Common Mistakes in Java EE CDI Conversation Scope
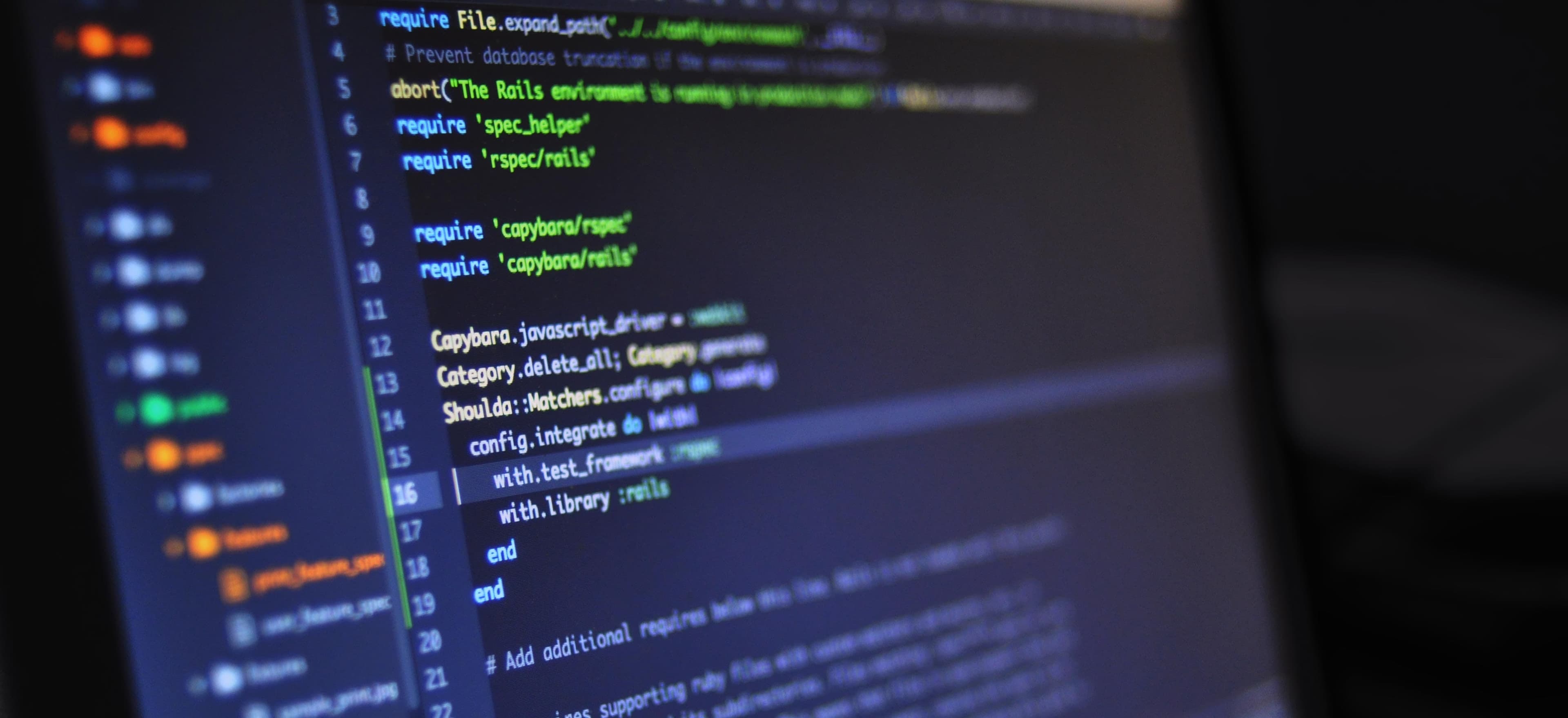
- Published on
Common Mistakes in Java EE CDI Conversation Scope
Java EE’s Context and Dependency Injection (CDI) is a powerful feature that simplifies the management of beans and their life cycles. While the concepts of scopes are fairly straightforward, developers often run into common pitfalls when working with the Conversation Scope. In this blog post, we will discuss these mistakes and provide insights on how to effectively use conversation scope in your Java EE applications.
Understanding Conversation Scope
Before diving into the common mistakes, it's essential to understand what conversation scope is in CDI. Conversation Scope allows developers to manage the lifecycle of beans based on a user's interaction. Unlike request scope (one-time use) or session scope (lifetime of the session), conversation scoped beans exist only for a specific user interaction, allowing you to store state beyond a single request but not indefinitely.
