Mastering If-Else Statements: Common Pitfalls to Avoid
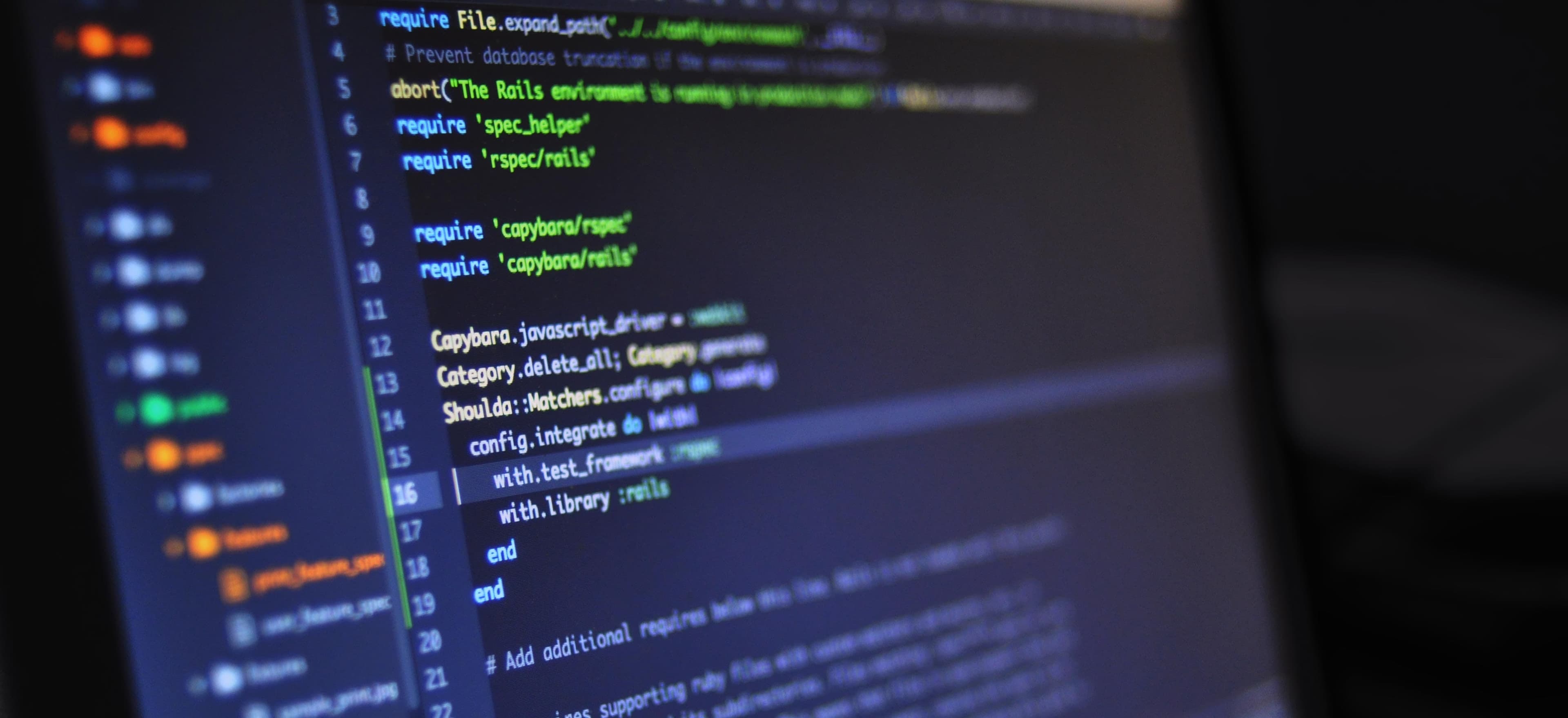
- Published on
Mastering If-Else Statements: Common Pitfalls to Avoid
Java is a robust programming language extensively used across various domains. One of the fundamental control structures in Java—and in programming in general—is the if-else statement. While simple in concept, improper use can lead to bugs and inefficient code. In this blog post, we will explore the nuances of if-else statements in Java, discuss common pitfalls to avoid, and provide clear examples to ensure your code remains clean and efficient.
