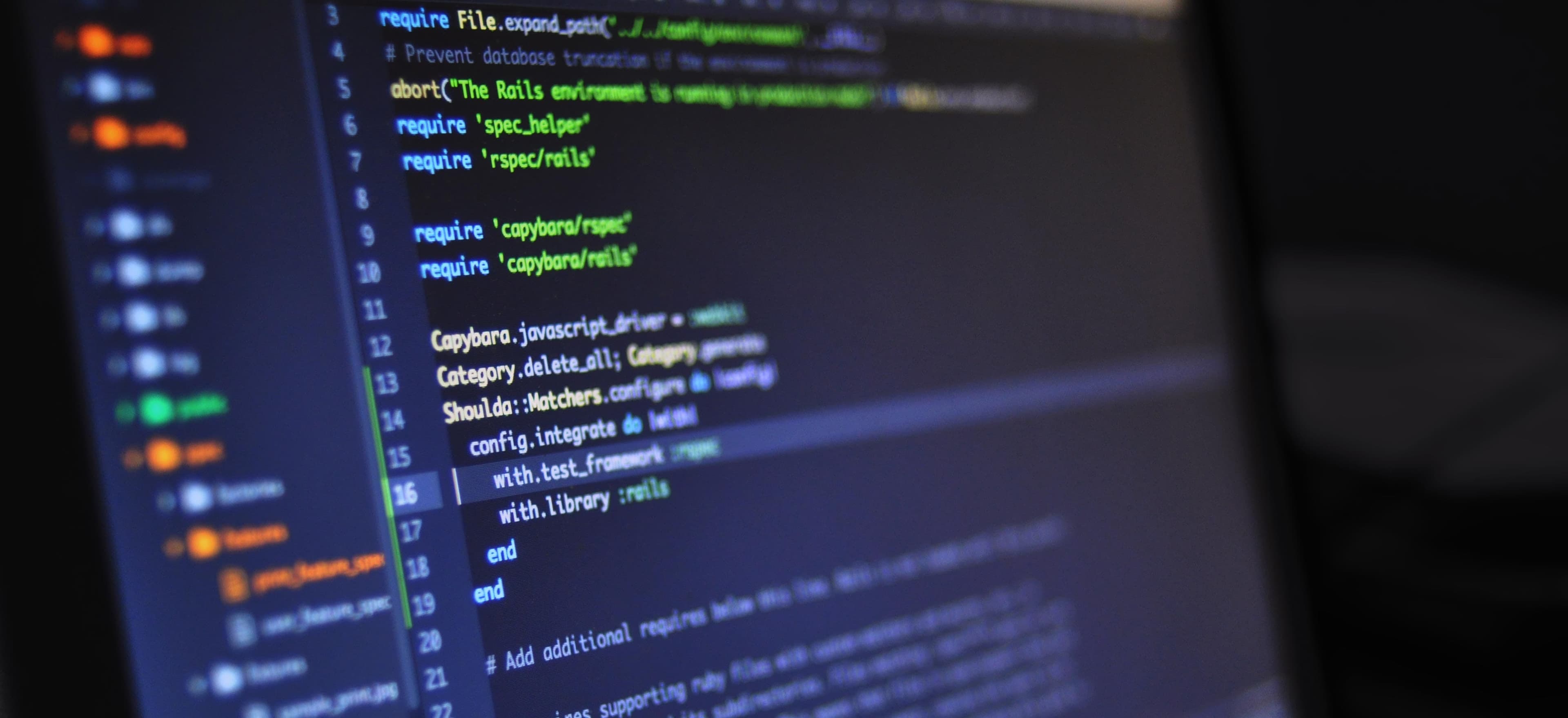
- Published on
Common H2 Database Issues in Spring Boot Projects
Spring Boot has revolutionized the way developers create and manage applications. Particularly for those working on smaller projects, the built-in H2 in-memory database can simplify development. However, as convenient as H2 may be, it is not without its challenges. In this blog post, we will explore some of the common H2 Database issues in Spring Boot projects and provide solutions to overcome them.
What is H2 Database?
H2 is an open-source, lightweight in-memory database written in Java. It is often used for testing and prototyping applications due to its fast performance and ease of setup. Since it can be embedded within Java applications, it's a popular choice for many developers working with Spring Boot.
