Why You Should Always Parameterize Your Database Queries
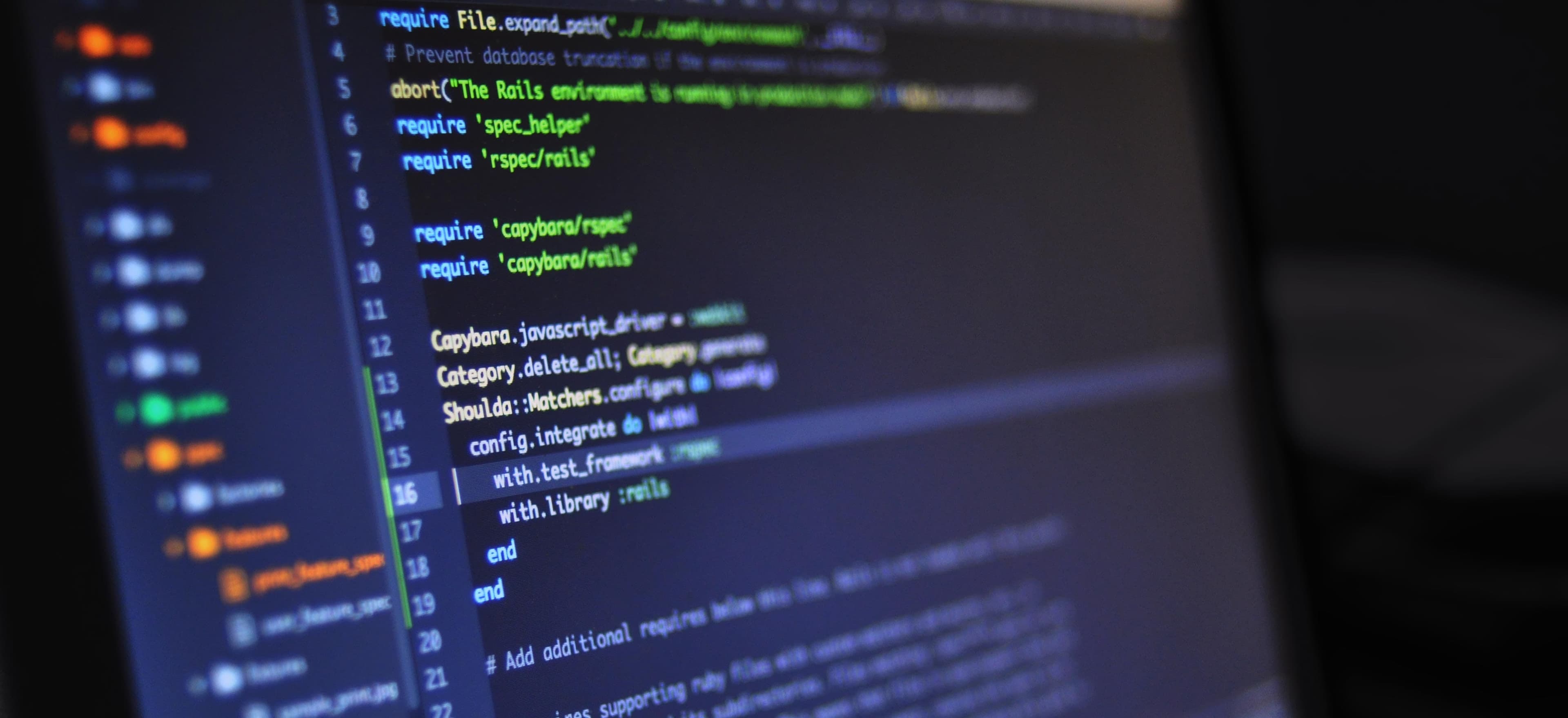
- Published on
Why You Should Always Parameterize Your Database Queries
As developers, we often find ourselves interacting with databases. Whether you’re building a simple application or an enterprise-level system, database queries are a foundational part of your coding experience. Despite this relevance, one critical element often overlooked is how these queries are constructed and executed. The method you choose can substantially affect your application's security, performance, and maintainability.
In this blog post, we will explore the importance of parameterizing your database queries, its benefits, and best practices when implementing this approach in Java.
What Does Parameterization Mean?
Parameterization, also known as prepared statements, is a programming technique used to prepare a SQL statement before it is executed. Instead of embedding user input directly into your SQL statements, which can lead to security vulnerabilities, parameterized queries allow you to define SQL queries with placeholders for parameters.
This means that the database engine can execute the query without the risk of SQL injection, ensuring that user input is treated as a value rather than executable SQL code.
