Streamline Your List: Common Pitfalls in Java Streams
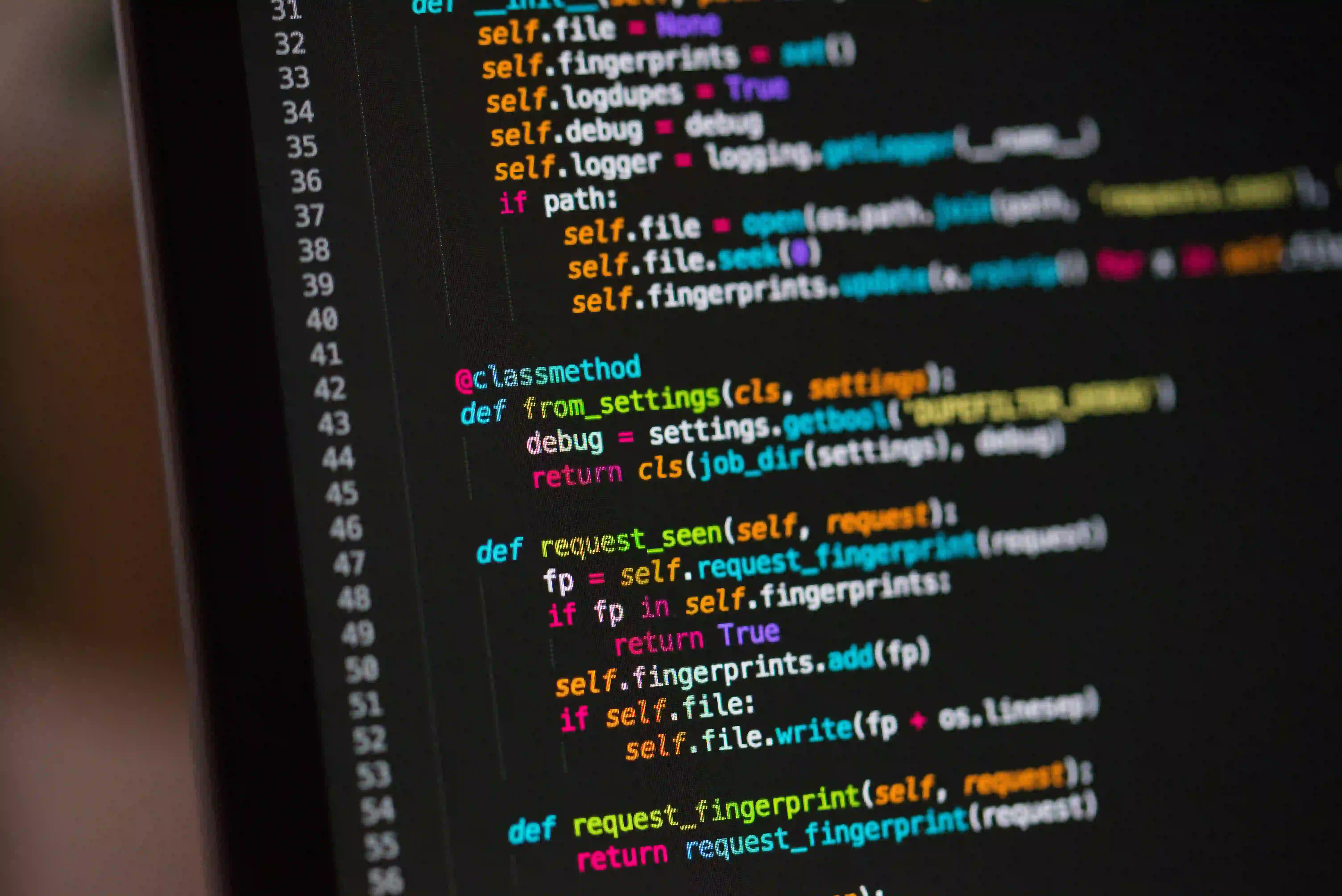
Streamline Your List: Common Pitfalls in Java Streams
Java Streams provide a powerful way to process collections of data in a functional style. Introduced in Java 8, they allow developers to run operations on collections in a concise, readable manner. However, when misused, Streams can lead to performance pitfalls and confusing code. In this article, we’ll explore some common pitfalls when using Java Streams and how to avoid them.
What are Java Streams?