Setting Up H2 in Spring Boot
Before diving into the common issues, let's ensure that you have H2 set up correctly in a Spring Boot application. You can integrate H2 using Maven with the following dependency in your pom.xml
:
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
Once you have this added, you can enable H2 console access in your application.properties
:
spring.h2.console.enabled=true
spring.h2.console.path=/h2-console
Now, you can access the H2 console at http://localhost:8080/h2-console
during development.
Common H2 Database Issues
1. Connection Issues
Description:
Connection problems to the H2 database are among the most frequently encountered issues. This could stem from incorrect URL configurations or server instability.
Solution:
Make sure your URL in application.properties
follows the correct structure. For example:
spring.datasource.url=jdbc:h2:mem:testdb
Why: The above configuration tells Spring Boot to create an in-memory database named testdb
every time the application runs.
2. Database Persistence
Description:
Many developers assume H2 will persist data once added, but by default, it's an in-memory database. Data is lost when the application stops.
Solution:
If you're looking for persistence, you can configure H2 to save data to the disk:
spring.datasource.url=jdbc:h2:file:./data/testdb
Why: The file:
option tells H2 to create a database file on disk, allowing data to persist between application restarts.
3. Data Not Reflecting in the Console
Description:
You might be able to connect and query your database, but the data you expect to see in the H2 console might not show up.
Solution:
Ensure that your repository methods or JPA operations are being performed properly and that the transactions are committed. For instance:
Entity entity = new Entity();
entity.setName("Test Name");
repository.save(entity);
Why: In Spring Data JPA, save operations are not automatically committed unless you are working within a transactional context. Using @Transactional
on your service method may resolve this.
4. Database Initialization Failures
Description:
If you see errors during startup relating to initializing the database schema, it could be due to an incorrect schema or data script.
Solution:
Make sure the path to your schema.sql and data.sql is correct. Add the following to your application properties:
spring.datasource.initialization-mode=always
spring.datasource.schema=classpath:schema.sql
spring.datasource.data=classpath:data.sql
Why: This configuration ensures that Spring Boot is set to initialize the database each time the application runs, sourcing scripts from your classpath.
5. Configuration Property Issues
Description:
Incorrectly configuring properties like username and password can lead to authentication failures.
Solution:
Ensure that you have defined the user in your application.properties
, as follows:
spring.datasource.username=sa
spring.datasource.password=
Why: H2 uses the sa
username with no password by default. Wrong credentials may lead to failed connections.
6. Case Sensitivity Issues
Description:
H2 is case-sensitive by default when dealing with table and column names. This could lead to Table not found
errors if names are referenced inaccurately.
Solution:
You can create tables with double quotes:
CREATE TABLE "User" (id INT PRIMARY KEY, name VARCHAR(255));
Why: Using double quotes allows H2 to treat table names as case-sensitive, adhering to their exact naming.
7. Incompatibility with Production Environment
Description:
Using H2 for development can lead to compatibility issues when deploying to production databases (e.g., MySQL, PostgreSQL) due to variance in SQL dialects.
Solution:
Employ profiles in Spring Boot to switch between H2 and your production database. For example, use:
# application-dev.properties for H2
spring.datasource.url=jdbc:h2:mem:testdb
# application-prod.properties for MySQL
spring.datasource.url=jdbc:mysql://localhost:3306/proddb
Why: Profiles help you manage different environments effectively, ensuring your development database resembles the production database as closely as possible.
8. Performance Issues for Large Queries
Description:
While H2 performs well for small data sets, performance may deteriorate with large queries due to its in-memory nature.
Solution:
Use indexing to speed up query performance if you must handle more data:
CREATE INDEX idx_name ON User(name);
Why: Indexes can dramatically improve query performance by enabling faster lookups, especially with large data sets.
Final Considerations
H2 is a great choice for many Spring Boot projects, particularly during development. However, understanding its quirks and limitations can save you from headaches down the line. By proactively addressing connection issues, data persistence, and compatibility challenges, you can harness H2 effectively.
For more details on managing databases with Spring Boot, check out Spring Data JPA Documentation.
Armed with the knowledge of common H2 issues and solutions, you can now confidently develop your next Spring Boot application!
Additional Resources
Feel free to leave comments and let us know your experiences with H2 in Spring Boot. Happy coding!
Checkout our other articles