Understanding If-Else Statements
At its core, the if-else statement allows a program to execute different pieces of code based on certain conditions. The syntax is straightforward:
if (condition) {
// code to execute if the condition is true
} else {
// code to execute if the condition is false
}
Example of a Basic If-Else Statement
int number = 10;
if (number > 5) {
System.out.println("Number is greater than 5");
} else {
System.out.println("Number is 5 or less");
}
In this example, the code checks if number
is greater than 5. If it is, the first block executes, otherwise the second block runs. While this appears simple, there are pitfalls that can affect performance and readability.
Common Pitfalls to Avoid
1. Overusing Nested If-Else Statements
While nesting if-else statements can provide powerful control flows, overuse can lead to a significant decrease in code readability.
Problem
A deeply nested structure can make it challenging to understand the flow of logic. Consider the following:
if (a > 0) {
if (b > 0) {
if (c > 0) {
System.out.println("All variables are positive");
}
}
}
Solution
Use logical operators to create compound conditions. This flattens the structure and enhances readability:
if (a > 0 && b > 0 && c > 0) {
System.out.println("All variables are positive");
}
2. Forgetting the Default Case
Failing to account for all possible conditions can lead to unexpected behavior.
Problem
Consider a situation in which you are checking a value but forgot an else statement:
switch (day) {
case 1:
System.out.println("Monday");
break;
case 2:
System.out.println("Tuesday");
break;
// Missing else for other days
}
Solution
Always provide a default case to handle unexpected values:
switch (day) {
case 1:
System.out.println("Monday");
break;
case 2:
System.out.println("Tuesday");
break;
default:
System.out.println("Invalid day");
}
3. Using Multiple Else If Statements Inefficiently
While sometimes necessary, long chains of else if statements can become cumbersome.
Problem
Consider the following code that checks multiple ranges:
if (score >= 90) {
System.out.println("Grade: A");
} else if (score >= 80) {
System.out.println("Grade: B");
} else if (score >= 70) {
System.out.println("Grade: C");
} else if (score >= 60) {
System.out.println("Grade: D");
} else {
System.out.println("Grade: F");
}
Solution
In this scenario, using a switch
statement or a method can enhance clarity and maintainability:
String grade;
if (score >= 90) {
grade = "A";
} else if (score >= 80) {
grade = "B";
} else if (score >= 70) {
grade = "C";
} else if (score >= 60) {
grade = "D";
} else {
grade = "F";
}
System.out.println("Grade: " + grade);
4. Neglecting Boolean Expressions and Logical Operators
Java supports complex boolean expressions, but often developers neglect using them effectively.
Problem
Using multiple nested conditions instead of leveraging &&
and ||
can complicate the code:
if (isWeekend) {
if (isRaining) {
System.out.println("Stay indoors");
}
}
Solution
Utilize logical operators for a cleaner condition:
if (isWeekend && isRaining) {
System.out.println("Stay indoors");
}
5. Inefficient Comparisons
Comparing objects using ‘==’ operator instead of using .equals()
can lead to bugs, especially with string comparisons.
Problem
Incorrect comparison can yield false results:
String str1 = new String("Hello");
String str2 = new String("Hello");
if (str1 == str2) {
System.out.println("Strings are equal");
} else {
System.out.println("Strings are NOT equal");
}
Solution
Always use .equals()
for comparing string content:
if (str1.equals(str2)) {
System.out.println("Strings are equal");
} else {
System.out.println("Strings are NOT equal");
}
6. Neglecting to Include Break Statements in Switch Cases
A common issue when using switch statements is forgetting to include break statements.
Problem
Omitting break statements can lead to "fall-through," where multiple cases execute unexpectedly.
switch (fruit) {
case "Apple":
System.out.println("It's an apple");
case "Banana":
System.out.println("It's a banana");
break;
}
Solution
Always include break statements unless fall-through is intentionally desired:
switch (fruit) {
case "Apple":
System.out.println("It's an apple");
break;
case "Banana":
System.out.println("It's a banana");
break;
}
The Last Word
Mastering if-else statements is an essential skill for Java developers. While they provide a robust means to control the flow of a program, their misuse can lead to inefficient, hard-to-maintain code. By avoiding the common pitfalls discussed in this article, including overusing nested statements, neglecting default cases, and not using correct comparison methods, you can ensure your Java code remains both functional and readable.
For further reading on Java programming, consider diving into the Java Documentation which offers comprehensive resources on various topics, including control structures.
Happy coding!