Example of a Vulnerable Query
To understand the problem more clearly, let’s examine an example of a non-parameterized query that is vulnerable to SQL injection.
String userInput = request.getParameter("username");
String query = "SELECT * FROM users WHERE username = '" + userInput + "'";
Statement stmt = connection.createStatement();
ResultSet rs = stmt.executeQuery(query);
In this code snippet, if an attacker enters a specially-crafted username, they could manipulate the SQL query. For example, inputting admin' OR '1'='1
would yield a query that returns all users, potentially compromising the database.
The Solution: Parameterized Queries
Let’s rewrite the above SQL statement using a parameterized query.
String userInput = request.getParameter("username");
String query = "SELECT * FROM users WHERE username = ?";
PreparedStatement pstmt = connection.prepareStatement(query);
pstmt.setString(1, userInput);
ResultSet rs = pstmt.executeQuery();
Advantages of Parameterization
Parameterization comes with a myriad of advantages. Here’s a look at some of the most significant benefits:
1. Prevention of SQL Injection Attacks
The foremost advantage of using parameterized queries is protection against SQL injection. Parameterization ensures that user input cannot alter the intended SQL command, thus defending your application from one of the most common vulnerabilities.
2. Improved Performance
When a SQL query is parameterized, the database can compile and optimize the execution plan once and reuse it multiple times, as the structure of the query remains the same. This can lead to performance improvements, especially in applications that execute the same queries repeatedly with different parameters.
3. Enhanced Readability and Maintainability
Using a parameterized approach makes your code clearer and easier to manage. Developers can quickly ascertain which parts of the query are static and which are variable. This clarity leads to easier debugging and maintenance.
// Example of a readable, parameterized query
String query = "DELETE FROM users WHERE user_id = ?";
PreparedStatement pstmt = connection.prepareStatement(query);
pstmt.setInt(1, userId);
pstmt.executeUpdate();
Best Practices for Parameterization
To ensure that you implement parameterization effectively, consider the following best practices:
1. Always Use Prepared Statements
Regardless of the database technology you are using, adopt a habit of always utilizing prepared statements whenever performing database operations.
2. Validate User Input
While parameterization helps sanitize user input, it's still essential to validate it. Always check for the expected format, length, or type of input.
3. Leverage ORM Frameworks
Object-Relational Mapping (ORM) frameworks like Hibernate or JPA simplify database interactions and often provide built-in support for parameterized queries, reducing the chances of mistakes.
4. Monitor and Audit SQL Execution
Regularly reviewing the SQL queries being executed can provide insights into potential vulnerabilities or performance bottlenecks.
Real-World Implementation in Java
To solidify our understanding, let’s take a deeper look at how parameterization works in a real-world Java application.
Setting Up the JDBC Connection
First, establish a JDBC connection to your database.
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
public class DatabaseExample {
private final String DB_URL = "jdbc:mysql://localhost:3306/your_database";
private final String USER = "root";
private final String PASS = "password";
public Connection connect() throws Exception {
return DriverManager.getConnection(DB_URL, USER, PASS);
}
}
In this snippet, we initialize a database connection using JDBC. Ensure to handle your credentials securely, perhaps using environment variables or a secrets manager.
Executing a Safely Parameterized Query
Next, let’s write a function to fetch user details by username safely.
public User getUserByUsername(String username) {
String query = "SELECT * FROM users WHERE username = ?";
User user = null;
try (Connection conn = connect();
PreparedStatement pstmt = conn.prepareStatement(query)) {
pstmt.setString(1, username);
ResultSet rs = pstmt.executeQuery();
if (rs.next()) {
user = new User(rs.getInt("user_id"), rs.getString("username"), rs.getString("email"));
}
} catch (Exception e) {
e.printStackTrace();
}
return user;
}
Why This Works
In this implementation, we systematically:
- Use a prepared statement to avoid SQL injection.
- Safely retrieve results using
ResultSet
. - Handle exceptions properly to avoid application crashes.
To Wrap Things Up
Parameterizing your database queries is fundamental to secure and efficient coding practices in Java. The potential risks posed by SQL injection are significant, and they can lead to data breaches, loss of user trust, and financial implications. By adopting prepared statements, you not only increase the security of your applications but also enhance their performance and maintainability.
If you're looking to delve deeper into what makes Java a powerful language for database management, check out Java Database Connectivity (JDBC) and explore the advantages of using Hibernate for your ORM needs.
Always remember: the right coding practices can save you from significant headaches down the road. Happy coding!
Checkout our other articles