When implemented correctly, conversation scope can lead to better performance and resource management, offering a more interactive user experience. But if mismanaged, it can cause unexpected behaviors in your application, leading to bugs and difficulties in maintaining your code.
Common Mistakes
1. Not Managing the Conversation Lifecycle Properly
One of the most frequent mistakes is neglecting to start and end conversations effectively.
import javax.enterprise.context.Conversation;
import javax.inject.Inject;
import javax.enterprise.context.ConversationScoped;
@ConversationScoped
public class MyBean {
@Inject
private Conversation conversation;
public void begin() {
if (conversation.isTransient()) {
conversation.begin();
}
}
public void end() {
if (!conversation.isTransient()) {
conversation.end();
}
}
}
Why this matters: If you forget to call conversation.begin()
, your bean behaves as if it's in request scope. Consequently, the state will not persist across multiple HTTP requests.
2. Ending a Conversation Too Soon
Another common pitfall occurs when developers incorrectly end the conversation before all necessary operations are complete.
public void handleRequest() {
begin();
// Perform actions, e.g., data manipulation.
end(); // Problematic if placed here.
}
Why this matters: An early conversation end can disrupt the flow of data, causing a mismatch between user expectations and application behavior.
3. Using Conversation Scope for Long-lived State
Developers often misunderstand the intent behind conversation scope and attempt to save long-lived states. Utilizing conversation scope as a means of storing user data for an extended period is a recipe for disaster.
@ConversationScoped
public class UserSession {
private String username;
// getter and setter
}
Why this matters: The conversation scope is not designed for long-lived states. Instead, leverage session scope when you need to maintain user data over an extended interaction period.
4. Failing to Clean Up Conversations
Conversations should always be properly managed, including their clean-up. Failing to manually manage the ending of conversations can lead to memory leaks.
public void completeTransaction() {
try {
// Transaction logic
} finally {
end(); // Make sure this gets called to prevent leaving the conversation open.
}
}
Why this matters: Not ending a conversation can lead to stale state information and consume resources unnecessarily.
5. Forgetting to Utilize @ConversationScoped
Properly
Sometimes developers overlook annotating beans appropriately or misplace annotations in the class hierarchy.
@ConversationScoped
public class SomeService {
// ...
}
Why this matters: Not specifying the @ConversationScoped
will default the behavior to request or application scope, negating your intention to maintain state.
6. Confusing the Context Injection
In some cases, developers might confuse the usage of @Inject
with @Produces
. Understanding the difference can prevent misbehavior:
@ConversationScoped
public class OrderBean {
@Inject
private Conversation conversation; // This is correct.
@Produces
@ConversationScoped
public AnotherBean produceAnotherBean() {
return new AnotherBean();
}
}
Why this matters: Misunderstanding these annotations can result in incorrect bean management, leading to unexpected behaviors during the application's execution.
7. Not Using Decorators or Interceptors
Another common mistake is failing to take advantage of decorators or interceptors for managing conversation scope behavior.
@Interceptor
@ConversationScoped
public class LoggingInterceptor {
// Implement logging behavior during the conversation
}
Why this matters: Decorators and interceptors can help manage state transitions, making your implementations cleaner and more modular.
Best Practices for Working with Conversation Scope
Begin the Conversation Early
Aim to begin the conversation at the earliest user interaction. This ensures that the state is maintained from the outset.
Always Validate Conversation Status
Before starting or ending a conversation, always validate its current state. This ensures you do not inadvertently alter its lifecycle.
Encapsulate Business Logic
Keep business logic encapsulated within the boundaries of your conversation-scoped beans. Expose only what is necessary to maintain clean separation and readability.
Utilize @Transactional with Conversation Scope
When working with conversation-scoped beans, managing transactions effectively with the @Transactional
annotation can streamline operations during a conversation.
Employ CDI Events
By employing CDI events, you can help manage conversations in a more dynamic way, allowing for better state transitions and management.
@Inject
private Event<SomeEvent> event;
public void completeOrder() {
event.fire(new SomeEvent(orderDetails));
end(); // End conversation here.
}
Wrapping Up
Understanding the nuances of conversation scope is vital for successful Java EE applications. While it brings about the potential for smoother, stateful interactions, mismanagement can lead to a tangled web of problems.
Awareness and forethought about the lifecycle and intended usage of conversation scoped beans will empower developers to leverage this powerful structure effectively. By avoiding the common pitfalls discussed in this post and adopting best practices, you can enhance your application's maintainability and overall user experience.
For more resources on Java EE and CDI, you can check out the official Java EE documentation.
Remember, being mindful of your scope management can make a significant difference in your Java EE applications. Happy coding!
Checkout our other articles