Before delving into the pitfalls, let’s define what Java Streams are. A Stream is a sequence of elements supporting sequential and parallel aggregate operations. Think of it as a pipeline that processes data in a way that is more fluent than traditional loops. Java Streams allow you to filter, map, reduce, and collect elements easily.
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
// Example of using Stream to filter names starting with 'A'
List<String> aNames = names.stream()
.filter(name -> name.startsWith("A"))
.collect(Collectors.toList());
System.out.println(aNames); // Output: [Alice]
In the code above, we begin by defining a list of names. We then convert this list into a Stream, filter for names starting with 'A', and collect the results into a new list. It’s concise and expressive!
Common Pitfalls in Java Streams
1. Not Understanding Intermediate vs. Terminal Operations
One of the most common pitfalls is not understanding the difference between intermediate and terminal operations. Intermediate operations (like filter
, map
) are lazy, meaning they don’t do anything until a terminal operation is invoked.
Example:
List<String> filteredNames = names.stream()
.filter(name -> name.length() > 3); // This does nothing yet!
// The terminal operation is required to execute the filtering.
long count = filteredNames.count();
Here, the filter
operation is not executed until count()
is called. If you don’t call a terminal operation, no processing happens at all.
2. Using collect()
Incorrectly
The collect()
method is used to gather the results of the stream into a collection. However, using it incorrectly can lead to unintended consequences.
Example:
List<String> resultList = names.stream()
.filter(name -> name.length() > 3)
.collect(Collectors.toSet()); // Converts to a Set
System.out.println(resultList.get(0)); // Throws error due to Set not supporting indexed access.
In the example above, using collect(Collectors.toSet())
eliminates duplicates but does not maintain insertion order. If you require a specific order, prefer using collect(Collectors.toList())
.
3. Creating Multiple Streams from a Collection
Another frequent mistake is creating multiple Streams from a single collection which can lead to unexpected results, especially if the collection is modified in parallel processes.
Example:
Stream<String> stream1 = names.stream();
Stream<String> stream2 = names.stream();
stream1.forEach(System.out::println); // Works as intended
stream2.forEach(System.out::println); // Works but unexpected results if names were modified in between.
Once one Stream has been consumed, that collection cannot be reused to create another Stream without resetting its state. To avoid this, consider storing the result in a new collection after processing.
4. Not Handling Null Values
Null values can lead to NullPointerException
when using Streams, and failing to account for them is a common oversight.
Example:
List<String> mixedNames = Arrays.asList("Alice", null, "Charlie");
// If you try to filter directly, this could throw an exception.
List<String> filteredNames = mixedNames.stream()
.filter(name -> name.length() > 3) // Throws NPE
.collect(Collectors.toList());
To avoid this, include a null check in your predicates.
List<String> filteredNames = mixedNames.stream()
.filter(name -> name != null && name.length() > 3)
.collect(Collectors.toList());
5. Excessive Use of Parallel Streams
Parallel Streams can provide significant performance benefits for large datasets, but they can also introduce complexity and performance degradation if not used correctly.
List<Integer> numbers = IntStream.rangeClosed(1, 1000).boxed().collect(Collectors.toList());
List<Integer> evenNumbers = numbers.parallelStream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
While this may work well for large lists, the overhead of managing multiple threads can make it inefficient for smaller ones. Always measure the performance before opting for parallelism.
Best Practices for Using Java Streams
To ensure you maximize performance and code clarity, here are some best practices to adopt:
- Understand the Operations: Know when operations are lazy vs. eager.
- Prefer
collect(Collectors.toList())
for Ordered Collections: It’s safer when ordering matters. - Reuse Collections Carefully: Stream instances should not be reused after terminal operations.
- Check for Nulls: Always handle null values to prevent exceptions.
- Consider Data Size: Use parallel streams wisely—performance gains depend on the dataset more than the logic.
In Conclusion, Here is What Matters
Java Streams can greatly enhance your ability to process collections efficiently. By understanding common pitfalls, you can leverage Streams effectively without falling into the traps that many developers encounter. Remember to keep the principles of stream usage in mind while writing clean, maintainable code.
For more in-depth learning on Java Streams, consider checking out the Java 8 Streams documentation and Effective Java for best practices.
By maintaining awareness of these common pitfalls and applying the best practices discussed, you can become proficient in Java Streams and streamline your data processing tasks. Happy coding!